ontology nlp
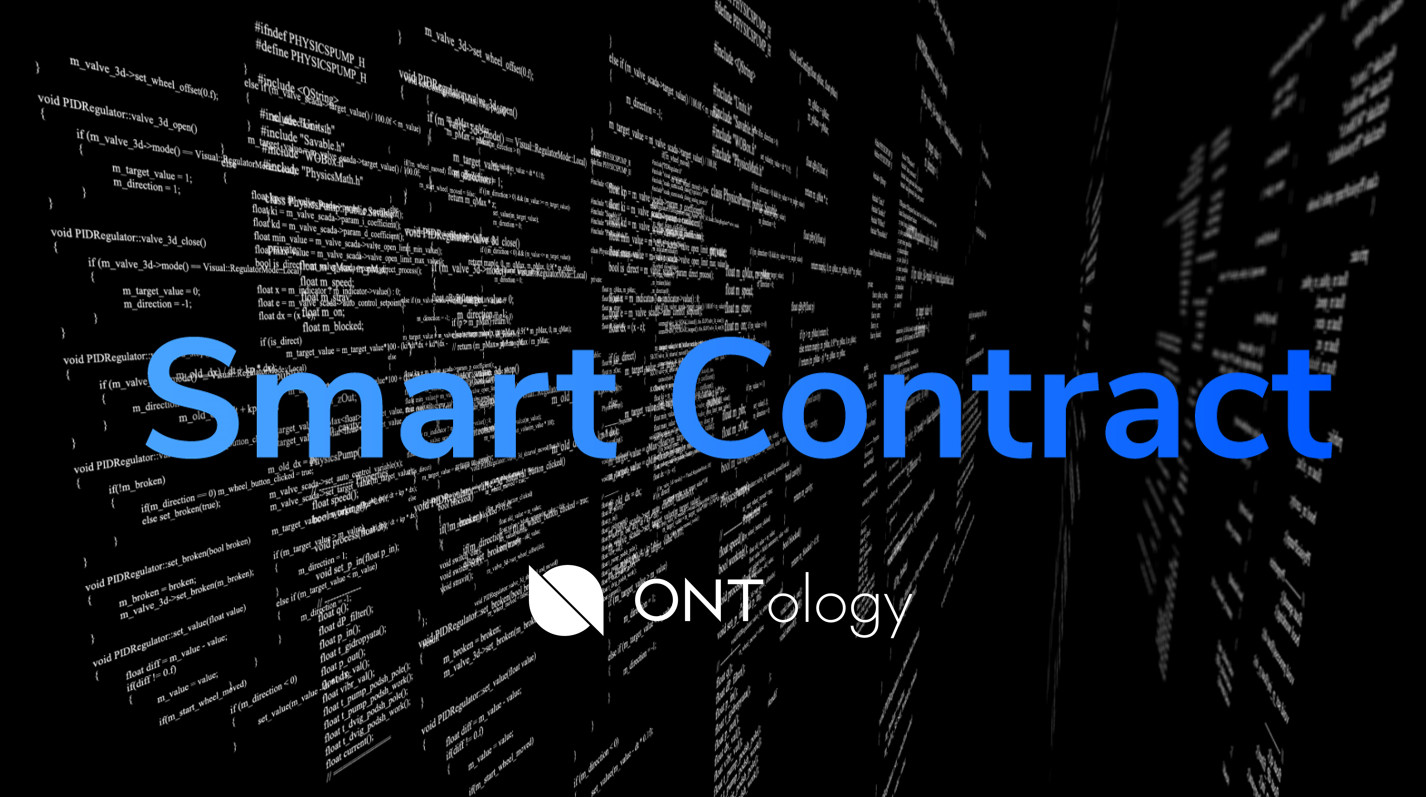
Earlier, I have introduced the Ontology Smart Contract in
之前,我在
Part 1: Blockchain & Block API and 第1部分:区块链和区块API和 Part 2: Storage API 第2部分:存储API Part 3: Runtime API 第3部分:运行时APIToday, let’s talk about how to invoke an Ontology native smart contract through the
今天,让我们谈谈如何通过
本机API (Native API)
. One of the most typical functions of invoking native contract is asset transfer.
。 调用本地合同的最典型功能之一是资产转移。
介绍 (Introduction)
The Native API has only one API. When you use the Invoke function, you need to use the built-in state function to help you encapsulate the parameters. See below to learn how to use the functions.
本机API只有一个API。 使用Invoke函数时,需要使用内置的状态函数来帮助您封装参数。 请参阅下文以了解如何使用这些功能。
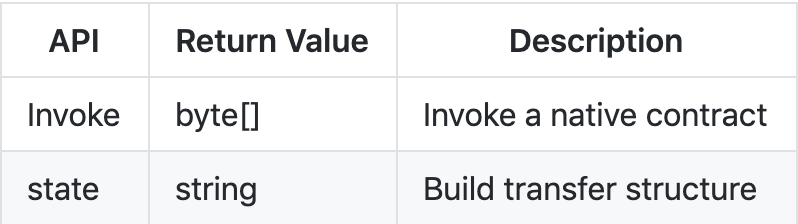
Now let’s go into more detail about how to use these 2 APIs. Before that, you can create a new contract in the Ontology smart contract development tool SmartX and follow the instructions below. As usual, at the end of the article, I will provide a link to the source code.
现在,让我们更详细地介绍如何使用这两个API。 在此之前,您可以在本体智能合约开发工具SmartX中创建新合约,并按照以下说明进行操作。 像往常一样,在文章结尾,我将提供到源代码的链接。
如何使用本机API (How to Use Native API)
As usual, you need to import the 2 functions as follows before using them.
与往常一样,在使用这两个功能之前,您需要按以下步骤导入它们。
from ontology.interop.Ontology.Native import Invoke
from ontology.builtins import state
本体本地合同清单 (List of Ontology Native Contract)
There are currently 6 Ontology native contracts available for developers. Below is the list of the 6 native contracts that can be invoked by the Native API.
当前,有6个本体本机合同可供开发人员使用。 以下是可以由本机API调用的6种本机合约的列表。
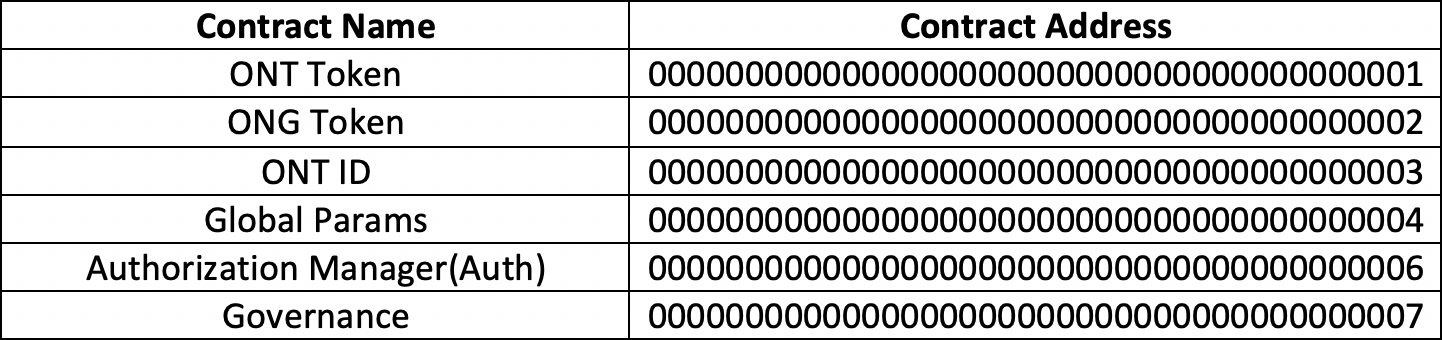
In the contract, you only need to convert the contract address into a bytearray format and invoke it.
在合同中,只需要将合同地址转换为字节数组格式并调用它。
例如 (For example)
, when you need to call an ONT Token contract, you can first convert the address of the ONT Token contract into bytearray format, and then call the Invoke function. When calling the Invoke function, the imported parameters are the version number, the contract address, the contract method invoked, and the transfer-related parameters encapsulated by the state function.
,当您需要调用ONT令牌合约时,可以先将ONT令牌合约的地址转换为字节数组格式,然后调用Invoke函数。 调用Invoke函数时,导入的参数是版本号,合同地址,调用的合同方法以及由state函数封装的与传输相关的参数。
One particular point to note here is that when making a contract transfer for ONG, the quantity filled is 10⁹ times the actual quantity. That is, if you need to transfer 10 ONG, the quantity needs to be filled in is 10¹⁰. When using a wallet, such as ONTO or Cyano to transfer, the quantity you enter is the transfer amount.
这里需要特别注意的一点是,在进行ONG的合同转让时,填写的数量是实际数量的10倍。 也就是说,如果您需要转移10 ONG,则需要填写的数量为10⁰。 当使用诸如ONTO或Cyano之类的钱包进行转账时,您输入的数量就是转账金额。
contract_address_ONT = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01')
param = state(from_acct, to_acct, ont_amount)
res = Invoke(1, contract_address_ONT, 'transfer', [param])
转让合同代码 (Transfer Contract Code)
Below we give a detailed example of how to use Python to
下面我们给出一个详细的示例,说明如何使用Python来
转移ONT和ONG (transfer ONT and ONG)
. In the code below, the parameter type of the imported sending account and receiving address is a string. In addition, it can also be delivered with an account parameter type of address, thereby saving the Gas fee for invoking the contract. The process is as follows:
。 在下面的代码中,导入的发送帐户和接收地址的参数类型是一个字符串。 此外,它还可以使用帐户参数类型的地址进行交付,从而节省了调用合同的Gas费用。 流程如下:
- Define the contract address variables contract_address_ONT and contract_address_ONG; 定义合同地址变量contract_address_ONT和contract_address_ONG;
- Convert the sending and receiving address from base58 format to bytearray format; 将发送和接收地址从base58格式转换为bytearray格式;
- Verify the signature and confirm that the sending address is the same as the contract invoke address; 验证签名并确认发送地址与合同调用地址相同;
- The state function encapsulates the transfer related parameters; 状态函数封装了与传输相关的参数;
- The Invoke function calls the ONT Token and ONG Token native contract for transfer; Invoke函数调用ONT令牌和ONG令牌本机合约进行传输;
- Determine whether the transfer was successful by returning res. If the return value is b’\x01', then the transfer is successful and the event “transfer succeed” will be pushed out. 通过返回res确定传输是否成功。 如果返回值为b'\ x01',则传输成功,并且将推出“传输成功”事件。
from ontology.interop.System.Runtime import Notify, CheckWitness
from ontology.interop.Ontology.Runtime import Base58ToAddress
from ontology.interop.Ontology.Native import Invoke
from ontology.builtins import state
# contract address
contract_address_ONT = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x01')
contract_address_ONG = bytearray(b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x02')
def Main(operation, args):
if operation == 'transfer':
from_acct = args[0]
to_acct = args[1]
ont_amount = args[2]
ong_amount = args[3]
return transfer(from_acct,to_acct,ont_amount,ong_amount)
return False
def transfer(from_acct, to_acct, ont_amount, ong_amount):
# convert base58 address to address in the form of byte array
from_acct=Base58ToAddress(from_acct)
to_acct=Base58ToAddress(to_acct)
# check whether the sender is the payer
if CheckWitness(from_acct):
# transfer ONT
if ont_amount > 0:
param = state(from_acct, to_acct, ont_amount)
res = Invoke(1, contract_address_ONT, 'transfer', [param])
if res and res == b'\x01':
Notify('transfer succeeded')
else:
Notify('transfer failed')
# transfer ONG
if ong_amount > 0:
param = state(from_acct, to_acct, ong_amount)
res = Invoke(1, contract_address_ONG, 'transfer', [param])
if res and res == b'\x01':
Notify('transfer succeeded')
else:
Notify('transfer failed')
else:
Notify('CheckWitness failed')
在SmartX上练习 (Practice on SmartX)
You can also compile and run the code sample above on
您还可以编译并运行上面的代码示例
SmarX (SmarX )
following the steps below:
请按照以下步骤操作:
编制合同。 (Compile the contract.)
First, create a project on SmartX and compile the code in this project.
首先,在SmartX上创建一个项目,然后编译该项目中的代码。
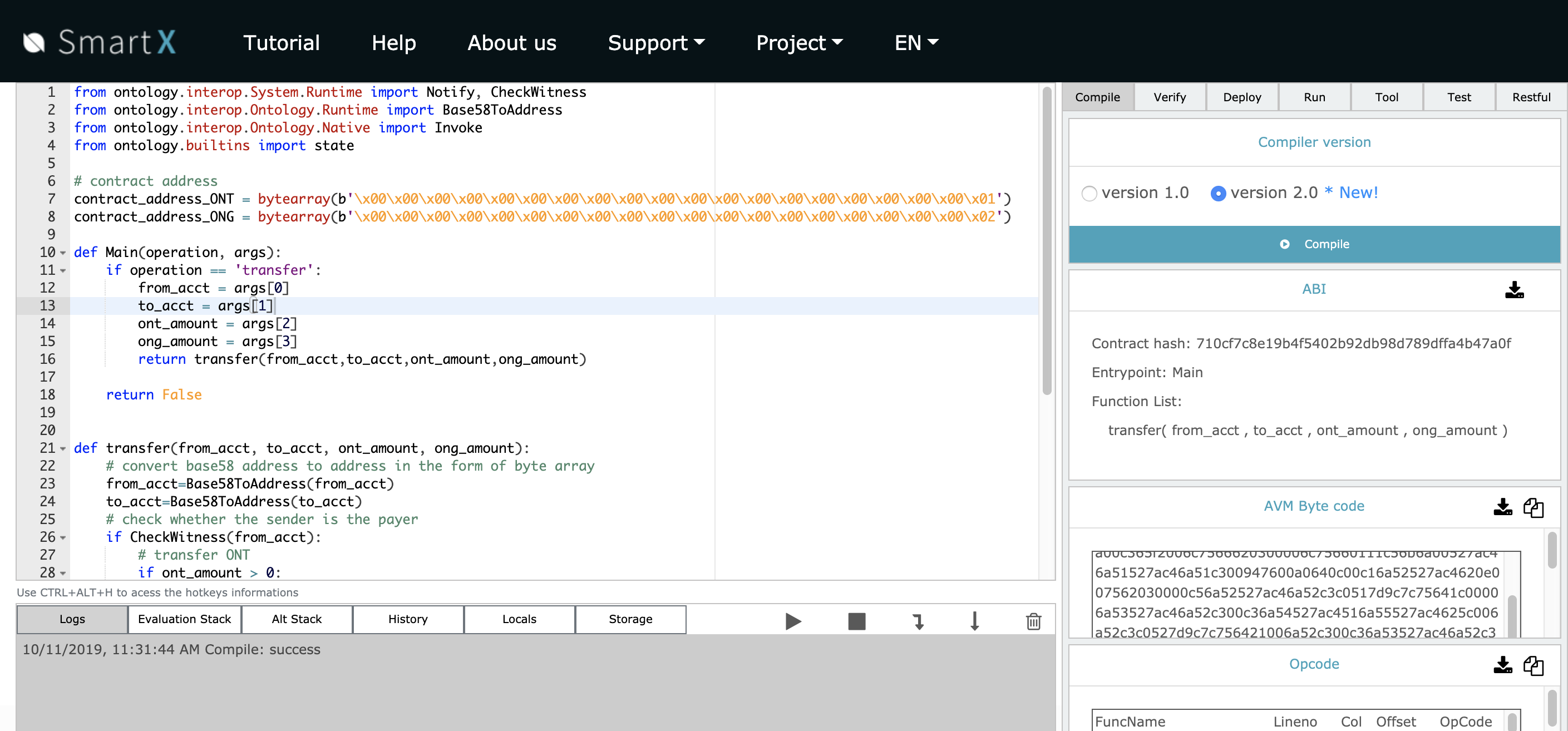
部署合同。 (Deploy the contract. )
If you need a test token to deploy the contract, apply
如果您需要测试令牌来部署合同,请申请
here. The deployment result is as follows:在这里 。 部署结果如下:

运行传递函数。 (Run the transfer function.)
Before running the transfer function, you need to configure the parameters as required. In the example below, you need to input the sending address, receiving address, and transferred ONT and ONG amount:
在运行传递函数之前,需要根据需要配置参数。 在下面的示例中,您需要输入发送地址,接收地址以及转移的ONT和ONG金额:
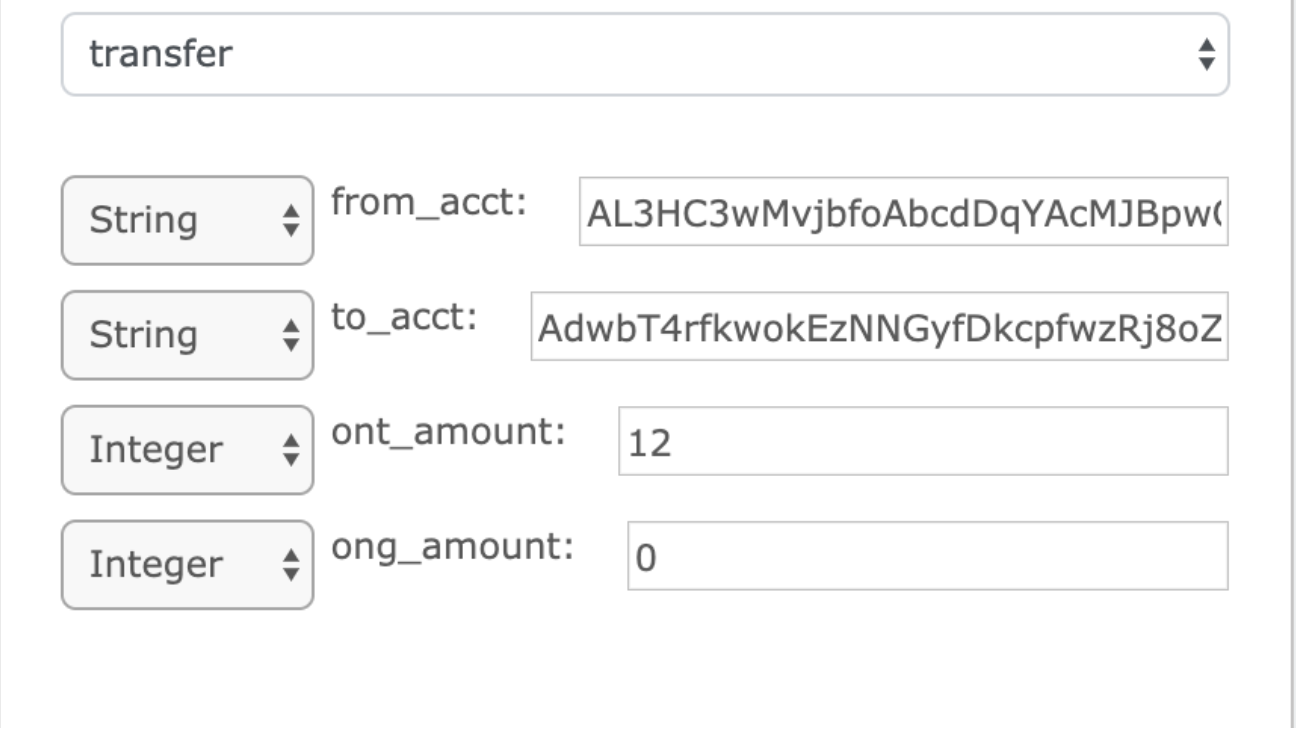
转移成功。 (Transfer succeeded.)
After you have configured the parameters properly, the transfer will be successful when running the transfer function. The transferred token will be displayed in the receiving address above:
正确配置参数后,运行传输功能时传输将成功。 转移的令牌将显示在上方的接收地址中:
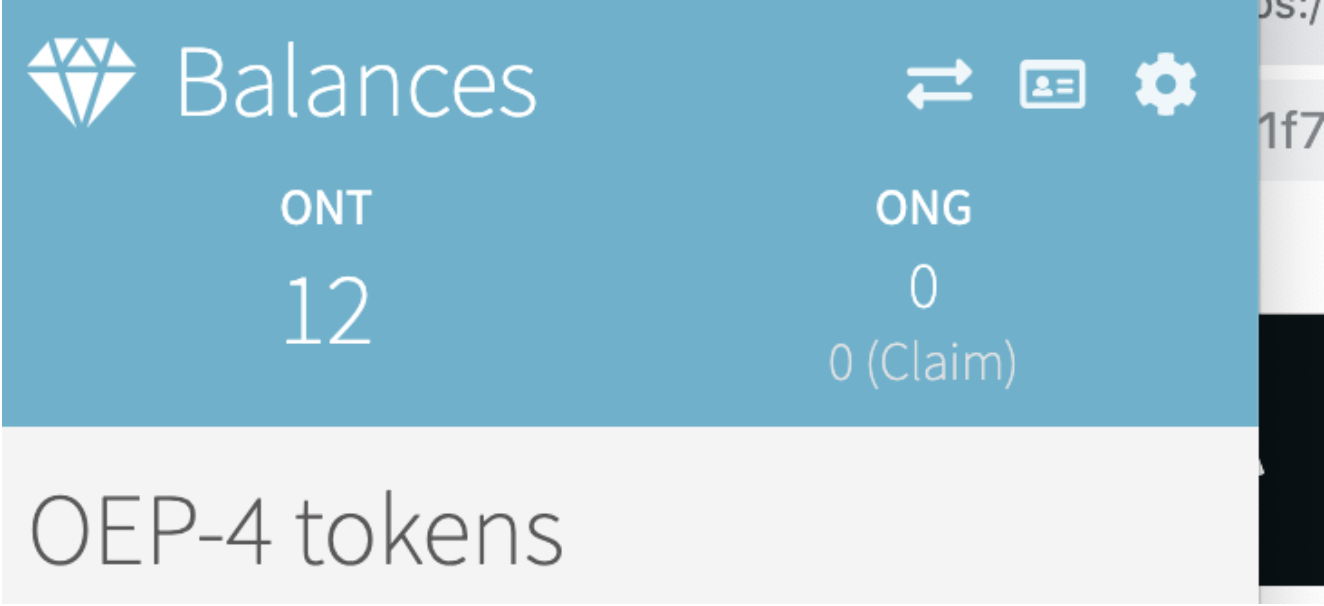
摘要 (Summary)
In this article, we introduced the
在本文中,我们介绍了
本机API ( Native API)
of the Ontology blockchain. Developers can use this API to invoke Ontology native contracts. In the next article, we will introduce the
本体区块链 开发人员可以使用此API调用本体本机合同。 在下一篇文章中,我们将介绍
升级API (Upgrade API)
to explore how to upgrade contract in Ontology smart contracts.
探索如何在本体智能合约中升级合约。
Find the tutorial on GitHub here.
在此处找到GitHub上的教程。
Medium blog Medium博客上发布的官方教程Discord. Also, take a look at the Discord上的我们的技术社区。 另外,请访问我们网站上的 Developer Center on our website, there you can find developer tools, documentation, and more. 开发人员中心,在那里您可以找到开发人员工具,文档等。
在其他地方找到本体 (Find Ontology elsewhere)
Ontology website 本体网站 GitHub / GitHub / Discord Discord Twitter / Twitter / Reddit Redditontology nlp