ontology nlp
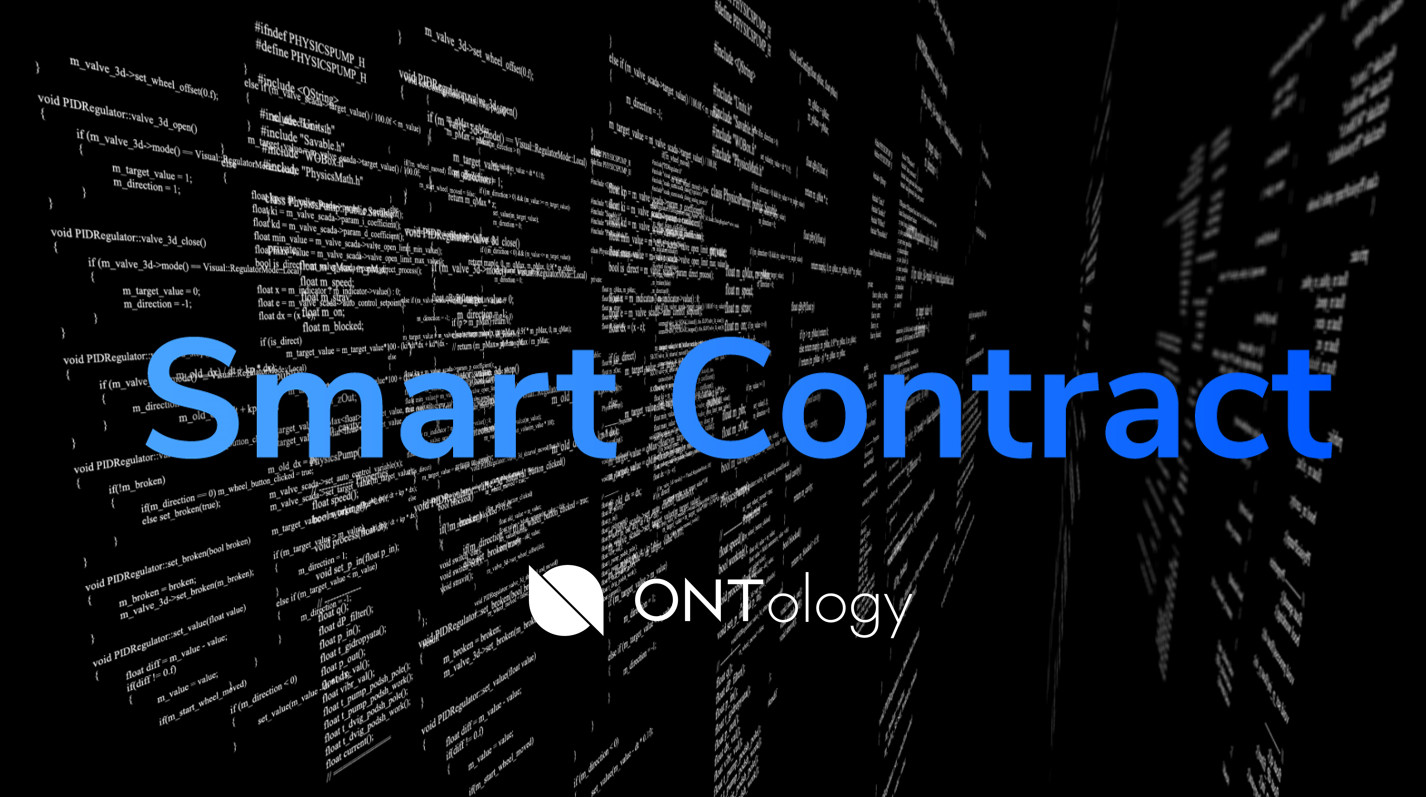
Medium blog Medium博客上发布的官方教程Excited to publish it for Habr readers. Feel free to ask any related questions and suggest a better format for tutorial materials
激动地将其发布给Habr读者。 随意提出任何相关问题,并为教程材料提出更好的格式
前言 (Foreword)
In this article, we will begin to introduce the smart contract API of Ontology. The Ontology’s smart contract API is divided into 7 modules:
在本文中,我们将开始介绍本体的智能合约API。 本体的智能合约API分为7个模块:
- Part 1: Blockchain & Block API 第1部分:区块链和区块API
- Part 5: Upgrade API 第5部分:升级API
- Part 6: Execution Engine API 第6部分:执行引擎API
- Part 7: Static & Dynamic Call API 第7部分:静态和动态调用API
In this article, we will introduce
在本文中,我们将介绍
区块链和区块API (the Blockchain & Block API)
, which is the most basic part of the Ontology smart contract system. The Blockchain API supports basic blockchain query operations, such as obtaining the current block height, whereas the Block API supports basic block query operations, such as querying the number of transactions for a given block.
,这是Ontology智能合约系统最基本的部分。 Blockchain API支持基本的区块链查询操作,例如获取当前区块高度,而Block API支持基本的区块查询操作,例如查询给定区块的交易数量。
让我们开始吧! (Let’s get started!)
First, create a new contract in SmartX and then follow the instructions below.
首先,在SmartX中创建新合同,然后按照以下说明进行操作。
1.如何使用区块链API (1. How to Use Blockchain API)
References to smart contract functions are identical to Python’s references. Developers can introduce the appropriate functions as needed. For example, the following statement introduces
智能合约功能的引用与Python的引用相同。 开发人员可以根据需要引入适当的功能。 例如,以下语句引入
GetHeight (GetHeight)
, the function to get the current block height, and
,用于获取当前块高度的函数,以及
GetHeader (GetHeader)
, the function to get the block header.
,获取块头的函数。
from ontology.interop.System.Blockchain import GetHeight, GetHeader
1.1。 GetHeight (1.1. GetHeight)
You can use GetHeight to get the latest block height, as shown in the following example. In the latter example, for convenience, we will omit the Main function, but you can add it if it’s needed.
您可以使用GetHeight获取最新的块高度,如以下示例所示。 在后一个示例中,为方便起见,我们将省略Main函数,但是如果需要可以添加它。
from ontology.interop.System.Runtime import Notify
from ontology.interop.System.Blockchain import GetHeight
def Main(operation):
if operation == 'demo':
return demo()
return False
def demo():
height=GetHeight()
Notify(height) # print height
return height #return height after running the function
1.2 GetHeader (1.2 GetHeader)
You can use GetHeader to get the block header, and the parameter is the block height of a block. Here’s an example:
您可以使用GetHeader来获取块标题,该参数是块的块高度。 这是一个例子:
from ontology.interop.System.Runtime import Notify
from ontology.interop.System.Blockchain import GetHeader
def demo():
block_height=10
header=GetHeader(block_height)
Notify(header)
return header
1.3 GetTransactionByHash (1.3 GetTransactionByHash)
You can use the
您可以使用
GetTransactionByHash (GetTransactionByHash)
function to get transactions through transaction hash. The transaction hash is sent to GetTransactionByHash as parameters in bytearray format. The key to this function is how to convert a transaction hash in hex format to a transaction hash in bytearray format. This is an important step. Otherwise, you would get an error which indicates that there is no block of this block hash.
通过交易哈希获取交易的功能。 事务哈希作为字节数组格式的参数发送到GetTransactionByHash。 此功能的关键是如何将十六进制格式的事务哈希转换为字节数组格式的事务哈希。 这是重要的一步。 否则,您将得到一个错误,指示该块哈希没有块。
Let’s take the transaction hash in hex format as an example to convert it to bytearray format. The example is as follows:
让我们以十六进制格式的交易哈希为例,将其转换为字节数组格式。 示例如下:
9f270aa3a4c13c46891ff0e1a2bdb3ea0525669d414994aadf2606734d0c89c1
First, reverse the transaction hash:
首先,反转交易哈希:
c1890c4d730626dfaa9449419d662505eab3bda2e1f01f89463cc1a4a30a279
Developers can implement this step with the conversion tool
开发人员可以使用转换工具实施此步骤
十六进制数字(小尾数)<->数字 (Hex Number(little endian)<--> Number)
provided by SmartX.
由SmartX提供。
Then, convert it to bytearray format:
然后,将其转换为字节数组格式:
{0xc1,0x89,0x0c,0x4d,0x73,0x06,0x26,0xdf,0xaa,0x94,0x49,0x41,0x9d,0x66,0x25,0x05,0xea,0xb3,0xbd,0xa2,0xe1,0xf0,0x1f,0x89,0x46,0x3c,0xc1,0xa4,0xa3,0x0a,0x27,0x9f}
Developers can do this with the conversion tool
开发人员可以使用转换工具来做到这一点
字符串<->字节数组 (String <-->Byte Array)
provided by SmartX. Finally, convert the resulting bytearray to the corresponding string:
由SmartX提供。 最后,将结果字节数组转换为相应的字符串:
\xc1\x89\x0c\x4d\x73\x06\x26\xdf\xaa\x94\x49\x41\x9d\x66\x25\x05\xea\xb3\xbd\xa2\xe1\xf0\x1f\x89\x46\x3c\xc1\xa4\xa3\x0a\x27\x9f
Below is an example of the
以下是一个示例
GetTransactionByHash (GetTransactionByHash)
function that gets a transaction through a transaction hash:
通过交易哈希获取交易的函数:
from ontology.interop.System.Blockchain import GetTransactionByHash
def demo():
# tx_hash="9f270aa3a4c13c46891ff0e1a2bdb3ea0525669d414994aadf2606734d0c89c1"
tx_hash=bytearray(b"\xc1\x89\x0c\x4d\x73\x06\x26\xdf\xaa\x94\x49\x41\x9d\x66\x25\x05\xea\xb3\xbd\xa2\xe1\xf0\x1f\x89\x46\x3c\xc1\xa4\xa3\x0a\x27\x9f")
tx=GetTransactionByHash(tx_hash)
return tx
1.4 GetTransactionHeight (1.4 GetTransactionHeight)
Developers can use the
开发人员可以使用
GetTransactionHeight (GetTransactionHeight)
function to get transaction height through transaction hash. Let’s take the hash in the above example:
函数通过事务散列获取事务高度。 让我们在上面的示例中使用哈希:
from ontology.interop.System.Blockchain import GetTransactionHeight
def demo():
# tx_hash="9f270aa3a4c13c46891ff0e1a2bdb3ea0525669d414994aadf2606734d0c89c1"
tx_hash=bytearray(b"\xc1\x89\x0c\x4d\x73\x06\x26\xdf\xaa\x94\x49\x41\x9d\x66\x25\x05\xea\xb3\xbd\xa2\xe1\xf0\x1f\x89\x46\x3c\xc1\xa4\xa3\x0a\x27\x9f")
height=GetTransactionHeight(tx_hash)
return height
1.5获取合同 (1.5 GetContract)
You can use the GetContract function to get a contract through contract hash. The conversion process of the contract hash is consistent with the transaction hash conversion process mentioned above.
您可以使用GetContract函数通过合同哈希获取合同。 合约哈希的转换过程与上述交易哈希的转换过程一致。
from ontology.interop.System.Blockchain import GetContract
def demo():
# contract_hash="d81a75a5ff9b95effa91239ff0bb3232219698fa"
contract_hash=bytearray(b"\xfa\x98\x96\x21\x32\x32\xbb\xf0\x9f\x23\x91\xfa\xef\x95\x9b\xff\xa5\x75\x1a\xd8")
contract=GetContract(contract_hash)
return contract
1.6 GetBlock (1.6 GetBlock)
You can use the GetBlock function to get the block. There are two ways to get a specific block:
您可以使用GetBlock函数获取块。 有两种方法可以获取特定的块:
1. Get the block by block height:
1.按块高度获取块:
from ontology.interop.System.Blockchain import GetBlock
def demo():
block=GetBlock(1408)
return block
2. Get the block by block hash:
2.逐块获取哈希:
from ontology.interop.System.Blockchain import GetBlock
def demo():
block_hash=bytearray(b'\x16\xe0\xc5\x40\x82\x79\x77\x30\x44\xea\x66\xc8\xc4\x5d\x17\xf7\x17\x73\x92\x33\x6d\x54\xe3\x48\x46\x0b\xc3\x2f\xe2\x15\x03\xe4')
block=GetBlock(block_hash)
2如何使用Block API (2 How to Use Block API)
There are three functions available in the Block API, which are
Block API中提供了三个功能,分别是
GetTransactions,GetTransactionCount和GetTransactionByIndex (GetTransactions, GetTransactionCount, and GetTransactionByIndex)
. We will introduce them one by one.
。 我们将一一介绍。
2.1 GetTransactionCount (2.1 GetTransactionCount)
You can use the
您可以使用
GetTransactionCount (GetTransactionCount)
function to get the number of transactions for a given block.
函数获取给定区块的交易数量。
from ontology.interop.System.Blockchain import GetBlock
from ontology.interop.System.Block import GetTransactionCount
def demo():
block=GetBlock(1408)
count=GetTransactionCount(block)
return count
2.2 GetTransactions (2.2 GetTransactions)
You can use the
您可以使用
GetTransactions (GetTransactions)
function to get all the transactions in a given block.
函数获取给定区块中的所有交易。
from ontology.interop.System.Blockchain import GetBlock
from ontology.interop.System.Block import GetTransactions
def demo():
block=GetBlock(1408)
txs=GetTransactions(block)
return txs
2.3 GetTransactionByIndex (2.3 GetTransactionByIndex)
You can use the
您可以使用
GetTransactionByIndex (GetTransactionByIndex)
function to get specific transactions in a given block.
函数以获取给定区块中的特定交易。
from ontology.interop.System.Blockchain import GetBlock
from ontology.interop.System.Block import GetTransactionByIndex
def demo():
block=GetBlock(1408)
tx=GetTransactionByIndex(block,0) # index starts from 0.
return tx
Find the complete tutorial on our GitHub here.
在此处找到我们GitHub上的完整教程。
后记 (Afterword)
The Blockchain & Block API is the most indispensable part of smart contracts since you can use them to query blockchain data and block data in smart contracts. In the next few articles, we will discuss how to use other APIs to explore their interaction with the Ontology blockchain.
区块链和区块API是智能合约中必不可少的部分,因为您可以使用它们查询智能合约中的区块链数据和区块数据。 在接下来的几篇文章中,我们将讨论如何使用其他API来探索它们与Ontology区块链的交互。
Are you a developer? Make sure you have joined our tech community on Discord. Also, take a look at the Developer Center on our website, there you can find developer tools, documentation, and more.
您是开发人员吗? 确保您已加入Discord上的我们的技术社区。 另外,请访问我们网站上的开发人员中心,在那里您可以找到开发人员工具,文档等。
在其他地方找到本体 (Find Ontology elsewhere)
Ontology website 本体网站 GitHub / GitHub / Discord Discord Twitter / Twitter / Reddit / Reddit /ontology nlp