mootools
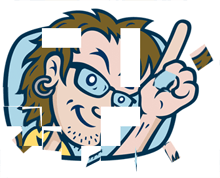
When MooTools contributor and moo4q creator Ryan Florence first showed me his outstanding CSS animation post, I was floored. His exploding text effect is an amazing example of the power of CSS3 and a dash of JavaScript. I wanted to implement this effect on my new blog redesign but with a bit more pop, so I wrote some MooTools code to take static image and make it an animated, exploding masterpiece. Let me show you how I did it, and as a bonus, I'm created a snippet of jQuery that accomplishes the same effect.
当MooTools的贡献者和moo4q的创建者Ryan Florence首次向我展示他出色CSS动画文章时 ,我感到非常震惊。 他爆炸性的文字效果是CSS3和少量JavaScript的强大示例。 我想在我的新博客重新设计中实现这种效果,但它要流行一些,所以我写了一些MooTools代码来拍摄静态图像,并使其成为动画,爆炸性的杰作。 让我向您展示我是如何做到的,此外,我还创建了一个jQuery片段来实现相同的效果。
瑞安·佛罗伦萨(Ryan Florence)的动画库 (Ryan Florence's Animation Library)
Ryan's CSS animation library, available with vanilla JavaScript, MooTools, or jQuery, and can only be described as a fucking work of art. His animation library is mobile-enabled, works a variety of A-grade browsers, and is very compact. Download and study Ryan's animation library before proceeding with this post.
RyanCSS动画库可与香草JavaScript,MooTools或jQuery一起使用,并且只能被描述为一件令人发指的艺术品。 他的动画库具有移动功能,可以使用各种A级浏览器,并且非常紧凑。 在继续本文之前,请下载并研究Ryan的动画库 。
Ryan's post also features an awesome demo and a few helpful functions. A few of these functions include:
Ryan的帖子还提供了一个很棒的演示和一些有用的功能。 其中一些功能包括:
// reset transforms to this
var zeros = {x:0, y:0, z:0};
// Implement animation methods on the element prototype
Element.implement({
// Scatter elements all over the place
scatter: function(){
return this.translate({
x: Number.random(-1000, 1000),
y: Number.random(-1000, 1000),
z: Number.random(-500, 500)
}).rotate({
x: Number.random(-720, 720),
y: Number.random(-720, 720),
z: Number.random(-720, 720)
});
},
// Return them to their original state
unscatter: function(){
return this.translate(zeros).rotate(zeros);
},
// Frighten the image! AHHHHHHHH!
frighten: function(d){
this.setTransition('timing-function', 'ease-out').scatter();
setTimeout(function(){
this.setTransition('timing-function', 'ease-in-out').unscatter();
}.bind(this), 500);
return this;
},
// Zoooooom into me
zoom: function(delay){
var self = this;
this.scale(0.01);
setTimeout(function(){
self.setTransition({
property: 'transform',
duration: '250ms',
'timing-function': 'ease-out'
}).scale(1.2);
setTimeout(function(){
self.setTransition('duration', '100ms').scale(1);
}, 250)
}, delay);
},
// Create a slider
makeSlider: function(){
var open = false,
next = this.getNext(),
height = next.getScrollSize().y,
transition = {
property: 'height',
duration: '500ms',
transition: 'ease-out'
};
next.setTransition(transition);
this.addEvent('click', function(){
next.setStyle('height', open ? 0 : height);
open = !open;
});
},
// Scatter, come back
fromChaos: (function(x){
var delay = 0;
return function(){
var element = this;
//element.scatter();
setTimeout(function(){
element.setTransition({
property: 'transform',
duration: '500ms',
'timing-function': 'ease-out'
});
setTimeout(function(){
element.unscatter();
element.addEvents({
mouseenter: element.frighten.bind(element),
touchstart: element.frighten.bind(element)
});
}, delay += x);
}, x);
}
}())
});
Now let's jump on the exploding logo!
现在,让我们跳上爆炸的徽标!
HTML (The HTML)
The exploding element can be of any type, but for the purposes of this example, we'll use an A element with a background image:
爆炸元素可以是任何类型,但是出于本示例的目的,我们将使用带有背景图像的A元素:
<a href="/" id="homeLogo">David Walsh Blog</a>
Make sure the element you use is a block element, or styled to be block.
确保您使用的元素是一个块元素,或样式设置为块。
CSS (The CSS)
The original element should be styled to size (width and height) with the background image that we'll use as the exploding image:
应使用我们将用作爆炸图像的背景图像将原始元素的样式设置为大小(宽度和高度):
a#homeLogo {
width:300px;
height:233px;
text-indent:-3000px;
background:url(/wp-content/themes/2k11/images/homeLogo.png) 0 0 no-repeat;
display:block;
z-index:2;
}
a#homeLogo span {
float:left;
display:block;
background-image:url(/wp-content/themes/2k11/images/homeLogo.png);
background-repeat:no-repeat;
}
.clear { clear:both; }
Remember to set the text-indent setting so that the link text will not display. The explosion shards will be JavaScript-generated SPAN elements which are displayed as in block format. Note that the SPAN has the same background image as the A element -- we'll simply modify the background position of the element to act as the piece of the logo that each SPAN represents.
记住要设置文本缩进设置,以使链接文本不会显示。 爆炸碎片将是JavaScript生成的SPAN元素,它们以块格式显示。 请注意,SPAN具有与A元素相同的背景图片-我们将简单地修改元素的背景位置,以充当每个SPAN所代表的徽标。
MooTools JavaScript (The MooTools JavaScript)
The first step is putting together a few variables we'll need to to calculate element dimensions:
第一步是将一些我们需要用来计算元素尺寸的变量放在一起:
// Get the proper CSS prefix from the page
var cssPrefix = false;
switch(Browser.name) { // Implement only for Chrome, Firefox, and Safari
case "safari":
case "chrome":
cssPrefix = "webkit";
break;
case "firefox":
cssPrefix = "moz";
break;
}
if(cssPrefix) {
// 300 x 233
var cols = 10; // Desired columns
var rows = 8; // Desired rows
var totalWidth = 300; // Logo width
var totalHeight = 233; // Logo height
var singleWidth = Math.ceil(totalWidth / cols); // Shard width
var singleHeight = Math.ceil(totalHeight / rows); // Shard height
var shards = []; // Array of SPANs
You'll note that I've explicitly set the number of columns and rows I want. You don't want the shards to be too large or too small, so feel free to experiment. You could probably use another calculation to get column and row numbers, but I'll leave that up to you.
您会注意到,我已经明确设置了所需的列数和行数。 您不希望碎片太大或太小,因此可以随时尝试。 您可能可以使用其他计算来获取列号和行号,但我将由您自己决定。
The next step is looping through each row and column, creating a new SPAN element for each shard. The background position, width, and height of the SPAN will be calculated with the ... calculations ... we ... calculated ... above.
下一步是遍历每一行和每一列,为每个分片创建一个新的SPAN元素。 SPAN的背景位置,宽度和高度将通过... ... ... ... ... ... ... ... ...计算出来。
// Remove the text and background image from the logo
var logo = document.id("homeLogo").set("html","").setStyles({ backgroundImage: "none" });
// For every desired row
rows.times(function(rowIndex) {
// For every desired column
cols.times(function(colIndex) {
// Create a SPAN element with the proper CSS settings
// Width, height, browser-specific CSS
var element = new Element("span",{
style: "width:" + (singleWidth) + "px;height:" + (singleHeight) + "px;background-position:-" + (singleHeight * colIndex) + "px -" + (singleWidth * rowIndex) + "px;-" + cssPrefix + "-transition-property: -" + cssPrefix + "-transform; -" + cssPrefix + "-transition-duration: 200ms; -" + cssPrefix + "-transition-timing-function: ease-out; -" + cssPrefix + "-transform: translateX(0%) translateY(0%) translateZ(0px) rotateX(0deg) rotateY(0deg) rotate(0deg);"
}).inject(logo);
// Save it
shards.push(element);
});
// Create a DIV clear for next row
new Element("div",{ clear: "clear" }).inject(logo);
});
With the SPAN elements, you'll note that several CSS3 properties are being set to it, allowing the browser to do its magic. Using CSS3 is much less resource-consuming within the browser than using JavaScript to do all of the animation.
使用SPAN元素,您会注意到已为其设置了多个CSS3属性,从而使浏览器可以发挥其魔力。 与使用JavaScript来制作所有动画相比,在浏览器中使用CSS3的资源消耗要少得多。
The last step is calling the fromChaos method provided by Ryan Florence's CSS animation code to set into motion the madness!
最后一步是调用Ryan FlorenceCSS动画代码提供的fromChaos方法,以使其疯狂起来!
// Chaos!
$$(shards).fromChaos(1000);
There you have it! A completely automated method of exploding an image using CSS3 and MooTools JavaScript!
你有它! 使用CSS3和MooTools JavaScript的完全自动化的爆炸图像方法!
jQuery JavaScript (The jQuery JavaScript)
Ryan also wrote the CSS animation code in jQuery so you can easily create a comparable effect with jQuery!
Ryan还在jQuery中编写了CSS动画代码,因此您可以轻松地创建与jQuery类似的效果!
Number.random = function(min, max){
return Math.floor(Math.random() * (max - min + 1) + min);
};
var zeros = {x:0, y:0, z:0};
jQuery.extend(jQuery.fn, {
scatter: function(){
return this.translate({
x: Number.random(-1000, 1000),
y: Number.random(-1000, 1000),
z: Number.random(-500, 500)
}).rotate({
x: Number.random(-720, 720),
y: Number.random(-720, 720),
z: Number.random(-720, 720)
});
},
unscatter: function(){
return this.translate(zeros).rotate(zeros);
},
frighten: function(d){
var self = this;
this.setTransition('timing-function', 'ease-out').scatter();
setTimeout(function(){
self.setTransition('timing-function', 'ease-in-out').unscatter();
}, 500);
return this;
},
zoom: function(delay){
var self = this;
this.scale(0.01);
setTimeout(function(){
self.setTransition({
property: 'transform',
duration: '250ms',
'timing-function': 'ease-out'
}).scale(1.2);
setTimeout(function(){
self.setTransition('duration', '100ms').scale(1);
}, 250)
}, delay);
return this;
},
makeSlider: function(){
return this.each(function(){
var $this = $(this),
open = false,
next = $this.next(),
height = next.attr('scrollHeight'),
transition = {
property: 'height',
duration: '500ms',
transition: 'ease-out'
};
next.setTransition(transition);
$this.bind('click', function(){
next.css('height', open ? 0 : height);
open = !open;
});
})
},
fromChaos: (function(){
var delay = 0;
return function(){
return this.each(function(){
var element = $(this);
//element.scatter();
setTimeout(function(){
element.setTransition({
property: 'transform',
duration: '500ms',
'timing-function': 'ease-out'
});
setTimeout(function(){
element.unscatter();
element.bind({
mouseenter: jQuery.proxy(element.frighten, element),
touchstart: jQuery.proxy(element.frighten, element)
});
}, delay += 100);
}, 1000);
})
}
}())
});
// When the DOM is ready...
$(document).ready(function() {
// Get the proper CSS prefix
var cssPrefix = false;
if(jQuery.browser.webkit) {
cssPrefix = "webkit";
}
else if(jQuery.browser.mozilla) {
cssPrefix = "moz";
}
// If we support this browser
if(cssPrefix) {
// 300 x 233
var cols = 10; // Desired columns
var rows = 8; // Desired rows
var totalWidth = 300; // Logo width
var totalHeight = 233; // Logo height
var singleWidth = Math.ceil(totalWidth / cols); // Shard width
var singleHeight = Math.ceil(totalHeight / rows); // Shard height
// Remove the text and background image from the logo
var logo = jQuery("#homeLogo").css("backgroundImage","none").html("");
// For every desired row
for(x = 0; x < rows; x++) {
var last;
//For every desired column
for(y = 0; y < cols; y++) {
// Create a SPAN element with the proper CSS settings
// Width, height, browser-specific CSS
last = jQuery("<span />").attr("style","width:" + (singleWidth) + "px;height:" + (singleHeight) + "px;background-position:-" + (singleHeight * y) + "px -" + (singleWidth * x) + "px;-" + cssPrefix + "-transition-property: -" + cssPrefix + "-transform; -" + cssPrefix + "-transition-duration: 200ms; -" + cssPrefix + "-transition-timing-function: ease-out; -" + cssPrefix + "-transform: translateX(0%) translateY(0%) translateZ(0px) rotateX(0deg) rotateY(0deg) rotate(0deg);");
// Insert into DOM
logo.append(last);
}
// Create a DIV clear for row
last.append(jQuery("<div />").addClass("clear"));
}
// Chaos!
jQuery("#homeLogo span").fromChaos();
}
});
Not as beautiful as the MooTools code, of course, but still effective!
虽然不如MooTools代码那么漂亮,但仍然有效!
And there you have it: CSS animations, JavaScript, and dynamic effects. My favorite part of this effect is how little code is involved. You get a lot of bang with your buck with this. Of course, using this effect everywhere would surely get groans so use it wisely!
在那里,您可以找到它:CSS动画,JavaScript和动态效果。 我最喜欢的效果是很少涉及代码。 您为此付出了很多努力。 当然,在任何地方使用此效果肯定会吟,因此请明智地使用它!
mootools