简单java程序
Simple java programs are good for assessing the coding skills of a programmer. You will find coding related questions in almost any interview.
简单的Java程序非常适合评估程序员的编码技能。 您几乎可以在任何面试中找到与编码相关的问题。
The idea of these programming questions is to look into the thought process of the candidate. Most of the times the initial solution is not efficient, which calls for further questions and see if interviewee can improve it further or not.
这些编程问题的想法是研究候选人的思考过程。 在大多数情况下,最初的解决方案效率不高,这就需要进一步的问题,并查看受访者是否可以进一步改进它。
简单的Java程序 (Simple Java Programs)
Here are I am providing 10 simple java programs. They are good for coding practice and can be used in interviews. Please try to write the solution yourself before looking at the answer, that will give you more confidence and you can check if your solution is good or not. Note that the solutions can be improved further, so if you got a better approach then please let us know through comments.
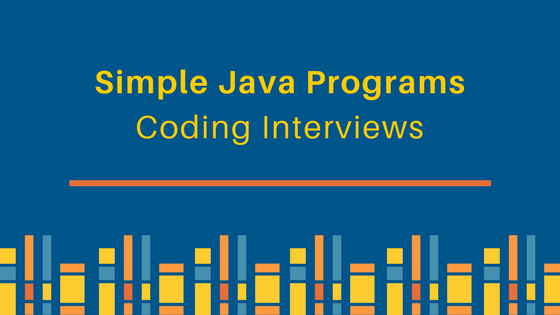
我在这里提供10个简单的Java程序。 它们非常适合编码实践,可以在访谈中使用。 在查看答案之前,请尝试自己编写解决方案,这将使您更有信心,并且可以检查您的解决方案是否良好。 请注意,可以进一步改善解决方案,因此,如果您有更好的方法,请通过评论告知我们。
反转字符串 (Reverse a String)
package com.journaldev.javaprograms;
public class JavaReverseString {
public static void main(String[] args) {
System.out.println(reverseString("abc"));
System.out.println(reverseString("123!@#098*"));
}
public static String reverseString(String in) {
if (in == null)
return null;
StringBuilder out = new StringBuilder();
int length = in.length();
for (int i = length - 1; i >= 0; i--) {
out.append(in.charAt(i));
}
return out.toString();
}
}
- Null check to avoid NullPointerException. 空检查以避免NullPointerException 。
- Using
StringBuilder
instead ofStringBuffer
for better performance. 使用StringBuilder
而不是StringBuffer
可获得更好的性能。 - Creating a local variable for input string length, rather than having it in if condition. Less number of function calls, better the performance. 创建用于输入字符串长度的局部变量,而不是将其置于if条件中。 函数调用次数越少,性能越好。
- Could have been improved by taking user input rather than static testing data 可以通过接受用户输入而不是静态测试数据来进行改进
斐波那契系列 (Fibonacci Series)
package com.journaldev.javaprograms;
public class FibonacciSeries {
public static void main(String[] args) {
printFibonacciSeries(10);
}
public static void printFibonacciSeries(int count) {
int a = 0;
int b = 1;
int c = 1;
for (int i = 1; i <= count; i++) {
System.out.print(a + ", ");
a = b;
b = c;
c = a + b;
}
}
}
- Program can be improved by taking user input for number of integers to print. 通过接受用户输入要打印的整数数可以改进程序。
- Notice the use of
System.out.print
function to print numbers in single line, good job. 注意,使用System.out.print
函数可以单行打印数字,这很不错。 - Fibonacci numbers starts from 0 or 1, above program can be extended to take user input for starting point. 斐波那契数从0或1开始,上述程序可以扩展为以用户输入为起点。
- Nicely written simple program, good to see no use of recursion or complex coding. 编写精美的简单程序,很高兴看到没有使用递归或复杂的编码。
素数检查 (Prime Number Check)
package com.journaldev.javaprograms;
import java.util.Scanner;
public class CheckPrimeNumber {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.print("Enter number to check for prime:");
int n = s.nextInt();
s.close();
checkPrime(n);
}
private static void checkPrime(int n) {
if (n == 0 || n == 1) {
System.out.println(n + " is not a prime number");
return;
}
if (n == 2) {
System.out.println(n + " is a prime number");
}
for (int i = 2; i <= n / 2; i++) {
if (n % i == 0) {
System.out.println(n + " is not a prime number");
return;
}
}
System.out.println(n + " is a prime number");
}
}
- Good to see use of Scanner class to take user input. 很高兴看到使用Scanner类接受用户输入。
- Notice the few if checks to check the numbers where rules are different i.e. 0,1 and 2. 0 and 1 are not prime numbers and 2 is the only even prime number. 注意少数检查规则的数字,即0,1和2。0和1不是质数,而2是唯一的偶质数。
- The division check is done only till
i <= n / 2
, smart thinking and good for performance. 仅在i <= n / 2
之前进行除法检查,思维敏锐且对性能有益。 - The method will fail incase of negative integers - Either let user know to enter positive integer in the main method Or make the integer positive and then process it. 在负整数的情况下,该方法将失败-要么让用户知道在主方法中输入正整数,要么使整数为正,然后对其进行处理。
检查回文字符串 (Check for Palindrome String)
package com.journaldev.javaprograms;
import java.util.Scanner;
public class PalindromeString {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.println("Enter String to check for Palindrome:");
String str = s.next();
s.close();
checkPalindrome(str);
}
private static void checkPalindrome(String str) {
char[] charArray = str.toCharArray();
StringBuilder sb = new StringBuilder();
for (int i = charArray.length - 1; i >= 0; i--) {
sb.append(charArray[i]);
}
if (sb.toString().equalsIgnoreCase(str))
System.out.println(str + " is palindrome.");
else
System.out.println(str + " is not palindrome");
}
}
- Creating a new string in reverse order using StringBuilder and then checking if its value is same as the original string. 使用StringBuilder以相反的顺序创建新字符串,然后检查其值是否与原始字符串相同。
- Implementation can be improved by comparing the characters from both end. If String length is 5 then it's palindrome if chars at 0th==4th and 1st==3rd. No need to create a separate string. Try to write code for this and post in comments section. 通过比较两端的字符可以改善实现。 如果字符串长度为5,则在0th == 4th和1st == 3rd时为回文。 无需创建单独的字符串。 尝试为此编写代码,然后在评论部分中发布。
以编程方式对数组进行排序 (Sort an array programmatically)
package com.journaldev.javaprograms;
import java.util.Arrays;
public class JavaArraySort {
public static void main(String[] args) {
int[] array = {2,1,5,3,4,6,7};
int[] sortedArray = bubbleSortAscending(array);
System.out.println(Arrays.toString(sortedArray));
}
public static int[] bubbleSortAscending(int[] arr){
int temp;
for(int i=0; i < arr.length-1; i++){
for(int j=1; j < arr.length-i; j++){
if(arr[j-1] > arr[j]){
temp=arr[j-1];
arr[j-1] = arr[j];
arr[j] = temp;
}
}
//check that last index has highest value in first loop,
// second last index has second last highest value and so on
System.out.println("Array after "+(i+1)+"th iteration:"+Arrays.toString(arr));
}
return arr;
}
}
- There are many sorting algorithms, bubble sort is easier to implement. 排序算法很多,气泡排序更易于实现。
- Sorting is complex and you should rely on Java API methods for sorting a collection or array for better performance that inventing the wheel again. 排序很复杂,您应该依靠Java API方法对集合或数组进行排序,以获得更好的性能,而这又需要重新发明轮子。
- Also mention the use of Comparable and Comparator in sorting will add bonus points for you. 还要提及在排序中使用Comparable和Comparator会为您增加奖励积分。
读取3个输入字符串,连接和打印 (Read 3 input Strings, concat and print)
package com.journaldev.javaprograms;
import java.util.Scanner;
public class ReadStringAndConcat {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter number of Strings to Concatenate:");
int n = scanner.nextInt();
String[] input = new String[n];
for(int i=0; i<n; i++) {
System.out.println("Please enter String number "+n+" and press enter:");
input[i] = scanner.next();
}
//close Scanner and avoid resource leak
scanner.close();
String output = concat(input);
System.out.println("Concatenation Result = "+output);
}
private static String concat(String[] input) {
StringBuilder sb = new StringBuilder();
for(String s : input) sb.append(s);
return sb.toString();
}
}
- Program is flexible to concat any number of strings, that shows thinking to code reuse and keeping it flexible. 程序可以灵活地连接任意数量的字符串,这表明了思考代码重用并保持灵活的想法。
- Proper messages to guide the user when someone runs the program. 当某人运行该程序时,正确的消息可以指导用户。
- Use of StringBuilder rather than String + operator for concatenation. 使用StringBuilder而不是String +运算符进行串联。
- Closing resources as soon as we are done with it, hence avoiding memory leak. Shows good programming habits. 我们一完成就关闭资源,从而避免内存泄漏。 显示良好的编程习惯。
从整数数组中删除奇数 (Remove odd numbers from integer array)
package com.journaldev.javaprograms;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Scanner;
public class RemoveOddNumbers {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter comma separated numbers for input int array, for example '1,2,3,4'");
String input = scanner.next();
scanner.close();
//convert to int array
String[] inArray = input.split(",");
int [] intArray = new int[inArray.length];
int index = 0;
for(String s : inArray) {
intArray[index] = Integer.parseInt(s.trim());
index++;
}
//call a function to remove odd numbers
Integer[] result = removeOddNumbers(intArray);
System.out.println(Arrays.toString(result));
}
private static Integer[] removeOddNumbers(int[] intArray) {
//we will have to use list because we don't know exact size of the result array
List<Integer> list = new ArrayList<>();
for(int i : intArray) {
if(i%2 == 0) list.add(i);
}
return list.toArray(new Integer[list.size()]);
}
}
If you are reading this code, you should see that it adheres to all the points mentioned above. Try to write clean and simple code, follow best practices, naming conventions for methods and variables and you will be good.
如果您正在阅读此代码,则应该看到它符合上述所有要点。 尝试编写简洁明了的代码,遵循最佳实践,为方法和变量命名约定,这将很不错。
从列表中删除所有匹配的元素 (Delete all matching elements from a list)
package com.journaldev.javaprograms;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Scanner;
public class DeleteFromList {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter comma separated list of Strings. For example 'a,b,c,b,a'");
String input = scanner.next();
System.out.println("Enter String to remove from the input list.");
String strToDelete = scanner.next();
scanner.close();
List<Object> inputList = new ArrayList<>();
String[] inputStrings = input.split(",");
for (String s : inputStrings)
inputList.add(s.trim());
inputList = removeAll(inputList, strToDelete);
System.out.println("Result List = "+inputList);
}
private static List<Object> removeAll(List<Object> inputList, Object objToDelete) {
Iterator<Object> it = inputList.iterator();
while(it.hasNext()) {
Object obj = it.next();
if(obj.equals(objToDelete)) it.remove();
}
return inputList;
}
}
- Notice the removeAll method is created for list of objects, so it will work with any type of list. This is how we write reusable code. 请注意,removeAll方法是为对象列表创建的,因此它将与任何类型的列表一起使用。 这就是我们编写可重用代码的方式。
- Using iterator to remove the element from the list. 使用迭代器从列表中删除元素。
- Trimming the input to remove any accidental white spaces from input strings. 修剪输入以消除输入字符串中的任何意外空格。
四舍五入和小数位数为2的数字的平均值 (Average of numbers with rounding half-up and scale 2)
package com.journaldev.javaprograms;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.util.Scanner;
public class AverageOfNumbers {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter the total number of integers.");
int count = scanner.nextInt();
int sum = 0;
for (int i = 0; i < count; i++) {
System.out.println("Please enter number " + (i + 1) + ":");
sum += scanner.nextInt();
}
System.out.println("Sum=" + sum + ",Count=" + count);
BigDecimal average = new BigDecimal((double) sum / count);
average = average.setScale(2, RoundingMode.HALF_UP);
System.out.println("Average of entered numbers = " + average);
scanner.close();
}
}
交换两个数字 (Swapping two numbers)
package com.journaldev.javaprograms;
import java.util.Scanner;
public class SwappingNumbers {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the first number:");
int first = scanner.nextInt();
System.out.println("Enter the second number:");
int second = scanner.nextInt();
scanner.close();
System.out.println("Initial value of numbers are: "+first+" and "+second);
first = first + second;
second = first -second;
first = first - second;
System.out.println("Value of numbers after swapping are: "+first+" and "+second);
}
}
We can easily swap numbers using a temporary variable. But if you can do it without that, then it's awesome.
我们可以使用一个临时变量轻松地交换数字。 但是,如果您不这样做就能做到,那就太好了。
That's all for simple java programs for interviews. Go through them and try to learn the best ways to write code and impress the interviewer.
这就是简单的Java面试程序。 仔细研究它们,尝试学习编写代码并打动面试官的最佳方法。
Reference: Really Big Index for Java
参考: Java的真正大索引
简单java程序