iphone 程序不足
Have you thought about creating an iPhone application (app), but didn't even know where to get started? Here's how:
您是否曾考虑过创建iPhone应用程序(应用程序),但甚至不知道从哪里开始呢? 这是如何做:
~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~
〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜
Important pre-programming comments:
重要的预编程注释:
I’ve never tried other options, only those recommended by Apple:
我从未尝试过其他选择,只有Apple推荐的选择:
1. Intel-based Mac computer running Snow Leopard
1.运行Snow Leopard的基于Intel的Mac计算机
2. iPhone SDK 3.2 installed on this computer
2.在此计算机上安装的iPhone SDK 3.2
Also, this article discusses only how to create and simulate a simple iPhone app,
此外,本文仅讨论如何创建和模拟简单的iPhone应用程序,
it does not get into the details of how to load and test on an actual iPhone.
它没有详细介绍如何在实际的iPhone上加载和测试。
~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~
〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜〜
Once you have the SDK, we can start.
有了SDK之后,我们就可以开始了。
ToDo
去做
1. Launch Xcode.
1.启动Xcode。
2. Create a new iPhone Window-based application.
2.创建一个新的基于iPhone Window的应用程序。
3. Modify the application delegate class.
3.修改应用程序委托类。
#import <UIKit/UIKit.h>
@interface FirstAppDelegate : NSObject <UIApplicationDelegate> {
UIWindow *window;
// add the following line to the generated code
UITextField *text;
}
@property (nonatomic, retain) IBOutlet UIWindow *window;
// add the following 2 lines to the generated code
@property (nonatomic, retain) IBOutlet UITextField *text;
- (IBAction) buttonClicked:(id)sender;
@end
#import "FirstAppDelegate.h"
@implementation FirstAppDelegate
@synthesize window;
// Add this block
@synthesize text;
- (IBAction) buttonClicked:(id)sender
{
[text setText:@"Hello"];
}
// End of the new block
- (BOOL)application:(UIApplication *)application
didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch
[window makeKeyAndVisible];
return YES;
}
- (void)dealloc {
[window release];
[super dealloc];
}
@end
4. Save the project.
4.保存项目。
5. Arrange the application GUI in Interface Builder.
5.在Interface Builder中安排应用程序GUI。
Control-drag from the Push Button in the “First” window to the FirstAppDelegate object in the Document window below. When you release the mouse, in the popup menu select “-buttonClicked” item.
将控件从“第一”窗口中的“按钮”拖动到下面的“文档”窗口中的FirstAppDelegate对象。 释放鼠标时,在弹出菜单中选择“ -buttonClicked”项。
Find Text field in the Library - type “Text” in the search field. Drag Text Field to the application window. Connect the application delegate with the Text Field - hold Control and drag with the mouse from the application delegate object to the text field. When you release the mouse button above the text field, select “text” item in the popup menu that appears.
在库中查找文本字段-在搜索字段中键入“文本”。 将文本字段拖到应用程序窗口。 将应用程序委托与文本字段连接-按住Control并用鼠标将鼠标从应用程序委托对象拖到文本字段。 释放文本字段上方的鼠标按钮时,在出现的弹出菜单中选择“文本”项。
6. Save your work in the Interface Builder.
6.将您的工作保存在Interface Builder中。
7. Build and Run.
7.生成并运行。
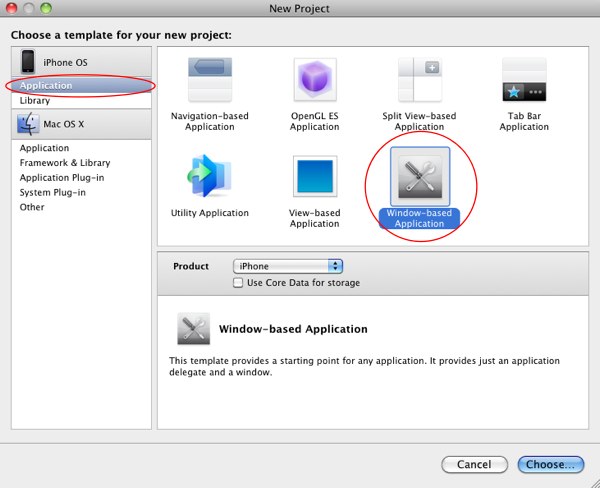
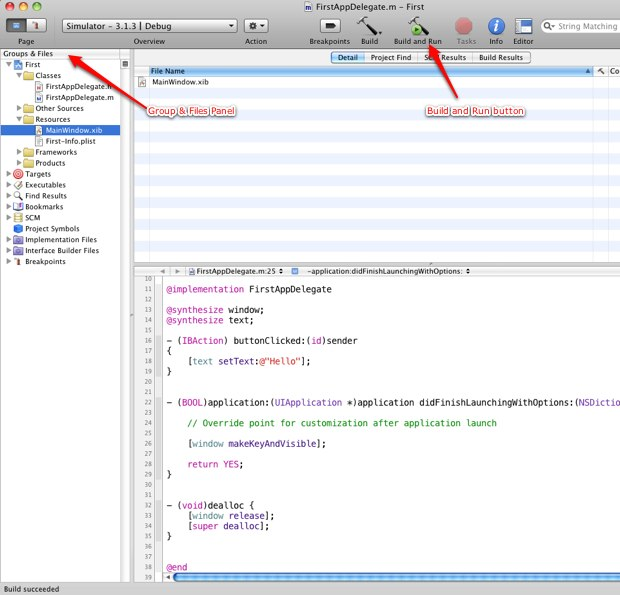
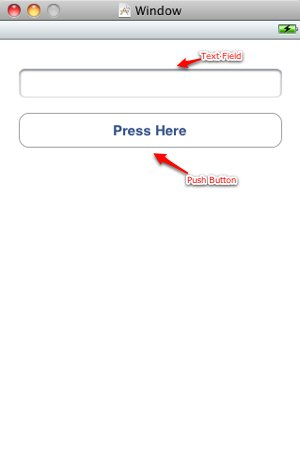
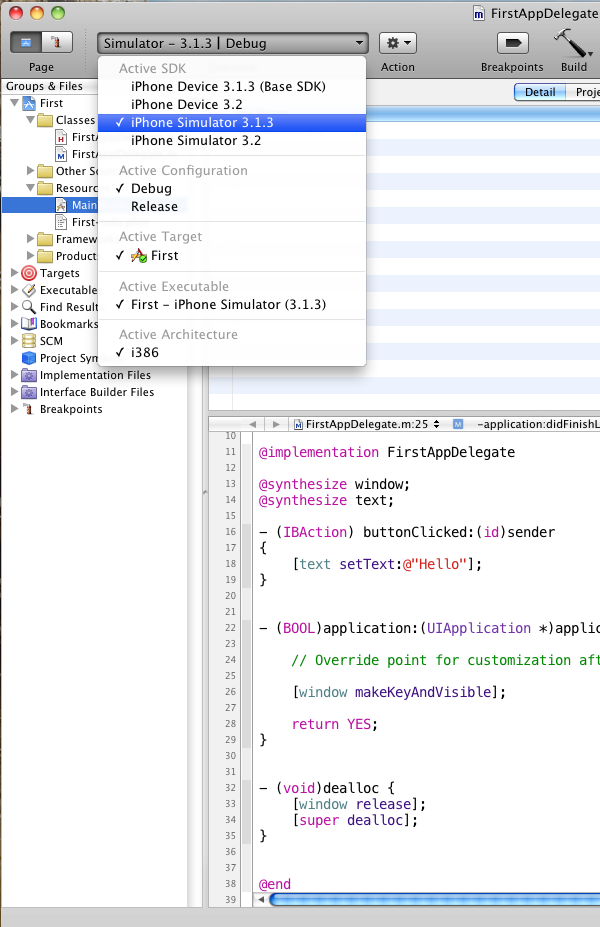
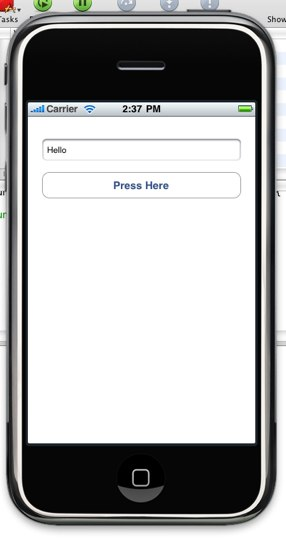
Recommendations
推荐建议
I'd like to propose a way I used to learn Objective-C and Cocoa. A few of my friends have tested this approach and found it helpful.
我想提出一种我用来学习Objective-C和Cocoa的方法。 我的一些朋友已经测试了这种方法,并发现它很有用。
1. Begin from the book “Programming in Objective-C 2.0” Stephen G. Kochan. With this book you will write small command-line tools for Mac OS and will learn the basics of Objective-C. You don’t have to finish this book, even a half of that will allow you to go up to the next step.
1.从“ Objective-C 2.0中的编程”一书开始,Stephen G. Kochan。 本书将为Mac OS编写小型命令行工具,并学习Objective-C的基础知识。 您不必完成这本书,即使其中的一半也可以使您继续进行下一步。
2. You can find a great tutorial on Mac OS Reference Library - Currency Converter. It will take you few days (it is better if it will be few days) to read and understand everything, to implement this Currency Converter application. This tutorial also explains the basic principles of Objective-C. It will balance and arrange this beginning knowledge in your head. After this step you can make simple GUI applications yourself. Find few ideas, something that you need in your day-to-day work, a decimal-to-hexadecimal converter for example, and implement it.
2.您可以在Mac OS参考库-Currency Converter上找到很棒的教程。 阅读并理解所有内容,以实现此Currency Converter应用程序将需要几天的时间(最好是几天的时间)。 本教程还介绍了Objective-C的基本原理。 它将平衡并安排您的入门知识。 完成此步骤后,您可以自己制作简单的GUI应用程序。 找出一些想法,例如日常工作中需要的东西,例如十进制到十六进制的转换器,然后加以实现。
3. At this stage you already beginning to understand the technical articles about Objective-C, Mac OS and iPhone programming. You can find many technical resources in the internet. A few of them are listed in the end of this article.
3.在这一阶段,您已经开始了解有关Objective-C,Mac OS和iPhone编程的技术文章。 您可以在Internet上找到许多技术资源。 本文结尾列出了其中的一些。
4. Now it’s time again for a book. I think the best book (for now) about Objective-C 2.0 and Cocoa is “Cocoa and Objective-C Up and Running” from Scott Stevenson. This book teaches you to develop in Cocoa for Mac OS, but always shows the differences between the Cocoa and Cocoa Touch (iPhone SDK). The author found the best words to explain everything about Objective-C and Cocoa.
4.现在是时候再来一本书了。 我认为关于Objective-C 2.0和Cocoa的最好的书(目前)是Scott Stevenson撰写的“ Cocoa and Objective-C Up and Running”。 本书教您如何在Mac OS的Cocoa中进行开发,但始终显示Cocoa和Cocoa Touch(iPhone SDK)之间的区别。 作者找到了最好的词汇来解释有关Objective-C和可可的所有内容。
5. You are ready to program for the iPhone. Everything iPhone SDK related should be clear for you now. There are a lot of books about the iPhone development, but most of them will be a short versions of the books I have mentioned. The differences between the Mac OS development and iPhone development are not that big. At least, in the beginning it seems to be not that big. I can also say that Cocoa Touch is like a small version of Cocoa.
5.您已准备好为iPhone编程。 与iPhone SDK相关的所有内容现在都应该清楚了。 关于iPhone开发的书籍很多,但是其中大多数是我提到的书籍的简短版本。 Mac OS开发和iPhone开发之间的差异不是很大。 至少在开始时似乎没有那么大。 我也可以说可可触摸就像可可的小版本。
This plan makes sense if you already have an Intel-based Mac computer, it already has Snow Leopard, you have registered yourself on the Apple developer portal, downloaded and installed iPhone SDK.
如果您已经有一台基于Intel的Mac计算机,它已经有Snow Leopard,并且已经在Apple开发人员门户网站上注册,下载并安装了iPhone SDK,那么该计划就很有意义。
I can't promise that this will be as short and simple as you may wish. It is likely to take longer than a month or two. It is not because Objective-C is a difficult programming language or Cocoa is a very rich framework to learn. It’s because you’ll love this language and this framework same way as you love your iPhone. Along the way you will find other attractive topics to learn, such as AppleScript or bash. You may wish to work with iPhoto or with a dozen of other famous Mac applications. It is an interesting path, and you are likely to enjoy it.
我不能保证这会如您所愿那样简短。 可能需要一两个月以上的时间。 这不是因为Objective-C是一种难于编程的语言,也不是因为Cocoa是一个非常丰富的学习框架。 这是因为您会像爱iPhone一样喜欢这种语言和框架。 在此过程中,您会发现其他有趣的主题需要学习,例如AppleScript或bash。 您可能希望使用iPhoto或其他许多著名的Mac应用程序。 这是一条有趣的道路,您可能会喜欢它。
References
参考资料
1. Mac OS参考库
2. 可可我的女朋友
3. 可可与爱
翻译自: https://www.experts-exchange.com/articles/3378/First-iPhone-Application.html
iphone 程序不足