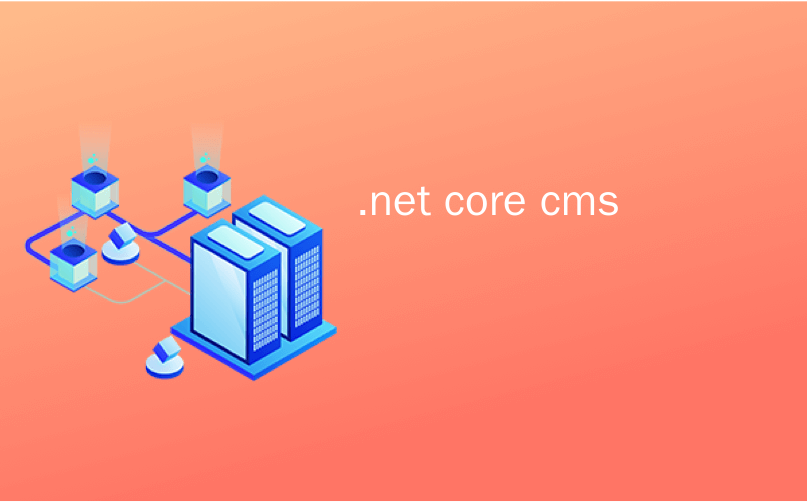
.net core cms
I'm sure I'll miss some, so if I do, please sound off in the comments and I'll update this post over the next week or so!
我敢肯定我会想念的,所以,如果有的话,请在评论中注明,我将在下一周左右更新此帖子!
Lately I've been noticing a lot of "Headless" CMSs (Content Management System). A ton, in fact. I wanted to explore this concept and see if it's a fad or if it's really something useful.
最近,我注意到了很多“无头” CMS(内容管理系统)。 实际上是一吨。 我想探索这个概念,看看它是一种时尚还是真的有用。
Given the rise of clean RESTful APIs has come the rise of Headless CMS systems. We've all evaluated CMS systems (ones that included both front- and back-ends) and found the front-end wanting. Perhaps it lacks flexibility OR it's way too flexible and overwhelming. In fact, when I wrote my podcast website I considered a CMS but decided it felt too heavy for just a small site.
随着干净的RESTful API的兴起,Headless CMS系统的兴起。 我们都对CMS系统(包括前端和后端的系统)进行了评估,并发现了前端系统的需求。 也许它缺乏灵活性,或者它过于灵活和压倒性。 实际上,当我编写播客网站时,我曾考虑过CMS,但认为它对于一个小型网站来说太沉重了。
A Headless CMS is a back-end only content management system (CMS) built from the ground up as a content repository that makes content accessible via a RESTful API for display on any device.
无头CMS是仅后端的内容管理系统(CMS) ,它是从头开始构建的内容存储库,可通过RESTful API访问内容以在任何设备上显示。
I could start with a database but what if I started with a CMS that was just a backend - a headless CMS. I'll handle the front end, and it'll handle the persistence.
我可以从数据库开始,但是如果我从只是后端的CMS(无头CMS)开始,该怎么办。 我将处理前端,它将处理持久性。
Here's what I found when exploring .NET Core-based Headless CMSs. One thing worth noting, is that given Docker containers and the ease with which we can deploy hybrid systems, some of these solutions have .NET Core front-ends and "who cares, it returns JSON" for the back-end!
这是我在探索基于.NET Core的Headless CMS时发现的。 值得注意的一件事是,鉴于Docker容器以及我们部署混合系统的简便性,其中一些解决方案具有.NET Core前端,并且“谁在乎,它会返回JSON”作为后端!
林肯 (Lynicon)
Lyncicon is literally implemented as a NuGet Library! It stores its data as structured JSON. It's built on top of ASP.NET Core and uses MVC concepts and architecture.
Lyncicon实际上是作为NuGet库实现的! 它将其数据存储为结构化JSON。 它基于ASP.NET Core构建,并使用MVC概念和体系结构。
It does include a front-end for administration but it's not required. It will return HTML or JSON depending on what HTTP headers are sent in. This means you can easily use it as the back-end for your Angular or existing SPA apps.
它的确包含一个管理前端,但这不是必需的。 它将根据发送的HTTP标头返回HTML或JSON。这意味着您可以轻松地将其用作Angular或现有SPA应用程序的后端。
Lyncion is largely open source at https://github.com/jamesej/lyniconanc. If you want to take it to the next level there's a small fee that gives you updated searching, publishing, and caching modules.
Lyncion在https://github.com/jamesej/lyniconanc很大程度上是开源的。 如果您想将其提升到一个新的水平,可以支付一小笔费用,为您提供更新的搜索,发布和缓存模块。
ButterCMS (ButterCMS)
ButterCMS is an API-based CMS that seamlessly integrates with ASP.NET applications. It has an SDK that drops into ASP.NET Core and also returns data as JSON. Pulling the data out and showing it in a few is easy.
ButterCMS是基于API的CMS,可与ASP.NET应用程序无缝集成。 它具有一个SDK,该SDK可以插入ASP.NET Core,并且还可以将数据作为JSON返回。 提取数据并将其显示出来很容易。
public class CaseStudyController : Controller
{
private ButterCMSClient Client;
private static string _apiToken = "";
public CaseStudyController()
{
Client = new ButterCMSClient(_apiToken);
}
[Route("customers/{slug}")]
public async Task<ActionResult> ShowCaseStudy(string slug)
{
var json = await Client.ListPageAsync("customer_case_study", slug)
dynamic page = ((dynamic)JsonConvert.DeserializeObject(json)).data.fields;
ViewBag.SeoTitle = page.seo_title;
ViewBag.FacebookTitle = page.facebook_open_graph_title;
ViewBag.Headline = page.headline;
ViewBag.CustomerLogo = page.customer_logo;
ViewBag.Testimonial = page.testimonial;
return View("Location");
}
}
Then of course output into Razor (or putting all of this into a RazorPage) is simple:
然后,当然输出到Razor(或将所有这些都放入RazorPage)很简单:
<html>
<head>
<title>@ViewBag.SeoTitle</title>
<meta property="og:title" content="@ViewBag.FacebookTitle" />
</head>
<body>
<h1>@ViewBag.Headline</h1>
<img width="100%" src="@ViewBag.CustomerLogo">
<p>@ViewBag.Testimonial</p>
</body>
</html>
Butter is a little different (and somewhat unusual) in that their backend API is a SaaS (Software as a Service) and they host it. They then have SDKs for lots of platforms including .NET Core. The backend is not open source while the front-end is https://github.com/ButterCMS/buttercms-csharp.
Butt稍有不同(并且有些不同寻常),因为它们的后端API是SaaS(软件即服务)并由其托管。 然后,他们有了适用于许多平台(包括.NET Core)的SDK。 后端不是开源的,而前端是https://github.com/ButterCMS/buttercms-csharp 。
食人鱼CMS (Piranha CMS)
Piranha CMS is built on ASP.NET Core and is open source on GitHub. It's also totally package-based using NuGet and can be easily started up with a dotnet new template like this:
Piranha CMS基于ASP.NET Core构建,并在GitHub上开源。 它还使用NuGet完全基于包,并且可以使用如下所示的dotnet新模板轻松启动:
dotnet new -i Piranha.BasicWeb.CSharp
dotnet new piranha
dotnet restore
dotnet run
It even includes a new Blog template that includes Bootstrap 4.0 and is all set for customization. It does include optional lightweight front-end but you can use those as guidelines to create your own client code. One nice touch is that Piranha also includes image resizing and cropping.
它甚至包括一个新的Blog模板,其中包括Bootstrap 4.0,并且都已针对自定义进行了设置。 它确实包括可选的轻量级前端,但是您可以将其用作准则来创建自己的客户端代码。 一个不错的感觉是食人鱼还包括图像大小调整和裁剪。
Umbraco无头 (Umbraco Headless)
The main ASP.NET website currently uses Umbraco as its CMS. Umbraco is a well-known open source CMS that will soon include a Headless option for more flexibility. The open source code for Umbraco is up here https://github.com/umbraco.
ASP.NET主要网站当前使用Umbraco作为其CMS。 Umbraco是著名的开源CMS,不久将包含Headless选项,以提供更大的灵活性。 Umbraco的开源代码在https://github.com/umbraco上。
果园核心 (Orchard Core)
Orchard is a CMS with a very strong community and fantastic documentation. Orchard Core is a redevelopment of Orchard using open source ASP.NET Core. While it's not "headless" it is using a Decoupled Architecture. Nothing would prevent you from removing the UI and presenting the content with your own front-end. It's also cross-platform and container friendly.
Orchard是一个具有非常强大的社区和出色文档的CMS。 Orchard Core是Orchard使用开源ASP.NET Core的重新开发。 虽然不是“无头”,但它使用的是Decoupled Architecture 。 没有什么可以阻止您删除UI并使用自己的前端呈现内容。 它也是跨平台和容器友好的。
鱿鱼 (Squidex)
"Squidex is an open source headless CMS and content management hub. In contrast to a traditional CMS Squidex provides a rich API with OData filter and Swagger definitions." Squidex is build with ASP.NET Core and the CQRS pattern and works with both Windows and Linux on today's browsers.
“ Squidex是一个开源的无头CMS和内容管理中心。与传统的CMS相比,Squidex提供了具有OData过滤器和Swagger定义的丰富API。” Squidex是使用ASP.NET Core和CQRS模式构建的,并且可以在当今的浏览器上同时使用Windows和Linux。
Squidex is open source with excellent docs at https://docs.squidex.io. Docs are at https://docs.squidex.io. They are also working on a hosted version you can play with here https://cloud.squidex.io. Samples on how to consume it are here https://github.com/Squidex/squidex-samples.
Squidex是https://docs.squidex.io上的开源文档,其中包含出色的文档。 文档位于https://docs.squidex.io 。 他们还在开发托管版本,您可以在这里使用https://cloud.squidex.io 。 有关如何使用它的示例,请参见https://github.com/Squidex/squidex-samples 。
The consumption is super clean:
消耗超级干净:
[Route("/{slug},{id}/")]
public async Task<IActionResult> Post(string slug, string id)
{
var post = await apiClient.GetBlogPostAsync(id);
var vm = new PostVM
{
Post = post
};
return View(vm);
}
And then the View:
然后查看:
@model PostVM
@{
ViewData["Title"] = Model.Post.Data.Title;
}
<div>
<h2>@Model.Post.Data.Title</h2>
@Html.Raw(Model.Post.Data.Text)
</div>
What .NET Core Headless CMSs did I miss? Let me know.
我错过了哪些.NET Core无头CMS? 让我知道。
This definitely isn't a fad. It makes a lot of sense to me architecturally. Given the proliferation of "backend as a service" systems, DocumentDBs like Cosmos and Mongo, it follows that a headless CMS could easily fit into my systems. One less DB schema to think about, no need to roll my own auth/auth.
这绝对不是时尚。 从结构上来说,这对我来说很有意义。 随着“后端即服务”系统(如Cosmos和Mongo)的DocumentDB的泛滥,无头CMS可以轻松地放入我的系统中。 少考虑一个数据库架构,无需滚动我自己的auth / auth。
*Photo "headless" by Wendy used under CC https://flic.kr/p/HkESxW
*由温迪在CC https://flic.kr/p/HkESxW下使用的“无头”照片
Sponsor: Telerik DevCraft is the comprehensive suite of .NET and JavaScript components and productivity tools developers use to build high-performant, modern web, mobile, desktop apps and chatbots. Try it!
赞助商: Telerik DevCraft是开发人员用来构建高性能,现代的Web,移动,桌面应用程序和聊天机器人的.NET和JavaScript组件以及生产力工具的综合套件。 试试吧!
翻译自: https://www.hanselman.com/blog/headless-cms-and-decoupled-cms-in-net-core
.net core cms