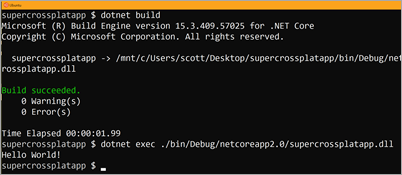
There's a couple of great utilities that have come out in the last few weeks in the .NET Core world that you should be aware of. They are deeply useful when porting/writing cross-platform code.
在过去的几周里,您应该意识到在.NET Core世界中出现了许多很棒的实用程序。 当移植/编写跨平台代码时,它们非常有用。
.NET API分析器 (.NET API Analyzer)
First is the API Analyzer. As you know, APIs sometimes get deprecated, or you'll use a method on Windows and find it doesn't work on Linux. The API Analyzer is a Roslyn (remember Roslyn is the name of the C#/.NET compiler) analyzer that's easily added to your project as a NuGet package. All you have to do is add it and you'll immediately start getting warnings and/or squiggles calling out APIs that might be a problem.
首先是API分析器。 如您所知,API有时会被弃用,或者您将在Windows上使用一种方法,但发现它在Linux上不起作用。 API分析器是一个Roslyn分析器(请记住Roslyn是C#/。NET编译器的名称)分析器,可以轻松地作为NuGet软件包添加到您的项目中。 您所要做的就是添加它,您将立即开始收到警告和/或花招,指出可能存在问题的API。
Check out this quick example. I'll make a quick console app, then add the analyzer. Note the version is current as of the time of this post. It'll change.
看看这个简单的例子。 我将制作一个快速的控制台应用程序,然后添加分析器。 请注意,截至本文发布时,该版本为最新版本。 会改变的。
C:\supercrossplatapp> dotnet new console
C:\supercrossplatapp> dotnet add package Microsoft.DotNet.Analyzers.Compatibility --version 0.1.2-alpha
Then I'll use an API that only works on Windows. However, I still want my app to run everywhere.
然后,我将使用仅适用于Windows的API。 但是,我仍然希望我的应用可以在任何地方运行。
static void Main(string[] args)
{
Console.WriteLine("Hello World!");
if (RuntimeInformation.IsOSPlatform(OSPlatform.Windows))
{
var w = Console.WindowWidth;
Console.WriteLine($"Console Width is {w}");
}
}
Then I'll "dotnet build" (or run, which implies build) and I get a nice warning that one API doesn't work everywhere.
然后,我将“ dotnet构建”(或运行,这意味着构建),并且我得到一个很好的警告,即一个API并非在所有地方都有效。
C:\supercrossplatapp> dotnet build
Program.cs(14,33): warning PC001: Console.WindowWidth isn't supported on Linux, MacOSX [C:\Users\scott\Desktop\supercr
ossplatapp\supercrossplatapp.csproj]
supercrossplatapp -> C:\supercrossplatapp\bin\Debug\netcoreapp2.0\supercrossplatapp.dll
Build succeeded.
Olia from the .NET Team did a great YouTube video where she shows off the API Analyzer and how it works. The code for the API Analyzer up here on GitHub. Please leave an issue if you find one!
.NET团队的Olia制作了一段很棒的YouTube视频,向她展示了API分析器及其工作原理。 GitHub上的API分析器代码。 如果找到一个,请留下问题!
.NET Core的Windows兼容包 (Windows Compatibility Pack for .NET Core)
Second, the Windows Compatibility Pack for .NET Core is a nice piece of tech. When .NET Core 2.0 come out and the .NET Standard 2.0 was finalized, it included over 32k APIs that made it extremely compatible with existing .NET Framework code. In fact, it's so compatible, I was able to easily take a 15 year old .NET app and port it over to .NET Core 2.0 without any trouble at all.
其次, .NET Core的Windows兼容包是一项不错的技术。 当.NET Core 2.0发布并最终确定.NET Standard 2.0时,它包括超过32k的API,这使其与现有.NET Framework代码极为兼容。 实际上,它是如此兼容,我能够轻松地使用一个已有15年历史的.NET应用程序并将其移植到.NET Core 2.0,而没有任何麻烦。
They have more than doubled the set of available APIs from 13k in .NET Standard 1.6 to 32k in .NET Standard 2.0.
从.NET Standard 1.6中的13k到.NET Standard 2.0中的32k ,它们使可用API的集合增加了一倍以上。
.NET Standard 2.0 is cool because it's supported on the following platforms:
.NET Standard 2.0很酷,因为它在以下平台上受支持:
- .NET Framework 4.6.1 .NET Framework 4.6.1
- .NET Core 2.0 .NET Core 2.0
- Mono 5.4 单声道5.4
- Xamarin.iOS 10.14 Xamarin.iOS 10.14
- Xamarin.Mac 3.8 Xamarin.Mac 3.8
- Xamarin.Android 7.5 Xamarin.Android 7.5
When you're porting code over to .NET Core that has lots of Windows-specific dependencies, you might find yourself bumping into APIs that aren't a part of .NET Standard 2.0. So, there's a new (preview) Microsoft.Windows.Compatibility NuGet package that "provides access to APIs that were previously available only for .NET Framework."
当您将代码移植到具有许多Windows特定依赖项的.NET Core时,您可能会发现自己陷入了.NET Standard 2.0不包含的API中。 因此,有一个新的(预览版) Microsoft.Windows.Compatibility NuGet程序包,它“提供对以前仅可用于.NET Framework的API的访问”。
There will be two kinds of APIs in the Compatibility Pack. APIs that were a part of Windows originally but can work cross-platform, and APIs that will always be Windows only, because they are super OS-specific. APIs calls to the Windows Registry will always be Windows-specific, for example. But the System.DirectoryServices or System.Drawing APIs could be written in a way that works anywhere. The Windows Compatibility Pack adds over 20,000 more APIs, on top of what's already available in .NET Core. Check out the great video that Immo shot on the compat pack.
兼容性包中将包含两种API。 API最初是Windows的一部分,但可以跨平台工作,而API始终仅是Windows,因为它们是特定于超级操作系统的。 例如,对Windows注册表的API调用将始终是特定于Windows的。 但是System.DirectoryServices或System.Drawing API可以在任何地方都可以使用的方式编写。 除了.NET Core中已提供的功能外, Windows兼容性包还增加了20,000多个API 。 看看Immo在compat包装上拍摄的精彩视频。
The point is, if the API that is blocking you from using .NET Core is now available in this compat pack, yay! But you should also know WHY you are pointing to .NET Core. Work continues on both .NET Core and .NET (Full) Framework on Windows. If your app works great today, there's no need to port unless you need a .NET Core specific feature. Here's a great list of rules of thumb from the docs:
问题的关键是,如果从使用.NET的核心阻碍了你的API是现在在这个compat的包可用,耶! 但是您也应该知道为什么要指向.NET Core。 Windows上的.NET Core和.NET(完整)框架都将继续工作。 如果您的应用程序今天运行良好,则无需移植,除非您需要特定于.NET Core的功能。 这是来自文档的大量经验法则列表:
Use .NET Core for your server application when:+
在以下情况下对服务器应用程序使用.NET Core: +
You have cross-platform needs.
您有跨平台的需求。
You are targeting microservices.
您正在针对微服务。
You are using Docker containers.
您正在使用Docker容器。
You need high-performance and scalable systems.
您需要高性能和可扩展的系统。
You need side-by-side .NET versions per application.
每个应用程序需要并行的.NET版本。
Use .NET Framework for your server application when:
在以下情况下,将.NET Framework用于服务器应用程序:
Your app currently uses .NET Framework (recommendation is to extend instead of migrating).
您的应用程序当前使用.NET Framework(建议扩展而不是迁移)。
Your app uses third-party .NET libraries or NuGet packages not available for .NET Core.
您的应用程序使用了.NET Core不可用的第三方.NET库或NuGet软件包。
Your app uses .NET technologies that aren't available for .NET Core.
您的应用使用了.NET Core无法使用的.NET技术。
Your app uses a platform that doesn’t support .NET Core.
您的应用程序使用的平台不支持.NET Core。
Finally, it's worth pointing out a few other tools that can aid you in using the right APIs for the job.
最后,值得指出一些其他工具,这些工具可以帮助您使用正确的API来完成工作。
Api Portability Analyzer - command line tool or Visual Studio Extension, a toolchain that can generate a report of how portable your code is between .NET Framework and .NET Core, with an assembly-by-assembly breakdown of issues. See Tooling to help you on the process for more information.
Api可移植性分析器-命令行工具或Visual Studio Extension ,这是一个工具链,可以生成有关代码在.NET Framework和.NET Core之间的可移植性的报告,并逐项分解问题。 有关更多信息,请参见工具以帮助您完成该过程。
Reverse Package Search - A useful web service that allows you to search for a type and find packages containing that type.
反向软件包搜索-有用的Web服务,允许您搜索类型并查找包含该类型的软件包。
Enjoy!
请享用!
Sponsor: Get the latest JetBrains Rider preview for .NET Core 2.0 support, Value Tracking and Call Tracking, MSTest runner, new code inspections and refactorings, and the Parallel Stacks view in debugger.
赞助商:获取有关.NET Core 2.0支持,值跟踪和呼叫跟踪,MSTest运行器,新代码检查和重构以及调试器中的“并行堆栈”视图的最新JetBrains Rider预览。