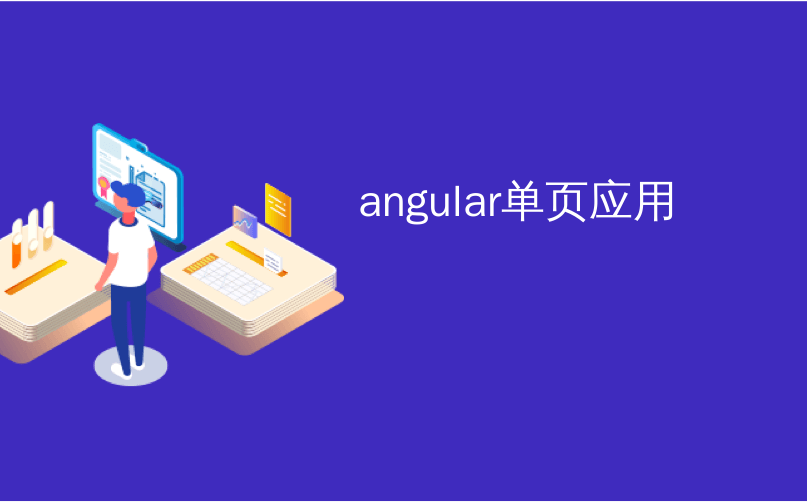
angular单页应用
I was doing some Angular then remembered that the ASP.NET "Angular Project Template" has a release candidate and is scheduled to release sometime soon in 2018.
我当时在做一些Angular,然后想起ASP.NET“ Angular Project Template”有一个候选发布版本,并计划于2018年某个时候发布。
Starting with just a .NET Core 2.0 install plus Node v6 or later, I installed the updated angular template. Note that this isn't the angular/react/redux templates that came with .NET Core's base install.
从仅安装.NET Core 2.0以及Node v6或更高版本开始,我安装了更新的角度模板。 请注意,这不是.NET Core基本安装随附的angular / react / redux模板。
I'll start by adding the updated SPA (single page application) template:
我将从添加更新的SPA(单页应用程序)模板开始:
dotnet new --install Microsoft.DotNet.Web.Spa.ProjectTemplates::2.0.0-rc1-final
Then from a new directory, just
然后从一个新目录
dotnet new angular
Then I can open it in either VSCode or Visual Studio Community (free for Open Source). If you're interested in the internals, open up the .csproj project file and note the checks for ensuring node is install, running npm, and running WebPack.
然后,我可以在VSCode或Visual Studio社区(对于开源免费)中打开它。 如果您对内部结构感兴趣,请打开.csproj项目文件,并注意检查以确保节点已安装,正在运行npm和正在运行WebPack。
If you've got the Angular "ng" command line tool installed you can do the usual ng related stuff, but you don't need to run "ng serve" because ASP.NET Core will run it automatically for you.
如果您已经安装了Angular“ ng”命令行工具,则可以执行与ng相关的常规操作,但是您无需运行“ ng serve”,因为ASP.NET Core会自动为您运行它。
I set development mode with "SET ASPNETCORE_Environment=Development" then do a "dotnet build." It will also restore your npm dependencies as part of the build. The client side app lives in ./ClientApp.
我使用“ SET ASPNETCORE_Environment = Development”设置开发模式,然后执行“ dotnet构建”。 它还将在构建过程中还原您的npm依赖项。 客户端应用程序位于./ClientApp中。
C:\Users\scott\Desktop\my-new-app> dotnet build
Microsoft (R) Build Engine version 15.5 for .NET Core
Copyright (C) Microsoft Corporation. All rights reserved.
Restore completed in 73.16 ms for C:\Users\scott\Desktop\my-new-app\my-new-app.csproj.
Restore completed in 99.72 ms for C:\Users\scott\Desktop\my-new-app\my-new-app.csproj.
my-new-app -> C:\Users\scott\Desktop\my-new-app\bin\Debug\netcoreapp2.0\my-new-app.dll
v8.9.4
Restoring dependencies using 'npm'. This may take several minutes...
"dotnet run" then starts the ng development server and ASP.NET all at once.
然后“ dotnet run”立即启动ng开发服务器和ASP.NET。
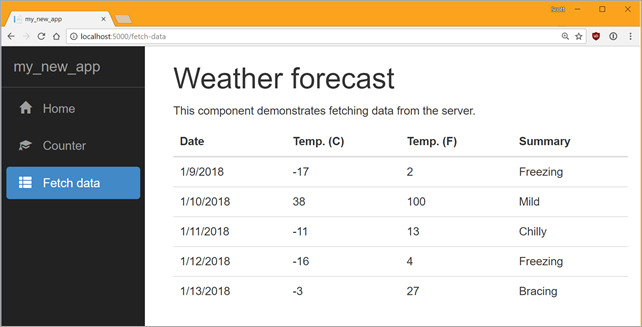
If we look at the "Fetch Data" menu item, you can see and example of how Angular and open source ASP.NET Core work together. Here's the Weather Forecast *client-side* template:
如果我们查看“获取数据”菜单项,则可以看到Angular和开源ASP.NET Core如何协同工作的示例。 这是天气预报*客户端*模板:
<p *ngIf="!forecasts"><em>Loading...</em></p>
<table class='table' *ngIf="forecasts">
<thead>
<tr>
<th>Date</th>
<th>Temp. (C)</th>
<th>Temp. (F)</th>
<th>Summary</th>
</tr>
</thead>
<tbody>
<tr *ngFor="let forecast of forecasts">
<td>{{ forecast.dateFormatted }}</td>
<td>{{ forecast.temperatureC }}</td>
<td>{{ forecast.temperatureF }}</td>
<td>{{ forecast.summary }}</td>
</tr>
</tbody>
</table>
And the TypeScript:
和TypeScript:
import { Component, Inject } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-fetch-data',
templateUrl: './fetch-data.component.html'
})
export class FetchDataComponent {
public forecasts: WeatherForecast[];
constructor(http: HttpClient, @Inject('BASE_URL') baseUrl: string) {
http.get<WeatherForecast[]>(baseUrl + 'api/SampleData/WeatherForecasts').subscribe(result => {
this.forecasts = result;
}, error => console.error(error));
}
}
interface WeatherForecast {
dateFormatted: string;
temperatureC: number;
temperatureF: number;
summary: string;
}
Note the URL. Here's the back-end. The request is serviced by ASP.NET Core. Note the interface as well as the TemperatureF server-side conversion.
记下URL。 这是后端。 该请求由ASP.NET Core服务。 注意接口以及TemperatureF服务器端转换。
[Route("api/[controller]")]
public class SampleDataController : Controller
{
private static string[] Summaries = new[]
{
"Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching"
};
[HttpGet("[action]")]
public IEnumerable<WeatherForecast> WeatherForecasts()
{
var rng = new Random();
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
DateFormatted = DateTime.Now.AddDays(index).ToString("d"),
TemperatureC = rng.Next(-20, 55),
Summary = Summaries[rng.Next(Summaries.Length)]
});
}
public class WeatherForecast
{
public string DateFormatted { get; set; }
public int TemperatureC { get; set; }
public string Summary { get; set; }
public int TemperatureF
{
get
{
return 32 + (int)(TemperatureC / 0.5556);
}
}
}
}
非常干净和直接。 不确定Date.Now,但是在大多数情况下,我理解了这一点,并且可以看到如何扩展它。 查看有关此发行候选版本的文档,还请注意,该文档还包括更新的React和Redux模板!
Sponsor: Scale your Python for big data & big science with Intel® Distribution for Python. Near-native code speed. Use with NumPy, SciPy & scikit-learn. Get it Today!
赞助商:借助适用于Python的英特尔®发行版,将Python扩展到大数据和大科学领域。 接近本机的代码速度。 与NumPy,SciPy和scikit-learn一起使用。 立即获取!
翻译自: https://www.hanselman.com/blog/aspnet-single-page-applications-angular-release-candidate
angular单页应用