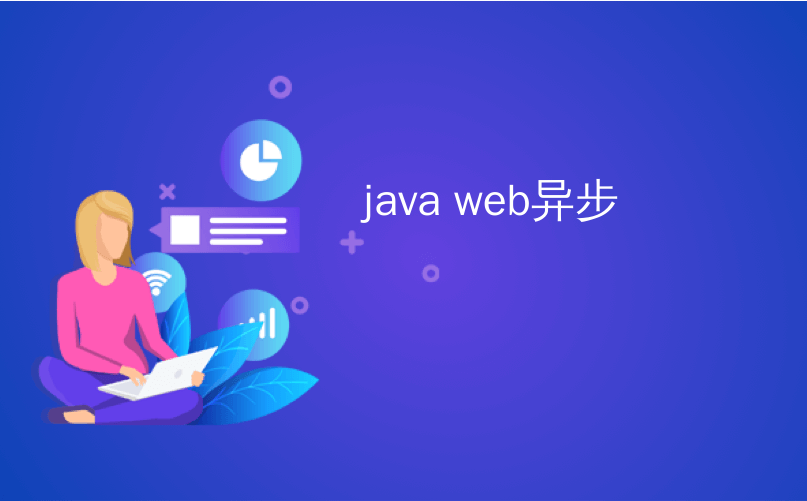
java web异步
I've been spending some time exploring asynchrony and scale recently. You may have seen my post about my explorations with node.js and iisnode running node on Windows.
最近,我一直在花一些时间探索异步和扩展。 您可能已经看过我关于在Windows上运行node.js和iisnode进行探索的文章。
Every application has different requirements such that rules to "make it scale" don't work for every kind of application. Scaling a web app that gets some data and for loops over it is different from an app that calls out to a high-latency mainframe is different from an app that needs to maintain a persistent connection to the server.
每个应用程序都有不同的要求,因此“使其扩展”的规则不适用于每种类型的应用程序。 缩放可获取一些数据并进行循环访问的Web应用程序,不同于调用高延迟大型机的应用程序,与需要保持与服务器的持久连接的应用程序不同。
The old adage "when all you have it is a hammer everything looks like a nail" really holds true in the programming and web space. The more tools - and the knowledge to use them - the better. That's why I'm an advocate not only of polyglot programming but also of going deep with your main languages. When you really learn LINQ for example and get really good at dynamics, C# becomes a much more fun and expressive language.
古老的格言“当您拥有的只是一把锤子时,一切看起来都像钉子”在编程和网站空间中确实适用。 工具越多-以及使用它们的知识-越好。 这就是为什么我不仅提倡多种语言编程,而且还提倡使用您的主要语言的原因。 例如,当您真正学习LINQ并真正掌握动态知识时,C#就会变得更加有趣和富有表现力。
Polling is a common example of hammering a screw. Trying to make a chat program? Poll every 5 seconds. Got a really long running transaction? Throw up an animated GIF and poll until eternity, my friend!
轮询是锤击螺丝的常见示例。 尝试制作聊天程序? 每5秒钟轮询一次。 有一个很长时间的交易吗? 扔动画GIF并投票直到永恒,我的朋友!
Long polling is another way to get things done. Basically open a connection and keep it open, forcing the client (browser) to wait, pretending it's taking a long time to return. If you have enough control on your server-side programming model, this can allow you to return data as you like over this "open connection." If the connection breaks, it's transparently re-opened and the break is hidden from both sides. In the future things like WebSockets will be another way to solve this problem when it's baked.
长轮询是完成工作的另一种方法。 基本上是打开一个连接并使其保持打开状态,从而迫使客户端(浏览器)等待,假装连接返回需要很长时间。 如果您对服务器端编程模型有足够的控制权,则可以通过此“打开的连接”随意返回数据。 如果连接断开,则会透明地重新打开该连接,并且断开从两侧都隐藏。 将来, WebSockets之类的东西将成为解决此问题的另一种方法。
ASP.NET中的持久连接 (Persistent Connections in ASP.NET)
Doing this kind of persistent connection in a chat application or stock ticker for example hasn't been easy in ASP.NET. There hasn't been a decent abstraction for this on the server or a client library to talk to it.
例如,在聊天应用程序或股票报价器中进行这种持久连接在ASP.NET中并非易事。 在服务器或客户端库上还没有与之对话的体面抽象。
SignalR is an asynchronous signaling library for ASP.NET that our team is working on to help build real-time multi-user web application.
SignalR是ASP.NET的异步信令库,我们的团队正在研究该库以帮助构建实时的多用户Web应用程序。
这不只是Socket.IO或nowjs吗? (Isn't this just Socket.IO or nowjs?)
Socket.IO is a client side JavaScript library that talks to node.js. Nowjs is a library that lets you call the client from the server. All these and Signalr are similar and related, but different perspectives on the same concepts. Both these JavaScript libraries expect certain things and conventions on the server-side, so it's probably possible to make the server look the way these clients would want it to look if one wanted.
Socket.IO是与node.js对话的客户端JavaScript库。 Nowjs是一个库,可让您从服务器调用客户端。 所有这些和Signalr都是相似和相关的,但是对相同概念的观点不同。 这两个JavaScript库都希望服务器端具有某些功能和约定,因此,有可能使服务器看起来像这些客户端希望的那样(如果有人愿意)。
SignalR is a complete client- and server-side solution with JS on client and ASP.NET on the back end to create these kinds of applications. You can get it up on GitHub.
SignalR是一个完整的客户端和服务器端解决方案,在客户端使用JS,在后端使用ASP.NET来创建此类应用程序。 您可以在GitHub上进行安装。
但是我可以用12行代码制作一个聊天应用程序吗? (But can I make a chat application in 12 lines of code?)
I like to say
我想说
"In code, any sufficient level of abstraction is indistinguishable from magic."
“在代码中,任何足够的抽象层次都无法与魔术区分开。 ”
That said, I suppose I could just say, sure!
就是说,我想我只能说,确定!
Chat.DoItBaby()
But that would be a lie. Here's a real chat application in SignalR for example:
但这将是一个谎言。 例如,这是SignalR中一个真正的聊天应用程序:
Client:
客户:
var chat = $.connection.chat;
chat.name = prompt("What's your name?", "");
chat.receive = function(name, message){
$("#messages").append("
"+name+": "+message);
}
$("#send-button").click(function(){
chat.distribute($("#text-input").val());
});
Server:
服务器:
public class Chat : Hub {
public void Distribute(string message) {
Clients.receive(Caller.name, message);
}
}
That's maybe 12, could be 9, depends on how you roll.
那可能是12,可能是9,取决于您的滚动方式。
有关SignalR的更多详细信息 (More details on SignalR)
SignalR is broken up into a few package on NuGet:
SignalR在NuGet上分为几个软件包:
SignalR - A meta package that brings in SignalR.Server and SignalR.Js (you should install this)
SignalR-引入SignalR.Server和SignalR.Js的元软件包(您应该安装此软件包)
SignalR.Server - Server side components needed to build SignalR endpoints
SignalR.Server-构建SignalR端点所需的服务器端组件
SignalR.Js - Javascript client for SignalR
SignalR.Js - SignalR的Javascript客户端
SignalR.Client - .NET client for SignalR
SignalR.Client - SignalR的.NET客户端
SignalR.Ninject - Ninject dependeny resolver for SignalR
SignalR.Ninject -SignalR的非依赖注入解析器
If you just want to play and make a small up, start up Visual Studio 2010.
如果您只想玩游戏并做些小巧的东西,请启动Visual Studio 2010。
First, make an Empty ASP.NET application, and install-package SignalR with NuGet, either with the UI or the Package Console.
首先,制作一个空的ASP.NET应用程序,并使用UI或程序包控制台安装带有NuGet的SignalR程序包。
Second, create a new default.aspx page and add a button, a textbox, references to jQuery and jQuery.signalR along with this script.
其次,创建一个新的default.aspx页面,并添加一个按钮,一个文本框,对jQuery和jQuery.signalR的引用以及此脚本。
低层连接(Low Level Connection)
Notice we're calling /echo from the client? That is hooked up in routing in Global.asax:
注意我们正在从客户端调用/ echo吗? 这与Global.asax中的路由相关:
RouteTable.Routes.MapConnection
("echo", "echo/{*operation}");
At this point, we've got two choices of models with SignalR. Let's look at the low level first.
至此,我们有了SignalR的两种型号选择。 让我们先看一下低层次。
using SignalR;
using System.Threading.Tasks;
public class MyConnection : PersistentConnection
{
protected override Task OnReceivedAsync(string clientId, string data)
{
// Broadcast data to all clients
return Connection.Broadcast(data);
}
}
We derive from PersistentConnection and can basically do whatever we want at this level. There's lots of choices:
我们从PersistentConnection派生而来,基本上可以在此级别上完成任何我们想做的事情。 有很多选择:
public abstract class PersistentConnection : HttpTaskAsyncHandler, IGroupManager
{
protected ITransport _transport;
protected PersistentConnection();
protected PersistentConnection(Signaler signaler, IMessageStore store, IJsonStringifier jsonStringifier);
public IConnection Connection { get; }
public override bool IsReusable { get; }
public void AddToGroup(string clientId, string groupName);
protected virtual IConnection CreateConnection(string clientId, IEnumerable
groups, HttpContextBase context);
protected virtual void OnConnected(HttpContextBase context, string clientId);
protected virtual Task OnConnectedAsync(HttpContextBase context, string clientId);
protected virtual void OnDisconnect(string clientId);
protected virtual Task OnDisconnectAsync(string clientId);
protected virtual void OnError(Exception e);
protected virtual Task OnErrorAsync(Exception e);
protected virtual void OnReceived(string clientId, string data);
protected virtual Task OnReceivedAsync(string clientId, string data);
public override Task ProcessRequestAsync(HttpContext context);
public void RemoveFromGroup(string clientId, string groupName);
public void Send(object value);
public void Send(string clientId, object value);
public void SendToGroup(string groupName, object value);
}
高级集线器 (High Level Hub)
Or, we can take it up a level and just do this for our chat client after adding
或者,我们可以将其升级,然后在添加后为我们的聊天客户端执行此操作
<script src="/signalr/hubs" type="text/javascript"></script>
to our page.
到我们的页面。
$(function () {
// Proxy created on the fly
var chat = $.connection.chat;
// Declare a function on the chat hub so the server can invoke it
chat.addMessage = function (message) {
$('#messages').append('
' + message + '');
'+消息+'');
};
};
$("#broadcast").click(function () {
$(“#broadcast”)。click(function(){
// Call the chat method on the server
//在服务器上调用chat方法
chat.send($('#msg').val());
chat.send($('#msg')。val());
});
});
// Start the connection
//开始连接
$.connection.hub.start();
$ .connection.hub.start();
});
});
Then there is no need for routing and the connection.chat will map to this on the server, and the server can then call the client back.
这样就不需要路由,并且connection.chat将映射到服务器上的该地址,然后服务器可以回叫客户端。
public class Chat : Hub
{
public void Send(string message)
{
// Call the addMessage method on all clients
Clients.addMessage(message);
}
}At this point your brain should have exploded and leaked out of your ears. This is C#, server-side code and we're telling all the clients to call the addMessage() JavaScript function. We're calling the client back from the server by sending the name of the client method to call down from the server via our persistent connection. It's similar to NowJS but not a lot of people are familiar with this technique.
此时,您的大脑应该已经爆炸并从您的耳朵漏出了。 这是C#服务器端代码,我们正在告诉所有客户端调用addMessage()JavaScript函数。 我们正在通过发送客户端方法的名称从服务器回调客户端,该方法通过我们的持久连接从服务器进行调用。 它与NowJS相似,但并不是很多人都熟悉这种技术。
SignalR will handle all the connection stuff on both client and server, making sure it stays open and alive. It'll use the right connection for your browser and will scale on the server with async and await techniques (like I talked about in the node.js post where I showed scalable async evented I/O on asp.net).
SignalR将处理客户端和服务器上的所有连接内容,并确保其保持打开状态和活动状态。 它将为您的浏览器使用正确的连接,并将通过异步和等待技术在服务器上扩展(就像我在node.js帖子中谈到的那样,我在asp.net上展示了可伸缩的异步事件I / O )。
是否希望看到此示例实时运行? (Want to see this sample running LIVE? )
We've got a tiny tiny chat app running on Azure over at http://jabbr.net/, so go beat on it. There are folks in /join aspnet. Try pasting in YouTube links or images!
我们在http://jabbr.net/上的Azure上运行了一个微型聊天应用程序,请继续关注它。 / join aspnet中有个人。 尝试粘贴YouTube链接或图像!
It's early, but it's an interesting new LEGO piece for .NET that didn't completely exist before. Feel free to check it out on GitHub and talk to the authors of SignalR, David Fowler and Damian Edwards. Enjoy.
还很早,但这是一个有趣的新乐高积木。NET之前还不完全存在。 随时在GitHub上进行检查,并与SignalR,David Fowler和Damian Edwards的作者进行交谈。 请享用。
java web异步