webkit
Under certain conditions, Webkit will not repaint the browser window to reflect DOM additions or changes. This is a known bug: in the case of Wednesday’s article on making an automatic image checkerboard with SVG and JavaScript, switching from rectangular to circular clipping areas in Chrome will produce an effect that looks like Figure 1:
在某些情况下,Webkit不会重绘浏览器窗口以反映DOM的添加或更改。 这是一个已知的错误:在周三的有关使用SVG和JavaScript制作自动图像棋盘的文章的情况下,在Chrome中从矩形剪切区域切换为圆形剪切区域将产生如图1所示的效果:
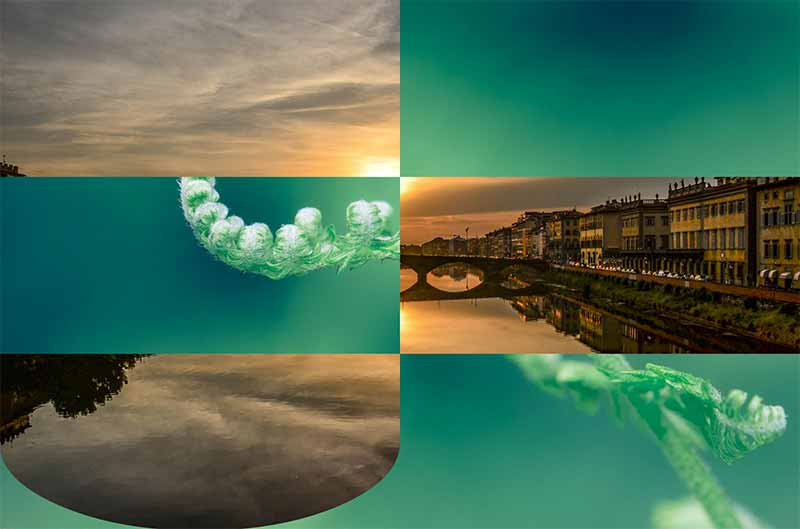
To address this in the CodePen version, I’ve added four lines to the end of the script:
为了在CodePen版本中解决此问题,我在脚本末尾添加了四行:
var bridge = document.querySelector("#clipit img");
bridge.style.display="none";
bridge.offsetHeight;
bridge.style.display="block";
This finds the image, quickly hides it, calculates its current height, and then sets it back to display: block
. For all purposes this happens instantaneously, so there is no visual “flicker” in the browser. There are just two things to note:
这将找到图像,快速将其隐藏,计算其当前高度,然后将其设置回display: block
。 出于所有目的,这是瞬间发生的,因此浏览器中没有视觉“闪烁”。 有两件事要注意:
We don’t have to store
offsetHeight
; referencing it is enough to give Webkit the equivalent of a smack upside the head.我们不必存储
offsetHeight
; 引用它就足以使Webkit头晕目眩。We reset the image to which the SVG is applied, not the SVG itself.
我们重置要应用SVG的图像 ,而不是SVG本身。
其他解决方案 (Other Solutions)
This is not an absolute suggestion, just the best one I’ve found. However, it may force both a reflow and repaint, making Webkit work overtime. There are some other solutions that may be more effective in certain cases:
这不是绝对的建议,只是我发现的最好的建议。 但是,这可能会强制重排和重绘,从而使Webkit超时工作。 在某些情况下,还有其他一些可能更有效的解决方案:
Adding an empty
<style></style>
tag after the script; either dynamically with JavaScript or writing it directly into the code of the page.在脚本之后添加一个空的
<style></style>
标记; 可以使用JavaScript动态生成,也可以直接将其写入页面代码中。Adding a transform that will not otherwise alter the appearance of the element:
添加一个不会改变元素外观的转换:
element.style.webkitTransform = "scale(1)";
Or in CSS:
或在CSS中:
.quickfix { transform: translateZ(0); }
If the element is absolutely positioned: adding a
z-index
value to the element can force a repaint:如果该元素绝对定位:向该元素添加
z-index
值可以强制重新绘制:element.style.zIndex = "2";
This fix is not needed in every case, and I hope it will be unnecessary in the future, but right now when you need it, you really need it.
并非在每种情况下都需要此修复程序,我希望将来不再需要此修复程序,但是现在当您需要它时,您确实需要它。
webkit