stoul() 函数
#include <string>
unsigned long stoul(const std::string& str, std::size_t* pos = 0, int base = 10);
unsigned long stoul(const std::wstring& str, std::size_t* pos = 0, int base = 10);
功能:将字符串 str 转成 unsigned long 整数
参数:
str:字符串
pos:存储将字符串 str 转成 unsigned long 整数,处理了 str 中字符的个数的地址,默认为 NULL
base:进制,10:十进制,8:八进制,16:十六进制,0:则自动检测数值进制,str 是 0 开头为八进制,str 是 0x 或 0X 开头是十六进制,默认为十进制
stoul() 函数指定转换字符串为十进制用法
stoul.cpp
#include <iostream>
#include <string>
#include <limits.h>
using namespace std;
int main(int argc, char *argv[])
{
unsigned long a; //x86_64构架下,unsigned long 8个字节
size_t pos = 0;
string str;
cout << "ULONG_MAX = " << ULONG_MAX << endl;
str = "-1";
a = stoul(str); //数据在内存中是以补码的形式存储的,负数的补码等于反码加1
/*
-1的原码是:10000000,00000000,00000000,00000000,00000000,00000000,00000000,00000001
-1的反码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111110
-1的补码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111111
*/
cout << "a = " << a << endl; // a = (最大值) +(负数)+ 1,即:((2^64) - 1) + (-1) + 1
str = "1235";
a = stoul(str);
cout << "a = " << a << endl; //a = 1235
str = " -12 35";
a = stoul(str, &pos); //会舍弃空白符
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-12) + 1
cout << "pos = " << pos << endl; //pos = 5
str = " -12ab35";
a = stoul(str, &pos);
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-12) + 1
cout << "pos = " << pos << endl; //pos = 5
str = "0123";
a = stoul(str);
cout << "a = " << a << endl; //a = 123
str = "0x123";
a = stoul(str);
cout << "a = " << a << endl; //a = 0
return 0;
}
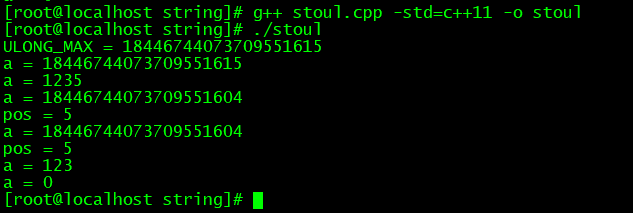
stoul() 函数指定转换字符串为十六进制用法
stoul.cpp
#include <iostream>
#include <string>
#include <limits.h>
using namespace std;
int main(int argc, char *argv[])
{
unsigned long a; //x86_64构架下,unsigned long 8个字节
size_t pos = 0;
string str;
cout << "ULONG_MAX = " << ULONG_MAX << endl;
/*
数据在内存中是以补码的形式存储的,负数的补码等于反码加1
-1的原码是:10000000,00000000,00000000,00000000,00000000,00000000,00000000,00000001
-1的反码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111110
-1的补码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111111
-1转成unsigned long:(最大值) +(负数)+ 1
-1转成unsigned long:((2^64) - 1) + (-1) + 1
*/
str = "0x123";
a = stoul(str, NULL, 16); //base = 16,指定十六进制
cout << "a = " << a << endl; //a = 3 + 2*16 + 1*16*16 = 291
str = "0x123";
a = stoul(str, NULL, 0); //base = 0,自动检测数值进制
cout << "a = " << a << endl; //a = 291
str = " -12";
a = stoul(str, &pos, 16); //会舍弃空白符
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-(2 + 1*16)) + 1
cout << "pos = " << pos << endl; //pos = 5
str = " -12 35";
a = stoul(str, &pos, 16);
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-(2 + 1*16)) + 1
cout << "pos = " << pos << endl; //pos = 5
str = " -ab";
a = stoul(str, NULL, 16);
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-(11 + 10*16)) + 1
str = "0123";
a = stoul(str, NULL, 16);
cout << "a = " << a << endl; //a = (3 + 2*16 + 1*16*16) = 291
return 0;
}
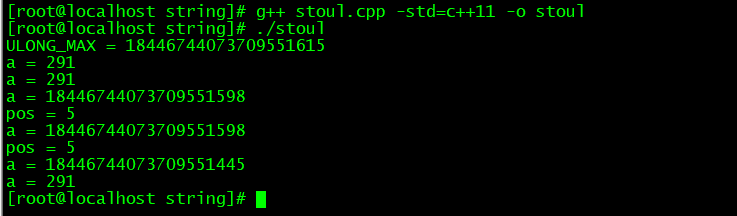
stoul() 函数指定转换字符串为八进制用法
stoul.cpp
#include <iostream>
#include <string>
#include <limits.h>
using namespace std;
int main(int argc, char *argv[])
{
unsigned long a; //x86_64构架下,unsigned long 8个字节
size_t pos = 0;
string str;
cout << "ULONG_MAX = " << ULONG_MAX << endl;
/*
数据在内存中是以补码的形式存储的,负数的补码等于反码加1
-1的原码是:10000000,00000000,00000000,00000000,00000000,00000000,00000000,00000001
-1的反码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111110
-1的补码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111111
-1转成unsigned long:(最大值) +(负数)+ 1
-1转成unsigned long:((2^64) - 1) + (-1) + 1
*/
str = "0x123";
a = stoul(str, NULL, 8); //base = 8,指定八进制
cout << "a = " << a << endl; //a = 0
str = "0123"; //(3 + 2*8 + 1*8*8)
a = stoul(str, NULL, 0); //base = 0,自动检测数值进制
cout << "a = " << a << endl; //a = (3 + 2*8 + 1*8*8) = 83
str = "-12";
a = stoul(str, &pos, 8); //-(2 + 1*8)
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-10) + 1
cout << "pos = " << pos << endl; //pos = 3
str = "12";
a = stoul(str, &pos, 8); //2 + 1*8
cout << "a = " << a << endl; //a = 10
cout << "pos = " << pos << endl; //pos = 2
str = " -12 35";
a = stoul(str, &pos, 8); //会舍弃空白符
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-10) + 1
cout << "pos = " << pos << endl; //pos = 5
// str = " -a78";
// a = stol(str, &pos, 8); //数字前有字母,调用会崩掉
// cout << "a = " << a << endl;
// cout << "pos = " << pos << endl;
return 0;
}
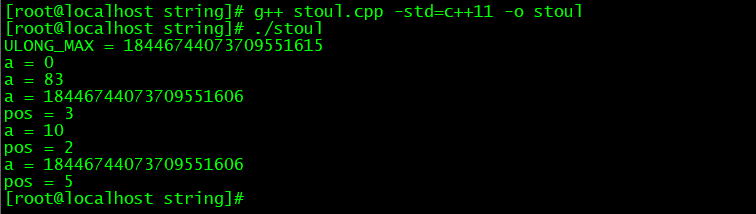
stoull() 函数
#include <string>
unsigned long long stoull(const std::string& str, std::size_t* pos = 0, int base = 10);
unsigned long long stoull(const std::wstring& str, std::size_t* pos = 0, int base = 10);
功能:将字符串 st r转成 unsigned long long 整数
参数:
str:字符串
pos:存储将字符串 str 转成 unsigned long long 整数,处理了 str 中字符的个数的地址,默认为 NULL
base:进制,10:十进制,8:八进制,16:十六进制,0:则自动检测数值进制,str 是 0 开头为八进制,str 是 0x 或 0X 开头是十六进制,默认为十进制
stoull() 函数指定转换字符串为十进制用法
stoull.cpp
#include <iostream>
#include <string>
#include <limits.h>
using namespace std;
int main(int argc, char *argv[])
{
unsigned long long a; //x86_64构架下,unsigned long long 8个字节
size_t pos = 0;
string str;
cout << "ULLONG_MAX = " << ULLONG_MAX << endl;
str = "-1";
a = stoull(str); //数据在内存中是以补码的形式存储的,负数的补码等于反码加1
/*
-1的原码是:10000000,00000000,00000000,00000000,00000000,00000000,00000000,00000001
-1的反码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111110
-1的补码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111111
*/
cout << "a = " << a << endl; // a = (最大值) +(负数)+ 1,即:((2^64) - 1) + (-1) + 1
str = "1235";
a = stoull(str);
cout << "a = " << a << endl; //a = 1235
str = " -12 35";
a = stoull(str, &pos); //会舍弃空白符
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-12) + 1
cout << "pos = " << pos << endl; //pos = 5
str = " -12ab35";
a = stoull(str, &pos);
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-12) + 1
cout << "pos = " << pos << endl; //pos = 5
str = "0123";
a = stoull(str);
cout << "a = " << a << endl; //a = 123
str = "0x123";
a = stoull(str);
cout << "a = " << a << endl; //a = 0
return 0;
}
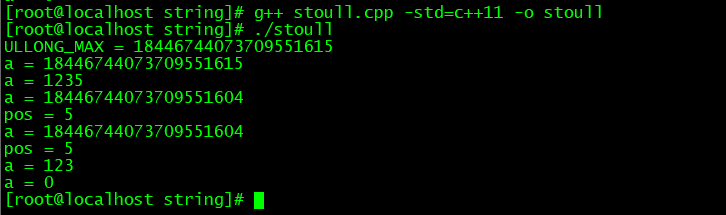
stoull() 函数指定转换字符串为十六进制用法
stoull.cpp
#include <iostream>
#include <string>
#include <limits.h>
using namespace std;
int main(int argc, char *argv[])
{
unsigned long long a; //x86_64构架下,unsigned long long 8个字节
size_t pos = 0;
string str;
cout << "ULLONG_MAX = " << ULLONG_MAX << endl;
/*
数据在内存中是以补码的形式存储的,负数的补码等于反码加1
-1的原码是:10000000,00000000,00000000,00000000,00000000,00000000,00000000,00000001
-1的反码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111110
-1的补码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111111
-1转成unsigned long long:(最大值) +(负数)+ 1
-1转成unsigned long long:((2^64) - 1) + (-1) + 1
*/
str = "0x123";
a = stoull(str, NULL, 16); //base = 16,指定十六进制
cout << "a = " << a << endl; //a = 3 + 2*16 + 1*16*16 = 291
str = "0x123";
a = stoull(str, NULL, 0); //base = 0,自动检测数值进制
cout << "a = " << a << endl; //a = 291
str = " -12";
a = stoull(str, &pos, 16); //会舍弃空白符
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-(2 + 1*16)) + 1
cout << "pos = " << pos << endl; //pos = 5
str = " -12 35";
a = stoull(str, &pos, 16);
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-(2 + 1*16)) + 1
cout << "pos = " << pos << endl; //pos = 5
str = " -ab";
a = stoull(str, NULL, 16);
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-(11 + 10*16)) + 1
str = "0123";
a = stoull(str, NULL, 16);
cout << "a = " << a << endl; //a = (3 + 2*16 + 1*16*16) = 291
return 0;
}
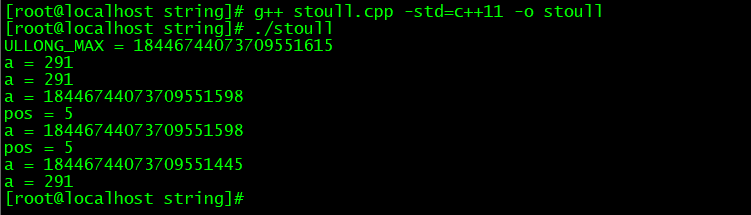
stoull() 函数指定转换字符串为八进制用法
stoul.cpp
#include <iostream>
#include <string>
#include <limits.h>
using namespace std;
int main(int argc, char *argv[])
{
unsigned long long a; //x86_64构架下,unsigned long long 8个字节
size_t pos = 0;
string str;
cout << "ULLONG_MAX = " << ULLONG_MAX << endl;
/*
数据在内存中是以补码的形式存储的,负数的补码等于反码加1
-1的原码是:10000000,00000000,00000000,00000000,00000000,00000000,00000000,00000001
-1的反码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111110
-1的补码是:11111111,11111111,11111111,11111111,11111111,11111111,11111111,11111111
-1转成unsigned long long:(最大值) +(负数)+ 1
-1转成unsigned long long:((2^64) - 1) + (-1) + 1
*/
str = "0x123";
a = stoul(str, NULL, 8); //base = 8,指定八进制
cout << "a = " << a << endl; //a = 0
str = "0123"; //(3 + 2*8 + 1*8*8)
a = stoul(str, NULL, 0); //base = 0,自动检测数值进制
cout << "a = " << a << endl; //a = (3 + 2*8 + 1*8*8) = 83
str = "-12";
a = stoul(str, &pos, 8); //-(2 + 1*8)
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-10) + 1
cout << "pos = " << pos << endl; //pos = 3
str = "12";
a = stoul(str, &pos, 8); //2 + 1*8
cout << "a = " << a << endl; //a = 10
cout << "pos = " << pos << endl; //pos = 2
str = " -12 35";
a = stoul(str, &pos, 8); //会舍弃空白符
cout << "a = " << a << endl; //a = ((2^64) - 1) + (-10) + 1
cout << "pos = " << pos << endl; //pos = 5
// str = " -a78";
// a = stol(str, &pos, 8); //数字前有字母,调用会崩掉
// cout << "a = " << a << endl;
// cout << "pos = " << pos << endl;
return 0;
}
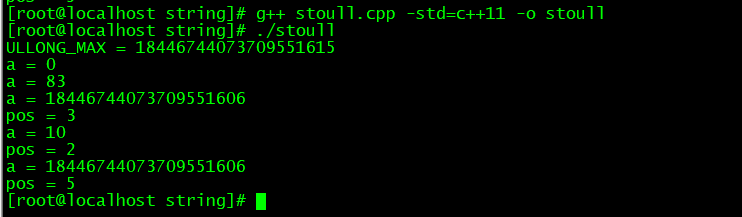
总结:
stoul 函数:将字符串转成 unsigned long 整数。
stoull 函数:将字符串转成 unsigned long long 整数。
使用时需要注意的是 stoul、stoull 函数是 C++11标准加入的,用 g++ 编译器编译时需要加参数:-std=c++11