WinUI3实现简易浏览器
包含UWP版
界面
winui3
XAML
<Rectangle Fill="{ThemeResource AcrylicInAppFillColorDefaultBrush}"/>
<Button x:Name="TurnLeft" Style="{StaticResource NavigationBackButtonSmallStyle}" Margin="10,10,0,0" VerticalAlignment="Top" Click="TurnLeft_Click" ToolTipService.ToolTip="Go back."/>
<Button x:Name="TurnRight" Style="{StaticResource NavigationBackButtonSmallStyle}" RenderTransformOrigin = "0.5,0.5" Margin="50,10,0,0" VerticalAlignment="Top" Click="TurnRight_Click" FlowDirection ="RightToLeft" ToolTipService.ToolTip="Go Forward."/>
<Button x:Name="ReloadBtn" VerticalAlignment="Top" FontSize="14" Margin="85,10,0,0" HorizontalAlignment="Left" Click="ReloadBtn_Click" ToolTipService.ToolTip="Refresh."/>
<WebView2 x:Name="HtmlWebViewSource" Source="https://baidu.com" Margin="0,55,0,0" Grid.ColumnSpan="2" NavigationCompleted="WebviewNavigated" NavigationStarting="WebviewNavigating"/>
<ProgressBar x:Name="WebProgressBar" Value="100" IsIndeterminate="False" ShowError="False" VerticalAlignment="Top" Margin="0,50,0,0" Height="5" Grid.ColumnSpan="2" />
<Button x:Name="AboutBtn" Margin="0,10,10,0" VerticalAlignment="Top" HorizontalAlignment="Right" Grid.ColumnSpan="3" Click="AboutBtn_Click" ToolTipService.ToolTip="About."/>
<AutoSuggestBox x:Name="TextBox_URL" Margin="150,10,250,0" Height="31" VerticalAlignment="Top" Grid.ColumnSpan="2" QueryIcon="Find" TextChanged="TextBox_URL_TextChanged" QuerySubmitted="TextBox_URL_QuerySubmitted"/>
UWP
XAML
<Grid>
<Grid.ColumnDefinitions>
<ColumnDefinition Width="915*"/>
<ColumnDefinition Width="586*"/>
</Grid.ColumnDefinitions>
<Button x:Name="TurnLeft" Style="{StaticResource NavigationBackButtonSmallStyle}" Margin="10,10,0,0" VerticalAlignment="Top" Click="TurnLeft_Click"/>
<Button x:Name="TurnRight" Style="{StaticResource NavigationBackButtonSmallStyle}" RenderTransformOrigin = "0.5,0.5" Margin="50,10,0,0" VerticalAlignment="Top" Click="TurnRight_Click" FlowDirection ="RightToLeft"/>
<Button x:Name="ReloadBtn" Content="刷新" VerticalAlignment="Top" FontSize="14" Margin="85,10,0,0" HorizontalAlignment="Left" Click="ReloadBtn_Click" />
<WebView x:Name="HtmlWebViewSource" Source="https://baidu.com" Margin="0,55,0,0" Grid.ColumnSpan="2" NavigationCompleted="WebviewNavigated" NavigationFailed="WebNavigationFailed" NavigationStarting="WebviewNavigating"/>
<!--<TextBox x:Name="TextBox_URL" Margin="150,10,250,0" TextWrapping="Wrap" Text="" Height="31" VerticalAlignment="Top" Grid.ColumnSpan="2"/>-->
<!--<Button x:Name="TurnURL" Content="转到URL" Margin="0,10,170,0" VerticalAlignment="Top" HorizontalAlignment="Right" Click="TurnURL_Click" Grid.Column="1"/>-->
<ProgressBar x:Name="WebProgressBar" Value="100" VerticalAlignment="Top" Margin="0,50,0,0" Height="5" Grid.ColumnSpan="2" />
<Button x:Name="AboutBtn" Content="关于" Margin="0,10,10,0" VerticalAlignment="Top" HorizontalAlignment="Right" Grid.ColumnSpan="3" Click="AboutBtn_Click"/>
<AutoSuggestBox x:Name="TextBox_URL" Margin="150,10,250,0" Height="31" VerticalAlignment="Top" Grid.ColumnSpan="2" PlaceholderText="输入Web地址,按Enter键或搜索按钮以访问" QueryIcon="Find" TextChanged="TextBox_URL_TextChanged" QuerySubmitted="TextBox_URL_QuerySubmitted"/>
</Grid>
事件处理
winui3
C#
using Microsoft.Web.WebView2.Core;
using Windows.Foundation.Metadata;
using Windows.UI.Notifications;
using System;
using Microsoft.UI.Xaml;
using Microsoft.UI.Xaml.Controls;
//code
private ObservableCollection<String> suggestions;
//code
public void Initialize()
{
AboutBtn.Content = "关于";
ReloadBtn.Content = "刷新";
TextBox_URL.PlaceholderText = "输入Web地址,按Enter键或搜索按钮以访问";
//_appWindow.Hide();
//_appWindow = GetAppWindowForCurrentWindow();
//_appWindow.SetPresenter(AppWindowPresenterKind.FullScreen);
//_appWindow.SetPresenter(AppWindowPresenterKind.Default);
}
public MainWindow()
{
this.InitializeComponent();
Initialize();
if (!CheckInternetAvailable())
{
SendMessage("你尚未连接\n请尝试:\n检查网络电缆、调制解调器和路由器\n重新连接到无线网络");
}
suggestions = new ObservableCollection<string>();
}
public bool CheckInternetAvailable()
{
if (true)
{
return true;
}
}
public void Check()
{
if (HtmlWebViewSource.CanGoBack)
{
TurnLeft.IsEnabled = true;
}
else
{
TurnLeft.IsEnabled = false;
}
if (HtmlWebViewSource.CanGoForward)
{
TurnRight.IsEnabled = true;
}
else
{
TurnRight.IsEnabled = false;
}
}
string CheckAndModifyLink(string link)
{
if (string.IsNullOrEmpty(link))
{
return "https://www.baidu.com";
}
if (link.StartsWith("https://") || link.StartsWith("http://"))
return link;
else
{
link = "https://" + link;
return link;
}
}
public void DisadledAll()
{
TurnLeft.IsEnabled = false;
TurnRight.IsEnabled = false;
}
public void WebviewNavigating(object sender, CoreWebView2NavigationStartingEventArgs e)
{
DisadledAll();
WebProgressBar.IsIndeterminate = true;
WebProgressBar.ShowError = false;
}
public void WebviewNavigated(object sender, CoreWebView2NavigationCompletedEventArgs e)
{
if(e.IsSuccess)
{
WebProgressBar.IsIndeterminate = false;
WebProgressBar.ShowError = false;
Check();
}
else
{
WebProgressBar.ShowError = true;
}
}
public void SendMessage(string Str)
{
var toastXml = ToastNotificationManager.GetTemplateContent(ToastTemplateType.ToastText01);
//toastXml.GetXml();
var node = toastXml.GetElementsByTagName("text")[0];
var text = toastXml.CreateTextNode(Str);
node.AppendChild(text);
var xmlstr = toastXml.GetXml();
// 创建并初始化 ToastNotification 的新实例
var notification = new ToastNotification(toastXml);
// 创建通知对象
var toastNotifier = ToastNotificationManager.CreateToastNotifier("WpfTest");
toastNotifier.Show(notification);
}
public async void SendMessageDialog(string MessageTitle, string Content)
{
Button btn = new Button();
var dialog = new ContentDialog()
{
Title = MessageTitle,
RequestedTheme = ElementTheme.Default,
//FullSizeDesired = true,
//MaxWidth = this.ActualWidth // Required for Mobile!
};
// Setup Content
var panel = new StackPanel();
panel.Children.Add(new TextBlock
{
Text = Content,
TextWrapping = TextWrapping.Wrap,
});
dialog.Content = panel;
// Add Buttons
dialog.IsPrimaryButtonEnabled = true;
dialog.PrimaryButtonClick += delegate
{
btn.Content = "Result: OK";
};
dialog.SecondaryButtonText = "好";
var result = await dialog.ShowAsync();
if (result == ContentDialogResult.None)
{
btn.Content = "Result: NONE";
}
}
private void TurnLeft_Click(object sender, RoutedEventArgs e)
{
if (HtmlWebViewSource.CanGoBack)
{
HtmlWebViewSource.GoBack();
}
else
{
Check();
SendMessage("发生错误:无法执行返回操作");
}
}
private void TurnRight_Click(object sender, RoutedEventArgs e)
{
if (HtmlWebViewSource.CanGoForward)
{
HtmlWebViewSource.GoForward();
}
else
{
Check();
SendMessage("发生错误:无法执行回退操作");
}
}
private void ReloadBtn_Click(object sender, RoutedEventArgs e)
{
HtmlWebViewSource.Reload();
}
private async void AboutBtn_Click(object sender, RoutedEventArgs e)
{
Button mybtn= sender as Button;
ContentDialog noWifiDialog = new ContentDialog
{
Title = "关于此软件-WebSearch.",
Content = "Version:\t暂无\n" + //"Version:\t.\n\n" +
"作者:\tCopyRight .",
CloseButtonText = "好",
//PrimaryButtonText = "",
//SecondaryButtonText = "",
//设置默认按钮
DefaultButton = ContentDialogButton.Close,
};
//必须设置XamlRoot,否则会报错误:Value does not fall within the expected range.
if (ApiInformation.IsApiContractPresent("Windows.Foundation.UniversalApiContract", 8))
{
noWifiDialog.XamlRoot = this.Content.XamlRoot;
}
ContentDialogResult result = await noWifiDialog.ShowAsync();
}
private void TextBox_URL_TextChanged(AutoSuggestBox sender, AutoSuggestBoxTextChangedEventArgs args)
{
suggestions.Clear();
if (sender.Text.StartsWith("https://") || sender.Text.StartsWith("http://"))
{
suggestions.Add("https://www.bing.com/");
suggestions.Add("https://www.bilibili.com/");
suggestions.Add("https://www.microsoft.com/");
suggestions.Add("https://www.thepaper.cn");
suggestions.Add("https://www.iqiyi.com/");
suggestions.Add("https://github.com/");
}
else
{
if (sender.Text == "")
{
suggestions.Add("https://www.bing.com/");
suggestions.Add("https://www.bilibili.com/");
suggestions.Add("https://www.microsoft.com/");
suggestions.Add("https://www.thepaper.cn");
suggestions.Add("https://www.iqiyi.com/");
suggestions.Add("https://github.com/");
}
else
{
if ("https://".StartsWith(sender.Text))
{
suggestions.Add("https://");
}
if ("www.".StartsWith(sender.Text))
{
suggestions.Add("https://www.");
}
else
{
suggestions.Add("https://" + sender.Text);
}
}
}
sender.ItemsSource = suggestions;
}
private void TextBox_URL_QuerySubmitted(AutoSuggestBox sender, AutoSuggestBoxQuerySubmittedEventArgs args)
{
if (args.ChosenSuggestion == null)
{
TextBox_URL.Text = sender.Text;
if (!CheckInternetAvailable())
{
SendMessage("你尚未连接\n请尝试:\n检查网络电缆、调制解调器和路由器\n重新连接到无线网络");
}
string URL = TextBox_URL.Text;
URL = CheckAndModifyLink(URL);
TextBox_URL.Text = URL;
HtmlWebViewSource.Source = new Uri(URL);
}
else
{
TextBox_URL.Text = args.ChosenSuggestion.ToString();
}
}
UWP
C#
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Reflection;
using System.Runtime.InteropServices.WindowsRuntime;
using System.Threading.Tasks;
using Windows.ApplicationModel.Core;
using Windows.Foundation;
using Windows.Foundation.Collections;
using Windows.UI;
using Windows.UI.Notifications;
using Windows.UI.WebUI;
using Windows.UI.WindowManagement;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Controls.Primitives;
using Windows.UI.Xaml.Data;
using Windows.UI.Xaml.Input;
using Windows.UI.Xaml.Media;
using Windows.UI.Xaml.Navigation;
using Microsoft.Toolkit.Uwp.Connectivity;
using System.Collections.ObjectModel;
//code
private ObservableCollection<String> suggestions;
//code
public void Initialize()
{
AboutBtn.Content = "关于";
ReloadBtn.Content = "刷新";
TextBox_URL.PlaceholderText = "输入Web地址,按Enter键或搜索按钮以访问";
//_appWindow.Hide();
//_appWindow = GetAppWindowForCurrentWindow();
//_appWindow.SetPresenter(AppWindowPresenterKind.FullScreen);
//_appWindow.SetPresenter(AppWindowPresenterKind.Default);
}
public MainWindow()
{
this.InitializeComponent();
Initialize();
if (!CheckInternetAvailable())
{
SendMessage("你尚未连接\n请尝试:\n检查网络电缆、调制解调器和路由器\n重新连接到无线网络");
}
suggestions = new ObservableCollection<string>();
}
public bool CheckInternetAvailable()
{
if (true)
{
return true;
}
}
public void Check()
{
if (HtmlWebViewSource.CanGoBack)
{
TurnLeft.IsEnabled = true;
}
else
{
TurnLeft.IsEnabled = false;
}
if (HtmlWebViewSource.CanGoForward)
{
TurnRight.IsEnabled = true;
}
else
{
TurnRight.IsEnabled = false;
}
}
string CheckAndModifyLink(string link)
{
if (string.IsNullOrEmpty(link))
{
return "https://www.baidu.com";
}
if (link.StartsWith("https://") || link.StartsWith("http://"))
return link;
else
{
link = "https://" + link;
return link;
}
}
public void DisadledAll()
{
TurnLeft.IsEnabled = false;
TurnRight.IsEnabled = false;
}
public void WebviewNavigating(object sender, CoreWebView2NavigationStartingEventArgs e)
{
DisadledAll();
WebProgressBar.IsIndeterminate = true;
WebProgressBar.ShowError = false;
}
public void WebviewNavigated(object sender, CoreWebView2NavigationCompletedEventArgs e)
{
if(e.IsSuccess)
{
WebProgressBar.IsIndeterminate = false;
WebProgressBar.ShowError = false;
Check();
}
else
{
WebProgressBar.ShowError = true;
}
}
public void SendMessage(string Str)
{
var toastXml = ToastNotificationManager.GetTemplateContent(ToastTemplateType.ToastText01);
//toastXml.GetXml();
var node = toastXml.GetElementsByTagName("text")[0];
var text = toastXml.CreateTextNode(Str);
node.AppendChild(text);
var xmlstr = toastXml.GetXml();
// 创建并初始化 ToastNotification 的新实例
var notification = new ToastNotification(toastXml);
// 创建通知对象
var toastNotifier = ToastNotificationManager.CreateToastNotifier("WpfTest");
toastNotifier.Show(notification);
}
public async void SendMessageDialog(string MessageTitle, string Content)
{
Button btn = new Button();
var dialog = new ContentDialog()
{
Title = MessageTitle,
RequestedTheme = ElementTheme.Default,
//FullSizeDesired = true,
//MaxWidth = this.ActualWidth // Required for Mobile!
};
// Setup Content
var panel = new StackPanel();
panel.Children.Add(new TextBlock
{
Text = Content,
TextWrapping = TextWrapping.Wrap,
});
dialog.Content = panel;
// Add Buttons
dialog.IsPrimaryButtonEnabled = true;
dialog.PrimaryButtonClick += delegate
{
btn.Content = "Result: OK";
};
dialog.SecondaryButtonText = "好";
var result = await dialog.ShowAsync();
if (result == ContentDialogResult.None)
{
btn.Content = "Result: NONE";
}
}
private void TurnLeft_Click(object sender, RoutedEventArgs e)
{
if (HtmlWebViewSource.CanGoBack)
{
HtmlWebViewSource.GoBack();
}
else
{
Check();
SendMessage("发生错误:无法执行返回操作");
}
}
private void TurnRight_Click(object sender, RoutedEventArgs e)
{
if (HtmlWebViewSource.CanGoForward)
{
HtmlWebViewSource.GoForward();
}
else
{
Check();
SendMessage("发生错误:无法执行回退操作");
}
}
private void ReloadBtn_Click(object sender, RoutedEventArgs e)
{
HtmlWebViewSource.Reload();
}
private async void AboutBtn_Click(object sender, RoutedEventArgs e)
{
Button mybtn= sender as Button;
ContentDialog noWifiDialog = new ContentDialog
{
Title = "关于此软件-WebSearch.",
Content = "Ve",
CloseButtonText = "好",
//PrimaryButtonText = "",
//SecondaryButtonText = "",
//设置默认按钮
DefaultButton = ContentDialogButton.Close,
};
//必须设置XamlRoot,否则会报错误:Value does not fall within the expected range.
if (ApiInformation.IsApiContractPresent("Windows.Foundation.UniversalApiContract", 8))
{
noWifiDialog.XamlRoot = this.Content.XamlRoot;
}
ContentDialogResult result = await noWifiDialog.ShowAsync();
}
private void TextBox_URL_TextChanged(AutoSuggestBox sender, AutoSuggestBoxTextChangedEventArgs args)
{
suggestions.Clear();
if (sender.Text.StartsWith("https://") || sender.Text.StartsWith("http://"))
{
suggestions.Add("https://www.bing.com/");
suggestions.Add("https://www.bilibili.com/");
suggestions.Add("https://www.microsoft.com/");
suggestions.Add("https://www.thepaper.cn");
suggestions.Add("https://www.iqiyi.com/");
suggestions.Add("https://github.com/");
}
else
{
if (sender.Text == "")
{
suggestions.Add("https://www.bing.com/");
suggestions.Add("https://www.bilibili.com/");
suggestions.Add("https://www.microsoft.com/");
suggestions.Add("https://www.thepaper.cn");
suggestions.Add("https://www.iqiyi.com/");
suggestions.Add("https://github.com/");
}
else
{
if ("https://".StartsWith(sender.Text))
{
suggestions.Add("https://");
}
if ("www.".StartsWith(sender.Text))
{
suggestions.Add("https://www.");
}
else
{
suggestions.Add("https://" + sender.Text);
}
}
}
sender.ItemsSource = suggestions;
}
private void TextBox_URL_QuerySubmitted(AutoSuggestBox sender, AutoSuggestBoxQuerySubmittedEventArgs args)
{
if (args.ChosenSuggestion == null)
{
TextBox_URL.Text = sender.Text;
if (!CheckInternetAvailable())
{
SendMessage("你尚未连接\n请尝试:\n检查网络电缆、调制解调器和路由器\n重新连接到无线网络");
}
string URL = TextBox_URL.Text;
URL = CheckAndModifyLink(URL);
TextBox_URL.Text = URL;
HtmlWebViewSource.Source = new Uri(URL);
}
else
{
TextBox_URL.Text = args.ChosenSuggestion.ToString();
}
}
using错误解决方案
参见:
WinUI 3入门过程的各种坑一_dmm128073411的博客-CSDN博客
效果展示:
winui3
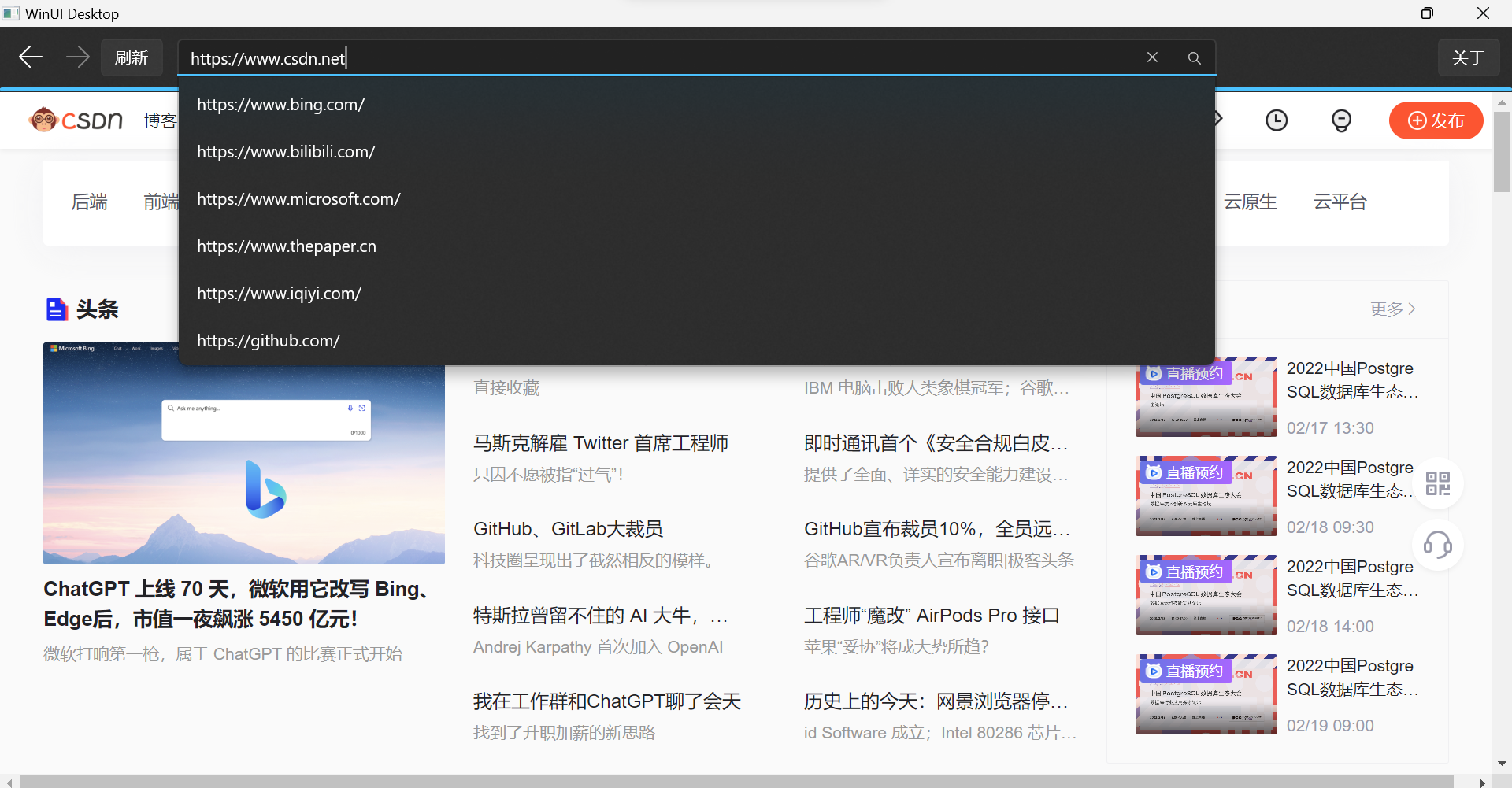
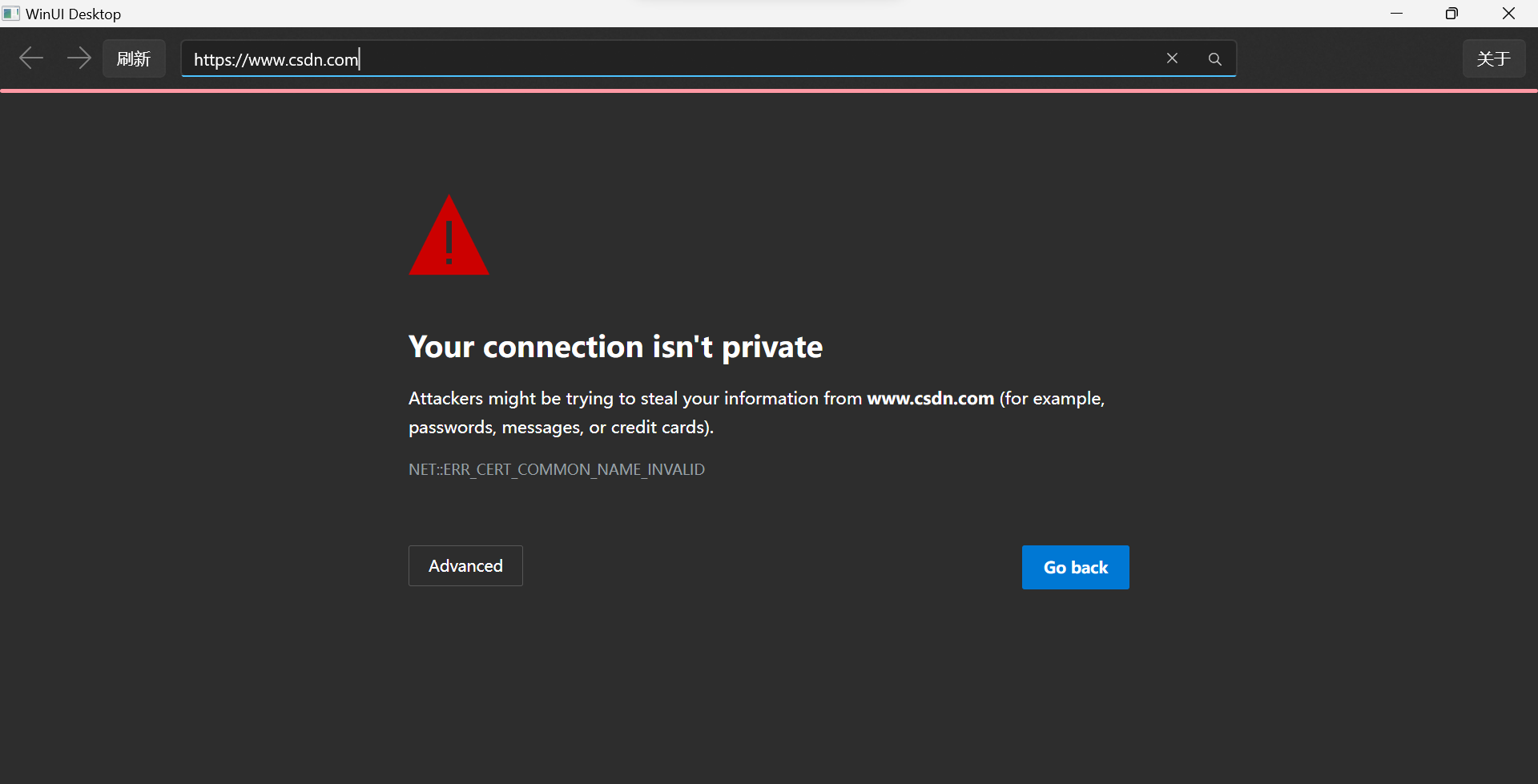
UWP
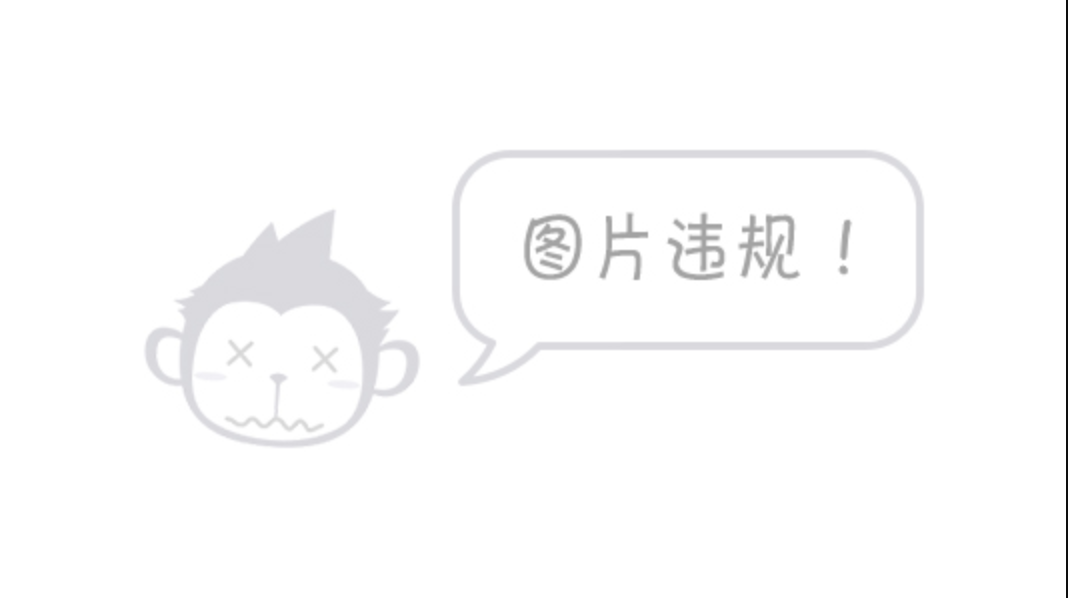
参考的链接:
Windows 开发文档 | Microsoft Learn