命令行参数可以分为两类,一类是短选项,一类是长选项,短选项在参数前加一杠"-",长选项在参数前连续加两杠"--"。如:-a,-A,-b都表示短选项;--all,--almost-all,--author都表示长选项。
getopt
短选项的解析
getopt_long
getopt_long函数的工作原理与getopt类似,只是它也接受以两个短划线开头的长选项。
getopt_long_only
getopt_long_only类似于getopt_long,但是'-'和"--"都可以表示长选项。如果以'-'(不是"--")开头的选项不匹配长选项,但匹配短选项,则将其解析为短选项。
ChatGPT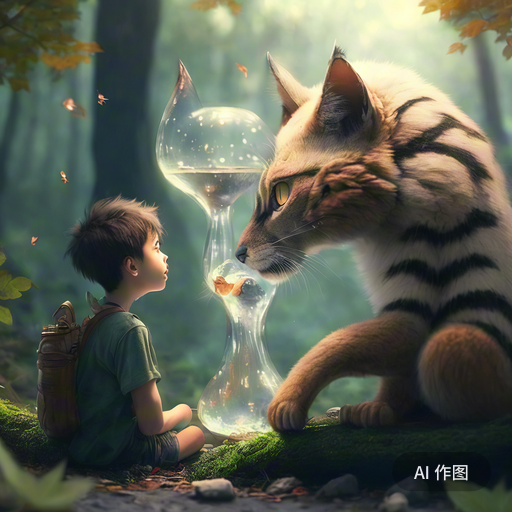
getopt, getopt_long, and getopt_long_only are functions used in C and C++ programming for parsing command-line arguments. They help in handling options passed to the program, making it easier to process arguments such as -f filename or --file filename.
Here's a brief explanation of each:
1. getopt:
- This is the standard function used for command-line argument parsing.
- It works with short options like -a or -b.
- It is defined by the POSIX standard and is available on Unix-like systems.
2. getopt_long:
- It is an extension to getopt which can process long options like --help or --version.
- It allows both short and long options to be used interchangeably.
- This function is not part of POSIX and is typically found in GNU C Library (glibc).
3. getopt_long_only:
- It is very similar to getopt_long, with the key difference being that it can process long options with a single hyphen - as well as the standard double hyphen --.
- This can be useful for supporting long options in a similar style to some Windows command-line tools.
Here is an example showing how these functions might be used in a C program:
#include <stdio.h>
#include <stdlib.h>
#include <getopt.h>
int main(int argc, char *argv[]) {
int c;
int digit_optind = 0;
static struct option long_options[] = {
{"add", required_argument, 0, 'a'},
{"delete", no_argument, 0, 'd'},
{"verbose", no_argument, 0, 'v'},
{"create", required_argument, 0, 'c'},
{"file", required_argument, 0, 'f'},
{0, 0, 0, 0}
};
int option_index = 0;
while ((c = getopt_long(argc, argv, "advc:f:", long_options, &option_index)) != -1) {
switch (c) {
case 'a':
printf("Option --add with value `%s`\n", optarg);
break;
case 'd':
printf("Option --delete\n");
break;
case 'v':
printf("Option --verbose\n");
break;
case 'c':
printf("Option --create with value `%s`\n", optarg);
break;
case 'f':
printf("Option --file with value `%s`\n", optarg);
break;
case '?': // getopt_long already printed an error message for unknown options
break;
default:
printf("?? getopt returned character code 0%o ??\n", c);
}
}
if (optind < argc) {
printf("Non-option ARGV-elements: ");
while (optind < argc)
printf("%s ", argv[optind++]);
printf("\n");
}
exit(EXIT_SUCCESS);
}
To use them in your project, you'll need to include the appropriate headers, and, especially for the non-POSIX functions, make sure the library support is available on your target platform.
Keep in mind that getopt, getopt_long, and getopt_long_only are standard in GNU C Library (glibc) and may not be available or behave differently on non-GNU platforms. Always check the documentation of the platform you're targeting.
getopt、`getopt_long` 和 getopt_long_only 是 C 和 C++ 编程中用于解析命令行参数的函数。它们帮助处理传递给程序的选项,使得处理例如 -f filename 或 --file filename 这样的参数变得更容易。
下面是对每个函数的简要说明:
1. getopt:
- 这是用于命令行参数解析的标准函数。
- 它仅处理短选项,如 -a 或 -b。
- 它由 POSIX 标准定义,并且在类 Unix 系统上可用。
2. getopt_long:
- 它是 getopt 的扩展,可以处理长选项,如 --help 或 --version。
- 它允许短选项和长选项可互换使用。
- 该函数不属于 POSIX,并且通常在 GNU C 库(`glibc`)中找到。
3. getopt_long_only:
- 它与 getopt_long 非常相似,关键区别在于它可以处理既有单个连字符 - 又有标准双连字符 -- 的长选项。
- 如果你希望以类似于某些 Windows 命令行工具的风格支持长选项,这会非常有用。
以下是一个在 C 程序中使用这些函数的示例:
#include <stdio.h>
#include <stdlib.h>
#include <getopt.h>
int main(int argc, char *argv[]) {
int c;
int digit_optind = 0;
static struct option long_options[] = {
{"add", required_argument, 0, 'a'},
{"delete", no_argument, 0, 'd'},
{"verbose", no_argument, 0, 'v'},
{"create", required_argument, 0, 'c'},
{"file", required_argument, 0, 'f'},
{0, 0, 0, 0}
};
int option_index = 0;
while ((c = getopt_long(argc, argv, "advc:f:", long_options, &option_index)) != -1) {
switch (c) {
case 'a':
printf("选项 --add 带有值 `%s`\n", optarg);
break;
case 'd':
printf("选项 --delete\n");
break;
case 'v':
printf("选项 --verbose\n");
break;
case 'c':
printf("选项 --create 带有值 `%s`\n", optarg);
break;
case 'f':
printf("选项 --file 带有值 `%s`\n", optarg);
break;
case '?': // getopt_long 已经为未知选项打印了错误信息
break;
default:
printf("?? getopt 返回字符代码 0%o ??\n", c);
}
}
if (optind < argc) {
printf("非选项 ARGV 元素: ");
while (optind < argc)
printf("%s ", argv[optind++]);
printf("\n");
}
exit(EXIT_SUCCESS);
}
要在项目中使用这些函数,您需要包含适当的头文件,尤其是对于非 POSIX 函数,您需要确保目标平台上有库支持。
请记住,getopt、getopt_long 和 getopt_long_only 在 GNU C 库(glibc)中是标准的,在非 GNU 平台上可能不可用或行为不同。始终检查您的目标平台的文档。
参考:
Linux命令行参数解析——getopt_long - 简书 (jianshu.com)
Linux函数学习:getopt、getopt_long、getopt_long_only。 - 知乎 (zhihu.com)
命令行参数解析之getopt | 彩色世界 (ibaoger.com)