How to connect Matlab with OpenCV on Windows7
相关文档及资料下载:http://download.csdn.net/detail/erlingmusan/7360649
---------------------------------------------------------------------------
---------------------------------------------------------------------------
1- The software you need to install first,
2- How to install OpenCV,
3- Connect Matlab to OpenCV library,
4- Examples on how to use the OpenCV library,
---------------------------------------------------------------------------
---------------------------------------------------------------------------
------------------------------------------------------------
1- The softwer you need to install first :
------------------------------------------------------------
This tutorial will show you how to connect Matlab (2010a 32bits version) to the OpenCV library version (2.3) and to do that we need to install the following softwere :
--> Matlab (2010a 32bits version),
--> Visual C++ Express Edition 2008 (32 bits version),
--> The compiled OpenCV library 2.3,
For the two first softwere use directly the default instalation parameters and for the OpenCV library see the next section.
------------------------------------------------------------
2- How to install OpenCV :
------------------------------------------------------------
After downloading the library, put it in the following folder: "C:\OpenCV2.3"
---------------------------------------------------------------------------
1- The software you need to install first,
2- How to install OpenCV,
3- Connect Matlab to OpenCV library,
4- Examples on how to use the OpenCV library,
---------------------------------------------------------------------------
---------------------------------------------------------------------------
------------------------------------------------------------
1- The softwer you need to install first :
------------------------------------------------------------
This tutorial will show you how to connect Matlab (2010a 32bits version) to the OpenCV library version (2.3) and to do that we need to install the following softwere :
--> Matlab (2010a 32bits version),
--> Visual C++ Express Edition 2008 (32 bits version),
--> The compiled OpenCV library 2.3,
For the two first softwere use directly the default instalation parameters and for the OpenCV library see the next section.
------------------------------------------------------------
2- How to install OpenCV :
------------------------------------------------------------
After downloading the library, put it in the following folder: "C:\OpenCV2.3"
<< see picture 0 >>
and inside this folder you will have two other folder (build & opencv)
<< see picture 1 >>.
Now we need to add to the "ENVIRONMENT VARIABLE" the appropriate folder, to do that go to "Advance System Parameters" and click on
<< see picture 2 >>
"Environment Variable"
<< see picture 3 >>
and in "System Variables" click new:
Variable name : OPENCV23
Variable Value : C:\OpenCV2.3\build
Variable name : OPENCV23
Variable Value : C:\OpenCV2.3\build
<< see picture 4 >>
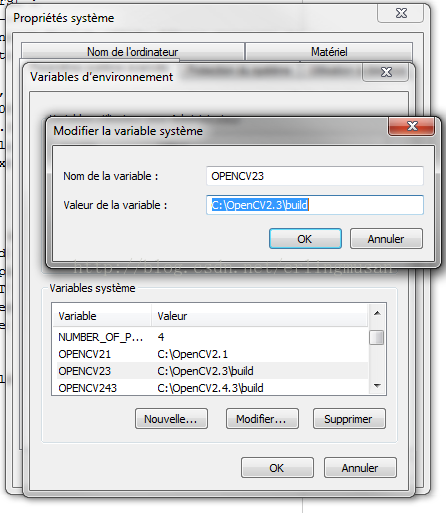
After that click on "Path" and add in the end of the line : %OPENCV23%\x86\vc9\bin;
After that click on "Path" and add in the end of the line : %OPENCV23%\x86\vc9\bin;
<< see picture 5 >>
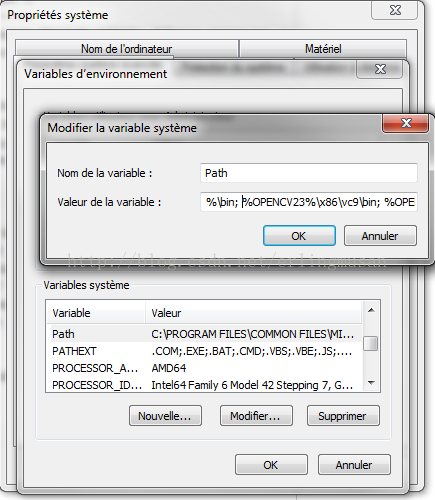
Click ok -> ok -> ok ====> and now we need to configure Matlab,
------------------------------------------------------------
3- Connect Matlab to OpenCV library :
------------------------------------------------------------
After runing Matlab 32bits version type the following command :
>> mex -setup % and if you did install visual c++ correctly you will have
% the following liste of compilers at minimum :
Please choose your compiler for building external interface (MEX) files:
Would you like mex to locate installed compilers [y]/n? y
Select a compiler:
[1] Lcc-win32 C 2.4.1 in C:\PROGRA~2\MATLAB\R2010a\sys\lcc
[2] Microsoft Visual C++ 2008 Express in C:\Program Files (x86)\Microsoft Visual Studio 9.0
Click ok -> ok -> ok ====> and now we need to configure Matlab,
------------------------------------------------------------
3- Connect Matlab to OpenCV library :
------------------------------------------------------------
After runing Matlab 32bits version type the following command :
>> mex -setup % and if you did install visual c++ correctly you will have
% the following liste of compilers at minimum :
Please choose your compiler for building external interface (MEX) files:
Would you like mex to locate installed compilers [y]/n? y
Select a compiler:
[1] Lcc-win32 C 2.4.1 in C:\PROGRA~2\MATLAB\R2010a\sys\lcc
[2] Microsoft Visual C++ 2008 Express in C:\Program Files (x86)\Microsoft Visual Studio 9.0
[0] None
We will choose the second compiler, and now we have to edit the MEXOPTS.BAT file, but first we need to find it :
>> fullfile(prefdir,'mexopts.bat')
In my machine, this file is in
C:\users\<username>\AppData\Roaming\MathWorks\MATLAB\R2009b\mexopts.bat
Once the file found you can open it using for exemple Notepad++ and add at
the end this lines :
rem rem ************
rem rem OpenCV2.3
rem rem ************
set OCVDIR=C:\OpenCV2.3\build
set INCLUDE=%OCVDIR%\include; %INCLUDE%
set LIB=%OCVDIR%\x86\vc9\lib; %LIB%
set PATH=%OCVDIR%\x86\vc9\bin; %OCVDIR%\bin; %PATH%
We will choose the second compiler, and now we have to edit the MEXOPTS.BAT file, but first we need to find it :
>> fullfile(prefdir,'mexopts.bat')
In my machine, this file is in
C:\users\<username>\AppData\Roaming\MathWorks\MATLAB\R2009b\mexopts.bat
Once the file found you can open it using for exemple Notepad++ and add at
the end this lines :
rem rem ************
rem rem OpenCV2.3
rem rem ************
set OCVDIR=C:\OpenCV2.3\build
set INCLUDE=%OCVDIR%\include; %INCLUDE%
set LIB=%OCVDIR%\x86\vc9\lib; %LIB%
set PATH=%OCVDIR%\x86\vc9\bin; %OCVDIR%\bin; %PATH%
In case you did not install OpenCV in the "C" Partition like i did you need to change the value of "OCVDIR" by giving it the correct path of the OpenCV library,
In the same file look for the section "Linker parameters", after that add the different lib files of OpenCV to the end of the line of the command:
set LINKFLAGS ....... opencv_calib3d230.lib opencv_calib3d230d.lib ....
The list of all this files:
opencv_calib3d230.lib
opencv_calib3d230d.lib
opencv_contrib230.lib
opencv_contrib230d.lib
opencv_core230.lib
opencv_core230d.lib
opencv_features2d230.lib
opencv_features2d230d.lib
opencv_flann230.lib
opencv_flann230d.lib
opencv_gpu230.lib
opencv_gpu230d.lib
opencv_haartraining_engine.lib
opencv_haartraining_engined.lib
opencv_highgui230.lib
opencv_highgui230d.lib
opencv_imgproc230.lib
opencv_imgproc230d.lib
opencv_legacy230.lib
opencv_legacy230d.lib
opencv_ml230.lib
opencv_ml230d.lib
opencv_objdetect230.lib
opencv_objdetect230d.lib
opencv_video230.lib
opencv_video230.lib
Now, all you have to do is restart Matlab and do not forget to save "MEXOPTS.BAT" you will find with inside the folder of the tutorial the MEXOPTS.BAT file which is used on my computer.
------------------------------------------------------------
4- Exemples on how to use the OpenCV library:
------------------------------------------------------------
To use the OpenCV library in Matlab we must use the "mex files" and because this is not the topics of this tutorial is will not talk about these files but you can read the PDF file: "introMEX-TR.pdf",
The examples that i will present here are the two examples given by
"Georgios Evangelidis" :
4- Exemples on how to use the OpenCV library:
------------------------------------------------------------
To use the OpenCV library in Matlab we must use the "mex files" and because this is not the topics of this tutorial is will not talk about these files but you can read the PDF file: "introMEX-TR.pdf",
The examples that i will present here are the two examples given by
"Georgios Evangelidis" :
--> displayImage.cpp (with no input argument)
--> smoothImage.cpp (with inputs argument)
--> smoothImage.cpp (with inputs argument)
Example 1: A simple MEX file without input/output arguments that uses simple OpenCV modules
/*******************************************************************
This is a simple MEX file. It just calls openCV functions for loading,
smothing and diaplaying the results
********************************************************************/
#include <stdio.h>
#include <stdlib.h>
#include <opencv/cv.h>
#include <opencv/highgui.h>
#include "mex.h"
int smoothImage(char* filename){
//load the image
IplImage* img = cvLoadImage(filename);
if(!img){
printf("Cannot load image file: %s\n",filename);
return -1;
}
//create another image
IplImage* img_smooth = cvCloneImage(img);
//smooth the image img and save the result to img_smooth
cvSmooth(img, img_smooth, CV_GAUSSIAN, 5, 5);
// create windows to show images
cvNamedWindow("Original Image", CV_WINDOW_AUTOSIZE);
cvMoveWindow("Original Image", 100, 100);
cvNamedWindow("Smoothed Image", CV_WINDOW_AUTOSIZE);
cvMoveWindow("Smoothed Image", 400, 400);
// show the images
cvShowImage("Original Image", img );
cvShowImage("Smoothed Image", img_smooth );
// wait for a key
cvWaitKey(0);
//destroy windows
cvDestroyWindow("Original Image");
cvDestroyWindow("Smoothed Image");
//release images
cvReleaseImage(& img);
cvReleaseImage(& img_smooth);
return 0;
};
void mexFunction(int nlhs, mxArray* plhs[], int nrhs, const mxArray* prhs[]){
if (nrhs != 0)
{
mexErrMsgTxt("Do not give input arguments.");
}
if (nlhs != 0)
{
mexErrMsgTxt("Do not give output arguments.");
}
char *name = "cameraman.png";
smoothImage(name);
}
To compile these files all you need to do is the following:
>> mex displayImage.cpp
And to call the function all you need to do is:
>> dispalayImage
Example 2: A MEX file with input/output arguments that uses OpenCV
/*******************************************************************
This is a simple MEX file that accepts as inputs an image and the
size of a Gausian filter(two parameters). Then it applies the filter
to the image by calling the OpenCV function cvSmooth and returns the
filtered image to a matlab variable.
********************************************************************/
#include <opencv/cv.h>
#include <opencv/highgui.h>
#include <opencv/cxcore.h>
#ifndef HAS_OPENCV
#define HAS_OPENCV
#endif
#include "mex.h"
#include "mc_convert.h"
#include "mc_convert.cpp"
void mexFunction(int nlhs, mxArray *plhs[], int nrhs,
const mxArray *prhs[]) {
//Read Matlab image and load it to an IplImage struct
IplImage* inputImg = mxArr_to_new_IplImage(prhs[0]);
//Read the filter parameters
double filterHeight, filterWidth;
mat_to_scalar (prhs[1], &filterHeight);
mat_to_scalar (prhs[2], &filterWidth);
//smooth the input image and save the result to outputImg
IplImage* outputImg = cvCloneImage(inputImg);
cvSmooth(inputImg, outputImg, CV_GAUSSIAN, filterWidth, filterHeight);
//Return output image to mxArray (Matlab matrix)
plhs[0] = IplImage_to_new_mxArr(outputImg);
cvReleaseImage(&inputImg);
cvReleaseImage(&outputImg);
}
>> mex smoothImage.cpp
will create the file smoothImage.mexw32
.Then, you can run the Matlab function as follows
>> im = imread('cameraman.png');
>> filterHeight = 7;
>> filterWidth = 7;
>> outImage = smoothImage(im, filterHeight, filterWidht);
参考文献:http://xanthippi.ceid.upatras.gr/people/evangelidis/matlab_opencv/