Camera Calibration
The functions in this section use a so-called pinhole camera model. In this model, a scene view is formed by projecting 3D points into the image plane using a perspective transformation.
or
where:
are the coordinates of a 3D point in the world coordinate space
are the coordinates of the projection point in pixels
is a camera matrix, or a matrix of intrinsic parameters
is a principal point that is usually at the image center
are the focal lengths expressed in pixel units.
Thus, if an image from the camera is scaled by a factor, all of these parameters should be scaled (multiplied/divided, respectively) by the same factor. The matrix of intrinsic parameters does not depend on the scene viewed. So, once estimated, it can be re-used as long as the focal length is fixed (in case of zoom lens). The joint rotation-translation matrix is called a matrix of extrinsic parameters. It is used to describe the camera motion around a static scene, or vice versa, rigid motion of an object in front of a still camera. That is,
translates coordinates of a point
to a coordinate system, fixed with respect to the camera. The transformation above is equivalent to the following (when
):
The following figure illustrates the pinhole camera model.
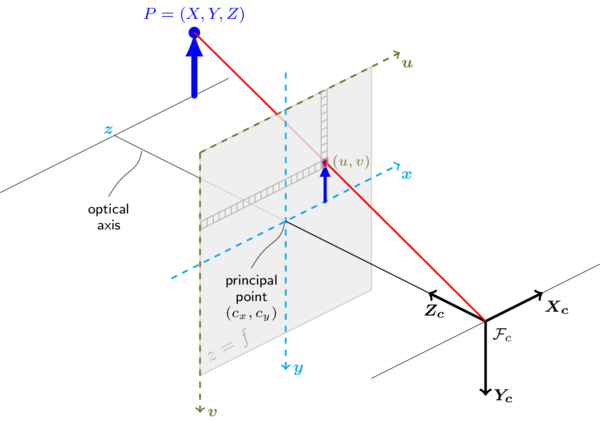
Real lenses usually have some distortion, mostly radial distortion and slight tangential distortion. So, the above model is extended as:
,
,
,
,
, and
are radial distortion coefficients.
and
are tangential distortion coefficients. Higher-order coefficients are not considered in OpenCV.
The next figure shows two common types of radial distortion: barrel distortion (typically and pincushion distortion (typically
).
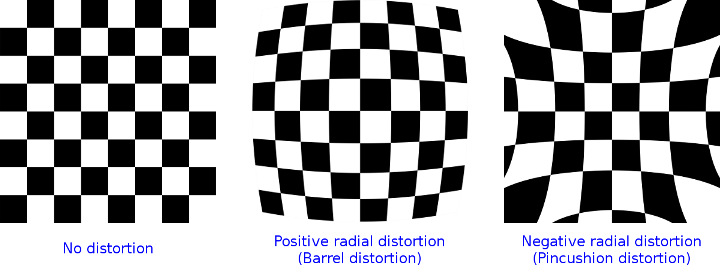
In the functions below the coefficients are passed or returned as
vector. That is, if the vector contains four elements, it means that . The distortion coefficients do not depend on the scene viewed. Thus, they also belong to the intrinsic camera parameters. And they remain the same regardless of the captured image resolution. If, for example, a camera has been calibrated on images of
320 x 240
resolution, absolutely the same distortion coefficients can be used for 640 x 480
images from the same camera while ,
,
, and
need to be scaled appropriately.
The functions below use the above model to do the following:
- Project 3D points to the image plane given intrinsic and extrinsic parameters.
- Compute extrinsic parameters given intrinsic parameters, a few 3D points, and their projections.
- Estimate intrinsic and extrinsic camera parameters from several views of a known calibration pattern (every view is described by several 3D-2D point correspondences).
- Estimate the relative position and orientation of the stereo camera “heads” and compute the rectification transformation that makes the camera optical axes parallel.
Note
- A calibration sample for 3 cameras in horizontal position can be found at opencv_source_code/samples/cpp/3calibration.cpp
- A calibration sample based on a sequence of images can be found at opencv_source_code/samples/cpp/calibration.cpp
- A calibration sample in order to do 3D reconstruction can be found at opencv_source_code/samples/cpp/build3dmodel.cpp
- A calibration sample of an artificially generated camera and chessboard patterns can be found at opencv_source_code/samples/cpp/calibration_artificial.cpp
- A calibration example on stereo calibration can be found at opencv_source_code/samples/cpp/stereo_calib.cpp
- A calibration example on stereo matching can be found at opencv_source_code/samples/cpp/stereo_match.cpp
- (Python) A camera calibration sample can be found at opencv_source_code/samples/python2/calibrate.py
calibrateCamera
Finds the camera intrinsic and extrinsic parameters from several views of a calibration pattern.
-
C++:
double
calibrateCamera
(InputArrayOfArrays
objectPoints, InputArrayOfArrays
imagePoints, Size
imageSize, InputOutputArray
cameraMatrix, InputOutputArray
distCoeffs, OutputArrayOfArrays
rvecs, OutputArrayOfArrays
tvecs, int
flags=0, TermCriteria
criteria=TermCriteria( TermCriteria::COUNT+TermCriteria::EPS, 30, DBL_EPSILON)
)
-
Python:
cv2.
calibrateCamera
(objectPoints, imagePoints, imageSize
[, cameraMatrix
[, distCoeffs
[, rvecs
[, tvecs
[, flags
[, criteria
]
]
]
]
]
]
) → retval, cameraMatrix, distCoeffs, rvecs, tvecs
-
C:
double
cvCalibrateCamera2
(const CvMat*
object_points, const CvMat*
image_points, const CvMat*
point_counts, CvSize
image_size, CvMat*
camera_matrix, CvMat*
distortion_coeffs, CvMat*
rotation_vectors=NULL, CvMat*
translation_vectors=NULL, int
flags=0, CvTermCriteria
term_crit=cvTermCriteria( CV_TERMCRIT_ITER+CV_TERMCRIT_EPS,30,DBL_EPSILON)
)
-
Python:
-
Parameters: - objectPoints –
In the new interface it is a vector of vectors of calibration pattern points in the calibration pattern coordinate space (e.g. std::vector<std::vector<cv::Vec3f>>). The outer vector contains as many elements as the number of the pattern views. If the same calibration pattern is shown in each view and it is fully visible, all the vectors will be the same. Although, it is possible to use partially occluded patterns, or even different patterns in different views. Then, the vectors will be different. The points are 3D, but since they are in a pattern coordinate system, then, if the rig is planar, it may make sense to put the model to a XY coordinate plane so that Z-coordinate of each input object point is 0.
In the old interface all the vectors of object points from different views are concatenated together.
- imagePoints –
In the new interface it is a vector of vectors of the projections of calibration pattern points (e.g. std::vector<std::vector<cv::Vec2f>>).
imagePoints.size()
andobjectPoints.size()
andimagePoints[i].size()
must be equal toobjectPoints[i].size()
for eachi
.In the old interface all the vectors of object points from different views are concatenated together.
- point_counts – In the old interface this is a vector of integers, containing as many elements, as the number of views of the calibration pattern. Each element is the number of points in each view. Usually, all the elements are the same and equal to the number of feature points on the calibration pattern.
- imageSize – Size of the image used only to initialize the intrinsic camera matrix.
- cameraMatrix – Output 3x3 floating-point camera matrix
. If
CV_CALIB_USE_INTRINSIC_GUESS
and/orCV_CALIB_FIX_ASPECT_RATIO
are specified, some or all offx, fy, cx, cy
must be initialized before calling the function. - distCoeffs – Output vector of distortion coefficients
of 4, 5, or 8 elements.
- rvecs – Output vector of rotation vectors (see
Rodrigues()
) estimated for each pattern view (e.g. std::vector<cv::Mat>>). That is, each k-th rotation vector together with the corresponding k-th translation vector (see the next output parameter description) brings the calibration pattern from the model coordinate space (in which object points are specified) to the world coordinate space, that is, a real position of the calibration pattern in the k-th pattern view (k=0.. M -1). - tvecs – Output vector of translation vectors estimated for each pattern view.
- flags –
Different flags that may be zero or a combination of the following values:
- CV_CALIB_USE_INTRINSIC_GUESS
cameraMatrix
contains valid initial values offx, fy, cx, cy
that are optimized further. Otherwise,(cx, cy)
is initially set to the image center (imageSize
is used), and focal distances are computed in a least-squares fashion. Note, that if intrinsic parameters are known, there is no need to use this function just to estimate extrinsic parameters. UsesolvePnP()
instead. - CV_CALIB_FIX_PRINCIPAL_POINT The principal point is not changed during the global optimization. It stays at the center or at a different location specified when
CV_CALIB_USE_INTRINSIC_GUESS
is set too. - CV_CALIB_FIX_ASPECT_RATIO The functions considers only
fy
as a free parameter. The ratiofx/fy
stays the same as in the inputcameraMatrix
. WhenCV_CALIB_USE_INTRINSIC_GUESS
is not set, the actual input values offx
andfy
are ignored, only their ratio is computed and used further. - CV_CALIB_ZERO_TANGENT_DIST Tangential distortion coefficients
are set to zeros and stay zero.
- CV_CALIB_FIX_K1,...,CV_CALIB_FIX_K6 The corresponding radial distortion coefficient is not changed during the optimization. If
CV_CALIB_USE_INTRINSIC_GUESS
is set, the coefficient from the supplieddistCoeffs
matrix is used. Otherwise, it is set to 0. - CV_CALIB_RATIONAL_MODEL Coefficients k4, k5, and k6 are enabled. To provide the backward compatibility, this extra flag should be explicitly specified to make the calibration function use the rational model and return 8 coefficients. If the flag is not set, the function computes and returns only 5 distortion coefficients.
- CV_CALIB_USE_INTRINSIC_GUESS
- criteria – Termination criteria for the iterative optimization algorithm.
- term_crit – same as
criteria
.
- objectPoints –
cv.
CalibrateCamera2
(objectPoints, imagePoints, pointCounts, imageSize, cameraMatrix, distCoeffs, rvecs, tvecs, flags=0
) → None
The function estimates the intrinsic camera parameters and extrinsic parameters for each of the views. The algorithm is based on [Zhang2000]and [BouguetMCT]. The coordinates of 3D object points and their corresponding 2D projections in each view must be specified. That may be achieved by using an object with a known geometry and easily detectable feature points. Such an object is called a calibration rig or calibration pattern, and OpenCV has built-in support for a chessboard as a calibration rig (see findChessboardCorners()
). Currently, initialization of intrinsic parameters (when CV_CALIB_USE_INTRINSIC_GUESS
is not set) is only implemented for planar calibration patterns (where Z-coordinates of the object points must be all zeros). 3D calibration rigs can also be used as long as initial cameraMatrix
is provided.
The algorithm performs the following steps:
- Compute the initial intrinsic parameters (the option only available for planar calibration patterns) or read them from the input parameters. The distortion coefficients are all set to zeros initially unless some of
CV_CALIB_FIX_K?
are specified. - Estimate the initial camera pose as if the intrinsic parameters have been already known. This is done using
solvePnP()
. - Run the global Levenberg-Marquardt optimization algorithm to minimize the reprojection error, that is, the total sum of squared distances between the observed feature points
imagePoints
and the projected (using the current estimates for camera parameters and the poses) object pointsobjectPoints
. SeeprojectPoints()
for details.
The function returns the final re-projection error.
Note
If you use a non-square (=non-NxN) grid and findChessboardCorners()
for calibration, and calibrateCamera
returns bad values (zero distortion coefficients, an image center very far from (w/2-0.5,h/2-0.5)
, and/or large differences between and
(ratios of 10:1 or more)), then you have probably used
patternSize=cvSize(rows,cols)
instead of using patternSize=cvSize(cols,rows)
in findChessboardCorners()
.