效果图
背景图片
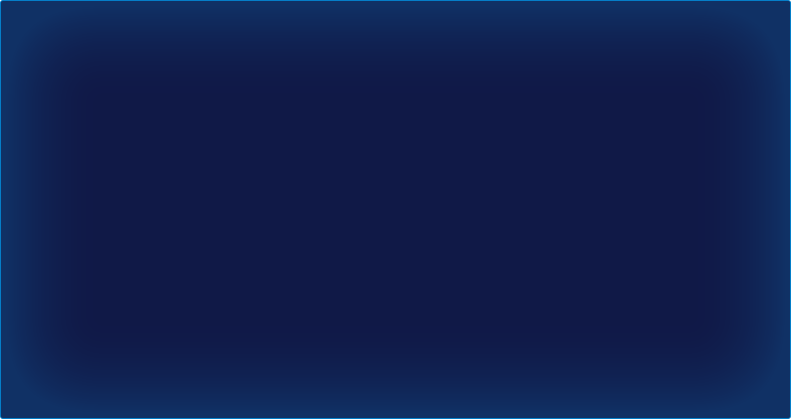
下载ECharts
npm install echarts --save
引入并注册全局ECharts
在 main.js 文件里引入并注册 ( 这里是 Vue3.0 的模板 )
import Vue from 'vue'
import App from './App.vue'
import router from './router'
import store from './store'
import echarts from 'echarts'
Vue.prototype.$echarts = echarts
Vue.config.productionTip = false
new Vue({
router,
store,
render: h => h(App)
}).$mount('#app')
在组件中使用ECharts
<template>
<div class='wrapper'>
<div class='chart' id='chart'></div>
</div>
</template>
<script>
export default {
data () {
return {}
},
mounted () {
this.drawChart()
},
methods: {
drawChart () {
// 基于准备好的dom,初始化echarts实例
const chart = this.$echarts.init(document.getElementById('chart'))
// 监听屏幕变化自动缩放图表
window.addEventListener('resize', function () { chart.resize() })
// 绘制图表
chart.setOption({
// 设置图表的位置
grid: {
x: 40, // 左间距
y: 70, // 上间距
x2: 50, // 右间距
y2: 30, // 下间距
containLabel: true // grid 区域是否包含坐标轴的刻度标签, 常用于『防止标签溢出』的场景
},
// dataZoom 组件 用于区域缩放
dataZoom: [
{
type: 'inside',
xAxisIndex: [0], // 设置 dataZoom-inside 组件控制的 x轴
// 数据窗口范围的起始和结束百分比 范围: 0 ~ 100
start: 0,
end: 100
}
],
// 图表主标题
title: {
text: '订单', // 主标题文本,支持使用 \n 换行
top: 10, // 定位 值: 'top', 'middle', 'bottom' 也可以是具体的值或者百分比
left: 'center', // 值: 'left', 'center', 'right' 同上
textStyle: { // 文本样式
fontSize: 24,
fontWeight: 600,
color: '#fff'
}
},
// 设置自定义文字
graphic: [
{
type: 'text', // 图形元素类型
left: 70, // 进行定位
bottom: 32,
style: { // 文本样式
fill: '#cdd3ee',
text: '(月份)',
font: 'normal 13px Microsoft' // style | weight | size | family
}
}
],
// 提示框组件
tooltip: {
trigger: 'axis', // 触发类型, axis: 坐标轴触发
axisPointer: {
type: 'line' // 指示器类型
},
textStyle: {
color: '#d5dbff' // 文字颜色
},
// 提示框浮层内容格式器,支持字符串模板和回调函数两种形式
// 折线(区域)图、柱状(条形)图、K线图 : {a}(系列名称),{b}(类目值),{c}(数值), {d}(无)
formatter: '{b}<br />{a0}: {c0}万<br />{a3}: {c3}%'
},
// 图例组件
legend: {
// 图例的数据数组
data: ['订单', '转化率'], // 图例项的名称 与 series 里的 name 相对应
top: 13, // 定位
right: 30,
textStyle: { // 文本样式
fontSize: 16,
color: '#cdd3ee'
}
},
// X轴
xAxis: {
show: true, // 不设置默认值为 true
type: 'category', // 坐标轴类型, 'category' 类目轴,适用于离散的类目数据,为该类型时必须通过 data 设置类目数据
// 坐标轴轴线
axisLine: {
lineStyle: {
type: 'solid', // 坐标轴线线的类型 'solid', 'dashed', 'dotted'
width: 1, // 坐标轴线线宽, 不设置默认值为 1
color: '#204756' // 坐标轴线线的颜色
}
},
// 坐标轴刻度
axisTick: {
show: false
},
// 分隔线
splitLine: {
show: false
},
// 坐标轴刻度标签
axisLabel: {
fontSize: 16, // 文字的字体大小
color: '#cdd3ee', // 刻度标签文字的颜色
// 使用函数模板 传入的数据值 -> value: number|Array,
formatter: function (value) {
return value.replace(/[\u4e00-\u9fa5]/g, '')
}
},
// 类目数据,在类目轴(type: 'category')中有效
data: ['1月', '2月', '3月', '4月', '5月', '6月', '7月', '8月', '9月', '10月', '11月', '12月']
},
yAxis: [
// 左侧Y轴
{
type: 'value', // 坐标轴类型, 'value' 数值轴,适用于连续数据
// 坐标轴刻度
axisTick: {
show: false // 是否显示坐标轴刻度 默认显示
},
// 坐标轴轴线
axisLine: { // 是否显示坐标轴轴线 默认显示
show: false, // 是否显示坐标轴轴线 默认显示
lineStyle: { // 坐标轴线线的颜色
color: '#204756'
}
},
// 坐标轴在 grid 区域中的分隔线
splitLine: {
show: true, // 是否显示分隔线,默认数值轴显示
lineStyle: {
color: '#204756', // 分隔线颜色
opacity: 0.5 // 分隔线透明度
}
},
// 坐标轴刻度标签
axisLabel: {
show: true, // 是否显示刻度标签 默认显示
fontSize: 16, // 文字的字体大小
color: '#cdd3ee', // 刻度标签文字的颜色
// 使用字符串模板,模板变量为刻度默认标签 {value}
formatter: '{value}'
}
},
// 右侧Y轴
{
type: 'value', // 坐标轴类型, 'value' 数值轴,适用于连续数据
min: 0, // 坐标轴刻度最小值
max: 100, // 坐标轴刻度最大值
splitNumber: 5, // 坐标轴的分割段数
// 坐标轴刻度
axisTick: {
show: false // 是否显示坐标轴刻度 默认显示
},
// 坐标轴轴线
axisLine: { // 是否显示坐标轴轴线 默认显示
show: false, // 是否显示坐标轴轴线 默认显示
lineStyle: { // 坐标轴线线的颜色
color: '#204756'
}
},
// 坐标轴在图表区域中的分隔线
splitLine: {
show: false // 是否显示分隔线 默认数值轴显示
},
// 坐标轴刻度标签
axisLabel: {
show: true,
fontSize: 16,
color: '#cdd3ee',
// 使用字符串模板,模板变量为刻度默认标签 {value}
formatter: '{value}%'
}
}
],
// 系列列表
series: [
// 最低下的圆片
{
name: '订单', // 系列名称, 用于tooltip的显示, legend 的图例筛选
type: 'pictorialBar', // 系列类型
symbolSize: [15, 10], // 标记的大小
symbolOffset: [0, 5], // 标记相对于原本位置的偏移
// 图例的图形样式
itemStyle: {
color: {
type: 'linear',
x: 0,
y: 0,
x2: 0,
y2: 1,
colorStops: [{
offset: 0,
color: '#d68e05' // 0% 处的颜色
}, {
offset: 1,
color: '#d68e05' // 100% 处的颜色
}]
}
},
z: 10, // 组件的所有图形的z值。控制图形的前后顺序。z值小的图形会被z值大的图形覆盖
data: [200, 330, 400, 600, 830, 650, 690, 430, 550, 420, 420, 320]
},
//下半截柱状图
{
name: '订单', // 系列名称,用于tooltip的显示,legend 的图例筛选
type: 'bar', // 系列类型
barWidth: 15, // 指定柱宽度,可以使用绝对数值或百分比,默认自适应
// 图例的图形样式
itemStyle: {
color: {
type: 'linear',
x: 0,
y: 0,
x2: 0,
y2: 1,
colorStops: [{
offset: 0,
color: '#96750c' // 0% 处的颜色
}, {
offset: 1,
color: '#9c6d2a' // 100% 处的颜色
}]
}
},
data: [200, 330, 400, 600, 830, 650, 690, 430, 550, 420, 420, 320]
},
// 最上面的圆片
{
name: '订单', // 系列名称, 用于tooltip的显示, legend 的图例筛选
type: 'pictorialBar', // 系列类型
symbolSize: [15, 10], // 标记的大小
symbolOffset: [0, -5], // 标记相对于原本位置的偏移
symbolPosition: 'end', // 图形的定位位置。可取值:start、end、center
// 图例的图形样式
itemStyle: {
color: {
type: 'linear',
x: 0,
y: 0,
x2: 0,
y2: 1,
colorStops: [{
offset: 0,
color: '#ffc600' // 0% 处的颜色
}, {
offset: 1,
color: '#ffc600' // 100% 处的颜色
}]
}
},
z: 10, // 组件的所有图形的z值。控制图形的前后顺序。z值小的图形会被z值大的图形覆盖
data: [200, 330, 400, 600, 830, 650, 690, 430, 550, 420, 420, 320]
},
{
name: '转化率', // 系列名称, 用于tooltip的显示, legend 的图例筛选
type: 'line', // 系列类型
smooth: true, // 是否平滑视觉引导线,默认不平滑,可以设置成 true 平滑显示,也可以设置为 0 到 1 的值,表示平滑程度
symbol: 'circle', // 标记的图形
symbolSize: 7, // 标记的大小
yAxisIndex: 1, // 使用的 y 轴的 index,在单个图表实例中存在多个 y轴的时候有用
// 图例图形中线的样式
lineStyle: {
type: 'solid', // 线的类型, 可选 solid、dashed、dotted
color: {
type: 'linear',
colorStops: [{
offset: 0,
color: '#2ec2fc' // 0% 处的颜色
}, {
offset: 1,
color: '#2cf8f0' // 100% 处的颜色
}],
},
width: 3, // 线宽
opacity: 1, // 图形透明度。支持从 0 到 1 的数字,为 0 时不绘制该图形
shadowColor: 'rgba(32,225,244, 0.3)', // 阴影颜色,支持的格式同color
shadowBlur: 3, // 图形阴影的模糊大小
shadowOffsetY: 15 // 阴影垂直方向上的偏移距离
},
// 图例的图形样式
itemStyle: {
color: '#2ec2fc', // 图形的颜色,默认从全局调色盘 option.color 获取颜色
borderColor: '#fff', // 图形的描边颜色
borderWidth: 2 // 描边线宽,为 0 时无描边
},
z: 20, // 组件的所有图形的z值。控制图形的前后顺序。z值小的图形会被z值大的图形覆盖
data: [30, 35, 40, 55, 89, 60, 52, 34, 33, 24, 30, 20]
}
]
})
}
}
}
</script>
<style scoped>
.wrapper {
width: 100%;
}
.wrapper .chart {
width: 60%;
height: 400px;
margin: 100px auto 0;
background: url(../../public/static/bg.png) no-repeat;
background-size: 100% 100%;
}
</style>