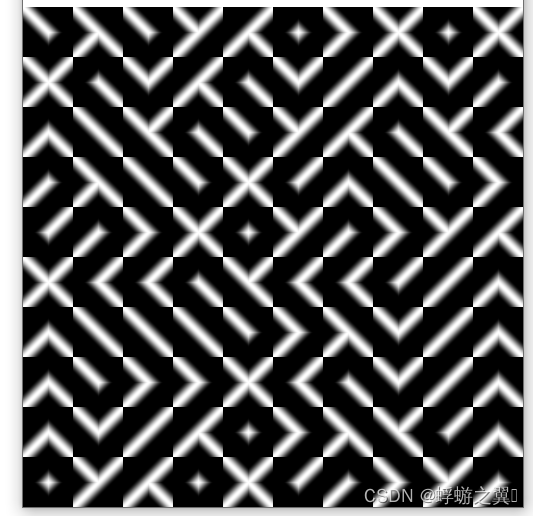
#include <opencv2/opencv.hpp>
#include <math.h>
using namespace cv;
Size u_resolution(500, 500);
Point2f u_mouse;
float u_time;
float fract(float value) {
return value - floor(value);
}
float dot(const float x, const float y, const Point2f& v2) {
return v2.dot(Point2f(x,y));
}
float random(Point2f st) {
return fract(sin(dot(st.x, st.y, Point2f(12.9898, 78.233))) * 43758.5453123);
}
Point2f truchetPattern(Point2f st, float index) {
index = fract((index - 0.5) * 2.0);
if (index > 0.75) {
st = Point2f(1.0, 1.0) - st;
}
else if (index > 0.5) {
st = Point2f(1.0 - st.x, st.y);
}
else if (index > 0.25) {
st = Point2f(1.0 - st.x, st.y);
}
return st;
}
float smoothstep(float t1, float t2, float x) {
x = std::clamp((double)(x - t1) / (t2 - t1), 0.0, 1.0);
return x * x * (3 - 2 * x);
}
int main() {
Mat img(u_resolution, CV_32FC1);
Point2f st;
for (int i = 0; i < img.rows; i++) {
for (int j = 0; j < img.cols; j++) {
st.x = (float)j / u_resolution.width;
st.y = (float)i / u_resolution.height;
st *= 10.0;
Point2i ipos = st;
Point2f fpos = Point2f{ fract(st.x) ,fract(st.y)};
Point2f tile = truchetPattern(fpos, random(ipos));
float color = 0.0;
color = smoothstep(tile.x - 0.3, tile.x, tile.y) -
smoothstep(tile.x, tile.x + 0.3, tile.y);
img.at<float>(i, j) = color;
}
}
imshow("Truchet Pattern", img);
waitKey(0);
return 0;
}
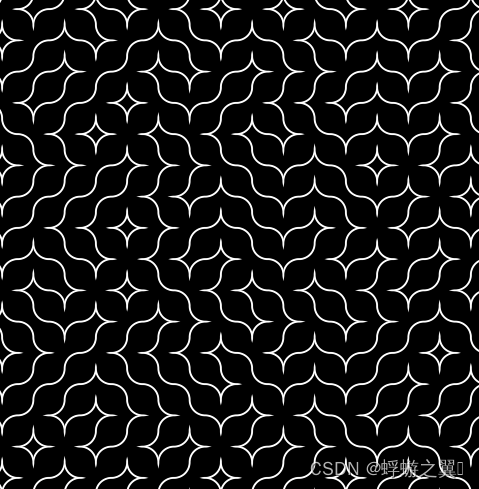
void arcMaze() {
Mat img(u_resolution, CV_32FC1);
Point2f st;
for (int i = 0; i < img.rows; i++) {
for (int j = 0; j < img.cols; j++) {
st.x = (float)j / u_resolution.width;
st.y = (float)i / u_resolution.height;
st *= 16;
Point2i ipos = st;
Point2f fpos = Point2f{ fract(st.x) ,fract(st.y) };
Point2f tile = truchetPattern(fpos, random(ipos));
float color = 0.0;
float dis = std::sqrt(tile.x * tile.x + tile.y * tile.y);
Point2f tile2 = tile - Point2f{1,1};
float dis2 = std::sqrt(tile2.x * tile2.x + tile2.y * tile2.y);
color = smoothstep(0.45, 0.49, dis) - smoothstep(0.51, 0.55, dis)+
smoothstep(0.45, 0.49, dis2) - smoothstep(0.51, 0.55, dis2);
img.at<float>(i, j) = color;
}
}
imshow("Truchet Pattern", img);
waitKey(0);
}