本文源码下载地址:http://www.80cxy.com/Blog/ResourceView?arId=202403191532545995NAAqJh
系列教程地址:http://www.80cxy.com/Blog/ArticleView?arId=202403191517574161ay3s5V
本文实现访问接口时对前端传过来的token进行校验配置,也可以说是项目权限校验。首页配置项目启用JWT校验,然后通过Filter进行校验。
一、配置JWT校验
SignUp.Common项目WebApplicationBuilderExtensions类的ConfigureExtraServices方法增加如下配置代码:
1 2 | JWTOptions jwtOpt = configuration.GetSection( "JWT" ).Get<JWTOptions>(); services.AddJWTAuthentication(jwtOpt); |
AddJWTAuthentication开展方法代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | using Microsoft.AspNetCore.Authentication; using Microsoft.AspNetCore.Authentication.JwtBearer; using Microsoft.Extensions.DependencyInjection; using Microsoft.IdentityModel.Tokens; using System.Text; namespace SignUp.Common.JWT { public static class AuthenticationExtensions { /// <summary> /// 对JWT进行配置 /// </summary> /// <param name="services"></param> /// <param name="jwtOpt"></param> /// <returns></returns> public static AuthenticationBuilder AddJWTAuthentication( this IServiceCollection services, JWTOptions jwtOpt) { return services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme) .AddJwtBearer(x => { x.TokenValidationParameters = new () { ValidateIssuer = true , ValidateAudience = true , ValidateLifetime = true , ValidateIssuerSigningKey = true , ValidIssuer = jwtOpt.Issuer, ValidAudience = jwtOpt.Audience, IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(jwtOpt.SecKey)) }; }); } } } |
在Program.cs文件app.MapControllers();代码上面增加如下配置:
1 2 3 | app.UseForwardedHeaders(); app.UseAuthentication(); app.UseAuthorization(); |
二、实现IAuthorizationFilter过滤器
SignUp.Common项目Filter文件夹创建ApiAuthorizeFilter类,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | using Microsoft.AspNetCore.Authorization; using Microsoft.AspNetCore.Mvc; using Microsoft.AspNetCore.Mvc.Filters; using SignUp.Common.Commons; using SignUp.Common.Enum; using SignUp.Common.Extensions; using System.IdentityModel.Tokens.Jwt; namespace SignUp.Common.Filter { /// <summary> /// 权限过滤 /// </summary> public class ApiAuthorizeFilter : IAuthorizationFilter { private static readonly string replaceTokenPath = "/api/AdminLogin/ReplaceToken" ; public void OnAuthorization(AuthorizationFilterContext context) { //控制器标记AllowAnonymous属性直接跳过 if (context.ActionDescriptor.EndpointMetadata.Any(c => c.GetType().Equals( typeof (AllowAnonymousAttribute)))) { return ; } // 获取当前用户的信息 var user = context.HttpContext.User; // 如果用户未登录返回无权访问 if (user == null || !user.Identity.IsAuthenticated) { context.Result = new JsonResult( new ResponseContent().Error(ResponseType.LoginExpiration)); return ; } //获取token过期时间 DateTime expDate = context.HttpContext.User.Claims.Where(x => x.Type == JwtRegisteredClaimNames.Exp) .Select(x => x.Value).FirstOrDefault().GetTimeSpmpToDate(); //判断jwt是否过期 if ((expDate - DateTime.Now).TotalMinutes < 0) { context.Result = new JsonResult( new ResponseContent().Error(ResponseType.TokenExpiration)); return ; } //前端刷新token标识,离过期5分钟时开始刷新 if ((expDate - DateTime.Now).TotalMinutes < 5 && context.HttpContext.Request.Path != replaceTokenPath) { context.HttpContext.Response.Headers.Add( "vol_exp" , "1" ); } return ; } } } |
三、接口增加校验规则
在需要JWT进行校验的接口上增加ApiAuthorizeFilter特性,代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | /// <summary> /// 获取用户信息 /// </summary> /// <returns></returns> [HttpPost] [TypeFilter( typeof (ApiAuthorizeFilter))] public async Task<ActionResult<ResponseContent>> GetUserInfo() { ResponseContent response = new ResponseContent(); string userId = this .User.FindFirstValue(ClaimTypes.NameIdentifier); var user = await _systemDomainService.FindOneUserById(userId); if (user == null ) //可能用户注销了 { return new JsonResult(response.Error(ResponseType.LoginExpiration)); } //出于安全考虑,不要机密信息传递到客户端 //除非确认没问题,否则尽量不要直接把实体类对象返回给前端 return new JsonResult(response.Ok( new { userId = user.Id, userName = user.UserName, avatar = "" , routes = new string [] { "Audit" , "Preliminary" , "Recheck" , "System" , "User" , "Menu" , "Role" , "IndexAuth" }, roles = new string [] { "Matt" , "Joanne" , "Robert" }, buttons = new string [] { "Matt" , "Joanne" , "Robert" } })); } |
学习交流
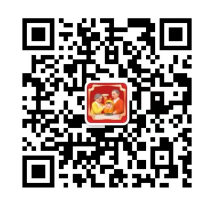
附笔者学习 .net core开发时参考相关项目实例源码:asp.net core webapi项目实例源代码锦集下载(72个)