Time Limit: 2000MS | Memory Limit: 65536K | |
Total Submissions: 1753 | Accepted: 821 |
Description
Wintokk has collected a huge amount of coins at THU. One day he had all his coins fallen on to the ground. Unfortunately, WangDong came by and decided to rob Wintokk of the coins.
They agreed to distribute the coins according to the following rules:
Consider the ground as a plane. Wintokk draws a horizontal line on the plane and then WangDong draws a vertical one so that the plane is divided into 4 parts, as shown below.
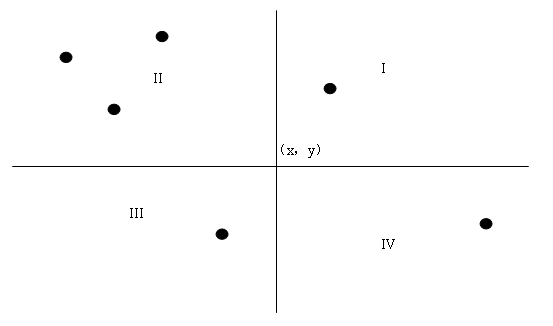
Wintokk will save the coins in I and III while those fit in II and IV will be taken away by the robber WangDong.
For fixed locations of the coins owned by Wintokk, they drew several pairs of lines. For each pair, Wintokk wants to know the difference between the number of the saved coins and that of the lost coins.
It's guaranteed that all the coins will lie on neither of the lines drew by that two guys.
Input
The first line contains an integer T, indicating the number of test cases. Then T blocks of test cases follow. For each case, the first line contains two integers N and M, where N is the number of coins on the ground and M indicates how many times they are going to draw the lines. The 2nd to (N+1)-th lines contain the co-ordinates of the coins and the last M lines consist of the M pairs integers (x, y) which means that the two splitting lines intersect at point (x, y).
(N,M ≤ 50000, 0 ≤x,y ≤ 500000)
Output
For each query, output a non-negative integer, the difference described above. Output a blank line between cases.
Sample Input
2 10 3 29 22 17 14 18 23 3 15 6 28 30 27 4 1 26 7 8 0 11 21 2 25 5 10 19 24 10 5 28 18 2 29 6 5 13 12 20 27 15 26 11 9 23 25 10 0 22 24 16 30 14 3 17 21 8 1 7 4
Sample Output
6 4 4 2 2 4 4 4
#include <stdio.h> #include <algorithm> #include <string.h> using namespace std; #define maxn 500005 int c1[maxn], c2[maxn]; int res[50001]; struct point{ int x, y; bool operator < (const point& v){ return y < v.y; } }a[50001]; struct query{ int x, y, id; bool operator < (const query& v){ return y < v.y; } }q[50001]; inline int lowbit(int x){ return x & (-x); } void add(int c[], int x){ while(x < maxn){ c[x]++; x += lowbit(x); } } int getsum(int c[], int x){ int ans = 0; while(x){ ans += c[x]; x -= lowbit(x); } return ans; } int main(){ int T, n, m; scanf("%d", &T); while(T--){ memset(c1, 0, sizeof(c1)); memset(c2, 0, sizeof(c2)); scanf("%d %d", &n, &m); for(int i = 1; i <= n; ++i){ scanf("%d %d", &a[i].x, &a[i].y); a[i].x++; a[i].y++; add(c1, a[i].x); } for(int i = 1; i <= m; ++i){ scanf("%d %d", &q[i].x, &q[i].y); q[i].x++; q[i].y++; q[i].id = i; } sort(a + 1, a + 1 + n); sort(q + 1, q + 1 + m); int pos = 1; for(int i = 1; i <= m; ++i){ while(a[pos].y < q[i].y && pos <= n){ add(c2, a[pos].x); pos++; } res[q[i].id] = getsum(c2, q[i].x) + n - getsum(c1, q[i].x) - (getsum(c2, maxn - 1) - getsum(c2, q[i].x)); } for(int i = 1; i <= m; ++i){ printf("%d\n", abs(2 * res[i] - n)); } if(T){ printf("\n"); } } } /* 题意:5e4个点,5e5次询问,每次询问给出两条分别平行于X,Y的直线,问1,3象限和2,4象限点数的差。 思路:如果点数不多,应该可以二维数组搞一搞,然而5e4的范围只能一维搞了。那么我们离线处理 先把所有点和询问读进来,然后按y从小到大排序。开两个树状数组,c1把所有点的x加进去。然后由于询问 已按Y排序,对于每次询问,我们先将y小于该询问的点的x加到c2中,那么getsum(c2, q[i].x)就是该询问 第3象限的点数(假设原坐标系原点在左下角),getsum(c1, q[i].x)就是该询问第2,3象限的点数和,n - getsum(c1, q[i].x) 就是该询问第1,4象限的点数和,getsum(c2, maxn - 1)就是该询问第3,4象限的点数和。加加减减搞一搞就行了。 然后按顺序输出答案。 */