import math
import random
from random import sample
import numpy as np
from numpy import *
import geatpy as ea
import xlrd
##已知数据
q1='F:\共享车选址调度\共享汽车数据\候选点之间的OD(13).xlsx'
T1='F:\共享车选址调度\共享汽车数据\候选点之间的最短期望时间Tik(13).xlsx'
wt1='F:\共享车选址调度\共享汽车数据\需求中心到备选点的步行时间(50行13列).xlsx'
D1='F:\共享车选址调度\共享汽车数据\每个需求中心的需求量(50网格).xlsx'
# ####excel转为矩阵
def excel_to_matrix(path,a): #路径,sheet
table = xlrd.open_workbook(path).sheets()[a]#获取第一个sheet表
row = table.nrows # 行数
col = table.ncols # 列数
datamatrix = np.zeros((row, col))#生成一个nrows行ncols列,且元素均为0的初始矩阵
for x in range(col):
cols = np.matrix(table.col_values(x)) # 把list转换为矩阵进行矩阵操作
datamatrix[:, x] = cols # 按列把数据存进矩阵中
return datamatrix
q=excel_to_matrix(q1,0)
T=excel_to_matrix(T1,0)
wt=excel_to_matrix(wt1,0)
D=excel_to_matrix(D1,1) # 多维数组,50行1列
# #############参数##########################
Cs=1*10
Cp=12*10
Cv=56*10
Ce=6
M=10000
alpha=0.8
beta=0.5
gama=2
I=13
J=50
Pmax=100
w1=50
H =500000 # 成本的最大值
##########################################
##距离衰减
s=zeros((J, I)) # 创建一个J*I的零矩阵,矩阵这里zeros函数的参数是一个tuple类型(J,I)
for i in range(I):
for j in range(J):
if wt[j,i]<w1:
a=-(wt[j,i])**4+w1**4
b=(w1**4)*(math.exp((wt[j,i]/40)**3))
s[j,i]=a/b
elif wt[j,i]>w1:
s[j,i]=0
## qik/qi
n=zeros((J, I))
for i in range(I):
for k in range(I):
if sum(q[i])>0: #每一行的和
n[i,k]=q[i,k]/sum(q[i])
else:
n[i,k]=0
'''1.目标函数'''
def objection(X,P,V,z):
z0=np.array(z).reshape(J,I) #列表转一维数组,转多维数组
for i in range(I):
Ti = T[i, :] # 多维数组,第i行
ni = n[i, :]
ui= [sum(D[:,0]*z0[:,i]*s[:,i]) for i in range(I)] # 输出为一个列表,其中含有13个元素,表示分布从13个备选站点出发的租车辆
# ob = [Cs * X[i] + Cp * P[i] + Cv * V[i] + Ce * sum(Ti * ni) * ui[i] for i in range(I)] # 输出为一个列表,其中含有13个元素,每个元素对应一个站点的成本
obj=sum([Cs * X[i] + Cp * P[i] + Cv * V[i] + Ce * sum(Ti * ni) * ui[i] for i in range(I)]) #输出结果为总成本,约29万
return obj
'''2.实数编码'''
def code():
Chrom = []
X_0 = np.random.randint(0, 2, I) # 随机产生13个只含0,1元素的列表,13为初始种群的个数
Chrom.extend(X_0)
V_0 = np.random.randint(0, 101, I) # 随机产生13个0到100中的正整数的列表
Chrom.extend(V_0)
P_0 = np.random.randint(0, 81, I) # 随机产生13个0到80中的正整数的列表.80=100*alpha
Chrom.extend(P_0)
z0 = np.random.random((J, I)) # J=50,I=30,50行13列的二维数组
z_0 = z0.flatten() # 转化为一维数组,按行转化
Chrom.extend(z_0)
u_0 = [sum(D[j, 0] * z_0[I * j + i] * s[j, i] * n[i, k] for j in range(J)) for i in range(I) for k in range(I)] # 含有I*I=169个元素,uik
Chrom.extend(u_0)
# print(Chrom) # 输出为一个列表,其中共(50+3)*13+169=858个元素
return Chrom
def initial_pop(Nind):
pop=[]
i=0
while i<=Nind:
IND=code()
pop.append(IND)
i += 1
return pop
'''3.区分各个变量'''
def Chrom_to_var(Chrom):
X=Chrom[:I] #前13个元素为X
V=Chrom[I:2*I]
P=Chrom[2*I:3*I]
z=Chrom[3*I:3*I+I*J]
u=Chrom[3*I+I*J:]
return X,V,P,z,u
'''5.需求约束'''
def demand_feasible(Chrom):
X,V,P,z,u=Chrom_to_var(Chrom)
g5 =[z[I*j+i]-X[i] for i in range(I) for j in range(J)] # J*I个约束
# g5_n = np.array(g5).reshape(J, I) # 将一维列表转化为J行I列的数组
# g5_c = [sum(g5_n[:, i]) for i in range(I)] # 对每一列求和,得到每个染色体(每个站点)对应的约束,输出为含有I个元素的列表
g6 =[sum(z[I * j + i] for i in range(I))-1 for j in range(J)] # J个约束
g7 =[sum(D[j,0]*z[I*j+i] for i in range(I))-D[j,0] for j in range(J)] # J个约束
g8 =[sum(D[j,0]*z[I*j+i] for j in range(J))-P[i] for i in range(I)] # I个约束
g9 =[beta*sum(D[j,0] for j in range(J))-sum(sum(D[j,0]*z[I*j+i]*s[j,i] for i in range(I)) for j in range(J))] # 1个约束
# g10 =[sum(D[j,0]*z[I*j+i]*s[j,i]*n[i,k] for j in range(J))-u[I*i+k] for i in range(I) for k in range(I)] # I*I个约束
# g11 =[u[I*i+k]-sum(D[j,0]*z[I*j+i]*s[j,i]*n[i,k] for j in range(J)) for i in range(I) for k in range(I)] # I*I个约束
g12 =[sum(u[I*i+k] for k in range(I))-V[i] for i in range(I)] # I个约束
g13 =[sum(u[I*i+k] for i in range(I) for k in range(I))*0.657+11.89-sum(V[i] for i in range(I))] # 1个约束
g_1 = g5+g6+g7+g8+g9+g12+g13 # 将前面所以约束放到同一个列表中,1116个元素
g_pos = sum([abs(i) for i in g_1]) # 将g_1中的元素转化为正值并求和
num_cons = I*J+J+J+I+1+I+1
rhs = [0] * num_cons # 输出为有I*J+J+J+I+1+I*I+I*I+I+1个元素的列表,每个元素都是0
cons_1 = [g_1[m] <= rhs[m] for m in range(len(rhs))] # 前面将约束写为<=0的形式,这里进行判断,输出为[True,False……]
return g_1,g_pos,cons_1
'''6.产生初始种群'''
def initial_pop(Nind): # Nind为种群数量
pop=[]
i=0
while i<Nind:
IND=code()
pop.append(IND)
i += 1
return pop # 输出为含有Nind个染色体的种群,列表
'''7.计算适应度'''
def get_fitness(pop, Nind,C,c): # C是一个足够大的数,目标函数的最大估计
fit=[]
for i in range(Nind):
X=Chrom_to_var(pop[i])[0]
P = Chrom_to_var(pop[i])[1]
V = Chrom_to_var(pop[i])[2]
z = Chrom_to_var(pop[i])[3]
H=objection(X,P,V,z)
if all(demand_feasible(pop[i])[2]) is True:
fitness=C-H # Z为目标函数
else:
fitness=C-H-c*demand_feasible(pop[i])[1] #g(x)为约束条件,c为正值常数
fit.append(fitness) # 将每次循环的结果存入列表中,输出为[89688.98555388054, 144668.3419467736, 97700.61376721218, 173935.77171023752, 160989.79620219418]
return fit
'''8.适应度排序'''
def fit_sort(fit):
fit2 = fit[:] # 通过分片操作将列表FitnV_l的元素全部拷贝给FitnV2,产生一个新副本,不改变fit的适应度排序,便于后面染色体的索引
fit2.sort(reverse=True) # 适应度降序排序,产生一个排序好的副本,同时保持原有列表不变
return fit2
'''8.得到精英个体'''
def get_elite(pop,fit,fit2,eli_n):
Se_eli =fit2[:eli_n] #选择前eli_n个个体直接进入子代
See_ind = [fit.index(i) for i in Se_eli] #得到被选择个体的索引
pop_e=[]
for i in See_ind:
pop_e.append(pop[i])
return pop_e # 返回值为直接进入子代的精英个体
'''9.自然选择:轮盘赌'''
def selection(pop,fit,Nsel):
# USe_eli = fit2[eli_n:] # 精英选择中未被选择的个体
USe_eli1=np.array(fit).reshape(7,1) # 转化为多维数组,N为种群数量
se_ind =ea.rws(USe_eli1,Nsel) # 得到被选择个体的索引, Nsel被选择个体的数目
pop_s = []
for i in se_ind:
pop_s.append(pop[i])
return pop_s
# pop_1 = selection(pop,fit,Nsel) # 参与交叉的个体
# print(pop_1)
'''10.交叉:线性交叉'''
def crossover(pop_s,pc):
j = 0
while j <= 2:
chrom = sample(pop_s, 2) # 抽取pop_1中的两个个体染色体进行交叉。列表
# print(chrom)
chrom_ind = [pop_s.index(i) for i in chrom] # 得到进行交叉的染色体在pop_1中的索引
# n_chorm=pop_1[row_rand_array[2:]]
c_point1 = random.randint(0, len(chrom[0])-1)
c_point2 = random.randint(0, len(chrom[0])-1)
if c_point1 <= c_point2:
s_point = c_point1
e_point = c_point2
else:
s_point = c_point2
e_point = c_point1
# print(s_point, e_point)
r = random.random()
if r <= pc:
new_C = chrom
# print(new_C)
else:
a = random.random()
b = random.random()
# print(a, b)
chrom_n1 = chrom[0] # 父代1
chrom_n2 = chrom[1] # 父代2
for i in range(s_point, e_point + 1): # 遍历每个交叉点
Ge1 = chrom_n1[i]
Ge2 = chrom_n2[i]
# print(Ge1, Ge2)
if 0<=i<=3*I:
Ge1 = int(Ge2 + a * (Ge1 - Ge2))
Ge2 = int(Ge1 + b * (Ge2 - Ge1)) # 交叉后的基因
else:
Ge1 = Ge2 + a * (Ge1 - Ge2)
Ge2 = Ge1 + b * (Ge2 - Ge1)
# print(Ge1, Ge2)
chrom_n1[i] = Ge1
chrom_n2[i] = Ge2 #更新染色体
# print(chorm_n1, chorm_n2)
# print(chorm_n1, chorm_n2) # 注意缩进,只要最后每个点交叉后最终的迭代结果
pop_s[chrom_ind[0]] = chrom_n1
pop_s[chrom_ind[1]] = chrom_n2 # 更新整个种群,将交叉后的染色体存入种群中
j+=2
return pop_s
def mutate(pop_s,pm):
j = 0
while j <= 1:
px = len(pop_s) # 种群中染色体的数目
py = len(pop_s[0]) #每个染色体中基因的数目
im = random.randint(0, px - 1) # im为进行变异的染色体的索引
# chrom_m = pop_1[im] # 进行变异的染色体
# 判断每条染色体的每个基因是否需要变异
for i in range(py):
if (0.5 <= pm):
pop_s[im][i] = pop_s[im][i]
else:
if 0 <= i <= I-1:
if pop_s[im][i]==0:
pop_s[im][i] = 1 # 变量的上下界
else:
pop_s[im][i]=0
elif i <=2*I-1:
pop_s[im][i] = random.randint(0, 100)
elif i <=3*I-1:
pop_s[im][i] = random.randint(0, 80)
else:
pop_s[im][i] = random.uniform(0, 1)
j+=1
return pop_s
pop = initial_pop(7) # 初始种群,内有7个个体,列表
print(pop)
print(len(pop))
fit = get_fitness(pop, 7, 500000, 0.5) # 得到每个个体的适应度,列表
fit2 = fit_sort(fit) #得到降序排列的适应度
pop_e = get_elite(pop, fit, fit2, 2) # 选取2个精英个体直接进入子代
pop_s = selection(pop, fit, 6) # 从初始种群中选择6个进行遗传操作
pop_c = crossover(pop_s, 0.7) # 得到交叉后的个体,列表,内含6个个体
# print(pop_c)
# print(len(pop_c))
fit_c=get_fitness(pop_c,6,500000,0.5) # 得到交叉后的种群中的每个个体的适应度
fit_c2=fit_sort(fit_c) #得到降序排列的适应度
pop_e2=get_elite(pop_c,fit_c,fit_c2,2) # 从交叉后的种群中选择eli_n个直接进入子代
pop_m = mutate(pop_c, 0.2) # 得到变异后的种群
# print(pop_m)
# print(len(pop_m))
fit_m=get_fitness(pop_m,6,500000,0.5) # 得到交叉后的种群中的每个个体的适应度
fit_m2=fit_sort(fit_m)
pop_e3 = get_elite(pop_c, fit_m, fit_m2, 3) # 种群中共7个个体,父代和交叉后各两个直接进入子代,则变异后只需适应度前3的个体
pop_new=pop_e+pop_e2+pop_e3
print(pop_new)
print(len(pop_new))
obj=[]
for i in range(len(pop_new)):
Chrom_a = pop_new[i] # 子代中的每一个个体
X = Chrom_to_var(Chrom_a)[0]
P = Chrom_to_var(Chrom_a)[1]
V = Chrom_to_var(Chrom_a)[2]
z = Chrom_to_var(Chrom_a)[3]
obj_a = objection(X, P, V, z) # 一个个体的目标函数值
obj.append(obj_a) # obj中包括每一个个体的目标值,列表
obj_max = max(obj) # 得到子代中的最优个体的目标值
opti_index = obj.index(obj_max) # 得到最优目标值的索引
chrom_opti = pop_new[opti_index] # 得到最优目标值对应的最优个体
print(obj)
print(obj_max)
print(opti_index)
print(chrom_opti)
'''输出
新子代,有7个个体,每个个体有13+13+13+13*50个基因[[1, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 1, 1, 84, 56, 90, 7, 83, 7, 70, 79, 83, 2, 25, 6, 12, 41, 55, 11, 37, 36, 22, 30, 60, 53, 26, 42, 76, 9, 0.2833708041723647, 0.7035236449013784, 0.7388995125388688, 0.48601576190786533, 0.2748826981715806, 0.039950877158715925, 0.16036818198567426, 0.19710310842524026, 0.7468459749469089, 0.7499767014939938, 0.4290164784457722, 0.7110683048051443, 0.25738837207037624, 0.5034051868008438, 0.5300125512194759, 0.3368547865639494, 0.3135944910640064, 0.2054372472223679, 0.4696002890084563, 0.7895281584830338, 0.18325766723807801, 0.7164713007967947, 0.43747370323031554, 0.050020773994953194, 0.8082241069163905, 0.37542308615545583, 0.4146978484294387, 0.5247658633386343, 0.733292481320159, 0.07597589357651602, 0.3423119969657896, 0.612209476356749, 0.6466737500712026, 0.7224583133191287, 0.8600667515690195, 0.42370752188767247, 0.14067462161252697, 0.009895832604997601, 0.685064045850556, 0.01202891386376026, 0.576184260407531, 0.2736271580417231, 0.5155178373835586, 0.9600945082477527, 0.20759515228684877, 0.47488272867104453, 0.6786065039244358, 0.06683235252297293, 0.07343563629591787, 0.9140581062911858, 0.3189679010422809, 0.334561487032055, 0.24672100999091584, 0.8123972054912327, 0.06558884769196227, 0.5529918690345262, 0.6037244411282147, 0.716051115525277, 0.4336743257702663, 0.16325396014255422, 0.054993588524897996, 0.1279936568538298, 0.0240161168337365, 0.5799055931478346, 0.7838885304900828, 0.2293742318247417, 0.4970616236747808, 0.708787962632707, 0.2860046515895671, 0.3084265738857386, 0.23452181073409661, 0.8108243035763218, 0.5634669774839416, 0.5689526536096937, 0.993344116121792, 0.623443291404663, 0.21687371367745545, 0.757031487958901, 0.610180159800997, 0.561174063498708, 0.8328924019129954, 0.17419577342823245, 0.028017318190715956, 0.477502301320906, 0.16141081503780264, 0.8830647968934745, 0.09534133918285947, 0.7866518624938906, 0.9934221140725785, 0.2832665474402212, 0.17000926260568283, 0.9934873891706184, 0.886024883644926, 0.1299624179819604, 0.1111481725548642, 0.4591560143075285, 0.10157970691384344, 0.014094307202021983, 0.010197896807318418, 0.19545555834089456, 0.14494952645350134, 0.43048644089986476, 0.7141209338082033, 0.4397175070120105, 0.8189055014268195, 0.9407003373501358, 0.10909983310294247, 0.7729051824782391, 0.7831682912842023, 0.9481847791754093, 0.8348213206664009, 0.6498876901254581, 0.060241367303420224, 0.9532180020743294, 0.523072914242004, 0.06175465493167143, 0.9680240389423339, 0.3113259996323502, 0.5171621835474615, 0.07367331439798763, 0.14099115368130655, 0.6085110426514192, 0.4694534440307737, 0.858878836327553, 0.6088547527736664, 0.5681892747877076, 0.02694737106238032, 0.5527309904490509, 0.1974527834474561, 0.8091847314293691, 0.6299042810106582, 0.7216305960770245, 0.36988795069769786, 0.6398001481235833, 0.2302235666058875, 0.5483504420746514, 0.7864609028106812, 0.8918090959879027, 0.49317616750674653, 0.9557940629960421, 0.4000491711380615, 0.4099000713626487, 0.9694604743519426, 0.2988384942792335, 0.42476903948660194, 0.46909620886657366, 0.44276871303967247, 0.910475060255931, 0.6051690491676255, 0.6536870973728024, 0.2736083089611707, 0.03795474412106292, 0.018753879360228876, 0.22186683067266433, 0.31034931169699576, 0.5798111327225284, 0.6920634142414784, 0.7071245607679228, 0.7877069740854957, 0.7452304323035801, 0.35778604275022885, 0.7709207728984306, 0.7915086657886373, 0.6916798737341032, 0.49811661003479324, 0.4355474218081147, 0.3838838697902487, 0.9599430701293478, 0.014319926434680719, 0.3177968122318099, 0.48716437285999703, 0.018414041075854093, 0.5792867954485518, 0.6963394268277495, 0.2740463646196546, 0.06910952525538738, 0.7020816782259427, 0.6975953980438678, 0.4303469395328092, 0.0020682712922966973, 0.7259971306333394, 0.9329150950563961, 0.974357208837245, 0.0866592173678481, 0.8696443425042825, 0.5245706001354662, 0.9014012657046958, 0.2930368238467712, 0.36822627331000646, 0.8480793252907031, 0.2746418984589174, 0.18699801757734713, 0.6457173440125646, 0.6450686884101253, 0.3759242168017015, 0.01999035783841374, 0.13715679189977625, 0.42461620509965403, 0.9817779339748008, 0.28202335380220445, 0.5599524450917294, 0.9534291657252688, 0.19622374878245663, 0.2532942481006334, 0.6798381533422061, 0.8299423720662756, 0.44350169067102774, 0.1055891869194453, 0.3125197721598114, 0.20741270558702707, 0.38394230087196124, 0.5904554914770634, 0.2707135544924827, 0.6041570854060168, 0.2784703853792818, 0.9496897066570366, 0.22504406934349863, 0.5681642519095623, 0.5949543445403096, 0.4682542199653269, 0.06807314904991102, 0.8538304847430515, 0.0021831025590065556, 0.02881063332031697, 0.019247633640714024, 0.35265015134205924, 0.6340756596074717, 0.180918057709048, 0.5902855851580664, 0.2710138743926269, 0.232527198350155, 0.9635753485595174, 0.7936400938705865, 0.9987126035993594, 0.15371102860267738, 0.6070451517478118, 0.8732555647656223, 0.6283097343012675, 0.9305182966022708, 0.5666027181090817, 0.9949989781470769, 0.7893319982424167, 0.1479640000863306, 0.7006138569650234, 0.41299940763695964, 0.3169395414542855, 0.9226024177531421, 0.2611607932977277, 0.4275006569279959, 0.4891505903343958, 0.8457558708985989, 0.6048269231512637, 0.08841244967779738, 0.31975921362140525, 0.6927586513674949, 0.7845591470613895, 0.9166625197633945, 0.9549828145309351, 0.21951554000005657, 0.9467817725922602, 0.6763008297856303, 0.5352381176655896, 0.41954294783758805, 0.030521478733030682, 0.21658519651052455, 0.436024895417776, 0.03714193396387755, 0.5571813459254545, 0.13608641384652032, 0.02413711937877827, 0.20661307602080548, 0.039082368009280444, 0.5620692654111155, 0.2157876374687675, 0.1662045065041058, 0.23420150812828644, 0.2809271775557458, 0.9232589680473885, 0.468862150735582, 0.6637209170662617, 0.8100035225598272, 0.5767296950709919, 0.19663546334488746, 0.9541214968518962, 0.09461303259321319, 0.9953812289680259, 0.8269621200690463, 0.9051950944246279, 0.0020443558722578192, 0.34508950680332207, 0.6018016393718499, 0.24768245630280983, 0.41509380511339444, 0.3165811158856623, 0.5463359200191661, 0.35899844774520184, 0.9057666837864972, 0.5704342751508387, 0.7995783331379702, 0.5432911849630759, 0.18565749093312822, 0.8044924081941489, 0.9087731716561545, 0.5743154818948253, 0.3728137184006586, 0.5803635706683373, 0.8329647728462389, 0.316922666455, 0.7773936606360906, 0.8194720310292638, 0.6230092913782511, 0.20969856242847928, 0.5617882232951316, 0.12134397298687949, 0.664654270447956, 0.759487192751921, 0.3952130911268532, 0.28403779016676867, 0.3506494186479022, 0.08348798406695068, 0.6683841321098696, 0.1486325629275368, 0.6461360438015606, 0.5011271805742649, 0.31843088608446246, 0.7393782329238772, 0.26959143976021793, 0.849749981445391, 0.28985991003582745, 0.06262903213517745, 0.6487493150414881, 0.9970139293496836, 0.36488144084646157, 0.3771734012346507, 0.027138922071964666, 0.7384902624761919, 0.8273164164947079, 0.9537331237853565, 0.17578608945090768, 0.4777231338861533, 0.0018457297031274633, 0.7477818623838531, 0.8196243354153068, 0.938978549438052, 0.74340651990715, 0.10089927040186164, 0.3188344060732976, 0.6012242553390986, 0.42279005699858796, 0.4210991312745954, 0.0834153119296176, 0.08037605992588626, 0.1841307923171006, 0.3557298582051003, 0.5547409972841385, 0.5802353128599511, 0.6581974282504806, 0.9719131545382388, 0.745608478287064, 0.33349719384254284, 0.02069355104488968, 0.0491618291587147, 0.27135630208268424, 0.4234596803394397, 0.44588902319224943, 0.15909720469335709, 0.3654223594317404, 0.4110511095500655, 0.6009334773734463, 0.8468643070338179, 0.02538138907364318, 0.2051456598644631, 0.6191364087000211, 0.8351656158659958, 0.14432392556203633, 0.5112465789600109, 0.01374830696714302, 0.06273240154698978, 0.9339468486287592, 0.42894259742930796, 0.7422989688999638, 0.13734277328915634, 0.8307326579984523, 0.3022267743567183, 0.8317545217299196, 0.16491860591148733, 0.5499048627638397, 0.802891982062627, 0.7688977307648327, 0.11936531224706382, 0.4816126742075234, 0.849684742095685, 0.6092174676860956, 0.3220038288643483, 0.06931141692391607, 0.938545765753185, 0.5089787544390267, 0.8392108721703297, 0.054624265437769104, 0.5387006143957361, 0.02570369252239013, 0.6030307168696115, 0.19243799945105533, 0.027610415374599295, 0.37457815145617335, 0.7944828569750545, 0.3604777360277248, 0.9772226340463483, 0.5897417913137146, 0.4660301250501728, 0.3778749368187452, 0.7755743292946893, 0.3143868770696886, 0.32839258973940144, 0.47646257974997763, 0.8837312878629973, 0.640636048075555, 0.24813090210978217, 0.9250392850142495, 0.7678346063216492, 0.9520691141509342, 0.9361094837620152, 0.020612228170447944, 0.834067375402707, 0.4851261551865935, 0.613871668716649, 0.9306486446454418, 0.6386175721747208, 0.863825287069897, 0.5862510345783434, 0.7282203395726542, 0.7364178053085315, 0.8866996475139015, 0.6194662800385703, 0.6290020956421526, 0.7491838147517992, 0.1049525100110551, 0.18304467612047493, 0.3627483660705835, 0.7960236511428941, 0.6718848098108042, 0.6992814417118154, 0.7486128834769967, 0.46545420288754735, 0.11915746072339006, 0.12310622489009715, 0.9130729925943634, 0.8306706232524054, 0.31662290942715277, 0.4649604168782543, 0.08815156619793252, 0.4742772938381672, 0.981142037903673, 0.5218619652413196, 0.007054133725641787, 0.1611214395096323, 0.6798552372375347, 0.9164754225385573, 0.42949562819760234, 0.8819104506094874, 0.5498970795549682, 0.7092142308681152, 0.7524881893005482, 0.3874468383254671, 0.5153112634084058, 0.9631722434636759, 0.6769476700199237, 0.9353300594019079, 0.9506847692692058, 0.20112145521023372, 0.4502185466241124, 0.7683293210883774, 0.40189240104100943, 0.562371778049337, 0.12051185321705149, 0.63759031497511, 0.9213339945499912, 0.4781937179657044, 0.7464994480048197, 0.7502164884047596, 0.9079530393034579, 0.839137295918075, 0.6127355105918209, 0.6708407274391404, 0.6673243375960226, 0.2493594664691261, 0.12776294192149296, 0.5815488877207242, 0.7955421514216101, 0.5708638932788334, 0.21858601698071844, 0.7690984277558858, 0.834064235514752, 0.14362561426793707, 0.0969384407682291, 0.7618905033726893, 0.16957600207692558, 0.10959552270915451, 0.5241798054347651, 0.2988081087229968, 0.33569094403302635, 0.36042330739081874, 0.3232874989733526, 0.3678335417956635, 0.5764131021847068, 0.5877610495439732, 0.5746707958690311, 0.734445534308571, 0.07245299910591885, 0.7182851257070634, 0.6106719809939707, 0.05267142910377953, 0.04707774439438406, 0.25761917972017656, 0.8419197237143154, 0.0833648683682664, 0.3105439644028418, 0.5136253241880253, 0.36680990770708044, 0.31097991256450597, 0.8097188124503302, 0.9045931136362662, 0.2405556767972552, 0.7429310932605151, 0.7051732025144789, 0.43717481151523574, 0.6309723985618344, 0.1642944657149239, 0.6461545003799715, 0.3871013306990919, 0.035951428586019074, 0.3093153606925495, 0.09715149214349039, 0.8960688614816107, 0.885513301674834, 0.7084672849453518, 0.7021064287364721, 0.5160031468870532, 0.17502433472065637, 0.41889780891620876, 0.5232795813645127, 0.6517520947499528, 0.4036967845894184, 0.8008376715793915, 0.1517961591465702, 0.28263221305706, 0.402604298425283, 0.08385650013557633, 0.6207920436516792, 0.180207146176677, 0.2990269139597217, 0.8771269371005258, 0.44519771569656397, 0.26378549360786663, 0.6505978234069467, 0.7908072069392466, 0.3812708896815461, 0.598316859125189, 0.7663917726849822, 0.1576868105425141, 0.5560342335522047, 0.09598249236164491, 0.6764707905635047, 0.11212773979210255, 0.1793131935233614, 0.20732179065838874, 0.6638656119615678, 0.4370587090015494, 0.3741423905761655, 0.8084084899164418, 0.07326909330071507, 0.5278691223184732, 0.3392193822197833, 0.7340745604571606, 0.847327095333289, 0.4874362884902925, 0.8740690352108385, 0.2579037325311143, 0.2278646168426648, 0.3339296142568764, 0.4225033541592681, 0.785571786797041, 0.5714584486085312, 0.31516722455064095, 0.16790836263239228, 0.5040585350995533, 0.520131863451707, 0.6135223248641407, 0.10062801448572856, 0.130931262301774, 0.24807721849044118, 0.1094480316335118, 0.15064903013800157, 0.30162875683667645, 0.7335970360275799, 0.9849684423228596, 0.6920372552372748, 0.11125835606834644, 0.17859342405373335, 0.5213374507427226, 0.6472860843780127, 0.29613672063547436, 0.28581685657747335, 0.3537507003674707, 0.10690189337272038, 0.9576126385959707, 0.8110913896511049, 0.12970174515977695, 0.4943134051803729, 0.06571451310885112, 0.24415762125155116, 0.3432162023305927, 0.1355374124394063, 0.6543105022793935, 0.8215071033574031, 0.5578230873418384, 0.4314519136507382, 0.9633407207927073, 0.847845840101353, 0.43128053097245833, 0.79477544158276, 0.27369826225086924, 0.6334137046872625, 0.7293715395434309, 0.6776558468571573, 0.8319212301945255, 0.06250558468073741, 0.8111311556710555, 0.8363455465552554, 0.17404506974931644, 0.025045532143128546, 0.4536836671944494, 0.5525732512580489, 0.4634976172383335, 0.07737485910575392, 0.7285511079027368, 0.29532634857379036, 0.3401375587804927, 0.4547843537159175, 0.6848017328123464, 0.23024231850901888, 0.6061587183603819, 0.2901407459493498, 0.3309358572912441, 0.17997961995796907, 0.11501580819019597, 0.005366826320221896, 0.32888033845351494, 0.898134992263572, 0.8044284599952828, 29.645111302903846, 4.082058215524006, 2.4090835370305603, 1.2045417685152802, 5.5542759325982365, 2.810597459868988, 0.8030278456768535, 0.6022708842576401, 0.5353518971179022, 0.06691898713973778, 0.06691898713973778, 0.0, 0.33459493569868903, 8.628668688393573, 13.218386075836959, 7.251753472160554, 2.0194756504750906, 2.0194756504750906, 2.111269998223959, 5.7830439081786675, 3.2128021712103725, 0.8261491297398099, 0.4589717387443388, 0.6425604342420744, 0.3671773909954711, 1.2851208684841489, 4.866333517082519, 5.7553752173187505, 24.09770924324517, 3.275416790344004, 0.7954583633692582, 0.9826250371032011, 3.9772918168462903, 2.854291774442632, 2.012041742639888, 0.3275416790344004, 0.7486666949357724, 0.2339583421674289, 0.7018750265022866, 1.044544188058431, 0.46082831826107246, 2.795691797450506, 5.3763303797125115, 0.3379407667247865, 0.2764969909566435, 0.36866265460885794, 0.3379407667247865, 1.2595974032469308, 0.3993845424929294, 1.290319291131003, 0.061443775768142986, 0.21505321518850046, 12.431321144178746, 1.8111858620657777, 1.7288592319718787, 1.8111858620657777, 13.83087385577503, 0.9879195611267877, 0.6586130407511916, 0.41163315046949484, 0.41163315046949484, 0.0, 0.3293065203755958, 0.1646532601877979, 0.41163315046949484, 9.440100555849622, 2.5100845408617496, 2.7283527618062493, 0.8185058285418747, 3.492291535111998, 6.111510186445997, 1.9098469332643744, 0.54567055236125, 0.4911034971251248, 0.16370116570837498, 0.32740233141674996, 0.43653644188899976, 0.32740233141674996, 3.849211044783694, 4.285719513779783, 5.476197156496387, 0.9920647022638385, 1.388890583169374, 1.0714298784449459, 16.309543705217507, 5.873023037401925, 1.3095254069882671,
遗传算法得到最优目标值及最优个体,一次迭代即产生子代1
最新推荐文章于 2021-12-08 16:28:01 发布
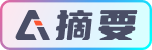