一、简单封装一下
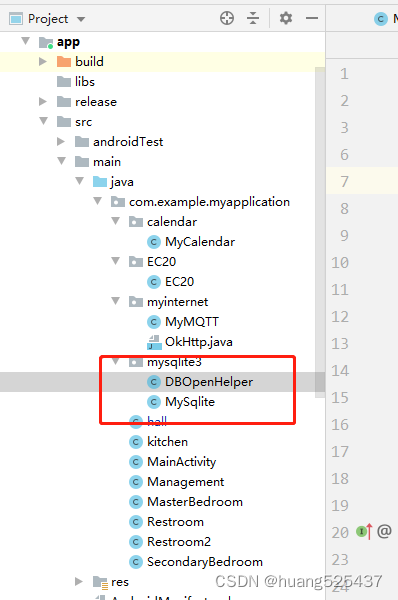
二、新建DBOpenHelper类
package com.example.myapplication.mysqlite3;
import android.content.Context;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.util.Log;
import com.example.myapplication.tool.MyConfig;
public class DBOpenHelper extends SQLiteOpenHelper {
private static final String TAG = "DBOpenHelper";
final String CREATE_TABLE_SQL1="create table "+MyConfig.table_gas8detectorwarm + "("
+"id integer primary key autoincrement,"
+"device_name text,"
+"topic_warm text,"
+"air text,"
+"temp_mim text,"
+"temp_max text,"
+"humi_min text,"
+"humi_max text,"
+"pm2_5 text,"
+"pm10 text,"
+"pm1_0 text,"
+"CO2 text,"
+"CH2O text,"
+"VOC text)"
;
final String CREATE_TABLE_SQL2="create table "+MyConfig.table_gas8detectorlog + "("
+"id integer primary key autoincrement,"
+"device_name text,"
+"topic text,"
+"logdata text,"
+"ctime text)"
;
SQLiteDatabase sqLiteDatabase;
public DBOpenHelper(Context context, String name, SQLiteDatabase.CursorFactory factory, int version) {
super(context, name, null, version);
Log.d(TAG, "DBOpenHelper: 创建数据库");
}
@Override
public void onCreate(SQLiteDatabase db) {
Log.d(TAG, "onCreate: 创建数据表1"+CREATE_TABLE_SQL1);
db.execSQL(CREATE_TABLE_SQL1);
Log.d(TAG, "onCreate: 创建数据表2"+CREATE_TABLE_SQL2);
db.execSQL(CREATE_TABLE_SQL2);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
String tring_sql1="drop table if exists "+MyConfig.table_gas8detectorwarm;
db.execSQL(tring_sql1);
String string_sql2="drop table if exists "+MyConfig.table_gas8detectorlog;
db.execSQL(string_sql2);
onCreate(db);
Log.d(TAG, "onUpgrade: 数据库版本更新到 V"+newVersion);
}
}
三、新建MySqlite类
package com.example.myapplication.mysqlite3;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.util.Log;
import com.example.myapplication.tool.MyApplication;
import com.example.myapplication.tool.MyConfig;
public class MySqlite {
final static String TAG="MySqlite";
public static MySqlite MySqlite1;
static DBOpenHelper dbOpenHelper;
private MySqlite(){ }
public static MySqlite getInstance(){
if (MySqlite1 == null) {
MySqlite1 = new MySqlite();
MySqliteCreate(MyApplication.getContext(), MyConfig.MySQLiteOpenHelper_DB_Name,null,MyConfig.MySQLiteOpenHelper_DB_version);
}
return MySqlite1;
}
public static void MySqliteCreate(Context context, String name, SQLiteDatabase.CursorFactory factory, int version) {
dbOpenHelper = new DBOpenHelper(context, name, null, version);
}
public void gas8detectorwarm_insert(String device_name,String topic_warm,String air,String temp_mim,String temp_max,
String humi_min,String humi_max,String pm2_5,String pm10,
String pm1_0,String CO2,String CH2O,String VOC){
ContentValues values = new ContentValues();
values.put("device_name",device_name);
values.put("topic_warm",topic_warm);
values.put("air",air);
values.put("temp_mim",temp_mim);
values.put("temp_max",temp_max);
values.put("humi_min",humi_min);
values.put("humi_max",humi_max);
values.put("pm2_5",pm2_5);
values.put("pm10",pm10);
values.put("pm1_0",pm1_0);
values.put("CO2",CO2);
values.put("CH2O",CH2O);
values.put("VOC",VOC);
dbOpenHelper.getWritableDatabase().insert(MyConfig.table_gas8detectorwarm,null,values);
}
public void gas8detectorwarm_delete(String device_name){
dbOpenHelper.getWritableDatabase().delete(MyConfig.table_gas8detectorwarm,"device_name == ?",new String[]{device_name});
}
public int gas8detectorwarm_query(String device_name){
int i=0;
Cursor cursor= dbOpenHelper.getWritableDatabase().query(MyConfig.table_gas8detectorwarm,null,"device_name = ?",new String[]{device_name},null,null,null);
if (cursor.moveToFirst()){
i= 1;
do{
int temp_id=cursor.getInt(cursor.getColumnIndex("id"));
Log.d(TAG, "gas8detectorwarm_query: temp_id="+temp_id);
String temp_device_name = cursor.getString(cursor.getColumnIndex("device_name"));
Log.d(TAG, "gas8detectorwarm_query: temp_device_name="+temp_device_name);
String temp_topic_warm = cursor.getString(cursor.getColumnIndex("topic_warm"));
Log.d(TAG, "gas8detectorwarm_query: temp_topic_warm="+temp_topic_warm);
String temp_air = cursor.getString(cursor.getColumnIndex("air"));
Log.d(TAG, "gas8detectorwarm_query: temp_air="+temp_air);
String temp_temp_mim = cursor.getString(cursor.getColumnIndex("temp_mim"));
Log.d(TAG, "gas8detectorwarm_query: temp_temp_mim="+temp_temp_mim);
String temp_temp_max = cursor.getString(cursor.getColumnIndex("temp_max"));
Log.d(TAG, "gas8detectorwarm_query: temp_temp_max="+temp_temp_max);
String temp_humi_min = cursor.getString(cursor.getColumnIndex("humi_min"));
Log.d(TAG, "gas8detectorwarm_query: temp_humi_min="+temp_humi_min);
String temp_humi_max = cursor.getString(cursor.getColumnIndex("humi_max"));
Log.d(TAG, "gas8detectorwarm_query: temp_humi_max="+temp_humi_max);
String temp_pm2_5 = cursor.getString(cursor.getColumnIndex("pm2_5"));
Log.d(TAG, "gas8detectorwarm_query: temp_pm2_5="+temp_pm2_5);
String temp_pm10 = cursor.getString(cursor.getColumnIndex("pm10"));
Log.d(TAG, "gas8detectorwarm_query: temp_pm10="+temp_pm10);
String temp_pm1_0 = cursor.getString(cursor.getColumnIndex("pm1_0"));
Log.d(TAG, "gas8detectorwarm_query: temp_pm1_0="+temp_pm1_0);
String temp_CO2 = cursor.getString(cursor.getColumnIndex("CO2"));
Log.d(TAG, "gas8detectorwarm_query: temp_CO2="+temp_CO2);
String temp_CH2O = cursor.getString(cursor.getColumnIndex("CH2O"));
Log.d(TAG, "gas8detectorwarm_query: temp_CH2O="+temp_CH2O);
String temp_VOC = cursor.getString(cursor.getColumnIndex("VOC"));
Log.d(TAG, "gas8detectorwarm_query: temp_VOC="+temp_VOC);
}while(cursor.moveToNext());
}else{
i=0;
}
return i;
}
}