12届
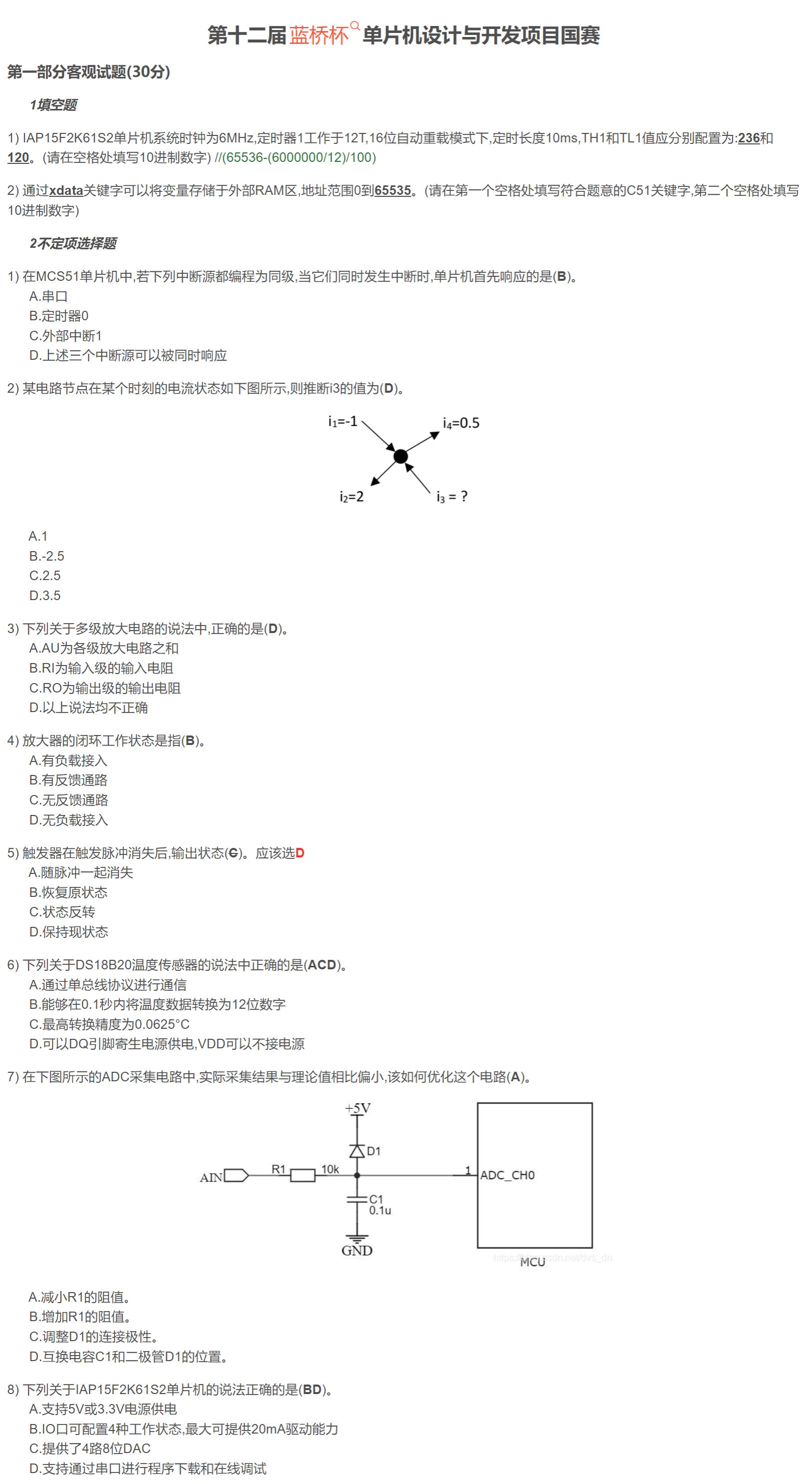

代码
main.c
#include"reg52.h"
#include"intrins.h"
#include"ds1302.h"
#include"iic.h"
sbit TX=P1^0;
sbit RX=P1^1;
sfr AUXR=0x8e;
sfr P4=0xC0;
sbit R1=P3^0;
sbit R2=P3^1;
sbit R3=P3^2;
sbit R4=P3^3;
sbit C4=P3^4;
sbit C3=P3^5;
sbit C2=P4^2;
sbit C1=P4^4;
char code write_add[3]={0x80,0x82,0x84};
char code read_add[3]={0x81,0x83,0x85};
char time[3]={0x01,0x20,0x20};
int last_distance;
int all_mode;
int smg_mode;
int set_mode;
int set_time=2;
int set_distance=20;
int distance_mode=0;
int num_mode=0;
int rb1;
int led=0xff;
int distance_work=0;
int alarm_work=0;
int max;int min;int avg;
char rom_distance[50];
int distance_num=0;
char code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xc7,0x8e,0xc6,0x89,0xfe,0xbf,0xf7,0x8c,0x7f};
void find_max();
void find_min();
void find_avg();
void smg_display();
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//=========================================时间
void write_time()
{
int i;
Write_Ds1302_Byte(0x8e,0x00);
for(i=0;i<3;i++)
{
Write_Ds1302_Byte(write_add[i],time[i]);
}
Write_Ds1302_Byte(0x8e,0x80);
}
void read_time()
{
int i;
for(i=0;i<3;i++)
{
time[i]=Read_Ds1302_Byte(read_add[i]);
}
}
//=========================================
//=========================================距离
void Delay12us() //@11.0592MHz
{
unsigned char i;
_nop_();
_nop_();
_nop_();
i = 30;
while (--i);
}
void send_wave()
{
int i;
for(i=0;i<8;i++)
{
TX=1;
Delay12us();
TX=0;
Delay12us();
}
}
void get_distance()
{
int time;
AUXR |= 0x40; //定时器时钟1T模式
TMOD &= 0x0F; //设置定时器模式
TL1 = 0xAE; //设置定时初始值
TH1 = 0xFB; //设置定时初始值
TF1 = 0; //清除TF1标志
TR1 = 0; //定时器1开始计时
TL1 = 0x00; //设置定时初始值
TH1 = 0x00; //设置定时初始值
send_wave();
TR1=1;
while(RX==1&&TF1==0);
TR1=0;
if(TF1==0)
{
time=TH1;
time=(time<<8)|TL1;
last_distance=time*0.017;
last_distance=last_distance/12;
}
else
{
last_distance=999;
TF1=0;
}
TL1 = 0x00; //设置定时初始值
TH1 = 0x00; //设置定时初始值
}
//=========================================
//=========================================按键
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
R1=R2=R4=1;R3=0;
C1=C2=C3=C4=1;
//S5
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(all_mode==0)
{
smg_mode++;
if(smg_mode==2)
{
num_mode=0;
find_max();
find_min();
find_avg();
}
if(smg_mode>=3)
{
smg_mode=0;
}
}
if(all_mode==1)
{
set_mode++;
if(set_mode>=2)
{
set_mode=0;
}
}
}
while(!C1);
{
smg_display();
}
}
//S9
if(C2==0&&all_mode==1)
{
Delay10ms();
if(C2==0&&all_mode==1)
{
if(set_mode==0)
{
set_time++;
if(set_time>9)
{
set_time=2;
}
}
if(set_mode==1)
{
set_distance=set_distance+10;
if(set_distance>80)
{
set_distance=10;
}
}
}
while(!C2)
{
smg_display();
}
}
R1=R2=R3=1;R4=0;
C1=C2=C3=C4=1;
//S4
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(all_mode==0)
{
all_mode=1;
set_mode=0;
}
else
{
all_mode=0;
smg_mode=0;
}
}
while(!C1)
{
smg_display();
}
}
//S8
if(C2==0&&all_mode==0)
{
Delay10ms();
if(C2==0&&all_mode==0)
{
if(smg_mode==1)
{
if(distance_mode==0)
{
distance_mode=1;
}
else
{
distance_mode=0;
}
}
if(smg_mode==2)
{
num_mode++;
if(num_mode>=3)
{
num_mode=0;
}
}
}
while(!C2)
{
smg_display();
}
}
}
//=========================================
//=========================================光敏and距离获得
void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count_1;
void timer0_server() interrupt 1
{
if(distance_mode==1)
{
count_1++;
if(count_1>=(set_time*200))
{
distance_work=1;
count_1=0;
}
}
else
{
count_1=0;
}
}
void get_rb1()
{
rb1=re_rb1();
}
int temp_mode_1=0;
void judge_distance()
{
if(distance_mode==0)//触发模式
{
if(rb1<=100&&temp_mode_1==0)
{
temp_mode_1=1;
get_distance();
rom_distance[distance_num]=last_distance;
distance_num++;
}
if(rb1>100)
{
temp_mode_1=0;
}
}
if(distance_mode==1)//定时模式
{
if(distance_work==1)
{
get_distance();
distance_work=0;
rom_distance[distance_num]=last_distance;
distance_num++;
if(distance_num>2)
{
if(rom_distance[distance_num-1]<(set_distance+5)&&
rom_distance[distance_num-1]>(set_distance-5)&&
rom_distance[distance_num-2]<(set_distance+5)&&
rom_distance[distance_num-2]>(set_distance-5)&&
rom_distance[distance_num-3]<(set_distance+5)&&
rom_distance[distance_num-3]>(set_distance-5))
{
alarm_work=1;
}
else
{
alarm_work=0;
}
}
}
}
}
//=========================================
//=========================================计算平均值,寻找最大最小值
void find_max()
{
int i=0;
for(i=1;i<distance_num;i++)
{
if(rom_distance[i-1]>=rom_distance[i])
{
max=rom_distance[i-1];
}
else
{
max=rom_distance[i];
}
}
}
void find_min()
{
int i=0;
for(i=1;i<distance_num;i++)
{
if(rom_distance[i-1]>=rom_distance[i])
{
min=rom_distance[i];
}
else
{
min=rom_distance[i-1];
}
}
}
void find_avg()
{
int i;int sum;
for(i=0;i<distance_num;i++)
{
sum=sum+rom_distance[i]*10;
}
avg=sum/distance_num;
}
//=========================================
//=========================================数码管
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
}
void smg_display()
{
if(all_mode==0)
{
if(smg_mode==0)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
SMG(3,16);
SMG(4,time[1]%16);
SMG(5,time[1]/16);
SMG(6,16);
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
if(smg_mode==1)
{
SMG(1,last_distance%10);
if(last_distance>=10)
{
SMG(2,(last_distance%100)/10);
}
else
{
SMG(2,10);
}
if(last_distance>=100)
{
SMG(3,(last_distance%1000)/100);
}
else
{
SMG(3,10);
}
SMG(4,10);
SMG(5,10);
// SMG(5,rb1/100);//测试
SMG(6,10);
if(distance_mode==0)
{
SMG(7,13);
}
else
{
SMG(7,12);
}
SMG(8,11);
}
if(smg_mode==2)
{
if(num_mode==0)
{
SMG(1,max%10);
SMG(2,max/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,15);
SMG(8,14);
}
if(num_mode==1)
{
SMG(1,min%10);
SMG(2,min/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,16);
SMG(8,14);
}
if(num_mode==2)
{
SMG(1,avg%10);
SMG(2,19);
SMG(2,(avg%100)/10);
SMG(3,(avg%1000)/100);
SMG(4,10);
SMG(5,10);
SMG(6,10);
// SMG(6,distance_num);//测试
SMG(7,17);
SMG(8,14);
}
}
}
if(all_mode==1)
{
if(set_mode==0)
{
SMG(1,set_time%10);
SMG(2,set_time/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,(set_mode+1));
SMG(8,18);
}
if(set_mode==1)
{
SMG(1,set_distance%10);
SMG(2,set_distance/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,(set_mode+1));
SMG(8,18);
}
}
}
//=========================================
//=========================================led
void led_work()
{
if(all_mode==0)
{
//L1
if(smg_mode==0)
{
led=(led&0xfe)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfe)|0x01;
choose_573(4);
P0=led;
choose_573(0);
}
//L2
if(smg_mode==1)
{
led=(led&0xfd)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfd)|0x02;
choose_573(4);
P0=led;
choose_573(0);
}
//L3
if(smg_mode==2)
{
led=(led&0xfb)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfb)|0x04;
choose_573(4);
P0=led;
choose_573(0);
}
//L4
if(smg_mode==1&&distance_mode==0)
{
led=(led&0xf7)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xf7)|0x08;
choose_573(4);
P0=led;
choose_573(0);
}
//L5
if(alarm_work==1)
{
led=(led&0xef)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xef)|0x10;
choose_573(4);
P0=led;
choose_573(0);
}
if(rb1>=100)
{
led=(led&0xdf)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xdf)|0x20;
choose_573(4);
P0=led;
choose_573(0);
}
}
}
//=========================================
//=========================================
void DA_out()
{
if(last_distance<=10)
{
DA(51);
}
if(last_distance>=80)
{
DA(255);
}
if(last_distance>10&&last_distance<80)
{
DA(((0.057*last_distance)+1)*51);
}
}
//=========================================
void main()
{
init_system();
write_time();
Timer0Init();
while(1)
{
read_time();
key_board();
smg_display();
// get_distance();//测试
get_rb1();
judge_distance();
led_work();
DA_out();
}
}
iic.c
/*
程序说明: IIC总线驱动程序
软件环境: Keil uVision 4.10
硬件环境: CT107单片机综合实训平台 8051,12MHz
日 期: 2011-8-9
*/
#include "reg52.h"
#include "intrins.h"
#define DELAY_TIME 5
#define SlaveAddrW 0xA0
#define SlaveAddrR 0xA1
//总线引脚定义
sbit SDA = P2^1; /* 数据线 */
sbit SCL = P2^0; /* 时钟线 */
void IIC_Delay(unsigned char i)
{
do{_nop_();}
while(i--);
}
//总线启动条件
void IIC_Start(void)
{
SDA = 1;
SCL = 1;
IIC_Delay(DELAY_TIME);
SDA = 0;
IIC_Delay(DELAY_TIME);
SCL = 0;
}
//总线停止条件
void IIC_Stop(void)
{
SDA = 0;
SCL = 1;
IIC_Delay(DELAY_TIME);
SDA = 1;
IIC_Delay(DELAY_TIME);
}
//发送应答
void IIC_SendAck(bit ackbit)
{
SCL = 0;
SDA = ackbit; // 0:应答,1:非应答
IIC_Delay(DELAY_TIME);
SCL = 1;
IIC_Delay(DELAY_TIME);
SCL = 0;
SDA = 1;
IIC_Delay(DELAY_TIME);
}
//等待应答
bit IIC_WaitAck(void)
{
bit ackbit;
SCL = 1;
IIC_Delay(DELAY_TIME);
ackbit = SDA;
SCL = 0;
IIC_Delay(DELAY_TIME);
return ackbit;
}
//通过I2C总线发送数据
void IIC_SendByte(unsigned char byt)
{
unsigned char i;
for(i=0; i<8; i++)
{
SCL = 0;
IIC_Delay(DELAY_TIME);
if(byt & 0x80) SDA = 1;
else SDA = 0;
IIC_Delay(DELAY_TIME);
SCL = 1;
byt <<= 1;
IIC_Delay(DELAY_TIME);
}
SCL = 0;
}
//从I2C总线上接收数据
unsigned char IIC_RecByte(void)
{
unsigned char i, da;
for(i=0; i<8; i++)
{
SCL = 1;
IIC_Delay(DELAY_TIME);
da <<= 1;
if(SDA) da |= 1;
SCL = 0;
IIC_Delay(DELAY_TIME);
}
return da;
}
int re_rb1()
{
int temp;
IIC_Start();
IIC_SendByte(0x90);
IIC_WaitAck();
IIC_SendByte(0x01);
IIC_WaitAck();
IIC_Stop();
IIC_Start();
IIC_SendByte(0x91);
IIC_WaitAck();
temp=IIC_RecByte();
IIC_SendAck(1);
IIC_Stop();
return temp;
}
void DA(int dat)
{
IIC_Start();
IIC_SendByte(0x90);
IIC_WaitAck();
IIC_SendByte(0x40);
IIC_WaitAck();
IIC_SendByte(dat);
IIC_WaitAck();
IIC_Stop();
}
iic.h
#ifndef _IIC_H
#define _IIC_H
void IIC_Start(void);
void IIC_Stop(void);
bit IIC_WaitAck(void);
void IIC_SendAck(bit ackbit);
void IIC_SendByte(unsigned char byt);
unsigned char IIC_RecByte(void);
int re_rb1();
void DA(int dat);
#endif
ds1302
/*
程序说明: DS1302驱动程序
软件环境: Keil uVision 4.10
硬件环境: CT107单片机综合实训平台 8051,12MHz
日 期: 2011-8-9
*/
#include <reg52.h>
#include <intrins.h>
sbit SCK=P1^7;
sbit SDA=P2^3;
sbit RST = P1^3; // DS1302复位
void Write_Ds1302(unsigned char temp)
{
unsigned char i;
for (i=0;i<8;i++)
{
SCK=0;
SDA=temp&0x01;
temp>>=1;
SCK=1;
}
}
void Write_Ds1302_Byte( unsigned char address,unsigned char dat )
{
RST=0; _nop_();
SCK=0; _nop_();
RST=1; _nop_();
Write_Ds1302(address);
Write_Ds1302(dat);
RST=0;
}
unsigned char Read_Ds1302_Byte ( unsigned char address )
{
unsigned char i,temp=0x00;
RST=0; _nop_();
SCK=0; _nop_();
RST=1; _nop_();
Write_Ds1302(address);
for (i=0;i<8;i++)
{
SCK=0;
temp>>=1;
if(SDA)
temp|=0x80;
SCK=1;
}
RST=0; _nop_();
SCK=0; _nop_();
SCK=1; _nop_();
SDA=0; _nop_();
SDA=1; _nop_();
return (temp);
}
1302.h
#ifndef __DS1302_H
#define __DS1302_H
void Write_Ds1302(unsigned char temp);
void Write_Ds1302_Byte( unsigned char address,unsigned char dat );
unsigned char Read_Ds1302_Byte( unsigned char address );
#endif
11届
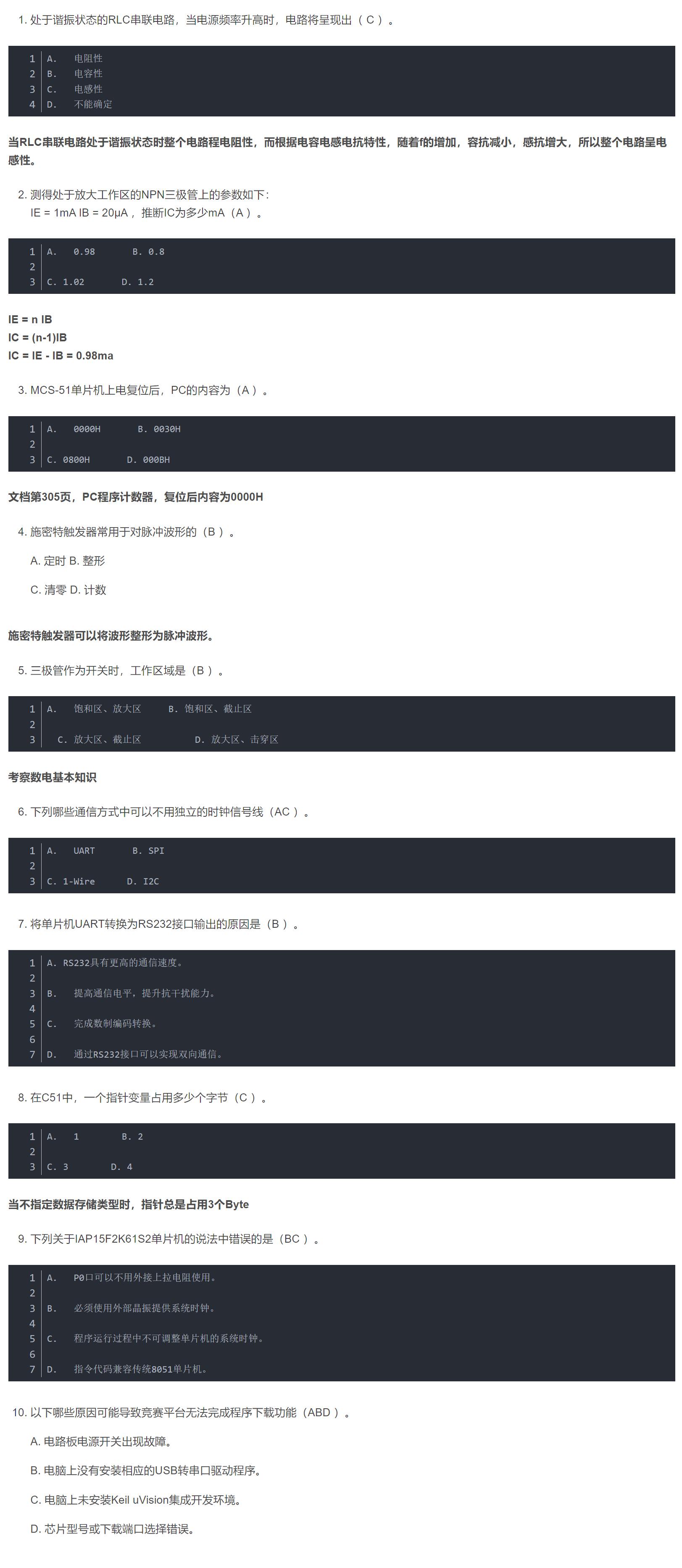

main.c
#include"reg52.h"
#include"intrins.h"
#include"ds1302.h"
#include"iic.h"
#include"onewire.h"
char code write_add[]={0x80,0x82,0x84};
char code read_add[]={0x81,0x83,0x85};
char time[]={0x50,0x59,0x16};
int Rb1;
int temperature;
int all_mode=0;
int smg_mode=0;
int set_mode=0;
int set_hour=17;
int set_temperature=25;
int set_led=4;
int led=0xff;
int L3_mode=0;
sfr AUXR=0x8e;
sfr P4=0xc0;
sbit R1=P3^0;
sbit R2=P3^1;
sbit R3=P3^2;
sbit R4=P3^3;
sbit C4=P3^4;
sbit C3=P3^5;
sbit C2=P4^2;
sbit C1=P4^4;
void smg_display();
char code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xbf,0xc6,0x86,0x8c,0x7f};
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//==================================时间
void write_time()
{
int i;
Write_Ds1302_Byte(0x8e,0x00);
for(i=0;i<3;i++)
{
Write_Ds1302_Byte(write_add[i],time[i]);
}
Write_Ds1302_Byte(0x8e,0x80);
}
void read_time()
{
int i;
for(i=0;i<3;i++)
{
time[i]=Read_Ds1302_Byte(read_add[i]);
}
}
//==================================
//==================================光敏and温度
void get_guang()
{
Rb1=guang();
}
void get_temperature()
{
temperature=rd_temperature();
}
//==================================
//==================================按键
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
R3=0;R2=R1=R4=1;
C1=C2=C3=C4=1;
//S5
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(all_mode==0)
{
smg_mode++;
if(smg_mode>=3)
{
smg_mode=0;
}
}
if(all_mode==1)
{
set_mode++;
if(set_mode>=3)
{
set_mode=0;
}
}
}
while(!C1)
{
smg_display();
}
}
//S9
if(C2==0&&all_mode==1)
{
Delay10ms();
if(C2==0&&all_mode==1)
{
if(set_mode==0)
{
set_hour++;
if(set_hour>23)
{
set_hour=23;
}
}
if(set_mode==1)
{
set_temperature++;
if(set_temperature>99)
{
set_temperature=99;
}
}
if(set_mode==2)
{
set_led++;
if(set_led>8)
{
set_led=8;
}
}
}
while(!C2)
{
smg_display();
}
}
R4=0;R2=R1=R3=1;
C1=C2=C3=C4=1;
//S4
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(all_mode==0)
{
all_mode=1;
set_mode=0;
}
else
{
all_mode=0;
smg_mode=0;
}
}
while(!C1)
{
smg_display();
}
}
//S8
if(C2==0&&all_mode==1)
{
Delay10ms();
if(C2==0&&all_mode==1)
{
if(set_mode==0)
{
set_hour--;
if(set_hour<0)
{
set_hour=0;
}
}
if(set_mode==1)
{
set_temperature--;
if(set_temperature<0)
{
set_temperature=0;
}
}
if(set_mode==2)
{
set_led--;
if(set_led<4)
{
set_led=4;
}
}
}
while(!C2)
{
smg_display();
}
}
}
//==================================
//==================================数码管
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
}
void smg_display()
{
if(all_mode==0)
{
if(smg_mode==0)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
SMG(3,11);
SMG(4,time[1]%16);
SMG(5,time[1]/16);
SMG(6,11);
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
if(smg_mode==1)
{
SMG(1,temperature%10);
SMG(2,(temperature%100)/10);
SMG(3,(temperature%1000)/100);
SMG(2,15);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,12);
}
if(smg_mode==2)
{
if(Rb1<100)
{
SMG(1,1);
}
else
{
SMG(1,0);
}
SMG(2,10);
SMG(3,10);
SMG(4,Rb1%10);
SMG(5,(Rb1%100)/10);
SMG(6,(Rb1%1000)/100);
SMG(6,15);
SMG(7,10);
SMG(8,13);
}
}
if(all_mode==1)
{
if(set_mode==0)
{
SMG(1,set_hour%10);
SMG(2,set_hour/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,1);
SMG(8,14);
}
if(set_mode==1)
{
SMG(1,set_temperature%10);
SMG(2,set_temperature/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,2);
SMG(8,14);
}
if(set_mode==2)
{
SMG(1,set_led);
SMG(2,10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,3);
SMG(8,14);
}
}
}
//==================================
//==================================中断函数
void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count_1;int count_2;int count_3;
void time0_service() interrupt 1
{
count_1++;
count_2++;
if(count_1>150)
{
count_1=0;
get_temperature();
}
if(count_2>80)
{
count_2=0;
get_guang();
}
if(Rb1<100)
{
count_3++;
if(count_3>=600)
{
L3_mode=1;
}
}
else
{
count_3=0;
L3_mode=0;
}
}
//==================================
//==================================led
void led_work()
{
//L1
if(set_hour>time[2]%16+(time[2]/16)*10)
{
led=(led&0xfe)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfe)|0x01;
choose_573(4);
P0=led;
choose_573(0);
}
//L2
if(temperature<set_temperature*10)
{
led=(led&0xfd)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfd)|0x02;
choose_573(4);
P0=led;
choose_573(0);
}
//L3
if(L3_mode==1)
{
led=(led&0xfb)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfb)|0x04;
choose_573(4);
P0=led;
choose_573(0);
}
//L4~L8
if(Rb1<100)
{
if(set_led==4)
{
led=(led&0xf7)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xf7)|0x08;
choose_573(4);
P0=led;
choose_573(0);
}
if(set_led==5)
{
led=(led&0xef)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xef)|0x10;
choose_573(4);
P0=led;
choose_573(0);
}if(set_led==6)
{
led=(led&0xdf)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xdf)|0x20;
choose_573(4);
P0=led;
choose_573(0);
}
if(set_led==7)
{
led=(led&0xbf)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xbf)|0x40;
choose_573(4);
P0=led;
choose_573(0);
}
if(set_led==8)
{
led=(led&0x7f)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0x7f)|0x80;
choose_573(4);
P0=led;
choose_573(0);
}
}
else
{
led=(led&0x07)|0xf8;
choose_573(4);
P0=led;
choose_573(0);
}
}
//==================================
void main()
{
init_system();
write_time();
Timer0Init();
while(1)
{
read_time();
key_board();
smg_display();
led_work();
}
}
10届
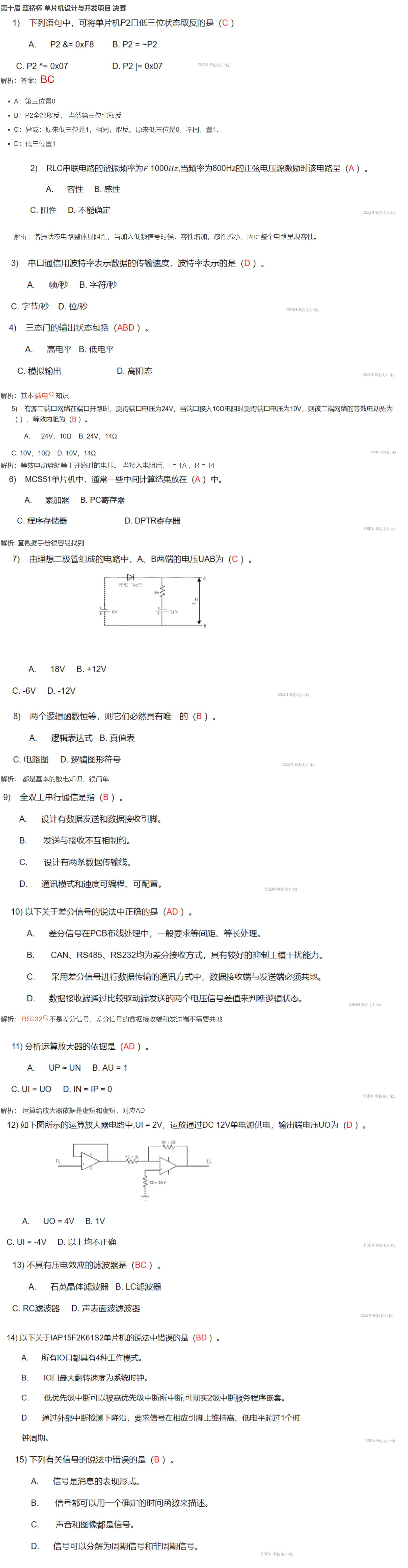

#include "reg52.h"
#include"intrins.h"
#include"iic.h"
#include"onewire.h"
#include"string.h"
sbit TX=P1^0;
sbit RX=P1^1;
sfr AUXR=0x8e;
sfr P4=0xC0;
sbit R1=P3^0;
sbit R2=P3^1;
sbit R3=P3^2;
sbit R4=P3^3;
sbit C4=P3^4;
sbit C3=P3^5;
sbit C2=P4^2;
sbit C1=P4^4;
int judge_temp=0;
int k12=0;int k13=0;
char uart_dat[10];
unsigned int change_time=0;
int distance;int set_distance=35;
int temperature;int set_temperature=30;
int all_mode=0;
int smg_mode=0;
int set_mode=0;
int da_mode=1;
int led=0xff;
char code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xc6,0xc7,0xc8,0x8c,0x7f};
void smg_display();
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//========================距离
void Delay12us() //@11.0592MHz
{
unsigned char i;
_nop_();
_nop_();
_nop_();
i = 30;
while (--i);
}
void send_wave()
{
int i=0;
for(i=0;i<8;i++)
{
TX=0;
Delay12us();
TX=1;
Delay12us();
}
}
void get_distance()
{
unsigned int time;
EA=1;
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 0; //定时器0开始计时
TL0 = 0x00; //设置定时初始值
TH0 = 0x00; //设置定时初始值
send_wave();
TR0=1;
while(RX==1&&TF0==0);
TR0=1;
if(TF0==0)
{
time=(TH0<<8)|TL0;
distance=time*0.017;
distance=distance/12;
if(distance>=99)
{
distance=99;
}
}
else
{
distance=99;
TF0=0;
}
TH0=TL0=0x00;
}
//========================
//========================温度
void get_temperature()
{
temperature=rd_temperature();
}
//========================
//========================按键
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
R1=R2=R4=1;R3=0;
C1=C2=C3=C4=1;
//S13
if(C3==0)
{
k13=1;
Delay10ms();
if(C3==0)
{
if(all_mode==0)
{
all_mode=1;
set_mode=0;
}
else
{
all_mode=0;
smg_mode=0;
}
}
while(!C3)
{
smg_display();
}
}
else
{
k13=0;
}
//S17
if(C4==0)
{
Delay10ms();
if(C4==0)
{
if(all_mode==1)
{
if(set_mode==0)
{
set_temperature=set_temperature+2;
if(set_temperature>98)
{
set_temperature=98;
}
change_time++;
write_EEPROM(0x01,change_time);
Delay10ms();
write_EEPROM(0x02,change_time>>8);
}
if(set_mode==1)
{
set_distance=set_distance+5;
if(set_distance>99)
{
set_distance=95;
}
change_time++;
write_EEPROM(0x01,change_time);
Delay10ms();
write_EEPROM(0x02,change_time>>8);
}
}
}
while(!C4)
{
smg_display();
}
}
R1=R2=R3=1;R4=0;
C1=C2=C3=C4=1;
//S12
if(C3==0)
{
k12=1;
Delay10ms();
if(C3==0)
{
if(all_mode==0)
{
smg_mode++;
if(smg_mode>=3)
{
smg_mode=0;
write_EEPROM(0x03,set_temperature);
Delay10ms();
write_EEPROM(0x04,set_distance);
}
}
if(all_mode==1)
{
set_mode++;
if(set_mode>=2)
{
set_mode=0;
}
}
}
while(!C3)
{
smg_display();
}
}
else
{
k12=0;
}
//S16
if(C4==0)
{
Delay10ms();
if(C4==0)
{
if(all_mode==1)
{
if(set_mode==0)
{
set_temperature=set_temperature-2;
if(set_temperature<0)
{
set_temperature=0;
}
change_time++;
write_EEPROM(0x01,change_time);
Delay10ms();
write_EEPROM(0x02,change_time>>8);
}
if(set_mode==1)
{
set_distance=set_distance-5;
if(set_distance<0)
{
set_distance=0;
}
change_time++;
write_EEPROM(0x01,change_time);
Delay10ms();
write_EEPROM(0x02,change_time>>8);
}
}
}
while(!C4)
{
smg_display();
}
}
}
//========================
//========================read_EEPROM
void readeeprom()
{
int change_time_h;int change_time_l;
set_temperature=read_EEPROM(0x03);
Delay10ms();
set_distance=read_EEPROM(0x04);
Delay10ms();
change_time_h=read_EEPROM(0x02);
Delay10ms();
change_time_l=read_EEPROM(0x01);
change_time=change_time_h*256+change_time_l;
}
//========================
//========================数码管
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
Delay400us();
choose_573(7);
P0=xianshi[10];
choose_573(0);
}
void smg_display()
{
if(all_mode==0)
{
if(smg_mode==0)
{
SMG(1,temperature%10);
SMG(2,(temperature%100)/10);
SMG(3,(temperature%1000)/100);
SMG(3,15);
if(temperature>=1000)
{
SMG(4,(temperature%10000)/1000);
}
else
{
SMG(4,10);
}
SMG(5,10);
// SMG(6,judge_temp);
SMG(6,10);
SMG(7,10);
SMG(8,11);
}
if(smg_mode==1)
{
SMG(1,distance%10);
SMG(2,(distance%100)/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,12);
}
if(smg_mode==2)
{
SMG(1,change_time%10);
if(change_time>=10)
{
SMG(2,(change_time%100)/10);
}
else
{
SMG(2,10);
}
if(change_time>=100)
{
SMG(3,(change_time%1000)/100);
}
else
{
SMG(3,10);
}
if(change_time>=1000)
{
SMG(4,(change_time%10000)/1000);
}
else
{
SMG(4,10);
}
if(change_time>=10000)
{
SMG(5,(change_time%100000)/10000);
}
else
{
SMG(5,10);
}
SMG(6,10);
SMG(7,10);
SMG(8,13);
}
}
if(all_mode==1)
{
if(set_mode==0)
{
SMG(1,set_temperature%10);
SMG(2,(set_temperature%100)/10);
SMG(3,10);
SMG(4,10);
SMG(5,1);
SMG(6,10);
SMG(7,10);
SMG(8,14);
}
if(set_mode==1)
{
SMG(1,set_distance%10);
SMG(2,(set_distance%100)/10);
SMG(3,10);
SMG(4,10);
SMG(5,2);
SMG(6,10);
SMG(7,10);
SMG(8,14);
}
}
}
//========================
//========================中断
void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count_1;int count_2;
void long_key_board() interrupt 1
{
// R1=R2=R3=1;R4=0;
// C1=C2=C3=C4=1;
if(k12==1)
{
count_1++;
if(count_1>=200)
{
change_time=0;
}
}
else
{
count_1=0;
}
if(k13==1)
{
count_2++;
if(count_2>=200)
{
if(da_mode==1)
{
da_mode=0;
}
else
{
da_mode=1;
}
}
}
else
{
count_2=0;
}
}
//========================
//========================da
void da_work()
{
if(da_mode==1)
{
if(distance<=set_distance)
{
DA(102);
}
else
{
DA(204);
}
}
if(da_mode==0)
{
DA(20);
}
}
//========================
//========================LED
void led_work()
{
if(all_mode==0)
{
//L1
if(smg_mode==0)
{
led=(led&0xfe)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfe)|0x01;
choose_573(4);
P0=led;
choose_573(0);
}
//L2
if(smg_mode==1)
{
led=(led&0xfd)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfd)|0x02;
choose_573(4);
P0=led;
choose_573(0);
}
}
//L3
if(da_mode==1)
{
led=(led&0xfb)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfb)|0x04;
choose_573(4);
P0=led;
choose_573(0);
}
}
//========================
//========================串口设置 4800
void UartInit(void) //4800bps@11.0592MHz
{
SCON = 0x50; //8位数据,可变波特率
AUXR |= 0x40; //定时器时钟1T模式
AUXR &= 0xFE; //串口1选择定时器1为波特率发生器
TMOD &= 0x0F; //设置定时器模式
TL1 = 0xC0; //设置定时初始值
TH1 = 0xFD; //设置定时初始值
ET1 = 0; //禁止定时器%d中断
TR1 = 1; //定时器1开始计时
ES=1;
EA=1;
}
int uart_temp;
void uart_service() interrupt 4
{
if(RI==1)
{
uart_dat[uart_temp]=SBUF;
uart_temp++;
RI=0;
}
}
void send_dat(unsigned char dat)
{
SBUF=dat;
while(TI==0);
TI=0;
}
void send_string(unsigned char *str)
{
while(*str!='\0')
{
send_dat(*str++);
}
}
void send_1()
{
send_dat('$');
send_dat((distance/10)+0x30);
send_dat((distance%10)+0x30);
send_dat(',');
send_dat((temperature/1000)+0x30);
send_dat(((temperature%1000)/100)+0x30);
send_dat('.');
send_dat(((temperature%100)/10)+0x30);
send_dat((temperature%10)+0x30);
send_string("\r\n");
}
void send_2()
{
send_dat('#');
send_dat((set_distance/10)+0x30);
send_dat((set_distance%10)+0x30);
send_dat(',');
send_dat((set_temperature/10)+0x30);
send_dat((set_temperature%10)+0x30);
send_string("\r\n");
}
//========================
//========================串口比较
void uart_judge()
{
// if(uart_temp>=1)
// {
// if(uart_dat[0]=="S")
// {
// judge_temp=1;
// }
// if(uart_dat[0]=="P")
// {
// judge_temp=2;
// }
// }
// if(judge_temp==1&&uart_temp==4)
// {
// if(strcmp(uart_dat,"ST\r\n")==0)
// {
// send_1();
// uart_temp=0;
// judge_temp=0;
// }
// else
// {
// uart_temp=0;
// judge_temp=0;
// }
// }
// if(judge_temp==2&&uart_temp==6)
// {
// if(strcmp(uart_dat,"PARA\r\n")==0)
// {
// send_2();
// uart_temp=0;
// judge_temp=0;
// }
// else
// {
// uart_temp=0;
// judge_temp=0;
// }
// }
if(uart_temp==4&&strcmp(uart_dat,"ST\r\n")==0)
{
send_1();
uart_temp=0;
judge_temp=0;
}
if(uart_temp==6&&strcmp(uart_dat,"PARA\r\n")==0)
{
send_2();
uart_temp=0;
judge_temp=0;
}
}
//========================
void main()
{
init_system();
Timer0Init();
readeeprom();
UartInit();
while(1)
{
get_distance();
get_temperature();
smg_display();
key_board();
da_work();
led_work();
// send_1();
// send_2();
uart_judge();
}
}
9届
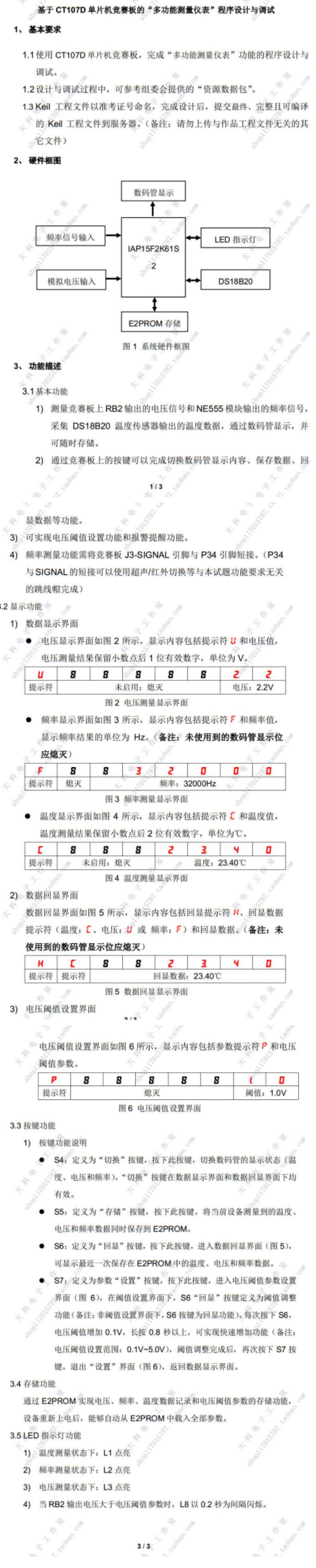
#include"reg52.h"
#include"intrins.h"
#include"iic.h"
#include"onewire.h"
sfr AUXR=0x8e;
int count_f;
int num_v;
int num_f;
int temperature;
int smg_mode;
int xian_mode;
int set_v=1;
int read_num_v;
int read_num_f;
int read_temperature;
int led=0xff;
int shan;
sbit S4=P3^3;
sbit S5=P3^2;
sbit S6=P3^1;
sbit S7=P3^0;
char code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xc1,0x8e,0xc6,0x89,0x8c,0x7f};
void smg_display();
void led_work();
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//================================iic and onewire
void get_v()
{
num_v=read_v();
}
void get_temperature()
{
temperature=rd_temperature();
}
//================================
//================================555 and 定时器
void Timer0Init(void) //100微秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD = 0x04; //设置定时器模式
TL0 = 0xff; //设置定时初始值
TH0 = 0xff; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
void timer0_server() interrupt 1
{
count_f++;
}
void Timer1Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x40; //定时器时钟1T模式
TMOD &= 0x0F; //设置定时器模式
TL1 = 0x00; //设置定时初始值
TH1 = 0x28; //设置定时初始值
TF1 = 0; //清除TF1标志
TR1 = 1; //定时器1开始计时
ET1=1;
EA=1;
}
int count_1;int count_2;int count_3;
void timer1_server() interrupt 3
{
count_1++;
if(count_1>=200)
{
num_f=count_f;
count_f=0;
count_1=0;
// get_v();
// get_temperature();
}
if(num_v>(set_v*10))
{
count_2++;
if(count_2>=40)
{
count_2=0;
if(shan==1)
{
shan=0;
}
else
{
shan=1;
}
}
}
else
{
shan=0;
}
if(S6==0&&smg_mode==2)
{
count_3++;
if(count_3>=160)
{
set_v=set_v+1;
if(set_v>=50)
{
set_v=1;
}
}
}
else
{
count_3=0;
}
}
//================================
//================================eeprom
void get_eeprom()
{
read_num_v=read_EEPROM(0x02);
read_num_v=(read_num_v<<8)|read_EEPROM(0x01);
read_num_f=read_EEPROM(0x04);
read_num_f=(read_num_f<<8)|read_EEPROM(0x03);
read_temperature=read_EEPROM(0x06);
read_temperature=(read_temperature<<8)|read_EEPROM(0x05);
}
//================================
//================================按键
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
//S4
if(S4==0)
{
Delay10ms();
if(S4==0)
{
smg_mode=0;
xian_mode++;
if(xian_mode>=3)
{
xian_mode=0;
}
}
while(!S4)
{
smg_display();
led_work();
}
}
//S5
if(S5==0)
{
Delay10ms();
if(S5==0)
{
write_EEPROM(0x01,num_v);//低位
Delay10ms();
write_EEPROM(0x02,num_v>>8);//高位
Delay10ms();
write_EEPROM(0x03,num_f);//低位
Delay10ms();
write_EEPROM(0x04,num_f>>8);//高位
Delay10ms();
write_EEPROM(0x05,temperature);//低位
Delay10ms();
write_EEPROM(0x06,temperature>>8);//高位
Delay10ms();
}
while(!S5)
{
smg_display();
led_work();
}
}
//S6
if(S6==0)
{
Delay10ms();
if(S6==0)
{
if(smg_mode==2)
{
set_v++;
if(set_v>50)
{
set_v=1;
}
write_EEPROM(0x07,set_v);
}
else
{
smg_mode=1;
get_eeprom();
}
}
while(!S6)
{
smg_display();
led_work();
}
}
//S7
if(S7==0)
{
Delay10ms();
if(S7==0)
{
if(smg_mode==0)
{
smg_mode=2;
}
else
{
smg_mode=0;
}
}
while(!S7)
{
smg_display();
led_work();
}
}
}
//================================
//================================数码管
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
choose_573(7);
P0=xianshi[10];
choose_573(0);
}
void smg_display()
{
if(smg_mode==0)
{
if(xian_mode==0) //电压
{
SMG(1,(num_v%100)/10);
SMG(2,(num_v%1000)/100);
SMG(2,16);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,11);
}
if(xian_mode==1) //频率
{
SMG(1,num_f%10);
if(num_f>=10)
{
SMG(2,(num_f%100)/10);
}
else
{
SMG(2,10);
}
if(num_f>=100)
{
SMG(3,(num_f%1000)/100);
}
else
{
SMG(3,10);
}
if(num_f>=1000)
{
SMG(4,(num_f%10000)/1000);
}
else
{
SMG(4,10);
}
if(num_f>=10000)
{
SMG(5,(num_f%100000)/10000);
}
else
{
SMG(5,10);
}
if(num_f>=100000)
{
SMG(6,(num_f%1000000)/100000);
}
else
{
SMG(6,10);
}
if(num_f>=1000000)
{
SMG(7,(num_f%10000000)/1000000);
}
else
{
SMG(7,10);
}
SMG(8,12);
}
if(xian_mode==2) //温度
{
SMG(1,temperature%10);
SMG(2,(temperature%100)/10);
SMG(3,16);
SMG(3,(temperature%1000)/100);
if(temperature>=1000)
{
SMG(4,(temperature%10000)/1000);
}
else
{
SMG(4,10);
}
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,13);
}
}
if(smg_mode==1)
{
if(xian_mode==0) //保存的电压
{
SMG(1,(read_num_v%100)/10);
SMG(2,(read_num_v%1000)/100);
SMG(2,16);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,11);
SMG(8,14);
}
if(xian_mode==1) //保存的频率
{
SMG(1,read_num_f%10);
if(read_num_f>=10)
{
SMG(2,(read_num_f%100)/10);
}
else
{
SMG(2,10);
}
if(read_num_f>=100)
{
SMG(3,(read_num_f%1000)/100);
}
else
{
SMG(3,10);
}
if(read_num_f>=1000)
{
SMG(4,(read_num_f%10000)/1000);
}
else
{
SMG(4,10);
}
if(read_num_f>=10000)
{
SMG(5,(read_num_f%100000)/10000);
}
else
{
SMG(5,10);
}
if(read_num_f>=100000)
{
SMG(6,(read_num_f%1000000)/100000);
}
else
{
SMG(6,10);
}
SMG(7,12);
SMG(8,14);
}
if(xian_mode==2) //保存的温度
{
SMG(1,read_temperature%10);
SMG(2,(read_temperature%100)/10);
SMG(3,16);
SMG(3,(read_temperature%1000)/100);
if(read_temperature>=1000)
{
SMG(4,(read_temperature%10000)/1000);
}
else
{
SMG(4,10);
}
SMG(5,10);
SMG(6,10);
SMG(7,13);
SMG(8,14);
}
}
if(smg_mode==2)
{
SMG(1,set_v%10);
SMG(2,set_v/10);
SMG(2,16);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,15);
}
}
//================================
//================================LED
void led_work()
{
if(smg_mode==0)
{
//L1
if(xian_mode==0)
{
led=(led&0xfe)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfe)|0x01;
choose_573(4);
P0=led;
choose_573(0);
}
//L2
if(xian_mode==1)
{
led=(led&0xfd)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfd)|0x02;
choose_573(4);
P0=led;
choose_573(0);
}
//L3
if(xian_mode==2)
{
led=(led&0xfb)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfb)|0x04;
choose_573(4);
P0=led;
choose_573(0);
}
}
//L8
if(shan==1)
{
led=(led&0x7f)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0x7f)|0x80;
choose_573(4);
P0=led;
choose_573(0);
}
}
//================================
void main()
{
init_system();
Timer0Init();
Timer1Init();
get_eeprom();
set_v=read_EEPROM(0x07);
while(1)
{
key_board();
smg_display();
get_v();
get_temperature();
led_work();
}
}
8届
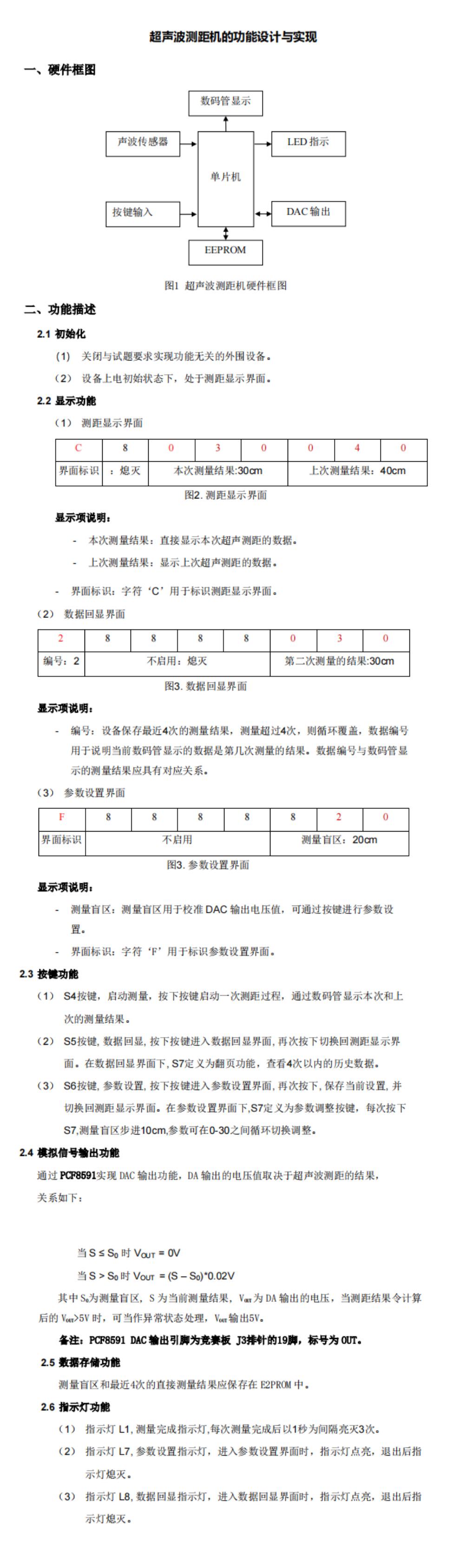
#include"reg52.h"
#include"iic.h"
#include"intrins.h"
int smg_mode=0;
int distance_work=0;
sbit TX=P1^0;
sbit RX=P1^1;
sfr AUXR=0x8e;
int distance=0;
int judge_distance=20;
sbit S4=P3^3;
sbit S5=P3^2;
sbit S6=P3^1;
sbit S7=P3^0;
int distance_history[]={0,0,0,0};
int distance_time=3;
int distance_temp_time=0;
int led=0xff;
int L1_temp=0;
char code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xc6,0x8e};
void smg_display();
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//===============================超声波
void Delay12us() //@12.000MHz
{
unsigned char i;
_nop_();
_nop_();
i = 33;
while (--i);
}
void send_wave()
{
int i;
for(i=0;i<8;i++)
{
TX=1;
Delay12us();
TX=0;
Delay12us();
}
}
void get_distance()
{
if(distance_work==1)
{
int time;
AUXR |= 0x40; //定时器时钟1T模式
TMOD &= 0x0F; //设置定时器模式
TL1 = 0x00; //设置定时初始值
TH1 = 0x00; //设置定时初始值
TF1 = 0; //清除TF1标志
TR1 = 0; //定时器1开始计时
send_wave();
TR1=1;
while(RX==1&&TF1==0);
TR1=0;
if(TF1==0)
{
time=TH1;
time=(time<<8)|TL1;
distance=time*0.0017;
}
else
{
TF1=0;
distance=999;
}
TH1=TL1=0x00;
L1_temp=1;
distance_work=0;
}
}
//===============================
//===============================按键
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
//S4
if(S4==0)
{
Delay10ms();
if(S4==0)
{
smg_mode=0;
distance_history[distance_temp_time]=distance;
distance_temp_time++;
if(distance_temp_time>=4)
{
distance_temp_time=0;
}
distance_work=1;
}
while(!S4)
{
smg_display();
}
}
//S5
if(S5==0)
{
Delay10ms();
if(S5==0)
{
if(smg_mode==1)
{
smg_mode=0;
}
else
{
smg_mode=1;
}
}
while(!S5)
{
smg_display();
}
}
//S6
if(S6==0)
{
Delay10ms();
if(S6==0) //参数保存
{
if(smg_mode==2)
{
smg_mode=0;
write_EEPROM(0x01,judge_distance);
}
else
{
smg_mode=2;
}
}
while(!S6)
{
smg_display();
}
}
//S7
if(S7==0)
{
Delay10ms();
if(S7==0)
{
if(smg_mode==1) //查询保存
{
distance_time++;
if(distance_time>=4)
{
distance_time=0;
}
}
if(smg_mode==2) //参数设置
{
judge_distance=judge_distance+10;
if(judge_distance>=40)
{
judge_distance=0;
}
}
}
while(!S7)
{
smg_display();
}
}
}
//===============================
//===============================数码管
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
choose_573(7);
P0=xianshi[10];
choose_573(0);
}
void smg_display()
{
if(smg_mode==0)
{
if(distance_temp_time==0)
{
SMG(1,distance_history[3]%10);
SMG(2,(distance_history[3]%100)/10);
SMG(3,(distance_history[3]%1000)/100);
}
else
{
SMG(1,distance_history[distance_temp_time-1]%10);
SMG(2,(distance_history[distance_temp_time-1]%100)/10);
SMG(3,(distance_history[distance_temp_time-1]%1000)/100);
}
SMG(4,distance%10);
SMG(5,(distance%100)/10);
SMG(6,(distance%1000)/100);
SMG(7,10);
SMG(8,11);
}
if(smg_mode==1)
{
SMG(1,distance_history[distance_time]%10);
SMG(2,(distance_history[distance_time]%100)/10);
SMG(3,(distance_history[distance_time]%1000)/100);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,2);
}
if(smg_mode==2)
{
SMG(1,judge_distance%10);
SMG(2,(judge_distance%100)/10);
SMG(3,(judge_distance%1000)/100);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,12);
}
}
//===============================
//===============================DA输出
void DA_out()
{
if(distance<=judge_distance)
{
DA(0);
}
else
{
if(((distance-judge_distance)*0.02)>255)
{
DA(255);
}
else
{
DA((distance-judge_distance)*0.02);
}
}
}
//===============================
//===============================LED
void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count;int L1_work=0;int i=0;
void time0_server() interrupt 1
{
if(L1_temp==1)
{
count++;
if(count>=200)
{
i++;
count=0;
if(i>=7)
{
i=1;
L1_temp=0;
}
}
}
}
void led_work()
{
//L8
if(smg_mode==1)
{
led=(led&0x7f)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0x7f)|0x80;
choose_573(4);
P0=led;
choose_573(0);
}
//L7
if(smg_mode==2)
{
led=(led&0xbf)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xbf)|0x40;
choose_573(4);
P0=led;
choose_573(0);
}
//L1
if(L1_temp==1)
{
// led=(led&0xfe)|0x00;
// choose_573(4);
// P0=led;
// choose_573(0);
//
if(i%2==1)
{
led=(led&0xfe)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfe)|0x01;
choose_573(4);
P0=led;
choose_573(0);
}
}
}
//===============================
void main()
{
init_system();
judge_distance=read_EEPROM(0x01);
Timer0Init();
while(1)
{
get_distance();
smg_display();
key_board();
DA_out();
led_work();
}
}
7届
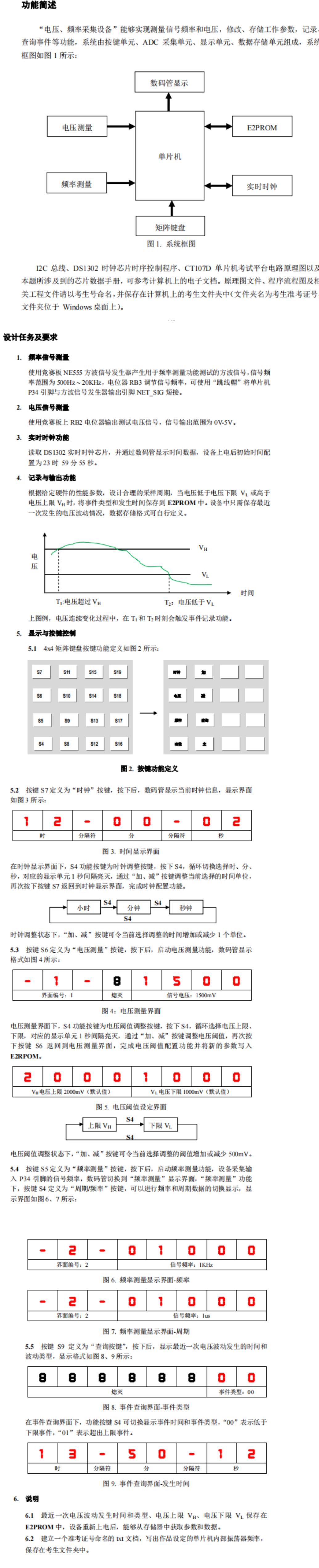
#include "reg52.h"
#include"intrins.h"
#include"ds1302.h"
#include"iic.h"
sfr AUXR=0x8e;
sfr P4=0xC0;
int code write_add[]={0x80,0x82,0x84};
int code read_add[]={0x81,0x83,0x85};
int time[]={0x55,0x59,0x23};
int judge_time[]={0x00,0x00,0x00};
int code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xbf};
int temp_h,temp_m,temp_s;
int time_mode=0;
int v_mode=0;
int f_mode=0;
int count_f;int num_f;
int num_t;//周期
int num_v;
int smg_mode=0;
int shanshuo=0;//闪烁
int v_h=2000;
int v_l=1000;
int judge_mode;
int judge_temp=0;
sbit R1=P3^0;
sbit R2=P3^1;
sbit R3=P3^2;
sbit R4=P3^3;
sbit C4=P3^4;
sbit C3=P3^5;
sbit C2=P4^2;
sbit C1=P4^4;
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//====================================时间
void write_time()
{
int i;
Write_Ds1302_Byte(0x8e,0x00);
for(i=0;i<3;i++)
{
Write_Ds1302_Byte(write_add[i],time[i]);
}
Write_Ds1302_Byte(0x8e,0x80);
}
void read_time()
{
int i;
for(i=0;i<3;i++)
{
time[i]=Read_Ds1302_Byte(read_add[i]);
}
}
//====================================
//====================================555 and v
void Timer0Init(void) //100微秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD = 0x04; //设置定时器模式
TL0 = 0xff; //设置定时初始值
TH0 = 0xff; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
void time0_service() interrupt 1
{
count_f++;
}
void V()
{
num_v=get_v();
}
//====================================
//====================================中断
void Timer1Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x40; //定时器时钟1T模式
TMOD &= 0x0F; //设置定时器模式
TL1 = 0x00; //设置定时初始值
TH1 = 0x28; //设置定时初始值
TF1 = 0; //清除TF1标志
TR1 = 1; //定时器1开始计时
ET1=1;
EA=1;
}
int count_1;int count_2;
void time1_server() interrupt 3
{
count_1++;
count_2++;
if(count_1>=100)
{
count_1=0;
num_f=count_f;
count_f=0;
// V();
num_t=1000000/num_f;
}
if(count_2>=200)
{
count_2=0;
if(shanshuo==0)
{
shanshuo=1;
}
else
{
shanshuo=0;
}
}
}
//====================================
//====================================键盘
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
R1=0;R2=R3=R4=1;
C1=C2=C3=C4=1;
//S7
if(C1==0)
{
Delay10ms();
if(C1==0)
{
smg_mode=0;
time_mode=0;
}
while(!C1);
}
//S11 加
if(C2==0)
{
Delay10ms();
if(C2==0)
{
if(smg_mode==0)//time
{
if(time_mode==1)
{
temp_h=(time[2]/16)*10+time[2]%16;
temp_h++;
if(temp_h>=24)
{
temp_h=0;
}
time[2]=((temp_h/10)*6)+temp_h;
write_time();
}
if(time_mode==2)
{
temp_m=(time[1]/16)*10+time[1]%16;
temp_m++;
if(temp_m>=60)
{
temp_m=0;
}
time[1]=((temp_m/10)*6)+temp_m;
write_time();
}
if(time_mode==3)
{
temp_s=(time[0]/16)*10+time[0]%16;
temp_s++;
if(temp_s>=60)
{
temp_s=0;
}
time[0]=((temp_s/10)*6)+temp_s;
write_time();
}
}
if(smg_mode==1)
{
if(v_mode==1)
{
v_h=v_h+500;
if(v_h>9500)
{
v_h=9500;
}
}
if(v_mode==2)
{
v_l=v_l+500;
if(v_l>v_h)
{
v_l=v_l-500;
}
}
}
}
while(!C2);
}
R2=0;R1=R3=R4=1;
C1=C2=C3=C4=1;
//S6
if(C1==0)
{
Delay10ms();
if(C1==0)
{
smg_mode=1;
v_mode=0;
}
while(!C1);
}
//S10减
if(C2==0)
{
Delay10ms();
if(C2==0)
{
if(smg_mode==0)//time
{
if(time_mode==1)
{
temp_h=(time[2]/16)*10+time[2]%16;
temp_h--;
if(temp_h<0)
{
temp_h=23;
}
time[2]=((temp_h/10)*6)+temp_h;
write_time();
}
if(time_mode==2)
{
temp_m=(time[1]/16)*10+time[1]%16;
temp_m--;
if(temp_m<0)
{
temp_m=59;
}
time[1]=((temp_m/10)*6)+temp_m;
write_time();
}
if(time_mode==3)
{
temp_s=(time[0]/16)*10+time[0]%16;
temp_s--;
if(temp_s<0)
{
temp_s=59;
}
time[0]=((temp_s/10)*6)+temp_s;
write_time();
}
}
if(smg_mode==1)
{
if(v_mode==1)
{
v_h=v_h-500;
if(v_h<v_l)
{
v_h=v_h+500;
}
}
if(v_mode==2)
{
v_l=v_l-500;
if(v_l<0)
{
v_l=0;
}
}
}
}
while(!C2);
}
R3=0;R1=R2=R4=1;
C1=C2=C3=C4=1;
//S5
if(C1==0)
{
Delay10ms();
if(C1==0)
{
smg_mode=2;
f_mode=0;
}
while(!C1);
}
//S9 查询
if(C2==0)
{
Delay10ms();
if(C2==0)
{
smg_mode=3;
judge_temp=0;
}
while(!C2);
}
R4=0;R1=R2=R3=1;
C1=C2=C3=C4=1;
//S4
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(smg_mode==0)
{
time_mode++;
if(time_mode>=4)
{
time_mode=0;
}
}
if(smg_mode==1)
{
v_mode++;
if(v_mode>=3)
{
v_mode=0;
write_EEPROM(0x01,v_h);
Delay10ms();
write_EEPROM(0x02,v_h>>8);
Delay10ms();
write_EEPROM(0x03,v_l);
Delay10ms();
write_EEPROM(0x04,v_l>>8);
}
}
if(smg_mode==2)
{
f_mode++;
if(f_mode>=2)
{
f_mode=0;
}
}
if(smg_mode==3)
{
judge_temp++;
if(judge_temp>=2)
{
judge_temp=0;
}
}
}
while(!C1);
}
}
//====================================
//====================================数码管
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
choose_573(7);
P0=xianshi[10];
choose_573(0);
}
void smg_display()
{
if(smg_mode==0)//时间显示
{
if(time_mode==3&&shanshuo==1)
{
SMG(1,10);
SMG(2,10);
}
else
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
}
SMG(3,11);
if(time_mode==2&&shanshuo==1)
{
SMG(4,10);
SMG(5,10);
}
else
{
SMG(4,time[1]%16);
SMG(5,time[1]/16);
}
SMG(6,11);
if(time_mode==1&&shanshuo==1)
{
SMG(7,10);
SMG(8,10);
}
else
{
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
}
if(smg_mode==1)
{
if(v_mode==0)
{
SMG(1,num_v%10);
SMG(2,(num_v%100)/10);
SMG(3,(num_v%1000)/100);
SMG(4,(num_v%10000)/1000);
SMG(5,10);
SMG(6,11);
SMG(7,smg_mode);
SMG(8,11);
}
if(v_mode==1&&shanshuo==1)
{
SMG(1,v_l%10);
SMG(2,(v_l%100)/10);
SMG(3,(v_l%1000)/100);
SMG(4,(v_l%10000)/1000);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,10);
}
else if(v_mode==2&&shanshuo==1)
{
SMG(1,10);
SMG(2,10);
SMG(3,10);
SMG(4,10);
SMG(5,v_h%10);
SMG(6,(v_h%100)/10);
SMG(7,(v_h%1000)/100);
SMG(8,(v_h%10000)/1000);
}
else if((v_mode==1||v_mode==2)&&shanshuo==0)
{
SMG(1,v_l%10);
SMG(2,(v_l%100)/10);
SMG(3,(v_l%1000)/100);
SMG(4,(v_l%10000)/1000);
SMG(5,v_h%10);
SMG(6,(v_h%100)/10);
SMG(7,(v_h%1000)/100);
SMG(8,(v_h%10000)/1000);
}
}
if(smg_mode==2)
{
if(f_mode==0)
{
SMG(1,num_f%10);
SMG(2,(num_f%100)/10);
SMG(3,(num_f%1000)/100);
SMG(4,(num_f%10000)/1000);
SMG(5,(num_f%100000)/10000);
SMG(6,11);
SMG(7,smg_mode);
SMG(8,11);
}
if(f_mode==1)
{
SMG(1,num_t%10);
SMG(2,(num_t%100)/10);
SMG(3,(num_t%1000)/100);
SMG(4,(num_t%10000)/1000);
SMG(5,(num_t%100000)/10000);
SMG(6,11);
SMG(7,smg_mode);
SMG(8,11);
}
}
if(smg_mode==3)
{
if(judge_temp==0)
{
SMG(1,judge_mode);
SMG(2,0);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,10);
}
if(judge_temp==1)
{
SMG(1,judge_time[0]%16);
SMG(2,judge_time[0]/16);
SMG(3,11);
SMG(4,judge_time[1]%16);
SMG(5,judge_time[1]/16);
SMG(6,11);
SMG(7,judge_time[2]%16);
SMG(8,judge_time[2]/16);
}
}
}
//====================================
//====================================判断
void judge_work()
{
//大于
if(num_v==v_h)
{
// Delay10ms();
// if(num_v<v_h)
{
int i;
judge_mode=1;
for(i=0;i<3;i++)
{
judge_time[i]=time[i];
}
}
}
//小于
if(num_v==v_l&&num_v<v_h)
{
Delay10ms();
if(num_v>=v_l)
{
int i;
judge_mode=0;
for(i=0;i<3;i++)
{
judge_time[i]=time[i];
}
}
}
}
//====================================
//====================================eeprom_read
void eeprom_read()
{
v_h=read_EEPROM(0x02);
v_h=(v_h<<8)|read_EEPROM(0x01);
v_l=read_EEPROM(0x04);
v_l=(v_l<<8)|read_EEPROM(0x03);
}
//====================================
void main()
{
init_system();
write_time();
Timer0Init();
Timer1Init();
eeprom_read();
while(1)
{
read_time();
key_board();
smg_display();
V();
judge_work();
}
}
6届
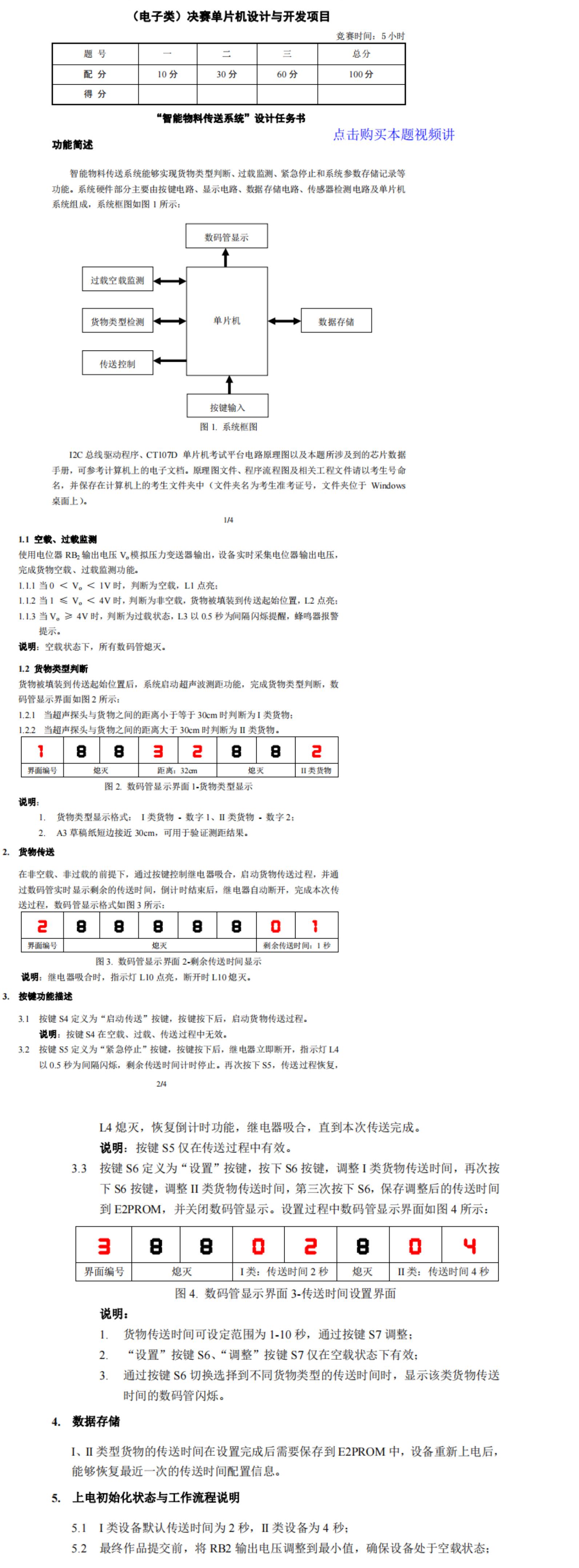
#include <STC12C5A60S2.H>
#include"intrins.h"
#include"iic.h"
sbit TX=P1^0;
sbit RX=P1^1;
int led_work=0xff;
int rb_2;
int L3_mode=0;
int distance;
int Y5_work=0x00;
char last_time_1=2;//一类货物传送时间
char last_time_2=4;//二类货物传送时间
int weight_mode=0;
int smg_mode=1;
int work_mode; //货物类型
sbit S4=P3^3;
sbit S5=P3^2;
sbit S6=P3^1;
sbit S7=P3^0;
int running=0;//是否运行
int last_time;
int L4_work=0;
int set_mode=0;
unsigned int code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff};
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//==============================定时器0
void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count_1=0;int count_2=0;
void service_timer0() interrupt 1
{
count_1++;
if(count_1>=100)
{
count_1=0;
L3_mode++;
if(L3_mode>=2)
{
L3_mode=0;
}
}
if(running!=0&&last_time!=0)
{
count_2++;
if(count_2>=200)
{
count_2=0;
last_time--;
if(last_time==0)
{
running=0;
smg_mode=1;
Y5_work=(Y5_work&0xef)|0x00;
choose_573(5);
P0=Y5_work;
choose_573(0);
}
}
}
}
//==============================
//==============================led
void rb_2_display()
{
rb_2=get_rb2();
}
void led_display()
{
//L1
if(rb_2<100)
{
weight_mode=1;
led_work=(led_work&0xfe)|0x00;
choose_573(4);
P0=led_work;
choose_573(0);
}
else
{
led_work=(led_work&0xfe)|0x01;
choose_573(4);
P0=led_work;
choose_573(0);
}
//L2
if(rb_2>=100&&rb_2<400)
{
weight_mode=2;
led_work=(led_work&0xfd)|0x00;
choose_573(4);
P0=led_work;
choose_573(0);
}
else
{
led_work=(led_work&0xfd)|0x02;
choose_573(4);
P0=led_work;
choose_573(0);
}
//L3
if(rb_2>=400&&L3_mode==1)
{
weight_mode=3;
led_work=(led_work&0xfb)|0x00;
Y5_work=(Y5_work&0xbf)|0x40;
choose_573(4);
P0=led_work;
choose_573(5);
P0=Y5_work;
choose_573(0);
}
else if(rb_2>=400&&L3_mode==0)
{
weight_mode=3;
led_work=(led_work&0xfb)|0x04;
Y5_work=(Y5_work&0xbf)|0x40;
choose_573(4);
P0=led_work;
choose_573(5);
P0=Y5_work;
choose_573(0);
}
else if(rb_2<400)
{
led_work=(led_work&0xfb)|0x04;
Y5_work=(Y5_work&0xbf)|0x00;
choose_573(4);
P0=led_work;
choose_573(5);
P0=Y5_work;
choose_573(0);
}
}
//==============================
//==============================超声波
void Delay12us() //@12.000MHz
{
unsigned char i;
_nop_();
_nop_();
i = 33;
while (--i);
}
void send_wave()
{
int i;
for(i=0;i<8;i++)
{
TX=1;
Delay12us();
TX=0;
Delay12us();
}
}
void get_distance()
{
unsigned int time=0;
AUXR |= 0x40; //定时器时钟1T模式
TMOD &= 0x0F; //设置定时器模式
TL1 = 0x00; //设置定时初始值
TH1 = 0x00; //设置定时初始值
TF1 = 0; //清除TF1标志
TR1 = 0; //定时器1开始计时
send_wave();
TR1=1;
while(RX==1&&TF1==0);
TR1=0;
if(TF1==0)
{
time=TH1;
time=(time<<8)|TL1;
distance=time*0.017;
distance=distance/12;
}
else
{
distance=999;
TF1=0;
}
TH1=TL1=0;
if(distance<=30)
{
work_mode=1;
}
else if(distance>30)
{
work_mode=2;
}
}
//==============================
//==============================按键
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
//S4
if(S4==0&&weight_mode==2)
{
Delay10ms();
if(S4==0&&weight_mode==2)
{
smg_mode=2;
Y5_work=(Y5_work&0xef)|0x10;
choose_573(5);
P0=Y5_work;
choose_573(0);
running=1;
if(work_mode==1)
{
last_time=last_time_1;
}
if(work_mode==2)
{
last_time=last_time_2;
}
}
while(!S4);
}
//S5
if(S5==0&&smg_mode==2)
{
Delay10ms();
if(S5==0&&smg_mode==2)
{
if(running==1)
{
running=0;
Y5_work=(Y5_work&0xef)|0x00;
choose_573(5);
P0=Y5_work;
choose_573(0);
L4_work=1;
}
else
{
running=1;
Y5_work=(Y5_work&0xef)|0x10;
choose_573(5);
P0=Y5_work;
choose_573(0);
L4_work=0;
}
}
while(!S5);
}
//S6
if(S6==0&&weight_mode==1)
{
Delay10ms();
if(S6==0&&weight_mode==1)
{
smg_mode=3;
set_mode++;
if(set_mode>=3)
{
set_mode=0;
smg_mode=1;
}
}
while(!S6);
}
//S7
if(S7==0&&smg_mode==3)
{
Delay10ms();
if(S7==0&&smg_mode==3)
{
if(set_mode==1)
{
last_time_1++;
if(last_time_1>10)
{
last_time_1=1;
}
}
if(set_mode==2)
{
last_time_2++;
if(last_time_2>10)
{
last_time_2=1;
}
}
}
while(!S7);
}
}
//==============================
//==============================L4
void L4_display()
{
if(L4_work==1)
{
if(L3_mode==1)
{
led_work=(led_work&0xf7)|0x00;
choose_573(4);
P0=led_work;
choose_573(0);
}
else
{
led_work=(led_work&0xf7)|0x08;
choose_573(4);
P0=led_work;
choose_573(0);
}
}
else if(L4_work==0)
{
led_work=(led_work&0xf7)|0x08;
choose_573(4);
P0=led_work;
choose_573(0);
}
}
//==============================
//==============================smg
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
choose_573(7);
P0=xianshi[10];
choose_573(0);
}
void smg_display()
{
//测试
// SMG(1,distance%10);
// SMG(2,(distance%100)/10);
// SMG(3,(distance%1000)/100);
// SMG(4,weight_mode);
// SMG(5,10);
// SMG(6,smg_mode);
// SMG(7,10);
// SMG(8,10);
if(smg_mode==1)
{
SMG(1,work_mode);
SMG(2,10);
SMG(3,10);
SMG(4,distance%10);
SMG(5,(distance%100)/10);
SMG(6,10);
SMG(7,10);
SMG(8,smg_mode);
}
if(smg_mode==2)
{
SMG(1,last_time%10);
SMG(2,(last_time%100)/10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,smg_mode);
}
if(smg_mode==3)
{
if(set_mode==2&&L3_mode==1)
{
SMG(1,last_time_2%10);
SMG(2,(last_time_2%100)/10);
}
else if(set_mode==2&&L3_mode==0)
{
SMG(1,10);
SMG(2,10);
}
else if(set_mode!=2)
{
SMG(1,last_time_2%10);
SMG(2,(last_time_2%100)/10);
}
SMG(3,10);
if(set_mode==1&&L3_mode==1)
{
SMG(4,last_time_1%10);
SMG(5,(last_time_1%100)/10);
}
else if(set_mode==1&&L3_mode==0)
{
SMG(4,10);
SMG(5,10);
}
else if(set_mode!=1)
{
SMG(4,last_time_1%10);
SMG(5,(last_time_1%100)/10);
}
SMG(6,10);
SMG(7,10);
SMG(8,smg_mode);
}
}
//==============================
//==============================eeprom
void eeprom_work()
{
if(set_mode==0)
{
write_EEPROM(0x01,last_time_1);
Delay10ms();
write_EEPROM(0x33,last_time_2);
}
}
//==============================
void main()
{
init_system();
Timer0Init();
last_time_1=read_EEPROM(0x01);
Delay10ms();
last_time_2=read_EEPROM(0x33);
while(1)
{
rb_2_display();
led_display();
smg_display();
get_distance();
key_board();
L4_display();
eeprom_work();
}
}
5届
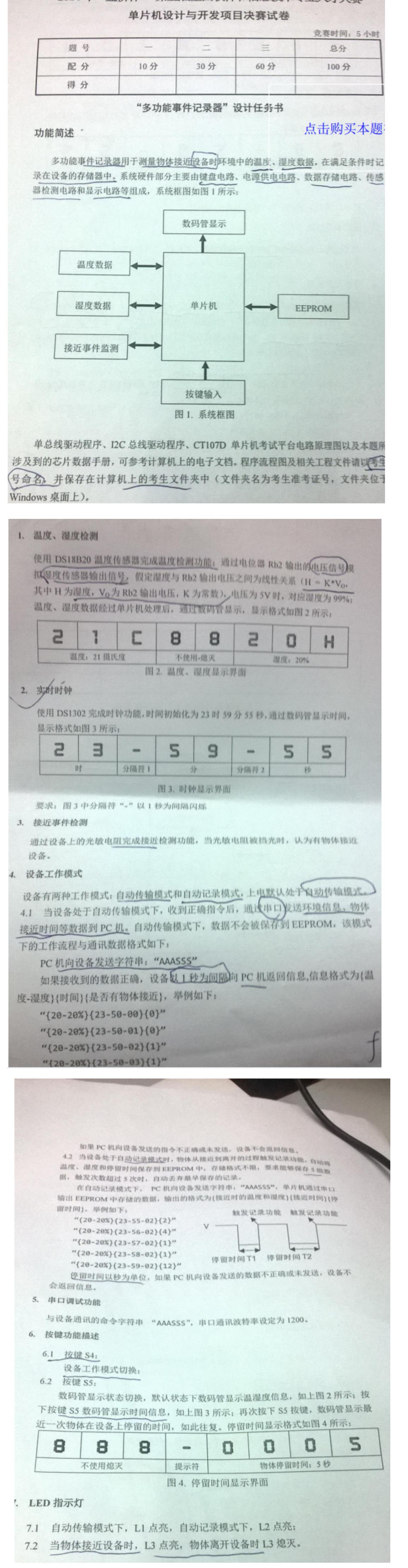
//#include"reg52.h"
#include <STC12C5A60S2.H>
#include"intrins.h"
#include"ds1302.h"
#include"onewire.h"
#include"iic.h"
int temperature;
unsigned int urat;
int code write_add[]={0x80,0x82,0x84};
int code read_add[]={0x81,0x83,0x85};
int time[]={0x55,0x59,0x23};
unsigned int code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xbf,0xc6,0x89};
sbit S4=P3^3;
sbit S5=P3^2;
int smg_mode=0;
int work_mode=0;//自动
int RD_1,RD_3;
int last_time;//停留时间
int led_work=0xff;
int eeprom_time;
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;//关闭
case(4):P2=(P2&0x1f)|0x80;break;//Y4
case(5):P2=(P2&0x1f)|0xa0;break;//Y5
case(6):P2=(P2&0x1f)|0xc0;break;//Y6
case(7):P2=(P2&0x1f)|0xe0;break;//Y7
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//=======================================time
void write_time()
{
int i;
Write_Ds1302_Byte(0x8e,0x00);
for(i=0;i<3;i++)
{
Write_Ds1302_Byte(write_add[i],time[i]);
}
Write_Ds1302_Byte(0x8e,0x80);
}
void read_time()
{
int i;
for(i=0;i<3;i++)
{
time[i]=Read_Ds1302_Byte(read_add[i]);
}
}
//=======================================
//=======================================temperature rd1 rd3
void get_temperature()
{
temperature=rd_temperature();
}
void get_rd1()//光敏
{
RD_1=Rd1();
}
void get_rd3()//电阻
{
RD_3=Rd3();
}void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count_1;
void service_time0() interrupt 1
{
if(RD_3<=100)
{
count_1++;
last_time=count_1/200;
}
else
{
count_1=0;
// last_time=0; //计时消零
}
}
//=======================================
//=======================================key_board
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
if(S5==0)
{
Delay10ms();
if(S5==0)
{
smg_mode++;
if(smg_mode>=3)
{
smg_mode=0;
}
}
while(!S5);
}
if(S4==0)
{
Delay10ms();
if(S4==0)
{
work_mode++;
if(work_mode>=2)
{
work_mode=0;
}
}
while(!S4);
}
}
//=======================================
//=======================================smg
void Delay300us() //@11.0592MHz
{
unsigned char i, j;
_nop_();
_nop_();
i = 4;
j = 54;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay300us();
choose_573(7);
P0=xianshi[10];
choose_573(0);
}
void smg_display()
{
if(smg_mode==0)
{
SMG(1,13);
SMG(2,RD_1%10);
SMG(3,RD_1/10);
SMG(4,10);
SMG(5,10);
SMG(6,12);
SMG(7,temperature%10);
SMG(8,temperature/10);
}
if(smg_mode==1)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
if(time[0]%2==0)
{
SMG(3,10);
}
else
{
SMG(3,11);
}
SMG(4,time[1]%16);
SMG(5,time[1]/16);
if(time[0]%2==0)
{
SMG(6,10);
}
else
{
SMG(6,11);
}
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
if(smg_mode==2)
{
SMG(1,last_time%10);
SMG(2,(last_time%100)/10);
SMG(3,(last_time%1000)/100);
SMG(4,(last_time%10000)/1000);
SMG(5,11);
// SMG(6,RD_3%10);
// SMG(7,(RD_3%100)/10);
// SMG(8,(RD_3%1000)/100);
SMG(6,10);
SMG(7,10);
SMG(8,10);
}
}
//=======================================
//=======================================串口
//void UartInit(void) //9600bps@11.0592MHz
//{
// SCON = 0x50; //8位数据,可变波特率
// AUXR |= 0x40; //定时器时钟1T模式
// AUXR &= 0xFE; //串口1选择定时器1为波特率发生器
// TMOD &= 0x0F; //设置定时器模式
// TL1 = 0xE0; //设置定时初始值
// TH1 = 0xFE; //设置定时初始值
// ET1 = 0; //禁止定时器%d中断
// TR1 = 1; //定时器1开始计时
//
// ES=1;
// EA=0;
//}
//void service_Uart() interrupt 4
//{
// if(RI==1)
// {
// urat=SBUF;
// RI=0;
// }
//}
//void sendByte(unsigned char dat)
//{
// SBUF=dat;
// while(TI=0);
// TI=0;
//}
//void sendString(unsigned char *str)
//{
// while(*str!='\0')
// {
// sendByte(*str++);
// }
//}
//void urat_work()
//{
// unsigned int *temp_urat=&temperature;
// if(RD_3<=30)
// {
// sendString(temp_urat);
// }
//}
//=======================================
//=======================================led
void led_display()
{
if(work_mode==0)
{
led_work=(led_work&0xfe)|0x00;
choose_573(4);
P0=led_work;
choose_573(0);
}
else
{
led_work=(led_work&0xfe)|0x01;
choose_573(4);
P0=led_work;
choose_573(0);
}
if(work_mode==1)
{
led_work=(led_work&0xfd)|0x00;
choose_573(4);
P0=led_work;
choose_573(0);
}
else
{
led_work=(led_work&0xfd)|0x02;
choose_573(4);
P0=led_work;
choose_573(0);
}
if(RD_3<=100)
{
led_work=(led_work&0xfb)|0x00;
choose_573(4);
P0=led_work;
choose_573(0);
}
else
{
led_work=(led_work&0xfb)|0x04;
choose_573(4);
P0=led_work;
choose_573(0);
}
}
//=======================================
//=======================================eeprom
void eeprom_time_work()
{
if(RD_3>=100)
{
Delay300us();
if(RD_3<=100)
{
eeprom_time++;
}
}
}
void eeprom()
{
if(work_mode==1)
{
if(eeprom_time%5==0)
{
write_EEPROM(0x01,temperature);
write_EEPROM(0x02,RD_3);
write_EEPROM(0x03,time[2]);
write_EEPROM(0x04,time[1]);
write_EEPROM(0x05,time[0]);
write_EEPROM(0x06,last_time);
}
if(eeprom_time%5==1)
{
write_EEPROM(0x11,temperature);
write_EEPROM(0x12,RD_3);
write_EEPROM(0x13,time[2]);
write_EEPROM(0x14,time[1]);
write_EEPROM(0x15,time[0]);
write_EEPROM(0x16,last_time);
}
if(eeprom_time%5==2)
{
write_EEPROM(0x21,temperature);
write_EEPROM(0x22,RD_3);
write_EEPROM(0x23,time[2]);
write_EEPROM(0x24,time[1]);
write_EEPROM(0x25,time[0]);
write_EEPROM(0x26,last_time);
}
if(eeprom_time%5==3)
{
write_EEPROM(0x31,temperature);
write_EEPROM(0x32,RD_3);
write_EEPROM(0x33,time[2]);
write_EEPROM(0x34,time[1]);
write_EEPROM(0x35,time[0]);
write_EEPROM(0x36,last_time);
}
if(eeprom_time%5==4)
{
write_EEPROM(0x41,temperature);
write_EEPROM(0x42,RD_3);
write_EEPROM(0x43,time[2]);
write_EEPROM(0x44,time[1]);
write_EEPROM(0x45,time[0]);
write_EEPROM(0x46,last_time);
}
}
}
//=======================================
void main()
{
init_system();
write_time();
Timer0Init();
while(1)
{
key_board();
get_temperature();
get_rd1();
get_rd3();
smg_display();
read_time();
led_display();
eeprom_time_work();
eeprom();
}
}
4届
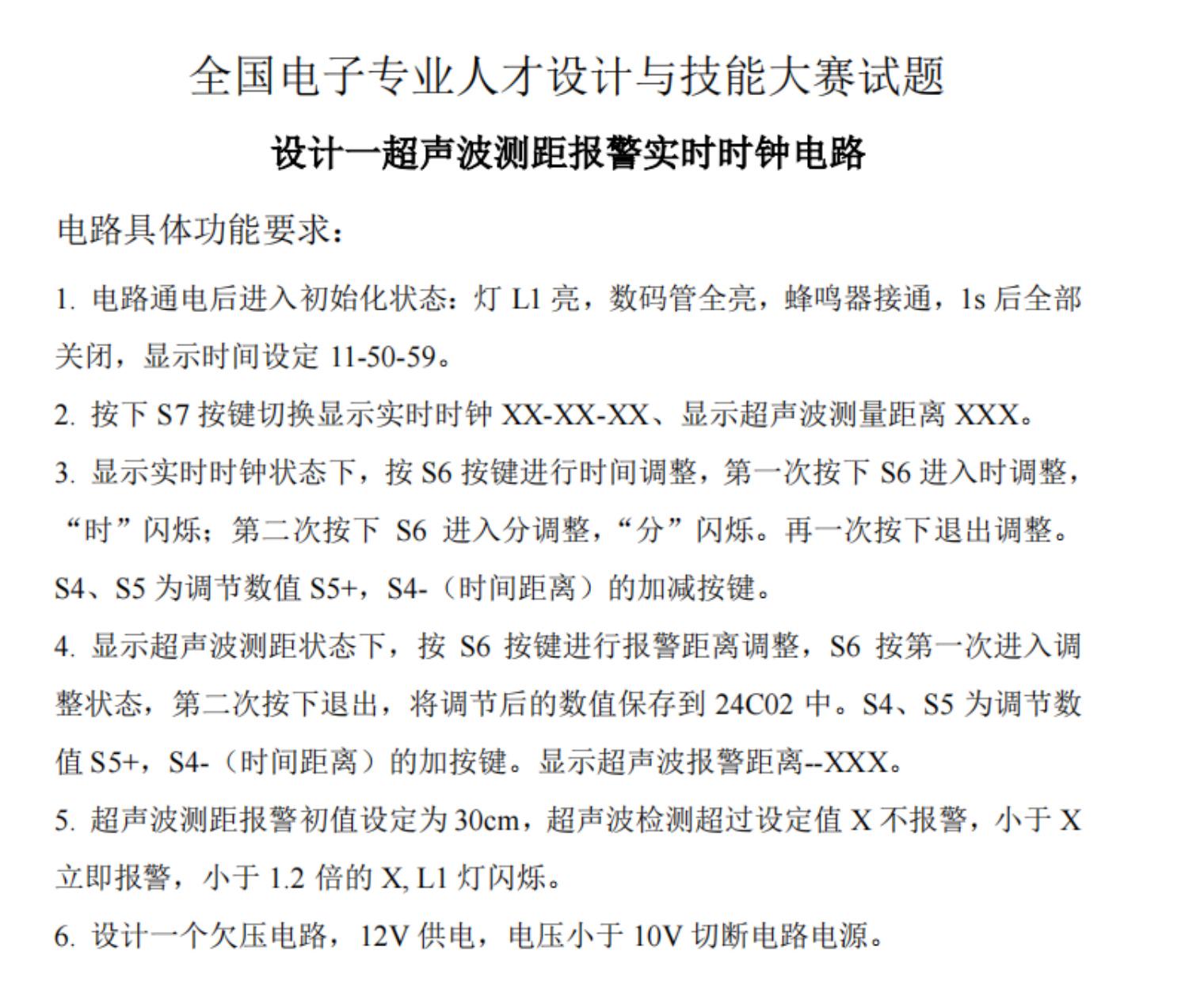
#include"reg52.h"
#include"intrins.h"
#include"ds1302.h"
sbit TX=P1^0;
sbit RX=P1^1;
int distance;
sfr AUXR=0x8e;
sbit S4=P3^3;
sbit S5=P3^2;
sbit S6=P3^1;
sbit S7=P3^0;
int smg_mode=0;
int time_mode=4;
int code write_add[]={0x80,0x82,0x84,0x86,0x88,0x8a,0x8c};
int code read_add[]={0x81,0x83,0x85,0x87,0x89,0x8b,0x8d};
unsigned int code xianshi[12]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xbf};
int time[]={0x59,0x50,0x11};
int distance_alarm=30;
int time_temp;
int shanshuo=0;
void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count_1=0;
void timer0_server() interrupt 1
{
count_1++;
if(count_1>=100)
{
count_1=0;
shanshuo++;
if(shanshuo>=2)
{
shanshuo=0;
}
}
}
void choose_573(int i)
{
switch(i)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void Delay1000ms() //@11.0592MHz
{
unsigned char i, j, k;
_nop_();
_nop_();
i = 43;
j = 6;
k = 203;
do
{
do
{
while (--k);
} while (--j);
} while (--i);
}
void init_system()
{
choose_573(4);
P0=0x00;
choose_573(5);
P0=0xff;
choose_573(0);
Delay1000ms();
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
}
//====================================time
void set_time()
{
int i;
Write_Ds1302_Byte(0x8e,0x00);
for(i=0;i<3;i++)
{
Write_Ds1302_Byte(write_add[i],time[i]);
}
Write_Ds1302_Byte(0x8e,0x80);
}
void read_time()
{
int i;
for(i=0;i<3;i++)
{
time[i]=Read_Ds1302_Byte(read_add[i]);
}
}
//====================================
//====================================超声波测距
void Delay12us() //@11.0592MHz
{
unsigned char i;
_nop_();
_nop_();
_nop_();
i = 30;
while (--i);
}
void send_ware()
{
int i;
for(i=0;i<8;i++)
{
TX=1;
Delay12us();
TX=0;
Delay12us();
}
}
void get_distance()
{
int time;
AUXR |= 0x40; //定时器时钟1T模式
TMOD &= 0x0F; //设置定时器模式
TL1 = 0x00; //设置定时初始值
TH1 = 0x00; //设置定时初始值
TF1 = 0; //清除TF1标志
TR1 = 0; //waring
send_ware();
TR1=1;
while(RX==1&&TF1==0);
TR1=0;
if(TF1==0)
{
time=TH1;
time=(time<<8)|TL1;
distance=time*0.017;
}
else
{
TF1=0;
distance=999;
}
TH1=TL1=0x00;
}
//====================================
//==================================== key_board
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
if(S7==0)
{
Delay10ms();
if(S7==0)
{
smg_mode++;
if(smg_mode>=2)
{
smg_mode=0;
}
}
while(!S7);
}
//S6
if(S6==0&&(smg_mode==0||smg_mode==2))
{
Delay10ms();
if(S6==0&&(smg_mode==0||smg_mode==2))
{
smg_mode=2;
time_mode++;
if(time_mode>=4)
{
time_mode=0;
}
}
while(!S6);
}
if(S6==0&&smg_mode==1)
{
Delay10ms();
if(S6==0&&smg_mode==1)
{
smg_mode=3;
}
while(!S6);
}
//S5
if(S5==0)
{
Delay10ms();
if(S5==0)
{
if(smg_mode==3)
{
distance_alarm++;
}
if(smg_mode==2)
{
if(time_mode==0)
{
time_temp=(time[2]/16*10)+time[2]%16;
time_temp++;
time_temp=time_temp+((time_temp/10)*6);
time[2]=time_temp;
set_time();
}
if(time_mode==1)
{
time_temp=(time[1]/16*10)+time[1]%16;
time_temp++;
time_temp=time_temp+((time_temp/10)*6);
time[1]=time_temp;
set_time();
}
if(time_mode==2)
{
time_temp=(time[0]/16*10)+time[0]%16;
time_temp++;
time_temp=time_temp+((time_temp/10)*6);
time[0]=time_temp;
set_time();
}
}
}
while(!S5);
}
//S4
if(S4==0)
{
Delay10ms();
if(S4==0)
{
if(smg_mode==3)
{
distance_alarm--;
}
if(smg_mode==2)
{
if(time_mode==0)
{
time_temp=(time[2]/16*10)+time[2]%16;
time_temp--;
time_temp=time_temp+((time_temp/10)*6);
time[2]=time_temp;
set_time();
}
if(time_mode==1)
{
time_temp=(time[1]/16*10)+time[1]%16;
time_temp--;
time_temp=time_temp+((time_temp/10)*6);
time[1]=time_temp;
set_time();
}
if(time_mode==2)
{
time_temp=(time[0]/16*10)+time[0]%16;
time_temp--;
time_temp=time_temp+((time_temp/10)*6);
time[0]=time_temp;
set_time();
}
}
}
while(!S4);
}
}
//====================================
//====================================smg
void Delay300us() //@11.0592MHz
{
unsigned char i, j;
_nop_();
_nop_();
i = 4;
j = 54;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay300us();
}
void smg_display()
{
if(smg_mode==0||time_mode==3)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
SMG(3,11);
SMG(4,time[1]%16);
SMG(5,time[1]/16);
SMG(6,11);
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
if(smg_mode==1)
{
SMG(1,distance%10);
SMG(2,(distance%100)/10);
SMG(3,(distance%1000)/100);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,10);
}
if(smg_mode==3)
{
SMG(1,distance_alarm%10);
SMG(2,(distance_alarm%100)/10);
SMG(3,(distance_alarm%1000)/100);
SMG(4,11);
SMG(5,11);
SMG(6,10);
SMG(7,10);
SMG(8,10);
}
if(smg_mode==2)
{
if(time_mode==0)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
SMG(3,11);
SMG(4,time[1]%16);
SMG(5,time[1]/16);
SMG(6,11);
if(shanshuo==1)
{
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
else
{
SMG(7,10);
SMG(8,10);
}
}
if(time_mode==1)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
SMG(3,11);
if(shanshuo==1)
{
SMG(4,time[1]%16);
SMG(5,time[1]/16);
}
else
{
SMG(4,10);
SMG(5,10);
}
SMG(6,11);
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
if(time_mode==2)
{
if(shanshuo==1)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
}
else
{
SMG(1,10);
SMG(1,10);
}
SMG(3,11);
SMG(4,time[1]%16);
SMG(5,time[1]/16);
SMG(6,11);
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
}
}
//====================================
//====================================waring
int led=0xff;
void work()
{
if(distance<distance_alarm*1.2&&shanshuo==1)
{
led=(led&0xfe)|0x00;
choose_573(4);
P0=led;
choose_573(0);
}
else
{
led=(led&0xfe)|0x01;
choose_573(4);
P0=led;
choose_573(0);
}
}
//====================================
void main()
{
init_system();
set_time();
Timer0Init();
while(1)
{
get_distance();
read_time();
smg_display();
key_board();
work();
}
}
3届
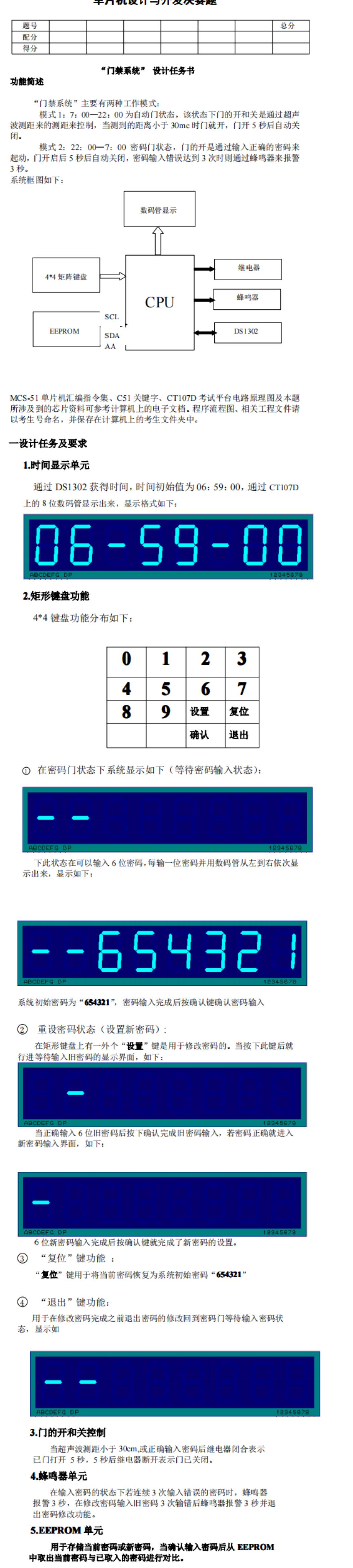
#include"reg52.h"
#include"intrins.h"
#include"ds1302.h"
#include"iic.h"
sfr P4=0xC0;
sbit R1=P3^0;
sbit R2=P3^1;
sbit R3=P3^2;
sbit R4=P3^3;
sbit C4=P3^4;
sbit C3=P3^5;
sbit C2=P4^2;
sbit C1=P4^4;
sbit TX=P1^0;
sbit RX=P1^1;
sfr AUXR=0x8e;
unsigned int code write_add[]={0x80,0x82,0x84,0x86,0x88,0x8a,0x8c};
unsigned int code read_add[]={0x81,0x83,0x85,0x87,0x89,0x8b,0x8d};
unsigned int code xianshi[]={0xc0,0xf9,0xa4,0xb0,0x99,0x92,0x82,0xf8,0x80,0x90,0xff,0xbf};
unsigned int time[]={0x00,0x59,0x06};
int mima_wei=0;
int mima[]={6,5,4,3,2,1};
int suru_mima[]={0,0,0,0,0,0};
int new_mima[]={0,0,0,0,0,0};
int smg_mode=1;
int door=0;
int distance=0;
int sure=0;
int error_time=0;
int alarm=0;
int control=0x00;
int temp1=654321;
void choose_573(int n)
{
switch(n)
{
case(0):P2=(P2&0x1f)|0x00;break;
case(4):P2=(P2&0x1f)|0x80;break;
case(5):P2=(P2&0x1f)|0xa0;break;
case(6):P2=(P2&0x1f)|0xc0;break;
case(7):P2=(P2&0x1f)|0xe0;break;
}
}
void init_system()
{
choose_573(4);
P0=0xff;
choose_573(5);
P0=0x00;
choose_573(0);
P0=0xff;
}
//=================================ds1302
void write_time()
{
int i;
Write_Ds1302_Byte(0x8e,0x00);
for(i=0;i<3;i++)
{
Write_Ds1302_Byte(write_add[i],time[i]);
}
Write_Ds1302_Byte(0x8e,0x80);
}
void read_time()
{
int i;
for(i=0;i<3;i++)
{
time[i]=Read_Ds1302_Byte(read_add[i]);
}
}
//=================================
//=================================keyboard
void Delay10ms() //@11.0592MHz
{
unsigned char i, j;
i = 108;
j = 145;
do
{
while (--j);
} while (--i);
}
void key_board()
{
R1=0;R2=R3=R4=1;
C1=C2=C3=C4=1;
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=0;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=0;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C1);
}
if(C2==0)
{
Delay10ms();
if(C2==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=1;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=1;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C2);
}
if(C3==0)
{
Delay10ms();
if(C3==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=2;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=2;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C3);
}
if(C4==0)
{
Delay10ms();
if(C4==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=3;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=3;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C4);
}
R2=0;R1=R3=R4=1;
C1=C2=C3=C4=1;
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=4;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=4;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C1);
}
if(C2==0)
{
Delay10ms();
if(C2==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=5;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=5;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C2);
}
if(C3==0)
{
Delay10ms();
if(C3==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=6;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=6;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C3);
}
if(C4==0)
{
Delay10ms();
if(C4==0)
{
Delay10ms();
if(smg_mode==1)
{
suru_mima[mima_wei]=7;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=7;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C4);
}
R3=0;R1=R2=R4=1;
C1=C2=C3=C4=1;
if(C1==0)
{
Delay10ms();
if(C1==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=8;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=8;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C1);
}
if(C2==0)
{
Delay10ms();
if(C2==0)
{
if(smg_mode==1)
{
suru_mima[mima_wei]=9;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
if(smg_mode==2)
{
new_mima[mima_wei]=9;
mima_wei++;
if(mima_wei==8)
{
mima_wei=0;
}
}
}
while(!C2);
}
//setting
if(C3==0)
{
int i;
Delay10ms();
if(C3==0)
{
smg_mode=2;
mima_wei=0;
for(i=0;i<6;i++)
{
suru_mima[i]=0;
new_mima[i]=0;
}
}
while(!C3);
}
//restart
if(C4==0)
{
Delay10ms();
if(C4==0)
{
mima[0]=6;mima[1]=5;mima[2]=4;mima[3]=3;mima[4]=2;mima[5]=1;
mima_wei=0;
smg_mode=1;
}
while(!C4);
}
R4=0;R1=R2=R3=1;
C1=C2=C3=C4=1;
//sure
if(C3==0)
{
Delay10ms();
if(C3==0)
{
if(smg_mode==1)
{
int i;int temp=0;
for(i=0;i<6;i++)
{
if(suru_mima[i]!=mima[i])
{
temp=1;
}
}
for(i=0;i<6;i++)
{
suru_mima[i]=0;
}
if(temp==0)
{
door=1;
error_time=0;
}
else
{
door=0;
temp=0;
error_time++; //alarm
}
if(error_time>=3) //alarm
{
alarm=1;
error_time=0;
}
mima_wei=0;
}
if(smg_mode==2)
{
int i=0;
for(i=0;i<6;i++)
{
mima[i]=new_mima[i];
}
sure=1;
mima_wei=0;
temp1=new_mima[5]+new_mima[4]*10+new_mima[3]*100+new_mima[2]*1000+new_mima[1]*10000+new_mima[0]*100000;
write_EEPROM(0x01,temp1);
}
}
while(!C3);
}
//quit
if(C4==0&&sure==1)
{
int i;
Delay10ms();
if(C4==0&&sure==1)
{
sure=0;
smg_mode=1;
for(i=0;i<6;i++)
{
new_mima[i]=0;
}
}
while(!C4);
}
}
//=================================
//=================================distance
void Delay12us() //@11.0592MHz
{
unsigned char i;
_nop_();
_nop_();
_nop_();
i = 30;
while (--i);
}
void send_wave()
{
int i;
for(i=0;i<8;i++)
{
TX=1;
Delay12us();
TX=0;
Delay12us();
}
}
void get_distance()
{
int time;
AUXR |= 0x40; //定时器时钟1T模式
TMOD &= 0x0F; //设置定时器模式
TL1 = 0x00; //设置定时初始值
TH1 = 0x28; //设置定时初始值
TF1 = 0; //清除TF1标志
TR1 = 0; //
TL1=0x00;
TH1=0x00;
TR1=1;
send_wave();
while(RX==1&&TF1==0);
TR1=0;
if(TF1==0)
{
time=TH1;
time=(time<<8)|TL1;
distance=time*0.017;
}
}
void distance_door()
{
if(smg_mode==0)
{
get_distance();
if(distance<=30)
{
door=1;
}
}
}
//=================================
//=================================smg_mode
void setting_smg()
{
if((time[2]%16+(time[2]/16)*10)>=7&&(time[2]%16+(time[2]/16)*10)<=21)
{
smg_mode=0;
}
// else if((time[2]%16+(time[2]/16)*10)<7&&(time[2]%16+(time[2]/16)*10)>21&&smg_mode==2)
// {
// smg_mode=2;
// }
// else
// {
// smg_mode=1;
// }
}
//=================================
//=================================smg
void Delay400us() //@11.0592MHz
{
unsigned char i, j;
i = 5;
j = 74;
do
{
while (--j);
} while (--i);
}
void SMG(int wei,int dat)
{
choose_573(6);
P0=0x80>>(wei-1);
choose_573(7);
P0=xianshi[dat];
choose_573(0);
Delay400us();
}
void smg_display()
{
if(smg_mode==0)
{
SMG(1,time[0]%16);
SMG(2,time[0]/16);
SMG(3,11);
SMG(4,time[1]%16);
SMG(5,time[1]/16);
SMG(6,11);
SMG(7,time[2]%16);
SMG(8,time[2]/16);
}
if(smg_mode==1)
{
SMG(1,suru_mima[5]);
SMG(2,suru_mima[4]);
SMG(3,suru_mima[3]);
SMG(4,suru_mima[2]);
SMG(5,suru_mima[1]);
SMG(6,suru_mima[0]);
SMG(7,11);
SMG(8,11);
}
if(smg_mode==2&&sure==0)
{
SMG(1,new_mima[5]);
SMG(2,new_mima[4]);
SMG(3,new_mima[3]);
SMG(4,new_mima[2]);
SMG(5,new_mima[1]);
SMG(6,new_mima[0]);
SMG(7,11);
SMG(8,10);
}
if(sure==1&&smg_mode==2)
{
SMG(1,10);
SMG(2,10);
SMG(3,10);
SMG(4,10);
SMG(5,10);
SMG(6,10);
SMG(7,10);
SMG(8,11);
}
}
//=================================
//=================================door and alarm
int door_control_close=0x00;
int door_control_open=0x10;
void door_work()
{
if(door==1)
{
control=(control&0xef)|0x10;
choose_573(5);
P0=control;
choose_573(0);
P0=0xff;
}
if(door==0)
{
control=(control&0xef)|0x00;
choose_573(5);
P0=control;
choose_573(0);
P0=0xff;
}
}
int alarm_control_open=0x40;
int alarm_control_close=0x00;
void alarm_work()
{
if(alarm==1)
{
control=(control&0xbf)|0x40;
choose_573(5);
P0=control; //open alarm
choose_573(0);
P0=0xff;
}
if(alarm==0)
{
control=(control&0xbf)|0x00;
choose_573(5);
P0=control; //close alarm
choose_573(0);
P0=0xff;
}
}
void Timer0Init(void) //5毫秒@11.0592MHz
{
AUXR |= 0x80; //定时器时钟1T模式
TMOD &= 0xF0; //设置定时器模式
TL0 = 0x00; //设置定时初始值
TH0 = 0x28; //设置定时初始值
TF0 = 0; //清除TF0标志
TR0 = 1; //定时器0开始计时
ET0=1;
EA=1;
}
int count_1=0;int count_2=0;
void Time0_server() interrupt 1
{
if(door==1)
{
count_1++;
if(count_1>=1000)
{
door=0;
count_1=0;
}
}
if(alarm==1)
{
count_2++;
if(count_2>=600)
{
count_2=0;
alarm=0;
}
}
}
//=================================
void read()
{
temp1=read_EEPROM(0x01);
mima[0]=temp1/100000;
mima[1]=(temp1%100000)/10000;
mima[2]=(temp1%10000)/1000;
mima[3]=(temp1%1000)/100;
mima[4]=(temp1%100)/10;
mima[5]=(temp1%10)/1;
}
void main()
{
init_system();
write_time();
Timer0Init();
read();
while(1)
{
key_board();
get_distance();
distance_door();
read_time();
smg_display();
setting_smg();
door_work();
alarm_work();
}
}