string.h
- strlen()
作用:返回字符数组的长度
错误例子:
#include <stdio.h>
#include <string.h>
#include <math.h>
int main(int argc, char const *argv[])
{
char ch[20];
ch[0] = '1', ch[1] = '1', ch[2] = '1';
printf("strlen(ch) is %d\n",strlen(ch));
return 0;
}
结果显示为:
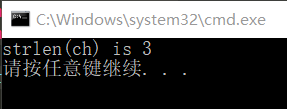
int main(int argc, char const *argv[])
{
char ch[20];
ch[0] = '1', ch[1] = '1', ch[2] = '1', ch[3] = '1';
printf("strlen(ch) is %d\n",strlen(ch));
return 0;
}
显示结果:
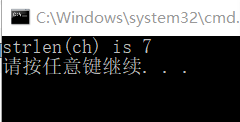
答案:
strlen所作的仅仅是一个计数器的工作,它从内存的某个位置(可以是字符串开头,中间某个位置,甚至是某个不确定的内存区域)开始扫描,直到碰到第一个字符串结束符’\0’为止,然后返回计数器值(长度不包含’\0’)。
而我们的数组ch并没有进行初始化,因此就可能会出现这样的问题。因此我们把代码进行修改:
int main(int argc, char const *argv[])
{
char ch[20];
ch[0] = '1', ch[1] = '1', ch[2] = '1', ch[3] = '1', ch[4] = '\0';
printf("strlen(ch) is %d\n",strlen(ch));
return 0;
}
运行结果:
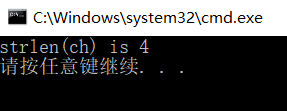
math.h/cmath:
- pow()
作用:求指数幂
错误例子:
#include <stdio.h>
#include <math.h>
int main(int argc, char const *argv[])
{
for (int i = 0; i < 5; i++)
{
int x = pow(10, i);
printf("base is 10 expon is %d and result is %d\n", i, x);
}
return 0;
}
显示结果:
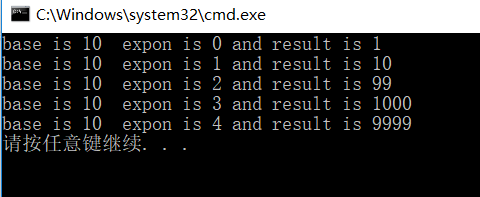
double pow (double base , double exponent);
float pow (float base , float exponent);
long double pow (long double base, long double exponent);
double pow (double base , int exponent);
long double pow (long double base, int exponent);
可以看出,在C++98中,不论是输出还是输入,底数和返回值都不存在int类型(虽然在C++11中出现了重载选项,没试过不清楚),所以在用pow函数的时候,即使是对整数为底求整数次幂,也建议大家都用double类型。
修改一下,正确写法之一为下:
int main(int argc, char const *argv[])
{
for (double i = 0; i < 5; i++)
{
double x = pow(10.0, i);
printf("base is 10 expon is %lf and result is %lf\n", i, x);
}
return 0;
}