# coding: utf-8
# # TensorFlow Tutorial
#
# Welcome to this week's programming assignment. Until now, you've always used numpy to
# build neural networks. Now we will step you through a deep learning framework that
# will allow you to build neural networks more easily. Machine learning frameworks like
# TensorFlow,PaddlePaddle, Torch, Caffe, Keras, and many others can speed up your machine
# learning development significantly. All of these frameworks also have a lot of
# documentation, which you should feel free to read. In this assignment, you will learn
# to do the following in TensorFlow:
#
# - Initialize variables
# - Start your own session
# - Train algorithms
# - Implement a Neural Network
#
# Programing frameworks can not only shorten your coding time, but sometimes also perform
# optimizations that speed up your code.
# ## 1 - Exploring the Tensorflow Library
# To start, you will import the library
# In[1]:# 调入Tensorflow的库
import scipy
from PIL import Image
from scipy import ndimage
import math
import numpy as np
import h5py
import os
import matplotlib.pyplot as plt
import tensorflow as tf
from tensorflow.python.framework import ops
from tf_utils import load_dataset, random_mini_batches, convert_to_one_hot, predict
#get_ipython().magic('matplotlib inline')
np.random.seed(1)
# Now that you have imported the library, we will walk you through its different applications.
# You will start with an example, where we compute for you the loss of one training example.
# loss = L(y^, y) = (y^(i) - y(i))^2
# In[2]:完成loss函数的测试
y_hat = tf.constant(36, name='y_hat') # Define y_hat constant. Set to 36.
y = tf.constant(39, name='y') # Define y. Set to 39
#设置利用GPU进行测试的环境
# os.environ["CUDA_VISIBLE_DEVICES"] = '1' #use GPU with ID=0
# config = tf.ConfigProto()
# config.gpu_options.per_process_gpu_memory_fraction = 0.5 # maximun alloc gpu50% of MEM
# config.gpu_options.allow_growth = True #allocate dynamically
loss = tf.Variable((y - y_hat)**2, name='loss') # Create a variable for the loss
#sess = tf.Session(config = config)
sess = tf.Session()
init = tf.global_variables_initializer() # When init is run later (session.run(init)),
# the loss variable will be initialized and ready to be computed
with tf.Session() as session: # Create a session and print the output
session.run(init) # Initializes the variables
print("session.run(loss):",session.run(loss)) # Prints the loss
# Writing and running programs in TensorFlow has the following steps:
#
# 1. Create Tensors (variables) that are not yet executed/evaluated.
# 2. Write operations between those Tensors.
# 3. Initialize your Tensors.
# 4. Create a Session.
# 5. Run the Session. This will run the operations you'd written above.
#
# Therefore, when we created a variable for the loss, we simply defined the
# loss as a function of other quantities, but did not evaluate its value. To evaluate it,
# we had to run `init=tf.global_variables_initializer()`. That initialized the
# loss variable, and in the last line we were finally able to evaluate the value of `loss`
# and print its value.
#
# Now let us look at an easy example. Run the cell below:
# In[3]:用简单的方法无法显示这个结果
a = tf.constant(2)
b = tf.constant(10)
c = tf.multiply(a,b)
print("c:",c)
#c: Tensor("Mul:0", shape=(), dtype=int32)
# As expected, you will not see 20! You got a tensor saying that the result is a
# tensor that does not have the shape attribute, and is of type "int32". All you
# did was put in the 'computation graph', but you have not run this computation yet.
# In order to actually multiply the two numbers, you will have to create a session and run it.
# In[4]:利用sess来运行结果
sess = tf.Session()
print("sess.run(c):",sess.run(c))
#sess.run(c): 20
# Great! To summarize, **remember to initialize your variables, create a session and
# run the operations inside the session**.
#
# Next, you'll also have to know about placeholders. A placeholder is an object whose
# value you can specify only later.
# To specify values for a placeholder, you can pass in values by using a "feed dictionary"
# (`feed_dict` variable). Below, we created a placeholder for x. This allows us to pass in
# a number later when we run the session.
# In[5]:用feed_dict来改变某一个变量的值
# Change the value of x in the feed_dict
x = tf.placeholder(tf.int64, name = 'x')
print(sess.run(2 * x, feed_dict = {x: 3}))
sess.close()
# When you first defined `x` you did not have to specify a value for it. A placeholder is
#simply a variable that you will assign data to only later, when running the session.
# We say that you **feed data** to these placeholders when running the session.
#
# Here's what's happening: When you specify the operations needed for a computation, you are
# telling TensorFlow how to construct a computation graph. The computation graph can have some
# placeholders whose values you will specify only later. Finally, when you run the session,
# you are telling TensorFlow to execute the computation graph.
# ### 1.1 - Linear function
#
# Lets start this programming exercise by computing the following equation: Y = WX + b,
# where W and X are random matrices and b is a random vector.
#
# **Exercise**: Compute WX + b where W, X, and b are drawn from a random normal
# distribution. W is of shape (4, 3), X is (3,1) and b is (4,1). As an example, here is how
# you would define a constant X that has shape (3,1):
# X = tf.constant(np.random.randn(3,1), name = "X")
# ```
# You might find the following functions helpful:
# - tf.matmul(..., ...) to do a matrix multiplication
# - tf.add(..., ...) to do an addition
# - np.random.randn(...) to initialize randomly
# In[6]:利用线性函数输出值
# GRADED FUNCTION: linear_function
def linear_function():
"""
Implements a linear function:
Initializes W to be a random tensor of shape (4,3)
Initializes X to be a random tensor of shape (3,1)
Initializes b to be a random tensor of shape (4,1)
Returns:
result -- runs the session for Y = WX + b
"""
np.random.seed(1)
### START CODE HERE ### (4 lines of code)
W = tf.constant(np.random.randn(4, 3), name="W")
X = tf.constant(np.random.randn(3,1), name = "X")
b = tf.constant(np.random.randn(4,1), name = "b")
Y = tf.add(tf.matmul(W,X),b)
### END CODE HERE ###
# Create the session using tf.Session() and run it with sess.run(...) on the variable
# you want to calculate
### START CODE HERE ###
sess = tf.Session()
result = sess.run(Y)
### END CODE HERE ###
# close the session
sess.close()
return result
# In[7]:#输出线性函数结果
print( "result = " + str(linear
吴恩达深度学习第二课第三周作业:识别手势
最新推荐文章于 2023-02-17 16:41:17 发布
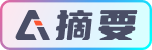