Peppa the Pig was walking and walked into the forest. What a strange coincidence! The forest has the shape of a rectangle, consisting of n rows and m columns. We enumerate the rows of the rectangle from top to bottom with numbers from 1 to n, and the columns — from left to right with numbers from 1 to m. Let's denote the cell at the intersection of the r-th row and the c-th column as (r, c).
Initially the pig stands in cell (1, 1), and in the end she wants to be in cell (n, m). Since the pig is in a hurry to get home, she can go from cell (r, c), only to either cell (r + 1, c) or (r, c + 1). She cannot leave the forest.
The forest, where the pig is, is very unusual. Some cells of the forest similar to each other, and some look very different. Peppa enjoys taking pictures and at every step she takes a picture of the cell where she is now. The path through the forest is considered to be beautiful if photographs taken on her way, can be viewed in both forward and in reverse order, showing the same sequence of photos. More formally, the line formed by the cells in order of visiting should be a palindrome (you can read a formal definition of a palindrome in the previous problem).
Count the number of beautiful paths from cell (1, 1) to cell (n, m). Since this number can be very large, determine the remainder after dividing it by 109 + 7.
The first line contains two integers n, m (1 ≤ n, m ≤ 500) — the height and width of the field.
Each of the following n lines contains m lowercase English letters identifying the types of cells of the forest. Identical cells are represented by identical letters, different cells are represented by different letters.
Print a single integer — the number of beautiful paths modulo 109 + 7.
3 4 aaab baaa abba
3
Picture illustrating possibilities for the sample test.
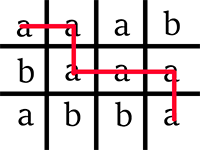
【题意】给定N*M的字符迷宫,要从(1,1)走到(n,m),只能向右和向下走,问路径形成回文串的路径有多少?
【解题方法】
//
考虑有2个人分别从(1,1)和(n,m)同时出发,在中间相遇
考虑状态f[x1][y1][x2][y2]:=第1个人到(x1,y1),第2个人到(x2,y2)符合条件的路径数
显然O(n4)会T,考虑一个常见优化,由于2个人走的步数是相同的,只要记录步数以及x坐标就可以算出对应的y坐标了
新的状态f[i][x1][x2]:=2个人走了i步,且第1个人的x坐标为x1,第2个人的为x2,的符合条件的路径数
转移就枚举,第1个人向右向下,第2个人向上向左,4种转移即可
统计答案的时候需要枚举相遇的点,显然ans+=∑ni=1f[step][i][i]
但是对于路径步数是偶数的,还可以ans+=∑ni=1f[step][i][i+1]
O(n3)的空间会炸,滚动数组优化一下就好了
【AC 代码】
//
//Created by just_sort 2016/9/12 16:50
//Copyright (c) 2016 just_sort.All Rights Reserved
//
#include <set>
#include <map>
#include <queue>
#include <stack>
#include <cmath>
#include <cstdio>
#include <cstring>
#include <iostream>
#include <algorithm>
using namespace std;
const int maxn = 505;
const int maxm = 505;
const int mod = 1e9+7;
char s[maxn][maxm];
int dp[2][maxn][maxm];
void add(int &x,int y){
if((x+=y)>=mod) x-=mod;
}
int main()
{
int n,m;
scanf("%d%d",&n,&m);
for(int i=1; i<=n; i++) scanf("%s",s[i]+1);
int t = 0;
memset(dp[t],0,sizeof(dp[t]));
dp[t][1][n] = s[n][m]==s[1][1];
int step = (n+m-2)/2;
for(int i=1; i<=step; i++){
memset(dp[!t],0,sizeof(dp[!t]));
for(int x1=1; x1<=i+1; x1++){
for(int x2=n; x2>=n-i; x2--){
int y1 = i+2-x1;
int y2 = n+m-i-x2;
if(s[x1][y1]!=s[x2][y2]) continue;
add(dp[!t][x1][x2],dp[t][x1][x2]);
add(dp[!t][x1][x2],dp[t][x1][x2+1]);
add(dp[!t][x1][x2],dp[t][x1-1][x2]);
add(dp[!t][x1][x2],dp[t][x1-1][x2+1]);
}
}
t = !t;
}
int ans = 0;
for(int i=1; i<=n; i++){
add(ans,dp[t][i][i]);
if(n+m & 1) add(ans,dp[t][i][i+1]);
}
printf("%d\n",ans);
return 0;
}