目录
创建tensor 可以直接使用python列表或者ndarray创建tensor
1.概念介绍
- 1.和tensorflow的tensor一样,它的张量也叫tensor
- tensor和numpy的ndarray一个意思,只不过tensor可以在GPU上加速计算
2.基本操作
创建tensor 可以直接使用python列表或者ndarray创建tensor
列表
torch.tensor([6,2],dtype = torch.int32)
注意,指定数据类型的方法和tensorflow不一样 tf.int32
元组
torch.tensor((6,2))
ndarray
torch.tensor(np.array([6,2]))
快速创建tensor的方法,和numpy的routines方法一样
ones,zeros,full,eye,random,normal,random.rand,random.random,arange
#创建一个0~1之间的随机数值组成的tensor
#2行3列
torch.rand(2,3)
torch.rand((2,3))
tensor的属性
- shape,size
可以通过shape或size获取tensor的形状, size可以具体制定获取哪一个维度的形状大小:
x = torch.ones(2,3,4)
print(x.shape)
#torch.Size([2,3,4])
#还可以通过size方法
x.size()
#size中可以传入某一维度,即shape的索引,从0开始
x.size(1)#3
tensor的基本数据类型
- 32位浮点型: torch.float32
- 64位浮点型: torch.float64
- 32位整型: torch.int32
- 16位整型: torch.int16
- 64位整型: torch.int64
- 可以在创建tensor的时候通过dtype指定数据类型:
x = torch.tensor([6, 2], dtype=torch.float32)
# 通过.type转换数据类型
x.type(torch.int64)
注意:tensorflow不能直接用tensor方法创建tensor;tensorflow提供constant常量,Variable变量
tensorflow更像自己的一个独立系统===TensorFlow中定义的数据叫做Tensor(张量), Tensor又分为常量和变量.
ndarray可以和tensor进行转换
from_numpy()
函数torch.from_numpy()
提供支持将numpy数组转换为PyTorch中的张量。它期望输入为numpy数组(numpy.ndarray)。输出类型为张量。返回的张量和ndarray共享相同的内存。返回的张量不可调整大小。当前它接受具有numpy.float64,numpy.float32,numpy.float16,numpy.int64,numpy.int32,numpy.int16,numpy.int8,numpy.uint8和numpy.bool的dtypes的ndarray。
参数:
ndarray:输入Numpy数组(numpy.ndarray)
返回类型:与x具有相同类型的张量。
pandas.DataFrame,pandas.Series与NumPy配列numpy.ndarray相互转换
- 获取具有DataFrame系列的值属性的ndarray
- 从NumPy数组ndarray生成DataFrame
- pandas.DataFrame和pandas.Series都可以获取带有值属性的NumPy数组numpy.ndarray。
从NumPy数组ndarray生成pandas.DataFrame,Series
可以在pandas.DataFrame和pandas.Series的构造函数的第一个参数数据中指定NumPy数组numpy.ndarray。
将numpy.ndarray传递给构造函数pandas.DataFrame()和pandas.Series()时,共享内存。更改一个值也会更改另一个值。
a = np.array([[1, 2, 3], [4, 5, 6]]) df_a = pd.DataFrame(a, columns=['a', 'b', 'c']) #如果不想共享内存,请使用numpy.ndarray的copy()将copy指定为构造函数的参数。 df_a_copy = pd.DataFrame(a.copy(), columns=['a', 'b', 'c'])
to_numpy()方法已添加到pandas.DataFrame和pandas.Series。返回numpy.ndarray,类似于上面的属性值。
df = pd.DataFrame(data=[[1, 2, 3], [4, 5, 6]], columns=['a', 'b', 'c']) print(df) # a b c # 0 1 2 3 # 1 4 5 6 a = df.to_numpy() print(a) # [[1 2 3] # [4 5 6]] print(type(a)) # <class 'numpy.ndarray'> ———————————————— 版权声明:本文为CSDN博主「饺子大人」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明。 原文链接:https://blog.csdn.net/qq_18351157/article/details/111433799
- numpy()
- 对比tensorflow的tensor和ndarray互相转化
Pandas.DataFrame是数字和字符串的组合,是对象类型ndarray。
t = tf.constant([[1., 2., 3.], [4., 5., 6.]])
输出:
tf.Tensor(
[[1. 2. 3.]
[4. 5. 6.]], shape=(2, 3), dtype=float32)
# .numpy()可以把tensor转化为ndarray
print(t.numpy())
np_t = np.array([[1., 2., 3.], [4., 5., 6.]])
# 直接使用ndarray生成一个tensor
print(tf.constant(np_t))
张量运算
- tensor的运算规则和numpy的运算规则很类似:
x = torch.ones(2, 3) # 和单个数字运算, tensor中每个元素分别和这个数字运算 x + 3 torch.add(x,3)
两个tensor相加,注意形状必须相同,element-wise,残差网络中是否有shortcut与主分支相加的操作
# 两个形状相同的tensor进行运算, 对应位置元素分别运算. x + x1 # 也可以调用pytorch的运算方法, 结果是一样的 x.add(x1)
注意以上相加的操作不改变原值,而是新生成一个tensor,跟tnesorflow操作常量一致
-
pytorch中带_的操作都会改变原值
# 加了下划线表示对x本来的值进行修改
x.add_(x1)
- 改变形状 shape() /view()都可以
# 改变tensor的形状, 使用.view, 相当于numpy中的reshape x.view(3, 2) x.view(-1, 1)
聚合运算
x.mean()
#求平均值
x.sum()
#求和
#指定维度,dim关键字,不指定时,默认会把所有维度聚合掉
x.sum(dim=1)
取出tensor的值
- 只有一个元素的tensor叫scalars(标量)(只有它才能用item方法),多个数据(用[]括起来)的tensor叫向量
# 单个元素的张量使用.item()转化为python数据
x = x.mean()
x.item()
# 输出一个数字
对比tensorflow
只有一个元素的tensor也叫scalars(标量)
取出常量的值 .numpy(),此时shape()输出为()
# scalar
t = tf.constant(2.718)
print(t.numpy())
print(t.shape)
'''
2.718
()
'''
-
如何取出多个值
跟numpy操作ndarray一样,可以进行索引和切片
索引:获取数组中特定位置元素的过程
切片:获取数组中特定片段元素的过程
VS tensorflow
t = tf.constant([[1., 2., 3.], [4., 5., 6.]])
# 可以像numpy的ndarray一样使用tensor
print(t)
print(t[:, 1:])
print(t[..., 1]) # 或t[:, 1]
练练手
输入CNN的图片数据shape
不要的维度写0,要的维度写:表示全部都要
矩阵操作 torch.matmul() 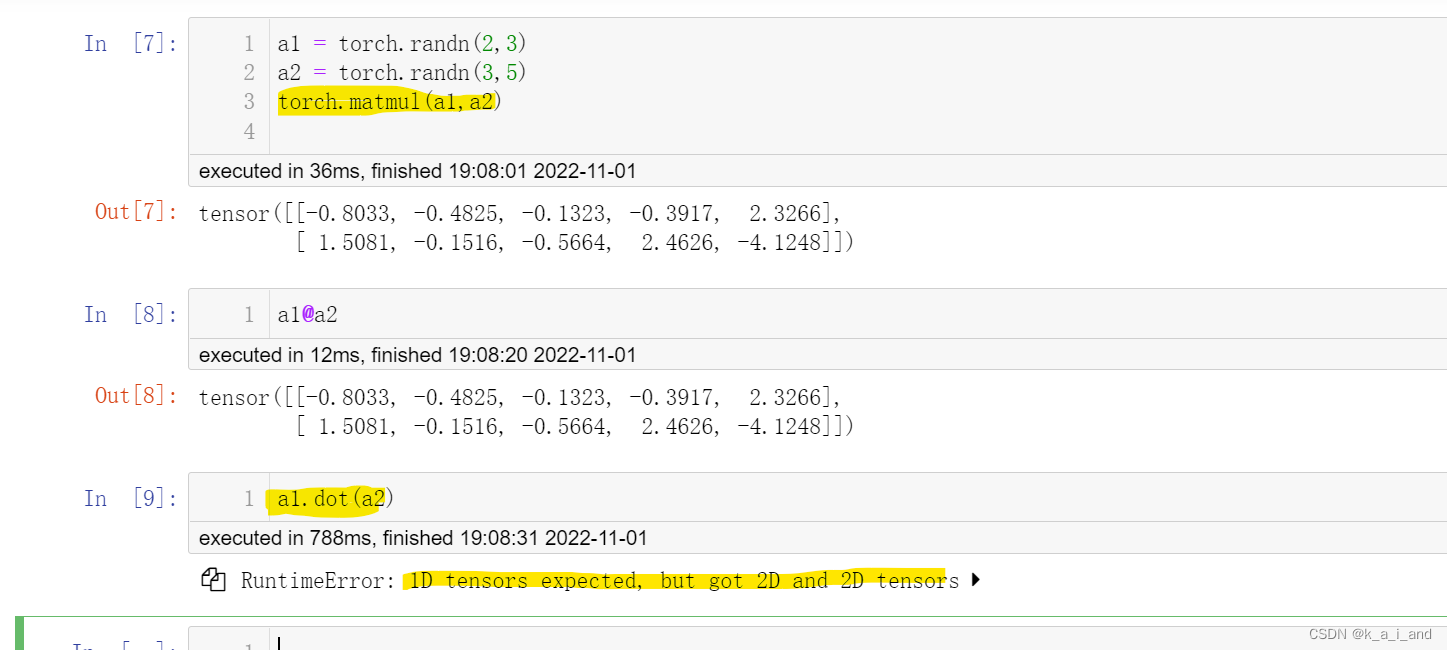
注意,pytorch中dot用于向量的乘法,且必须是一维向量