一、安装vue-baidu-map
npm install vue-baidu-map --save
二、全局注册(也可以局部注册,具体参考vue-baidu-map文档)
import Vue from 'vue'
import BaiduMap from 'vue-baidu-map'
Vue.use(BaiduMap, {
ak: 'YOUR_APP_KEY'
})
三、页面中使用组件
1.模板中使用组件
<baidu-map
class="map-wrap"
:center="mapData.center"
:zoom="mapData.zoom"
@ready="mapHandler"
@click="getLocation"
>
<bm-navigation anchor="BMAP_ANCHOR_TOP_LEFT"></bm-navigation>
<bm-city-list anchor="BMAP_ANCHOR_TOP_RIGHT"></bm-city-list>
<bm-geolocation
anchor="BMAP_ANCHOR_BOTTOM_RIGHT"
:showAddressBar="true"
:autoLocation="true"
></bm-geolocation>
</baidu-map>
2.定义数据
data() {
return {
businessDetail:{}
mapData: {
center: { lng: 0, lat: 0 },
zoom: 13
},
BMap: null,
map: null
}
},
3.方法事件处理
async mapHandler({ BMap, map }) {
if (this.businessId) {
await this.getMallBusinessDetail()
}
this.BMap = BMap
this.map = map
if (this.businessDetail.longitude && this.businessDetail.latitude) {
this.mapData.center.lng = this.businessDetail.longitude
this.mapData.center.lat = this.businessDetail.latitude
} else {
let geolocation = new BMap.Geolocation()
await new Promise((resolve) => {
geolocation.getCurrentPosition((r) => {
this.mapData.center.lng = this.businessDetail.longitude =
r.point.lng
this.mapData.center.lat = this.businessDetail.latitude = r.point.lat
resolve()
})
})
}
let marker = new BMap.Marker(
new BMap.Point(
this.businessDetail.longitude,
this.businessDetail.latitude
)
)
map.addOverlay(marker)
},
getLocation(e) {
this.businessDetail.longitude = e.point.lng
this.businessDetail.latitude = e.point.lat
let BMapGL = this.BMap
let map = this.map
map.clearOverlays()
let marker = new BMapGL.Marker(new BMapGL.Point(e.point.lng, e.point.lat))
map.addOverlay(marker)
let geoc = new BMapGL.Geocoder()
geoc.getLocation(e.point, (rs) => {
let addressComp = rs.addressComponents
this.businessDetail.address =
addressComp.province +
addressComp.city +
addressComp.district +
addressComp.street +
addressComp.streetNumber
})
},
四、效果
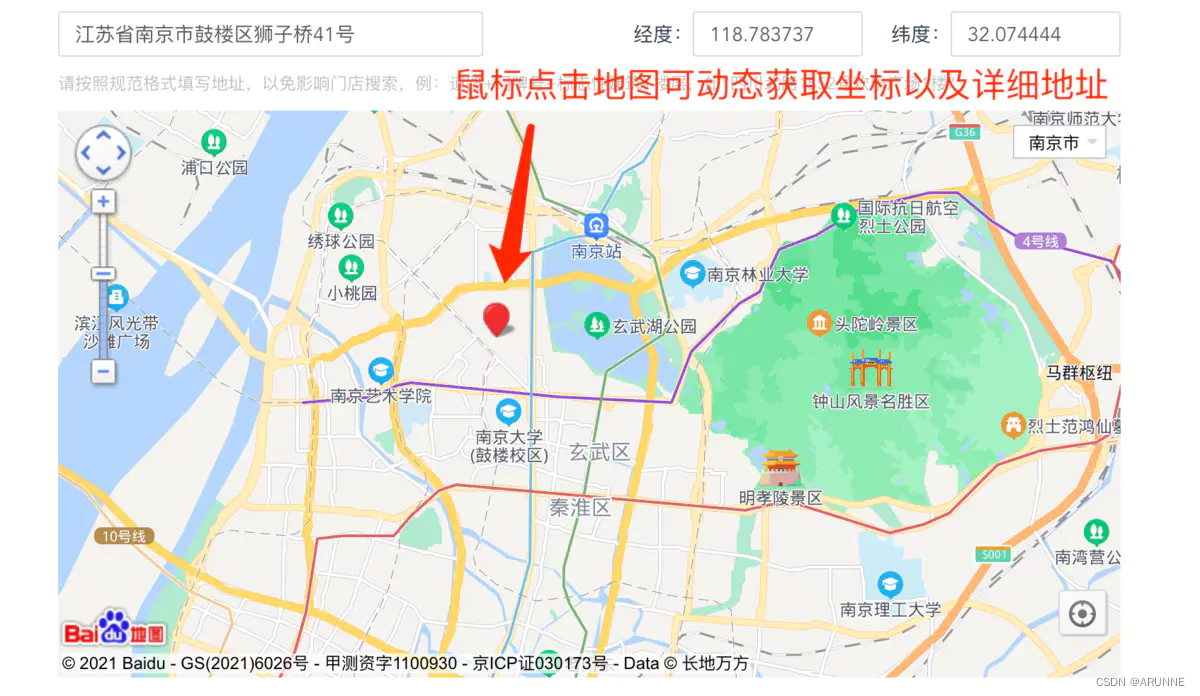