原理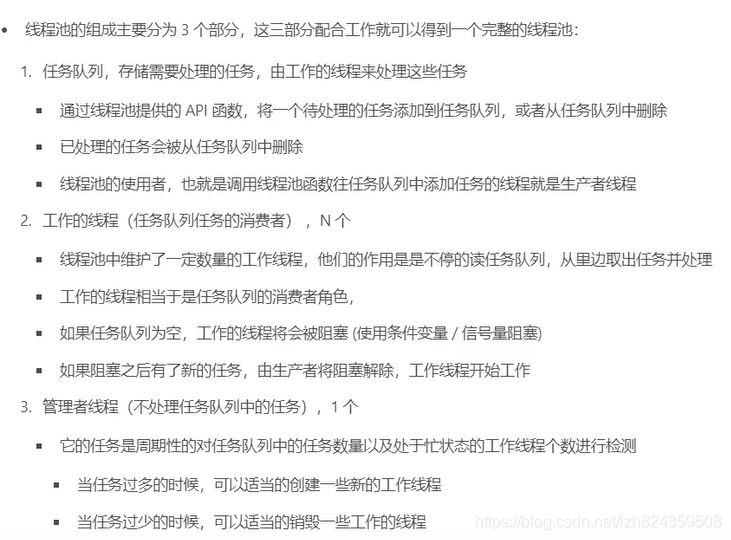
#include<pthread.h>
#include<stdio.h>
#include<stdlib.h>
#include<unistd.h>
#include<string.h>
//任务结构体
typedef struct Task{
void (*function)(void* arg);
void* arg;
}Task;
//线程池结构体
typedef struct ThreadPool{
//任务队列
Task* taskQ;
int queueCapacity;
int queueSize;
int queueFront;
int queueRear;
pthread_t managerID;//管理者线程
pthread_t *threadIDs;//工作的线程ID
int minNum,maxNum;//最大,最小线程数
int busyNum;//正在工作的线程
int liveNum;//存活的线程
int exitNum;//要销毁的线程个数
pthread_mutex_t mutexpool;//锁整个线程池
pthread_mutex_t mutexBusy;//锁busynum变量
pthread_cond_t notFull;//任务队列是否为满
pthread_cond_t notEmpty;//任务队列是否为空
int shutdown;//是否销毁线程池,是为1
}ThreadPool;
void threadExit(ThreadPool *pool){
pthread_t tid=pthread_self();
for(int i=0;i<pool->maxNum;++i){
if(pool->threadIDs[i]==tid){
pool->threadIDs[i]=0;
printf("threadExit() called, %ld exiting...\n",tid);
break;
}
}
pthread_exit(NULL);
}
void* worker(void* arg){
ThreadPool* pool=(ThreadPool*)arg;
while(1){
pthread_mutex_lock(&pool->mutexpool);
while(pool->queueSize==0&&!pool->shutdown){
//阻塞工作线程
pthread_cond_wait(&pool->notEmpty,&pool->mutexpool);
//判断是否要销毁线程
if(pool->exitNum>0){
pool->exitNum--;
if(pool->liveNum>pool->minNum){
pool->liveNum--;
pthread_mutex_unlock(&pool->mutexpool);
threadExit(pool);
}
}
}
//判断线程池是否关闭
if(pool->shutdown){
pthread_mutex_unlock(&pool->mutexpool);
threadExit(pool);
}
//从任务队列取一个任务
Task task;
task.function=pool->taskQ[pool->queueFront].function;
task.arg=pool->taskQ[pool->queueFront].arg;
//移动头结点(环形队列)
pool->queueFront=(pool->queueFront+1)%pool->queueCapacity;
pool->queueSize--;
//解锁
pthread_cond_signal(&pool->notFull);
pthread_mutex_unlock(&pool->mutexpool);
printf("thread %ld start working...\n",pthread_self());
pthread_mutex_lock(&pool->mutexBusy);
pool->busyNum++;
pthread_mutex_unlock(&pool->mutexBusy);
task.function(task.arg);
free(task.arg);
task.arg=NULL;
printf("thread %ld end working...\n",pthread_self());
pthread_mutex_lock(&pool->mutexBusy);
pool->busyNum--;
pthread_mutex_unlock(&pool->mutexBusy);
}
}
void* manager(void* arg){
ThreadPool* pool=(ThreadPool*)arg;
while(!pool->shutdown){
//每隔三秒检测一次
sleep(3);
//取出线程池中任务的数量和当前线程数量
pthread_mutex_lock(&pool->mutexpool);
int queueSize=pool->queueSize;
int liveNum=pool->liveNum;
pthread_mutex_unlock(&pool->mutexpool);
//取出忙线程数量
pthread_mutex_lock(&pool->mutexBusy);
int busyNum=pool->busyNum;
pthread_mutex_unlock(&pool->mutexBusy);
//添加线程
//任务个数>存活线程个数&&存活的个数<最大线程数
if(queueSize>liveNum&&liveNum<pool->maxNum){
int counter=0;
pthread_mutex_lock(&pool->mutexpool);
for(int i=0;i<pool->maxNum&&counter<2&&pool->liveNum<pool->maxNum;i++){
if(pool->threadIDs[i]==0){
pthread_create(&pool->threadIDs[i],NULL,worker,pool);
counter++;
pool->liveNum++;
}
}
pthread_mutex_unlock(&pool->mutexpool);
}
//销毁线程
//忙线程*2<存活的线程&&存活的线程>最小线程数
if(busyNum*2<liveNum&&liveNum<pool->minNum){
pthread_mutex_lock(&pool->mutexpool);
pool->exitNum=2;
pthread_mutex_unlock(&pool->mutexpool);
//让工作线程自杀
for(int i=0;i<2;i++){
pthread_cond_signal(&pool->notEmpty);
}
}
}
}
void threadPoolAdd(ThreadPool* pool, void(*func)(void*),void* arg){
pthread_mutex_lock(&pool->mutexpool);
while(pool->queueSize==pool->queueCapacity&&!pool->shutdown){
pthread_cond_wait(&pool->notFull,&pool->mutexpool);
}
if(pool->shutdown){
pthread_mutex_unlock(&pool->mutexpool);
return;
}
pool->taskQ[pool->queueRear].function=func;
pool->taskQ[pool->queueRear].arg=arg;
pool->queueRear=(pool->queueRear+1)%pool->queueCapacity;
pool->queueSize++;
pthread_cond_signal(&pool->notEmpty);
pthread_mutex_unlock(&pool->mutexpool);
}
int threadPoolBusyNum(ThreadPool* pool){
pthread_mutex_lock(&pool->mutexBusy);
int temp= pool->busyNum;
pthread_mutex_unlock(&pool->mutexBusy);
return temp;
}
int threadPoolliveNum(ThreadPool* pool){
pthread_mutex_lock(&pool->mutexpool);
int temp= pool->liveNum;
pthread_mutex_unlock(&pool->mutexpool);
return temp;
}
//销毁线程池
int threadPoolDestory(ThreadPool* pool){
if(pool==NULL) return -1;
//关闭线程池
pool->shutdown=1;
//阻塞回收管理者线程
pthread_join(pool->managerID,NULL);
//唤醒阻塞的消费者线程
for(int i=0;i<pool->liveNum;i++){
pthread_cond_signal(&pool->notEmpty);
}
if(pool->taskQ) free(pool->taskQ);
if(pool->threadIDs) free(pool->threadIDs);
free(pool);
pthread_mutex_destroy(&pool->mutexpool);
pthread_mutex_destroy(&pool->mutexBusy);
pthread_cond_destroy(&pool->notEmpty);
pthread_cond_destroy(&pool->notFull);
pool=NULL;
}
//创建线程池并初始化
ThreadPool* threadPoolCreate(int min, int max, int queueSize){
ThreadPool* pool=(ThreadPool*)malloc(sizeof(ThreadPool));
do{
if(pool==NULL){
printf("malloc threadpool fail");
break;
}
pool->threadIDs=(pthread_t*)malloc(sizeof(pthread_t)*max);
if(pool->threadIDs==NULL){
printf("malloc threadIDs fail");
break;
}
memset(pool->threadIDs,0,sizeof(pthread_t)*max);
pool->minNum=min;
pool->maxNum=max;
pool->busyNum=0;
pool->liveNum=min;
pool->exitNum=0;
if(pthread_mutex_init(&pool->mutexpool,NULL)!=0||pthread_mutex_init(&pool->mutexBusy,NULL)!=0||
pthread_cond_init(&pool->notEmpty,NULL)!=0||pthread_cond_init(&pool->notFull,NULL)!=0){
printf("init mutex failed");
break;
}
//任务队列
pool->taskQ=(Task*)malloc(sizeof(Task)*queueSize);
pool->queueCapacity=queueSize;
pool->queueSize=0;
pool->queueFront=0;
pool->queueRear=0;
pool->shutdown=0;
//创建管理者线程
pthread_create(&pool->managerID,NULL,manager,pool);
for(int i=0;i<min;++i){
pthread_create(&pool->threadIDs[i],NULL,worker,pool);
}
return pool;
}while(0);
//释放资源
if(pool&&pool->threadIDs) free(pool->threadIDs);
if(pool&&pool->taskQ) free(pool->taskQ);
if(pool) free(pool);
return NULL;
}
void taskFunc(void* arg){
int num=*(int*)arg;
printf("thread %ld is working ,number=%d\n",pthread_self(),num);
sleep(1);
}
int main(){
ThreadPool* pool = threadPoolCreate(3,10,100);
for(int i=0;i<100;i++){
int *num=(int*) malloc(sizeof(int));
*num=i+100;
threadPoolAdd(pool,taskFunc,num);
}
sleep(30);
threadPoolDestory(pool);
return 0;
}
输出结果:线程工作100次,主线程等待30秒后释放资源