文章目录
C++类和对象(二)
1.六个默认成员函数
如果一个类中什么成员都没有,简称为空类。空类中真的什么都没有吗?并不是,任何类在什么都不写时,编译器会自动生成以下6个默认成员函数。默认成员函数:用户没有显式实现,编译器会生成的成员函数称为默认成员函数。
六个默认成员函数:构造函数(初始化工作)、析构函数(清理工作)、拷贝构造(使用同类对象初始化创建对象)、赋值重载(把一个对象赋值给另外一个对象)、取地址重载(普通对象和const对象取地址)
2. 构造函数
2.1 背景
在我们写程序的时候,一般都是使用结构体来布局框架,一般使用结构体来布局的话就是malloc出来的空间,放在堆区,不易丢失数据,这样也少不了初始化这个操作,但是在实现过程中,我们很容易忘记调用初始化,这里C++就给了一个构造函数来解决忘记调用初始化的这个问题,也就是构造函数编译器会自动调用。
//实现一个栈
struct stack
{
int* _a;
int top;
int capacity;
};
void Init(stack* s) {
int* tmp = (int*)malloc(sizeof(int) * 4);
if (tmp == nullptr) {
exit(-1);
}
s->_a = tmp;
s->top = 0;
s->capacity = 4;
}
int main()
{
stack s;
Init(&s); //需要手动调用Init函数(很有可能忘记)
return 0;
}
2.2 特性
构造函数是特殊的成员函数,需要注意的是,构造函数虽然名称叫构造,但是构造函数的主要任
务并不是开空间创建对象,而是初始化对象。
特性
- 函数名与类名相同
- 无返回值
- 对象实例化时编译器自动调用对应的构造函数
- 构造函数可以重载
基本语法
class Date
{
public:
Date() { //无参构造函数 (支持重载,参数不同即可重载)(自动完成初始化)
}
Date(int year = 0, int month = 0, int day = 0) { //带参构造函数 (自动完成初始化)
_year = year;
_month = month;
_day = day;
}
private:
int _year = 0; //内置类型成员变量在类中声明时可以给默认值
int _month = 0;
int _day = 0;
};
void Test(){
Date d1; //可调用无参构造函数也可调用有参构造函数
Date d2(2023,2,4); //调用带参构造函数
Date d3(); //err(编译器认为是函数声明,编译器报错:未调用原型函数)
}
注意
-
内置类型成员变量在类中声明时可以给默认值
-
无参构造函数、全缺省构造函数、我们没写编译器默认生成的构造函数,都可以认为是默认构造函数
3. 析构函数
3.1 背景
上述构造函数背景中提到了用malloc去初始化,那么malloc需要手动释放空间,还给操作系统做管理,那么这里就需要销毁,但是我们写程序的时候很容易忘记释放空间,导致内存泄漏,这里析构函数就很容易解决这个问题。
//实现栈
struct stack
{
int* _a;
int _top;
int _capacity;
};
void Destroy(stack* s) {
if (s->_a != nullptr) {
free(s->_a);
s->_a = NULL;
s->_top = s->_capacity = 0;
}
}
int main()
{
stack s;
Destroy(&s); //需要手动调用Destroy函数(很有可能忘记)
return 0;
}
3.2 特性
析构函数:与构造函数功能相反,析构函数不是完成对对象本身的销毁,局部对象销毁工作是由
编译器完成的。而对象在销毁时会自动调用析构函数,完成对象中资源的清理工作。
特性
- 析构函数名是在类名前加上字符 ~
- 无参数无返回值类型
- 一个类只能有一个析构函数。若未显式定义,系统会自动生成默认的析构函数。注意:析构
函数不能重载 - 对象生命周期结束时,C++编译系统系统自动调用析构函数
基本语法
class Stack
{
public:
Stack(int size = 4) {
int* tmp = (int*)malloc(sizeof(int) * size);
if (tmp == nullptr) {
exit(-1);
}
_a = tmp;
_top = -1;
_capacity = size;
}
void Push(int x) {
_a[++_top] = x;
}
~Stack() { //析构函数
if (_a != nullptr) {
free(_a);
_a = nullptr;
_top = -1;
_capacity = 0;
}
}
private:
int* _a;
int _top;
int _capacity;
};
int main()
{
Stack d1(8);
d1.Push(1);
d1.Push(2);
d1.Push(3);
return 0; //程序结束时,自动调用析构函数
}
类自动生成默认成员函数解释
如果没有自己写默认构造函数,编译器会自动生成默认构造函数吗?会。补充:如果我们不写,编译器也会自动生成对应的默认成员函数,只是我们看到会给数据生成随机值而已,但是确实已经自动调用了默认成员函数,但是只要我们实现了,编译器就不会自动生成,而是用我们实现的对应的默认成员函数。
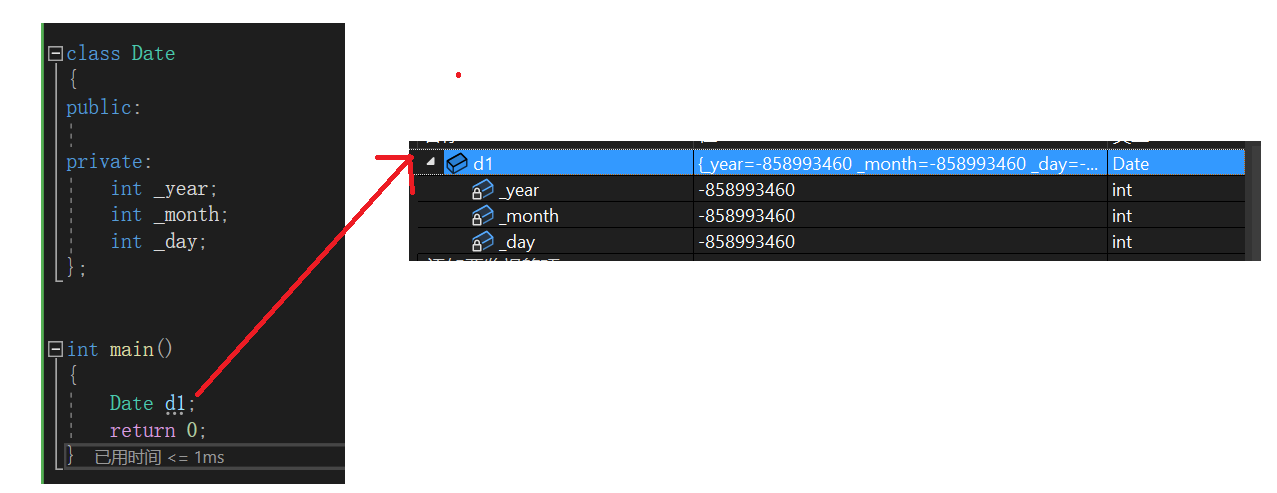
上面图片中默认生成的成员函数使得变量随机值,解释:C++把类型分成内置类型(比如:int/char/double/任意指针类型等等)和自定义类型(struct/class/enum/union等),默认生成构造函数或者析构函数都是内置类型成员不做处理(也就是随机值),对自定义类型的成员会去调用它的默认构造函数或者析构函数
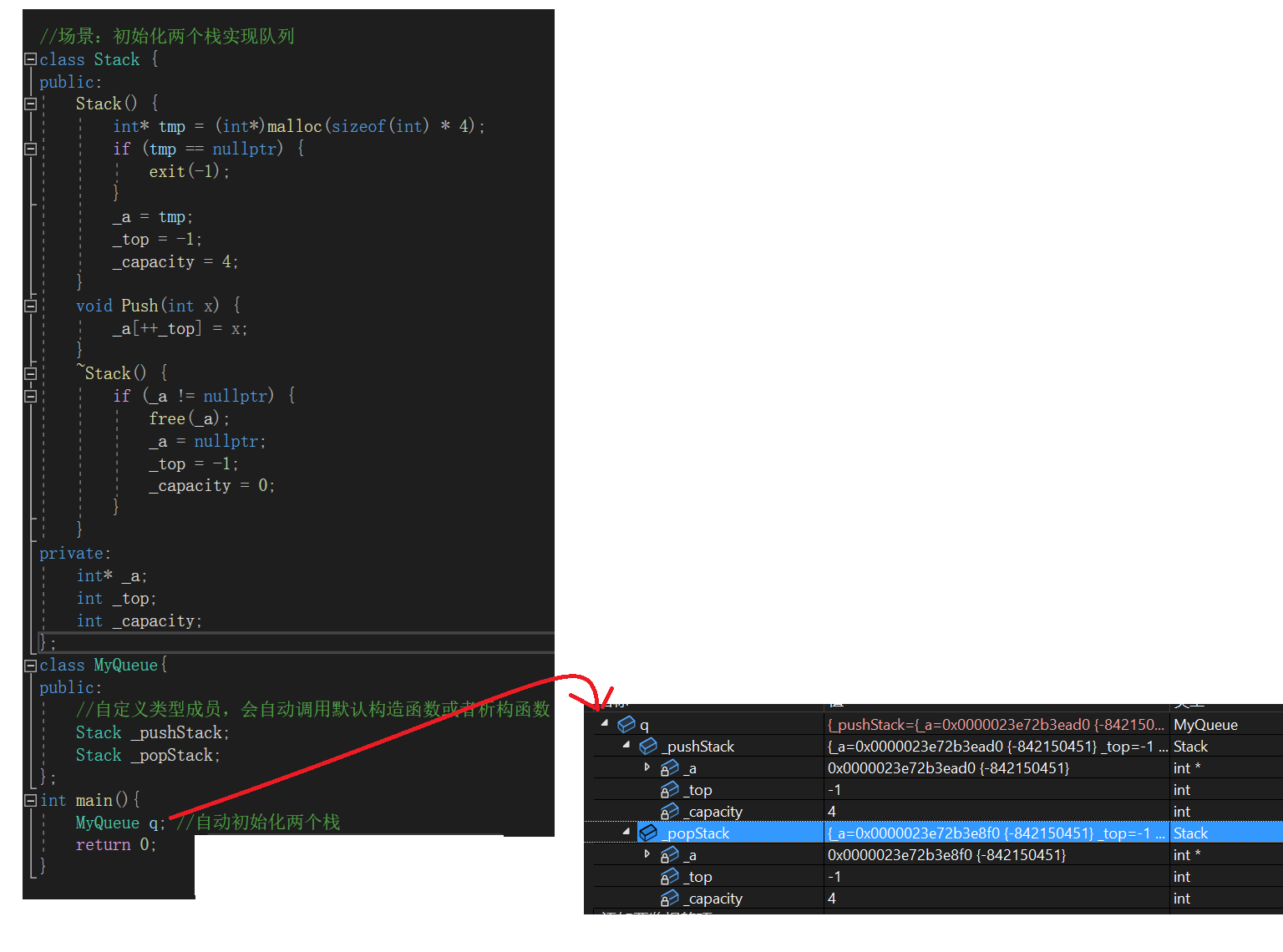
4. 拷贝构造函数
4.1 背景
假设,我创建了一个对象,然后进行构造并且赋值,此时我想有一个同样的对象,这里又要完成构造并赋值的操作会很麻烦,然后我们想直接拷贝,这里就用到了拷贝构造函数
4.2 特性
那在创建对象时,可否创建一个与已存在对象一某一样的新对象呢?拷贝构造函数:只有单个形参,该形参是对本类的类型对象的引用(一般常用const修饰),在用已存在的类的类型对象创建新对象时由编译器自动调用。
特性
-
拷贝构造函数是构造函数的一个重载形式
-
拷贝构造函数的参数只有一个且必须是类类型对象的引用,使用传值方式编译器直接报错,
因为会引发无穷递归调用 -
若未显式定义,编译器会生成默认的拷贝构造函数。 默认的拷贝构造函数对象按内存存储按
字节序完成拷贝,这种拷贝叫做浅拷贝,或者值拷贝 -
拷贝构造函数典型调用场景:
函数传值传参中或者函数传值返回中,有自定义类型就需要拷贝构造
基本语法
class Date
{
public:
Date(int year = 0, int month = 0, int day = 0) {
_year = year;
_month = month;
_day = day;
}
//拷贝构造函数
//构造函数的重载形式
Date(const Date& d) {
_year = d._year;
_month = d._year;
_day = d._day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2023, 2, 4);
Date d2(d1); //d2变成d1的拷贝
//另一种写法:Date d2 = d1;(拷贝构造函数)
return 0;
}
**问题:拷贝构造函数中的形参可以不使用引用吗?**不行。
原因:
- 先了解传值和传引用
//传值传参(拷贝)
void fun1(int x1) {
}
//传引用传参(别名,共用一块空间)
void fun2(int& x2) {
}
传值传参会在此函数栈帧中开辟形参空间,实参数据给到形参;传引用就相当于传地址。传值传参中,内置类型通常空间很小,可以用寄存器来进行拷贝操作,也就是先把实参的数据一个字节一个字节先拷贝到寄存器中,然后寄存器再一个字节一个字节拷贝到形参空间中,这种叫做浅拷贝,编译器也只能完成这种傻瓜式操作,但是自定义类型就不行,假设是下面场景:
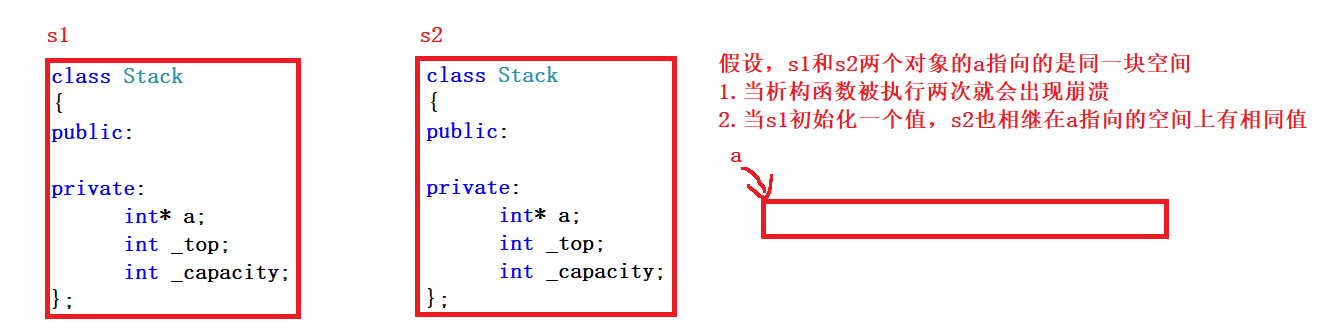
传值传参中,内置类型:编译器会浅拷贝;自定义类型:不能解决上述两个问题,就需要调用拷贝构造
- 自定义类型传值传参中,传参会调用拷贝构造,现象:
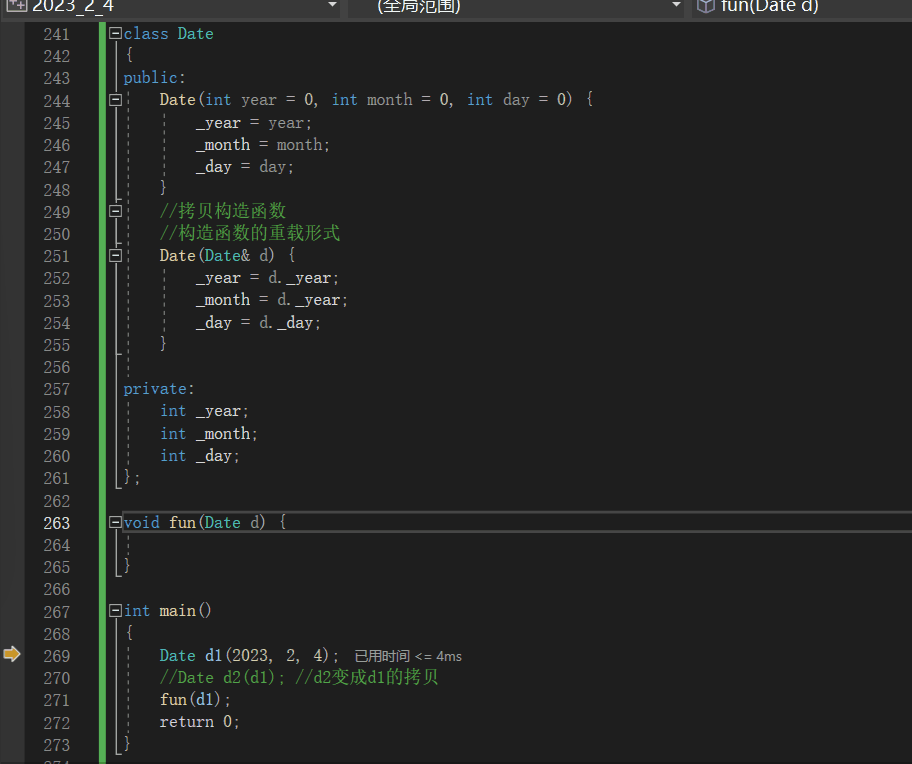
- 为什么拷贝构造函数中形参必须使用引用?
自定义类型传值传参中,传参会调用拷贝构造;那么当我们使用自定义类型传参,就会自动调用拷贝构造函数,此时我们的形参如果是Date d,那么这个形参是自定义类型就又需要调用拷贝构造函数,如从导致是个无穷递归调用
- 画图理解传值传参中,自定义类型调用拷贝构造
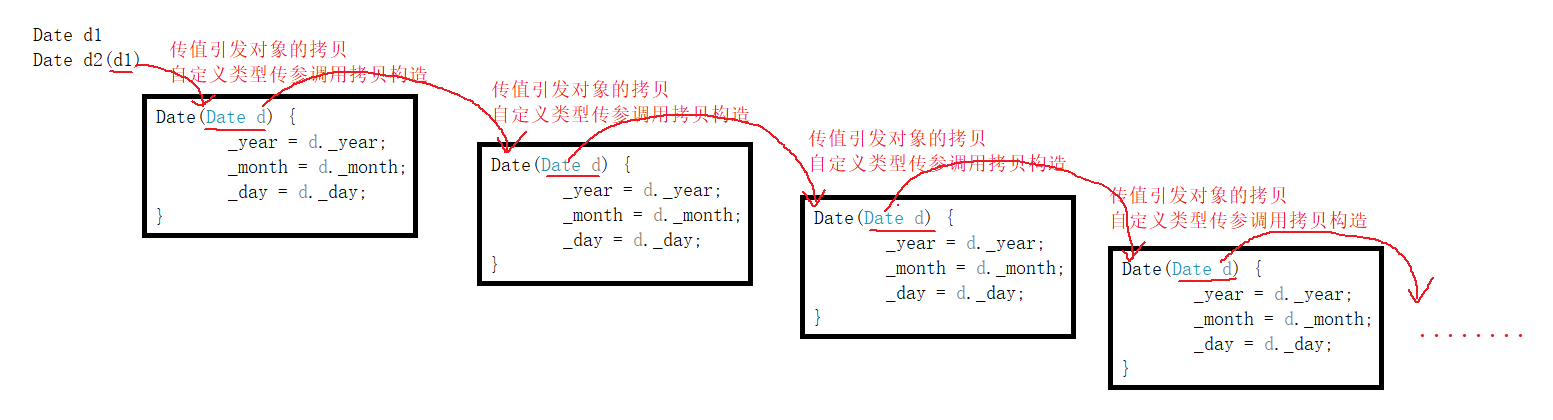
Date(const Date& d):这里d就是d1,传参就不需要拷贝构造,d和d1共用的同一块空间
**注意:类中如果没有涉及资源申请时,拷贝构造函数是否写都可以;一旦涉及到资源申请时,则拷贝构造函数是一定要写的,否则就是浅拷贝。**像上面的Stack类中int *a成员变量就需要malloc出空间。
- 拷贝构造函数只有单个形参,对本类的类型对象的引用(一般常用const修饰),在用已存在的类的类型对象创建新对象时由编译器自动调用。这里的单个形参中是引用那么可以用指针?是可以的,但是不方遍使用,也不叫作拷贝构造函数,因为拷贝构造函数是定义定死的。
4.3 使用场景
问题:怎么获取这个日期后的x天的日期?
class Date
{
public:
Date(int year = 0, int month = 0, int day = 0) { //构造函数初始化
_year = year;
_month = month;
_day = day;
}
Date(const Date& d) { //拷贝构造函数,使用存在对象初始化另外一个对象
_year = d._year;
_month = d._month;
_day = d._day;
}
int getMonthDay(int year, int month) {
assert(month > 0 && month < 13);
int monthArr[13] = { 0,31,28,31,30,31,30,31,31,30,31,30,31 };
if (month == 2 && ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0))) {
return 29;
}
else {
return monthArr[month];
}
}
//写法一:
//获取x天后的日期
//Date& getAfterDay(int x) {
// _day += x;
// while (_day > getMonthDay(_year, _month)){
// _day -= getMonthDay(_year, _month);
// ++_month;
// if (_month == 13) {
// _year++;
// _month = 1;
// }
// }
// return *this;
//}
//写法二:
Date getAfterDay(int x) {
Date tmp(*this);
tmp._day += x;
while (tmp._day > getMonthDay(tmp._year, tmp._month)) {
tmp._day -= getMonthDay(tmp._year, tmp._month);
++tmp._month;
if (tmp._month == 13) {
tmp._year++;
tmp._month = 1;
}
}
return tmp;
}
void print(){
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2023, 2, 3);
Date d2 = d1.getAfterDay(1000);
d1.print();
d2.print();
return 0;
}
上述程序中getAfterDay函数有两个写法,对比:
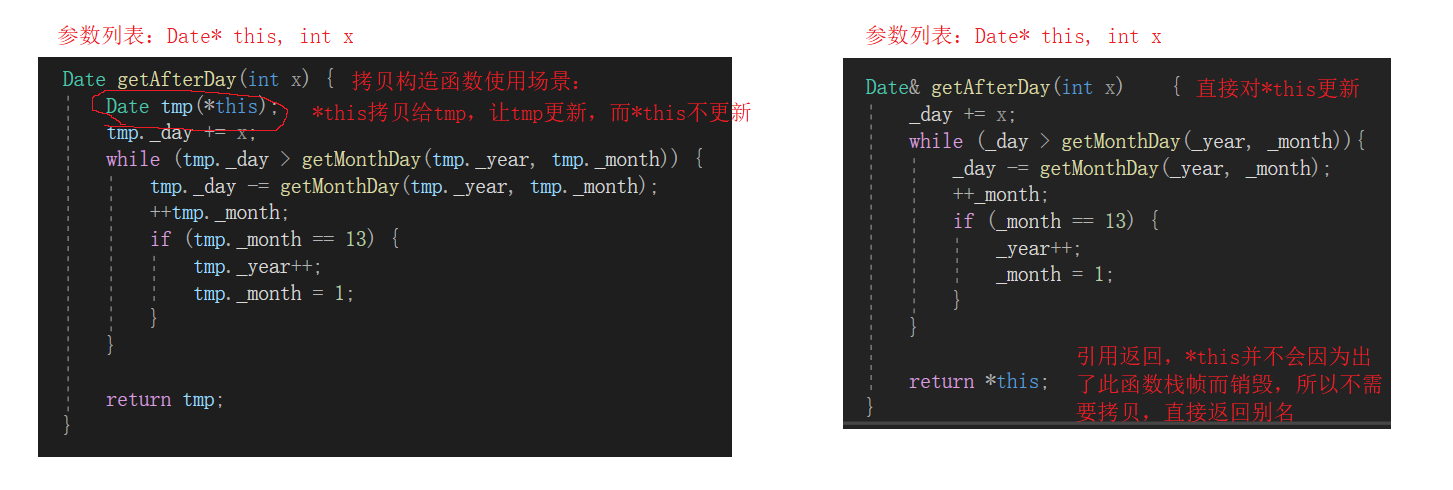
这里Date getAfterDay(int x)函数中,return tmp是返回的是tmp的拷贝,因为tmp是自定义类型的,所以需要调用拷贝构造,visual studio 2022这里做的处理是浅拷贝,直接放进rax寄存器中,visual studio 2013是调用的拷贝构造函数。这个也和编译器相关。
默认生成拷贝构造函数和赋值运算符重载总结
- 对于内置类型完成浅拷贝或者值拷贝,按一个字节一个字节的拷贝
- 对于自定义类型,调用拷贝构造或者赋值运算符重载
5. 运算符重载
5.1 背景
为什么需要运算符重载?
为了增强程序的可读性,自定义类型再进行符号运算的时候需要用到重载
class Date
{
public:
Date(int year = 0, int month = 0, int day = 0)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2023, 2, 4);
Date d2(2023, 3, 4);
//内置类型进行运算(ok)
int a = 10;
int b = 20;
bool ret = a == b ? true : false;
cout << ret << endl;
//自定义类型进行运算(err)
bool ret = d1 == d2 ? true : false;
cout << ret << endl;
return 0;
}
怎么解决上面自定义类型运算的问题呢?
class Date
{
public:
Date(int year = 0, int month = 0, int day = 0)
{
_year = year;
_month = month;
_day = day;
}
//d1.equal(d2)
bool equal(const Date& d) {
return _year == d._year
&& _month == d._month
&& _day == d._day;
}
private:
int _year;
int _month;
int _day;
};
比较日期是否相同
//bool equal(const Date& d1, const Date& d2) {
// return d1._year == d2._year
// && d1._month == d2._month
// && d1._day == d2._day;
//}
int main()
{
Date d1(2023, 2, 4);
Date d2(2023, 3, 4);
bool ret = d1.equal(d2);
cout << ret << endl;
return 0;
}
上述方法中解决了这个问题,但是d1.equal(d2)这种风格的代码不易阅读,可以改成d1 == d2 ? true : false这种吗?可以,需要用到重载
class Date
{
public:
Date(int year = 0, int month = 0, int day = 0)
{
_year = year;
_month = month;
_day = day;
}
//d1 == d2
bool operator==(const Date& d) { //运算符重载(*this:d1,d:d2)
return _year == d._year
&& _month == d._month
&& _day == d._day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2023, 2, 4);
Date d2(2023, 3, 4);
cout << (d1 == d2) << endl;
return 0;
}
5.2 特性
运算符重载就是具有特殊函数名的函数,这里需要用到operator关键字,这个关键字后面接需要重载的运算符号,函数原型:返回值+operator+操作符+(参数列表)
注意
- 不能通过连接其他符号(不是运算符的符号)来创建新的操作符:比如operator@
- 重载操作符必须有一个类的类型参数(因为是对自定义类型进行运算)
- 作为类成员函数重载时,其形参看起来比操作数数目少1,因为成员函数的第一个参数为隐藏的this
- (.*)、(:😃(域限定符)、(sizeof)、( ? : )(三目运算符)、(.)(成员访问运算符)这五个运算符不能重载
5.3 赋值运算符重载
class Date
{
public:
Date(int year = 0, int month = 0, int day = 0)
{
_year = year;
_month = month;
_day = day;
}
void out() {
cout << _year << "/" << _month << "/" << _day;
}
//Date d2 = d1;
//*this:d2 d:d1
void operator=(const Date& d) { //赋值重载
_year = d._year;
_month = d._month;
_day = d._day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2023, 2, 7);
Date d2 = d1;
d2.out();
return 0;
}
上面这种写法不适用连续赋值,改进:
class Date
{
public:
Date(int year = 0, int month = 0, int day = 0)
{
_year = year;
_month = month;
_day = day;
}
void out() {
cout << _year << "/" << _month << "/" << _day;
}
//Date d2 = d1;
//*this:d2 d:d1
Date& operator=(const Date& d) { //赋值重载 (带返回值是为了连续赋值)
if(this != &d){ //防止d1 = d1
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2023, 2, 7);
Date d2;
Date d3;
d3 = d2 = d1;
d3.out();
return 0;
}
拷贝构造和赋值重载形式上容易混淆:
Date d1(2023, 2, 7);
Date d2(d1); //拷贝构造
Date d2 = d1; //拷贝构造(一个没有实例化,那一个实例化的对象去实例化这个对象)
Date x1(2023, 3, 5);
Date x2
x2 = x1; //赋值重载(两个对象都是已经实例化的对象)
那么<<(流插入运算符)和>>(流提取运算符)是怎么实现的?
其实<<和>>符号是被重载的,库中只是对内置类型做处理,对自定义类型没有做处理
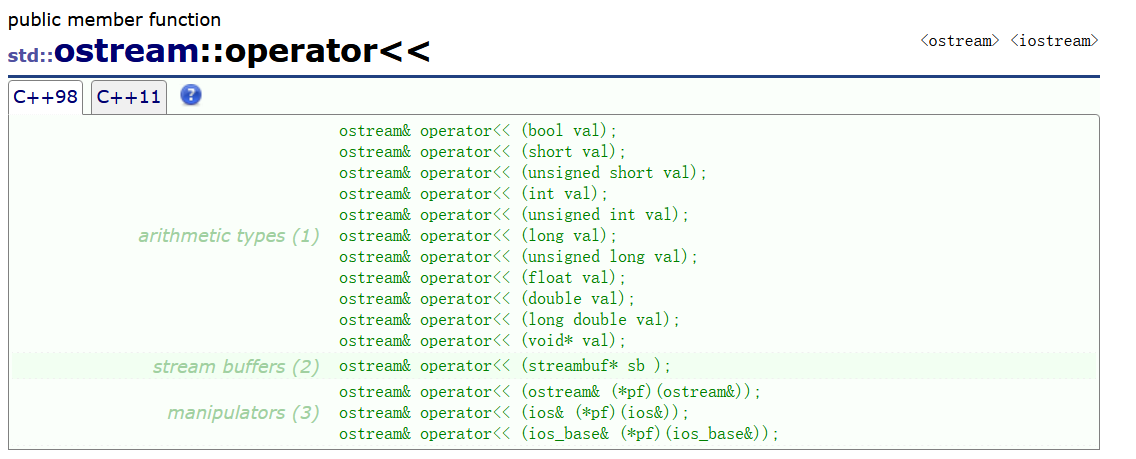
//自定义类型实现流插入和流提取
class Date
{
friend ostream& operator<<(ostream& out, const Date& d); //友元函数
friend istream& operator>>(istream& cin, Date& d);
public:
private:
int _year;
int _month;
int _day;
};
//Date d1(20232,2,7)
//cout << d1
ostream& operator<<(ostream& out, const Date& d) {
out << d._year << "/" << d._month << "/" << d._day << endl;
return out;
}
istream& operator>>(istream& cin, Date& d) {
cin >> d._year >> d._month >> d._day;
return cin;
}
6. const成员
6.1 背景
const修饰变量,如:const int a = 10; 那么这个a变量的权限只是只读,不可修改,所以const修饰后权限仅可读
// const修饰类
class Test
{
public:
void out() //this指针类型:Test*
{
cout << _a << endl;
}
private:
int _a = 10;
};
int main()
{
const Test t; //只读
t.out(); //成员函数调用,成员函数可读可修改,类型是cosnt Test*,因此err
return 0;
}
怎么解决上面的权限问题呢?那么就要用到const成员函数
6.2 特性
将const修饰的“成员函数”称之为const成员函数,const修饰类成员函数,实际修饰该成员函数隐含的this指针,表明在该成员函数中不能对类的任何成员进行修改。
class Test
{
public:
void out() const //(权限不变)
{
cout << _a << endl;
}
private:
int _a = 10;
};
int main()
{
const Test t; //只读
t.out();
return 0;
}
class A
{
void _A() {const //this指针类型: const A*
_a(); //void A::_a(void)”: 不能将“this”指针从“const A”转换为“A &”
}
void _a() { //this指针类型:A* (const A* -> A* 权限放大err)
}
private:
int _c;
};
- const对象不可调用非const成员函数
- 非const对象可调用const成员函数
- const成员函数不可调用其他非const成员函数
- 非const成员函数可调用其他const成员函数
7. 取地址及const取地址操作符重载
这两个默认成员函数一般不用重新定义 ,编译器默认会生成。只有特殊情况,才需要重载,比如想让别人获取到指定的内容!
class Date
{
public:
Date* operator&()
{
return nullptr;
}
const Date* operator&()const
{
return nullptr;
}
private:
int _year;
int _month;
int _day;
};