一、图书管理模块
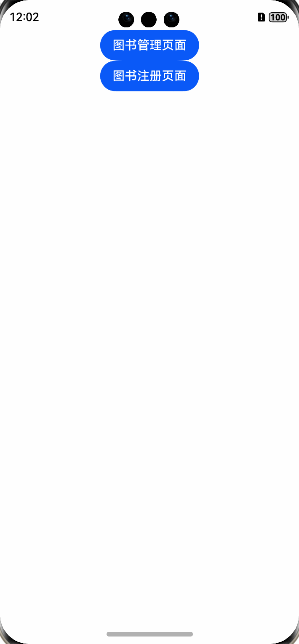
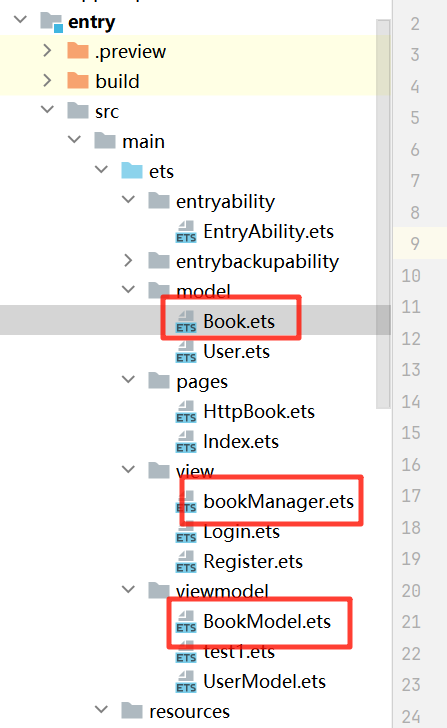
1、model
@Observed
export default class Book{
id?:number
title?:string
author?:string
publicationYear?:string
type?:string
constructor(id?:number,title?:string,author?:string,publicationYear?:string,type?:string) {
this.id=id
this.title=title
this.author=author
this.publicationYear=publicationYear
this.type=type
}
}
import { relationalStore, ValuesBucket } from "@kit.ArkData";
import Book from "../model/Book";
import { UIContext } from '@ohos.arkui.UIContext';
import { common } from '@kit.AbilityKit';
class BookModel {
private Rdb: relationalStore.RdbStore = {} as relationalStore.RdbStore
private RdbName: string = 'books'
// RdbMap:Map<string,relationalStore.RdbStore>=new Map()
// 1、创建实例,数据库实例需要在onWindowStage创建时,进行创建
initDB(context:common.UIAbilityContext) {
// 设置当前数据库配置信息
let Rdb_config: relationalStore.StoreConfig = {
name: this.RdbName,
securityLevel: relationalStore.SecurityLevel.S1,
}
const createBookSql = `CREATE TABLE IF NOT EXISTS books (
id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT,
author TEXT,
publicationYear TEXT,
type TEXT
)`
relationalStore.getRdbStore(context, Rdb_config, (err, data) => {
if (err) {
console.error('testTag', '获取Rdb失败!')
return
} else {
console.log('testTag', '获取Rdb成功')
this.Rdb = data
data.executeSql(createBookSql)
}
})
}
// 2、增加
addBook(title: string, author: string, publicationYear: string, type: string): number {
// 添加数据,返回id
try {
console.log('testTag','添加数据成功')
return this.Rdb.insertSync(this.RdbName, {
title,
author,
publicationYear,
type
})
}catch (e){
console.error('testTag','添加数据异常'+e)
return e
}
}
// 3、删除(根据Id进行删除)
deleteBookById(id: number) {
// 3.1 定义删除的条件
let predicate = new relationalStore.RdbPredicates(this.RdbName)
predicate.equalTo('id',id)
// 3.2 定义删除操作
this.Rdb.delete(predicate)
}
// 4、修改
updateBook(title: string, author: string, publicationYear: string, type: string) {
// 4.1 定义要更新的数据
// 不能定义Book类型,Book类型和ValuesBucket不兼容
let data: ValuesBucket = {
title: title,
author: author,
publicationYear: publicationYear,
type: type
}
// 4.2 定义更新的条件
let predicate = new relationalStore.RdbPredicates(this.RdbName)
predicate.equalTo('title', title)
predicate.equalTo('author', author)
predicate.equalTo('publicationYear', publicationYear)
predicate.equalTo('type', type)
// 4.3 更新
return this.Rdb.updateSync(data as ValuesBucket, predicate)
}
// 5、查询
// 5.1 根据title进行查询
queryBookById(title: string) :Book|null{
let predicate = new relationalStore.RdbPredicates(this.RdbName)
predicate.equalTo('title',title)
const resultSet=this.Rdb.querySync(predicate, ['id', 'title', 'author', 'publicationYear', 'type'])
if (resultSet.rowCount > 0) {
// 假设 resultSet[0] 是我们要的 Book 对象
return new Book(resultSet[0].id, resultSet[0].title, resultSet[0].author, resultSet[0].publicationYear,
resultSet[0].type)
}
return null; // 如果没有找到书,返回 null
}
// 5.2 查询所有
queryAllBooks() :Book[]{
let predicate = new relationalStore.RdbPredicates(this.RdbName)
let result = this.Rdb.querySync(predicate, ['id', 'title', 'author', 'publicationYear', 'type'])
let books: Book[] = []
// 遍历封装
while (!result.isAtLastRow) {
// 指针移动到下一行
result.goToNextRow()
let id = result.getLong(result.getColumnIndex('id'))
let title = result.getString(result.getColumnIndex('title'))
let author = result.getString(result.getColumnIndex('title'))
let publicationYear = result.getString(result.getColumnIndex('publicationYear'))
let type = result.getString(result.getColumnIndex('type'))
books.push(new Book(id,title,author,publicationYear,type))
}
console.log('testTag', '查询到所有数据', JSON.stringify(books))
return books
}
}
const bookModel=new BookModel()
export default bookModel as BookModel
3、view
import Book from '../model/Book'
import BookModel from "../viewmodel/BookModel"
import { common } from '@kit.AbilityKit'
@Entry
@Component
struct bookManager {
// context=getContext(this) as common.UIAbilityContext
@State books:Book[]=[]
controller:CustomDialogController=new CustomDialogController({builder:AddBook({books:this.books})})
change(){
this.books=BookModel.queryAllBooks()
}
async onPageShow() {
// BookModel.initDB(this.context)
this.books=BookModel.queryAllBooks()
}
build() {
Column() {
List({space:10}){
ForEach(this.books,(item:Book)=>{
ListItem(){
BookCard(item)
}.swipeAction({end:this.deleteBook(item.id)})
})
}.height('80%')
.width('100%')
.alignListItem(ListItemAlign.Center)
Button('获取图书')
.onClick(() => {
this.books=BookModel.queryAllBooks()
console.log('testTag',JSON.stringify(this.books))
})
Button('添加图书')
.onClick(() => {
this.controller.open()
console.log('testTag','弹出添加book弹窗')
})
}.height('100%')
.width('100%')
.justifyContent(FlexAlign.Center)
}
@Builder deleteBook(id:number){
Button(){
Image($r('app.media.app_icon'))
.width(24)
.height(24)
}.type(ButtonType.Circle)
.onClick(() => {
BookModel.deleteBookById(id)
this.books=BookModel.queryAllBooks()
})
}
}
// 添加图书弹窗
@CustomDialog
struct AddBook{
@Link books:Book[]
controller:CustomDialogController
private book:Book=new Book()
build() {
Column(){
Row(){
Text("书名:").fontSize(30)
TextInput({placeholder:'请输入书名'})
.onChange(val=>{
this.book.title=val
})
}.width('90%')
Row(){
Text("作者:").fontSize(30)
TextInput({placeholder:'请输入作者'})
.onChange(val=>{
this.book.author=val
})
}.width('90%')
Row(){
Text("出版日期:").fontSize(30)
TextInput({placeholder:'请输入出版日期'})
.onChange(val=>{
this.book.publicationYear=val
})
}.width('90%')
Row(){
Text("类型:").fontSize(30)
TextInput({placeholder:'请输入类型'})
.onChange(val=>{
this.book.type=val
})
}.width('90%')
Row(){
Button('取消')
.onClick(() => {
this.controller.close()
})
Button('确定')
.onClick(() => {
BookModel.addBook(this.book.title as string,this.book.author as string,this.book.publicationYear as string,this.book.type as string)
this.books=BookModel.queryAllBooks()
this.controller.close()
})
}
}
}
}
@Builder function BookCard(item:Book){
Column(){
Text('书名:'+item.title)
Text('作者:'+item.author)
Text('出版日期:'+item.publicationYear)
Text('类型:'+item.type)
}.borderRadius(30)
.borderWidth(5)
.width('90%')
.height(200)
}
二、用户管理模块
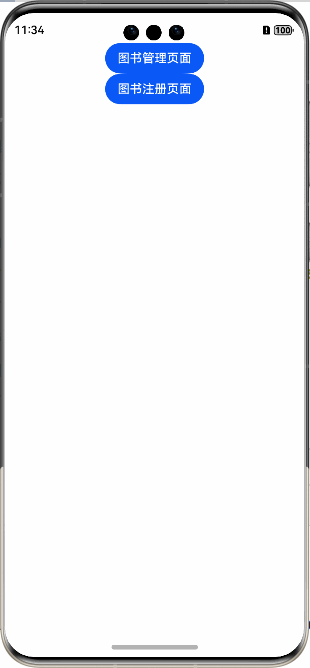
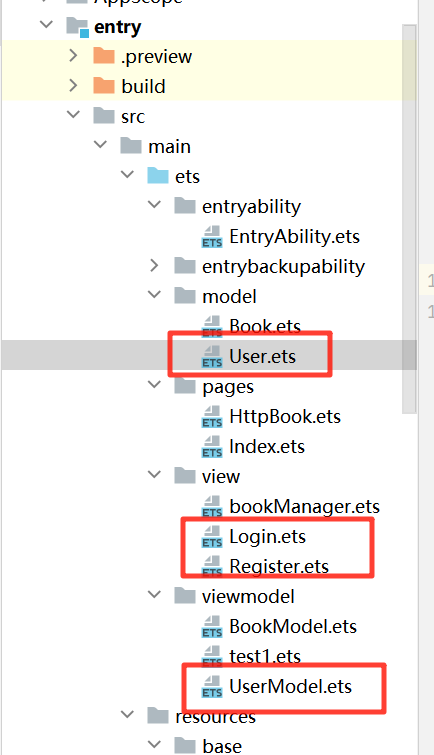
1、model
export default class User{
id?:number
username?:string
password?:string
constructor(id?:number,username?:string,password?:string) {
this.id=id
this.username=username
this.password=password
}
}
2、viewmodel
import { relationalStore, ValuesBucket } from '@kit.ArkData'
import { Context } from '@kit.AbilityKit'
import { BusinessError } from '@kit.BasicServicesKit'
import User from '../model/User'
import Book from '../model/Book'
class UserModel {
// 定义创建的数据库名称
private RdbName: string = 'users'
// 定义一个全局变量,用于获取创建出来的实例
private Rdb: relationalStore.RdbStore = {} as relationalStore.RdbStore
// 获取用户数据库实例
init(context: Context) {
// 初始化数据库信息
let config: relationalStore.StoreConfig = {
name: this.RdbName,
securityLevel: relationalStore.SecurityLevel.S1
}
let sql = `CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY AUTOINCREMENT,
username TEXT,
password TEXT
)`
relationalStore.getRdbStore(context, config, (err, data) => {
if (err) {
console.error('userTag', `创建实例${this.Rdb}失败`, err)
}
this.Rdb = data
console.log('userTag', `创建实例${this.Rdb}成功`, JSON.stringify(data))
// 执行sql语句,创建对应的表
data.executeSql(sql).then(() => {
console.log('userTag', `实例${this.Rdb}创建${this.RdbName}表成功`, err)
}).catch((e: BusinessError) => {
console.error('userTag', `实例${this.Rdb}创建${this.RdbName}表失败`, e)
})
})
}
// user增加
addUser(username: string, password: string): boolean {
try {
this.Rdb.insertSync(this.RdbName, { username, password })
console.log('userTag', `在${this.RdbName}插入数据成功`)
return true
} catch (e) {
console.error('userTag', `在${this.RdbName}插入数据失败`, e)
return false
}
}
// 根据用户名查询
queryUserByName(username: string): string|null {
// 查询条件
let predicate = new relationalStore.RdbPredicates(this.RdbName)
predicate.equalTo('username', username)
// predicate.equalTo('password',password)
// let user:User=new User('','')
// 查询,查询结果集
let result = this.Rdb.querySync(predicate, ['username'])
if (result.rowCount > 0) {
console.log('userTag', `在${this.RdbName}查询到${username}`)
return 'findit'
// if (result[0].username!=username){
// return true
} else {
console.log('userTag', `在${this.RdbName}的${username}不存在`)
return null
}
// return true
}
}
let userModel = new UserModel()
export default userModel as UserModel
3、view
(1)register页面
import User from '../model/User';
import UserModel from '../viewmodel/UserModel'
import { promptAction } from '@kit.ArkUI';
@Entry
@Component
struct UserManager {
@State message: string = 'Hello World';
@State user: User = new User()
build() {
Column() {
Row() {
Text('用户')
TextInput({ placeholder: '请输入用户名' })
.onChange(val => {
this.user.username = val
})
}
Row() {
Text('密码')
TextInput({ placeholder: '请输入密码' })
.onChange(val => {
this.user.password = val
})
}
Button('注册')
.onClick(() => {
const username = (this.user.username || '').trim();
const password = (this.user.password || '').trim();
if (!username || !password) {
// 如果用户名或密码为空(或仅包含空白字符),则显示提示
promptAction.showDialog({ message: '请输入有效的用户名和密码' });
} else {
const userExists = UserModel.queryUserByName(username);
if (userExists) {
// 用户已存在
promptAction.showDialog({ message: '用户已注册' });
} else {
// 用户不存在,尝试注册
const registrationSuccess = UserModel.addUser(username, password);
if (registrationSuccess) {
// 注册成功
promptAction.showDialog({ message: '注册成功' });
} else {
// 注册失败
promptAction.showDialog({ message: '注册失败' });
}
}
}
// const username=this.user.username?.trim() as string
// const password=this.user.password?.trim() as string
// if (username && password) {
// let u = UserModel.queryUserByName(username)
// if (u == null) {
// let res = UserModel.addUser(username, password)
// if (res) {
// promptAction.showDialog({ message: '注册成功' })
// } else {
// promptAction.showDialog({ message: '注册失败' })
// }
// } else {
// promptAction.showDialog({ message: '用户已注册' })
// }
// } else {
// promptAction.showDialog({ message: '请输入用户名和密码' })
// }
})
}.height('100%')
.width('100%')
}
}