导入依赖
<dependency>
<groupId>com.github.penggle</groupId>
<artifactId>kaptcha</artifactId>
<version>2.3.2</version>
</dependency>
代码实现
@Controller
public class VerificationCodeController {
@Resource
private DefaultKaptcha defaultKaptcha;
@GetMapping("/common/kaptcha")
public void defaultKaptch(HttpServletRequest httpServletRequest,HttpServletResponse httpServletResponse) throws IOException {
byte[] captchaOutPutStream = null;
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
try {
String kaptchaText = defaultKaptcha.createText();
httpServletRequest.getSession().setAttribute("verifyCode",kaptchaText);
BufferedImage image = defaultKaptcha.createImage(kaptchaText);
ImageIO.write(image,"jpg",byteArrayOutputStream);
} catch (IOException e) {
httpServletResponse.sendError(HttpServletResponse.SC_NOT_FOUND);
return;
}
captchaOutPutStream = byteArrayOutputStream.toByteArray();
httpServletResponse.setHeader("Cache-Control", "no-store");
httpServletResponse.setHeader("Pragma", "no-cache");
httpServletResponse.setDateHeader("Expires", 0);
httpServletResponse.setContentType("image/jpeg");
ServletOutputStream responseOutputStream = httpServletResponse.getOutputStream();
responseOutputStream.write(captchaOutPutStream);
responseOutputStream.flush();
responseOutputStream.close();
}
}
package com.cheng.controller.common;
import com.google.code.kaptcha.impl.DefaultKaptcha;
import com.google.code.kaptcha.util.Config;
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;
import sun.security.krb5.KrbException;
import java.util.Properties;
@Component
public class KaptchaConfig {
@Bean
public DefaultKaptcha getDefaultKaptcha() {
com.google.code.kaptcha.impl.DefaultKaptcha defaultKaptcha = new com.google.code.kaptcha.impl.DefaultKaptcha();
Properties properties = new Properties();
properties.put("kaptcha.border","no");
properties.put("kaptcha.textproducer.font.color", "black");
properties.put("kaptcha.image.width", "150");
properties.put("kaptcha.image.height", "40");
properties.put("kaptcha.textproducer.font.size", "30");
properties.put("kaptcha.session.key", "verifyCode");
properties.put("kaptcha.textproducer.char.space", "5");
Config config = new Config(properties);
defaultKaptcha.setConfig(config);
return defaultKaptcha;
}
}
效果图
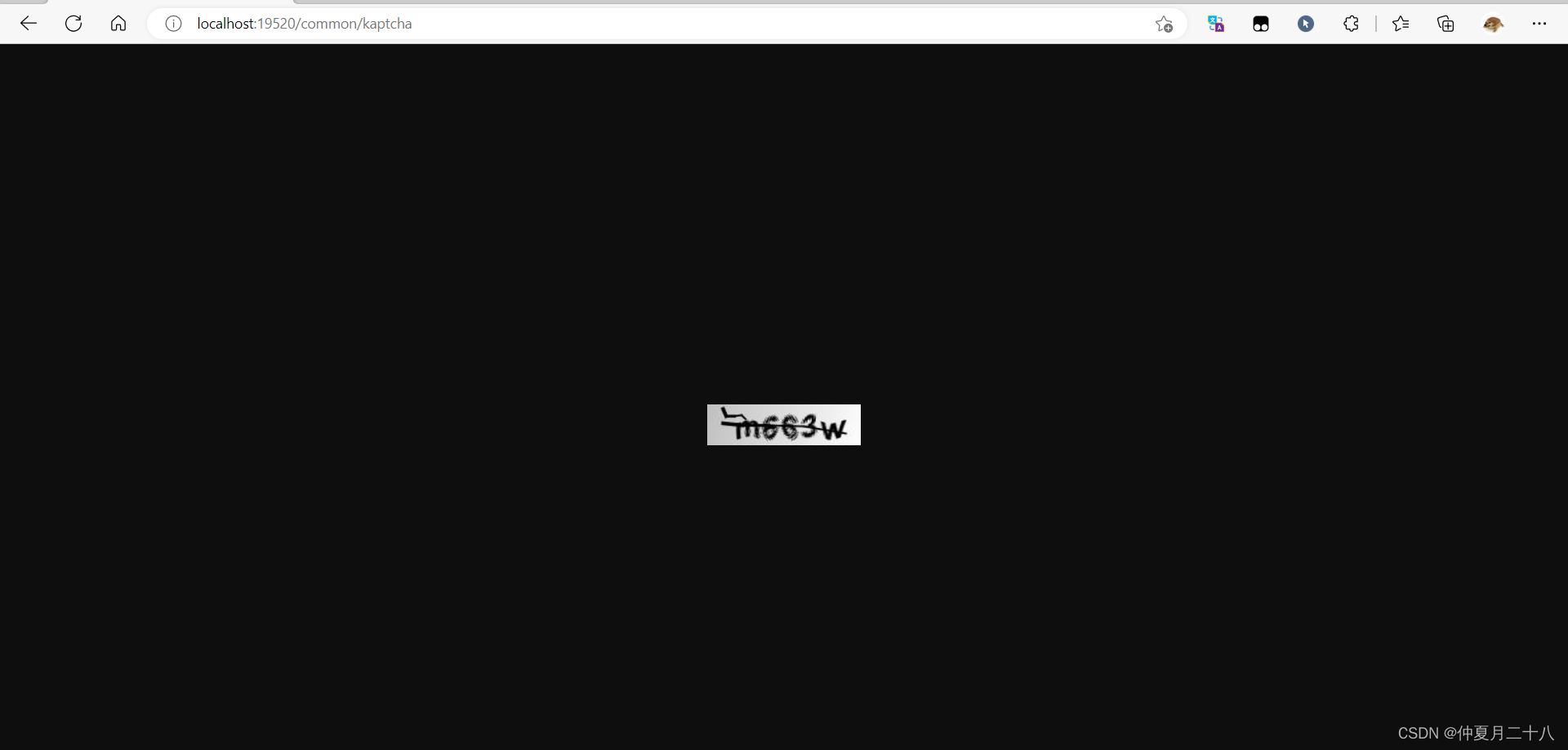