链表:
逻辑结构为线性结构,存储结构为链式存储的一种数据结构。数据元素之间的位置是任意(需要有这个元素才创建申请空间),在一个数据元素中由两部分构成,数据内容与关系。关系:通过指针元素来表示,存储下一个数据元素的地址。
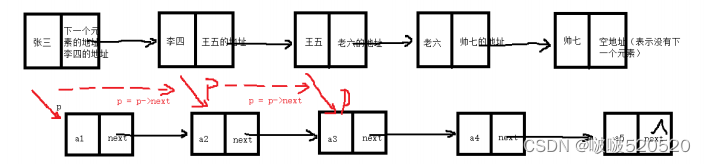
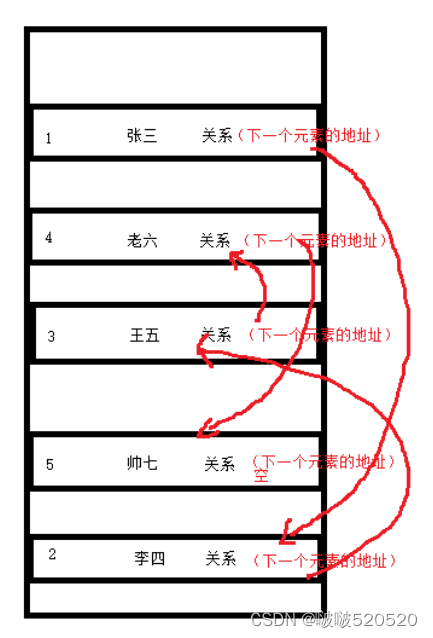
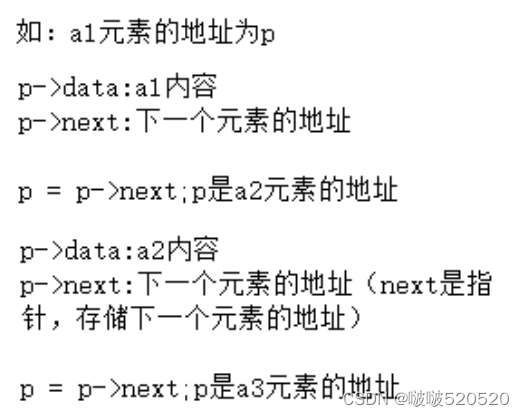
头指针:是一个指针(
存储地址
)
,存储链表中第一个节点
(
元素
)
,便于指明链表的位置,方便查找链表
节点: 链表的一个数据元素就叫做一个节点(数据、关系)
首元素节点:第一个数据节点,存储数据的元素节点,叫做首元素节点
头节点 :在链表中,通常会添加一个不存储数据的节点,就是空节点,作为链表的第一个节点,之后的元素节 点,从第二个开始,操作链表就不会操作到第一个节点,方便链表的操作。把人为添加的第一个节点叫做头节点
单向链表
双向链表
双向链表关系:前一个元素的地址,以及后一个元素的地址
单向链表与双向链表的区别在与多了一个头元素存储关系。除此之外还有循环链表,其差别是其最后一个元素指向的是头节点。
下面是一个单向链表的例句:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct node //结构体
{
char name[20];
struct node * next;
};
struct node * create() //创建头节点
{
struct node * head = malloc(sizeof(struct node));
if(head == NULL)
{
printf("malloc linklist error\n");
return NULL;
}
head->next = NULL;
return head;
}
void insert_list(struct node * head,int pos,char * data) //插入元素
{
struct node * p = head;
int i = 0;
while(i < pos - 1 && p->next != NULL)
{
p = p->next;
i++;
}
struct node * new = malloc(sizeof(struct node ));
new->next = p->next;
p->next = new;
strcpy(new->name,data);
}
int empty(struct node * head) //判断为空函数
{
if(head->next == NULL)
{
printf("is empty\n");
return 1;
}
return 0;
}
void delete_list(struct node * head,int pos) //删除元素
{
if(empty(head))
{
return ;
}
struct node * p = head;
int i = 0;
while(i < pos - 1 && p->next != NULL)
{
p = p->next;
i++;
}
if(p->next == NULL)
{
printf("pos is not found\n");
return ;
}
struct node * q = p->next;
p->next = q->next;
printf("delete data is : %s\n",q->name);
free(q);
}
void update_pos_list(struct node * head,int pos ,char * newdata) //在一定位置插入数据
{
struct node * p = head;
int i = 0;
while(i < pos)
{
p = p->next;
if(p == NULL)
{
printf("error pos\n");
return ;
}
i++;
}
strcpy(p->name,newdata);
}
void update_data_list(struct node * head,char * data,char * newdata) //根据数据换数据
{
struct node * p = head;
while(p->next != NULL)
{
p = p->next;
if( strcmp(p->name,data) == 0 )
{
strcpy(p->name,newdata);
}
}
}
void show_list(struct node * head) //打印全部元素
{
struct node * p = head;
while(p->next != NULL)
{
p = p->next;
printf("%s\t",p->name);
}
printf("\n");
}
int main()
{
struct node * head = create();
delete_list(head,5);
insert_list(head,10,"zhangsan");
insert_list(head,10,"laoliu");
show_list(head);
}