- 字符集
ASCII字符集:《美国信息交换标准代码》,包含英文字母、数字、标点符号、控制字符
特点:1个字符占1个字节
GBK字符集:中国人自己的字符集,兼容ASCII字符集,还包含2万多个汉字
特点:1个字母占用1个字节;1个汉字占用2个字节
Unicode字符集:包含世界上所有国家的文字,有三种编码方案,最常用的是UTF-8
UTF-8编码方案:英文字母、数字占1个字节兼容(ASCII编码)、汉字字符占3个字节
- IO流(字节流)
- 分类:字节流,字符流
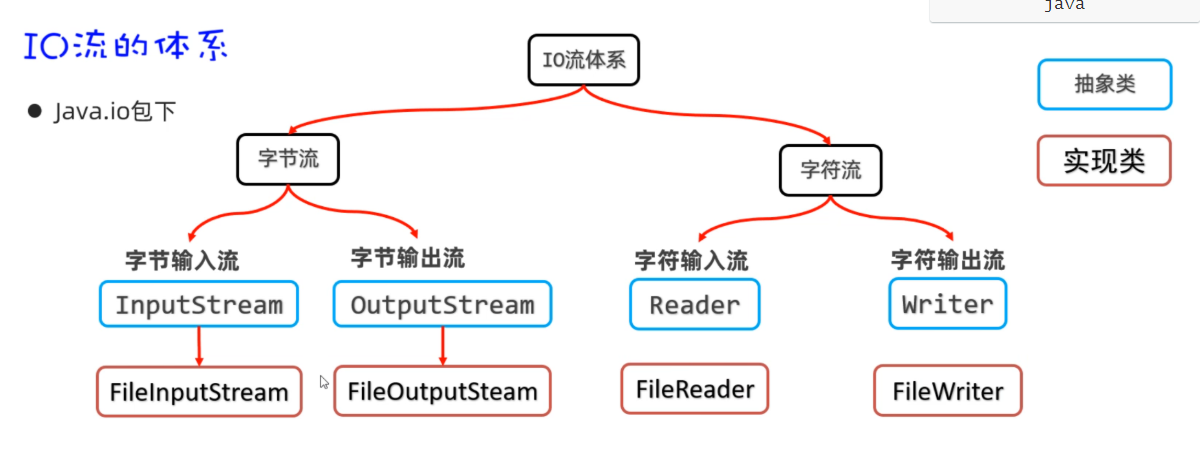
- 读取一个字节
public class FileInputStreamTest1 {
public static void main(String[] args) throws Exception {
InputStream is = new FileInputStream(("file-io-app\\src\\itheima01.txt"));
int b;
while ((b = is.read()) != -1){
System.out.print((char) b);
}
is.close();
}
}
- 读取多个字节
public class FileInputStreamTest2 {
public static void main(String[] args) throws Exception {
InputStream is = new FileInputStream("file-io-app\\src\\itheima02.txt");
byte[] buffer = new byte[3];
int len;
while ((len = is.read(buffer)) != -1){
String rs = new String(buffer, 0 , len);
System.out.print(rs);
}
is.close();
}
}
- 读取全部字节
InputStream is = new FileInputStream("file-io-app\\src\\itheima03.txt");
File f = new File("file-io-app\\src\\itheima03.txt");
long size = f.length();
byte[] buffer = new byte[(int) size];
int len = is.read(buffer);
System.out.println(new String(buffer));
is.close();
InputStream is = new FileInputStream("file-io-app\\src\\itheima03.txt");
byte[] buffer = is.readAllBytes();
System.out.println(new String(buffer));
is.close();
- 写字节
public class FileOutputStreamTest4 {
public static void main(String[] args) throws Exception {
OutputStream os =
new FileOutputStream("file-io-app/src/itheima04out.txt", true);
os.write(97);
os.write('b');
byte[] bytes = "我爱你中国abc".getBytes();
os.write(bytes);
os.write(bytes, 0, 15);
os.write("\r\n".getBytes());
os.close();
}
}
- 复制文件
public class CopyTest5 {
public static void main(String[] args) throws Exception {
InputStream is = new FileInputStream("D:/resource/meinv.png");
OutputStream os = new FileOutputStream("C:/data/meinv.png");
System.out.println(10 / 0);
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1){
os.write(buffer, 0, len);
}
os.close();
is.close();
System.out.println("复制完成!!");
}
}
- 资源释放
- JDK7以前
public class Test2 {
public static void main(String[] args) {
InputStream is = null;
OutputStream os = null;
try {
System.out.println(10 / 0);
is = new FileInputStream("file-io-app\\src\\itheima03.txt");
os = new FileOutputStream("file-io-app\\src\\itheima03copy.txt");
System.out.println(10 / 0);
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1){
os.write(buffer, 0, len);
}
System.out.println("复制完成!!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(os != null) os.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
if(is != null) is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
- JDK7以后
try(资源对象1; 资源对象2;){
使用资源的代码
}catch(异常类 e){
处理异常的代码
}
public class Test3 {
public static void main(String[] args) {
try (
InputStream is = new FileInputStream("D:/resource/meinv.png");
OutputStream os = new FileOutputStream("C:/data/meinv.png");
){
byte[] buffer = new byte[1024];
int len;
while ((len = is.read(buffer)) != -1){
os.write(buffer, 0, len);
}
System.out.println(conn);
System.out.println("复制完成!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}