一、实验内容
1、设计一个交通工具类Vehicle,其中的属性包括:速度speed、类别kind、颜色color;方法至少包括:设置速度、设置颜色、取得类别、取得颜色。创建Vehicle类的对象,为其设置速度和颜色,并显示其状态(所有属性)。
输入案例:
car
220
white
输出案例:You have a/an white car, its speed is 220 km/h.
package com.HelloWorld;
//设计一个交通工具类Vehicle,其中的属性包括:速度speed、类别kind、颜色color;
// 方法至少包括:设置速度、设置颜色、取得类别、取得颜色。创建Vehicle类的对象,为其设置速度和颜色,并显示其状态(所有属性)。
//设置速度setSpeed( )、设置颜色setColor( )、获取类别getKind( )、取得颜色getColor( )。
import java.util.Scanner;
class Vehicle{
private int speed;
private String kind;
private String color;
public String getKind(){
return kind;
}
public void setKind(String kind){
this.kind= kind;
}
public int getSpeed(){
return speed;
}
public void setSpeed(int speed){
this.speed=speed;
}
public String getColor(){
return color;
}
public void setColor(String color){
this.color=color;
}
}
public class test201 {
public static void main (String[] args){
Scanner sc=new Scanner(System.in);
String kind=sc.next();
int speed=sc.nextInt();
String color=sc.next();
Vehicle v=new Vehicle();
v.setKind(kind);
v.setSpeed(speed);
v.setColor(color);
System.out.println("You have a/an "+ v.getColor()+" "+v.getKind()+", "+"its speed is "+ v.getSpeed()+" Km/h.");
}
}
2、编写程序,模拟银行账户 Account的功能,此类要求如下:
属性:账号account、储户姓名name、地址address、存款余额balance、最小余额minbalance(默认为100元)。
方法中至少包括:存款、取款、查询。
在测试类中,根据用户操作显示储户相关信息。如存款操作后,显示储户原有余额、今日存款数额及最终存款余额;取款时,若最后余额小于最小余额,拒绝取款,并显示“at least keep the balance XXX”之类提示语句;查询时直接显示该账户的余额。(数据输入顺序依次为:原始金额、存款金额、取款金额、取款金额)
输入案例:3000 200 2200 2000
输出案例:
The original balance of your current account is 3000.0
Saving cash 200.0
The existing balance of your current account is 3200.0
Your withdrawal amount is 2200.0
Operation succeeded! The current balance is 1000.0
Your withdrawal amount is 2000.0
Operation failed, at least keep the balance 100.0
Your current account balance is 1000.0
package com.HelloWorld;
import java.util.Scanner;
public class test202 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
double balance = scanner.nextDouble();
double savingcash= scanner.nextDouble();
double getcash1 = scanner.nextDouble();
double getcash2 = scanner.nextDouble();
Account moon = new Account();
moon.setBalance(balance);
moon.savingCash(savingcash);
moon.getCash(getcash1);
moon.getCash(getcash2);
moon.query();
}
}
class Account {
private String account;
private String name;
private String address;
private double balance;
private static double minbalance = 100;
public void setAccount(String account) {
this.account = account;
}
public String getAccount() {
return account;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setAddress(String address) {
this.address = address;
}
public String getAddress() {
return address;
}
public void setBalance(double balance) {
this.balance = balance;
}
public double getBalance() {
return balance;
}
public static void setMinbalance(double minbalances) {
minbalance = minbalances;
}
public void savingCash(double cash) {
System.out.println("The original balance of your current account is " + balance);
System.out.println("Saving cash " + cash);
balance += cash;
System.out.println("The existing balance of your current account is " + balance);
System.out.println();
}
public void getCash(double cash) {
double temp = balance - cash;
System.out.println("Your withdrawal amount is " + cash);
if (temp < minbalance) {
System.out.println("Operation failed, at least keep the balance " + minbalance);
} else {
balance = temp;
System.out.println("Operation succeeded! The current balance is " + balance);
}
System.out.println();
}
public void query() {
System.out.println("Your current account balance is " + balance);
System.out.println();
}
}
3、创建银行账号类SavingAccount,用类变量interest存储年利率(默认是1.5%),用私有实例变量balance存储存款额。至少提供计算年利息的方法和计算月利息(年利息/12)的方法。编写一个测试程序测试该类,建立SavingAccount的对象saver,通过键盘输入存款额与年利率,计算并显示saver的存款额、年利息和月利息。
输入案例:
3000 0.03
输出案例:
Your balance is 3000.0
The annual interest is 90.0
The monthly interest is 7.5
package com.HelloWorld;
import java.util.Scanner;
public class test203 {
private double balance;
static double interest=1.5;
public double YearRate() {
return balance*interest;
}
public double MonthRate() {
return YearRate()/12;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
public static void setInterest(double interest) {
test203.interest = interest;
}
public static void main(String[] args) {
test203 saver = new test203();
Scanner scan = new Scanner(System.in);
saver.setBalance(scan.nextDouble());
saver.setInterest(scan.nextDouble());
System.out.println("Your balance is "+saver.getBalance());
System.out.println("The annual interest is "+saver.YearRate());
System.out.println("The monthly interest is "+saver.MonthRate());
}
}
四、实验结果与分析(包括:输入数据、输出数据、程序效率及正确性等)(此处写清题号与其答案,可截图)
程序运行结果截图如下:
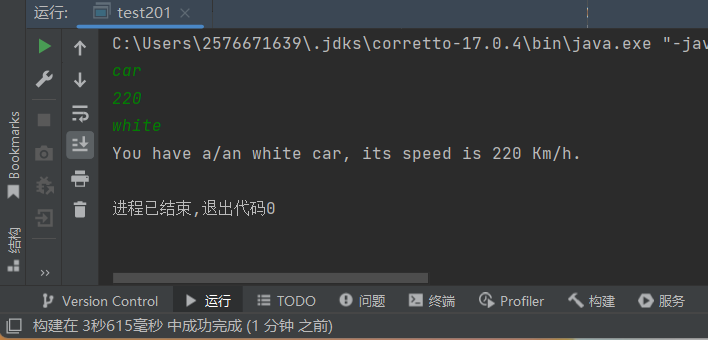
程序运行结果截图如下:
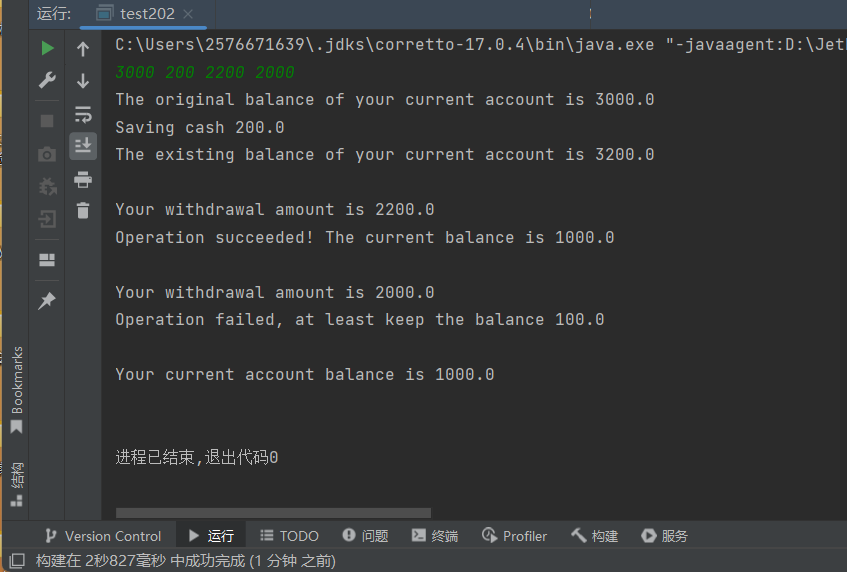
程序运行结果截图如下:
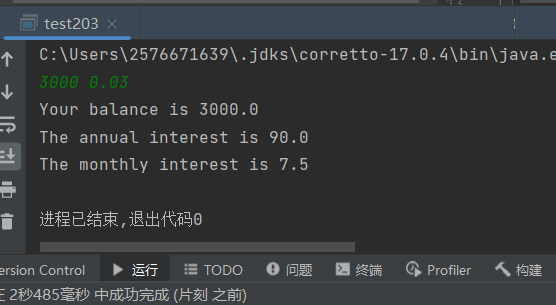
二、问题与讨论
1.在eclipse或者IEDA下结果正确,但是学习通提交完之后显示答案错误,看看输入输出是否一致。(1)是全部输入之后再输出,还是输入和输出交替;(2)输出是否完全一样,包括大小写、空格、标点符号、换行、数据类型!
2.提交的代码必须把public类放在前面,因为系统会把文档名命名为第一个出现的类的名字,而java要求文档名必须以public类命名。例如Test是public类,Vehicle放在前面,文档会被系统命名为Vehicle.java,就会出现上面的错误。改成Test放在前面就可以了。