- 我们将使用 read() 方法读取每一帧并将它们存储到各自的变量中。
- 我们定义了一个可变运动并将其初始化为零。
- 我们使用 cv2 函数 cvtColor 和 GaussianBlur 创建了另外两个变量 grayImage 和 grayFrame 来查找运动的变化。
- 如果我们的 initialState 是 None 那么我们将当前的 grayFrame 分配给 initialState 否则并使用“continue”关键字停止下一个进程。
- 在下一节中,我们计算了我们在当前迭代中创建的初始帧和灰度帧之间的差异。
- 然后我们将使用 cv2 阈值和扩张函数突出显示初始帧和当前帧之间的变化。
- 我们将从当前图像或帧中的移动对象中找到轮廓,并通过使用矩形函数在其周围创建绿色边界来指示移动对象。
- 在此之后,我们将通过添加当前检测到的元素来附加我们的motionTrackList。
- 我们使用imshow方法显示了所有的帧,如灰度和原始帧等。
- 此外,我们使用 cv2 模块的 witkey() 方法创建了一个键来结束进程,我们可以使用“m”键来结束我们的进程。
# starting the webCam to capture the video using cv2 module
video = cv2.VideoCapture(0)
# using infinite loop to capture the frames from the video
while True:
# Reading each image or frame from the video using read function
check, cur_frame = video.read()
# Defining 'motion' variable equal to zero as initial frame
var_motion = 0
# From colour images creating a gray frame
gray_image = cv2.cvtColor(cur_frame, cv2.COLOR_BGR2GRAY)
# To find the changes creating a GaussianBlur from the gray scale image
gray_frame = cv2.GaussianBlur(gray_image, (21, 21), 0)
# For the first iteration checking the condition
# we will assign grayFrame to initalState if is none
if initialState is None:
initialState = gray_frame
continue
# Calculation of difference between static or initial and gray frame we created
differ_frame = cv2.absdiff(initialState, gray_frame)
# the change between static or initial background and current gray frame are highlighted
thresh_frame = cv2.threshold(differ_frame, 30, 255, cv2.THRESH_BINARY)[1]
thresh_frame = cv2.dilate(thresh_frame, None, iterations = 2)
# For the moving object in the frame finding the coutours
cont,_ = cv2.findContours(thresh_frame.copy(),
cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for cur in cont:
if cv2.contourArea(cur) < 10000:
continue
var_motion = 1
(cur_x, cur_y,cur_w, cur_h) = cv2.boundingRect(cur)
# To create a rectangle of green color around the moving object
cv2.rectangle(cur_frame, (cur_x, cur_y), (cur_x + cur_w, cur_y + cur_h), (0, 255, 0), 3)
# from the frame adding the motion status
motionTrackList.append(var_motion)
motionTrackList = motionTrackList[-2:]
# Adding the Start time of the motion
if motionTrackList[-1] == 1 and motionTrackList[-2] == 0:
motionTime.append(datetime.now())
# Adding the End time of the motion
if motionTrackList[-1] == 0 and motionTrackList[-2] == 1:
motionTime.append(datetime.now())
# In the gray scale displaying the captured image
cv2.imshow("The image captured in the Gray Frame is shown below: ", gray_frame)
# To display the difference between inital static frame and the current frame
cv2.imshow("Difference between the inital static frame and the current frame: ", differ_frame)
# To display on the frame screen the black and white images from the video
cv2.imshow("Threshold Frame created from the PC or Laptop Webcam is: ", thresh_frame)
# Through the colour frame displaying the contour of the object
cv2.imshow("From the PC or Laptop webcam, this is one example of the Colour Frame:", cur_frame)
# Creating a key to wait
wait_key = cv2.waitKey(1)
# With the help of the 'm' key ending the whole process of our system
if wait_key == ord('m'):
# adding the motion variable value to motiontime list when something is moving on the screen
if var_motion == 1:
motionTime.append(datetime.now())
break
完成代码
关闭循环后,我们会将 dataFrame 和 motionTime 列表中的数据添加到 CSV 文件中,最后关闭视频。
# At last we are adding the time of motion or var\_motion inside the data frame
for a in range(0, len(motionTime), 2):
dataFrame = dataFrame.append({"Initial" : time[a], "Final" : motionTime[a + 1]}, ignore_index = True)
# To record all the movements, creating a CSV file
dataFrame.to_csv("EachMovement.csv")
# Releasing the video
video.release()
# Now, Closing or destroying all the open windows with the help of openCV
cv2.destroyAllWindows()
流程总结
我们已经创建了代码;现在让我们再次简要讨论该过程。
首先,我们使用设备的网络摄像头拍摄视频,然后将输入视频的初始帧作为参考,并不时检查下一帧。如果找到与第一个不同的帧,则存在运动。这将在绿色矩形中标记。
组合代码
我们已经在不同的部分看到了代码。现在,让我们结合起来:
# Importing the Pandas libraries
import pandas as panda
# Importing the OpenCV libraries
import cv2
# Importing the time module
import time
# Importing the datetime function of the datetime module
from datetime import datetime
# Assigning our initial state in the form of variable initialState as None for initial frames
initialState = None
# List of all the tracks when there is any detected of motion in the frames
motionTrackList= [ None, None ]
# A new list 'time' for storing the time when movement detected
motionTime = []
# Initialising DataFrame variable 'dataFrame' using pandas libraries panda with Initial and Final column
dataFrame = panda.DataFrame(columns = ["Initial", "Final"])
# starting the webCam to capture the video using cv2 module
video = cv2.VideoCapture(0)
# using infinite loop to capture the frames from the video
while True:
# Reading each image or frame from the video using read function
check, cur_frame = video.read()
# Defining 'motion' variable equal to zero as initial frame
var_motion = 0
# From colour images creating a gray frame
gray_image = cv2.cvtColor(cur_frame, cv2.COLOR_BGR2GRAY)
# To find the changes creating a GaussianBlur from the gray scale image
gray_frame = cv2.GaussianBlur(gray_image, (21, 21), 0)
# For the first iteration checking the condition
# we will assign grayFrame to initalState if is none
if initialState is None:
initialState = gray_frame
continue
# Calculation of difference between static or initial and gray frame we created
differ_frame = cv2.absdiff(initialState, gray_frame)
# the change between static or initial background and current gray frame are highlighted
thresh_frame = cv2.threshold(differ_frame, 30, 255, cv2.THRESH_BINARY)[1]
thresh_frame = cv2.dilate(thresh_frame, None, iterations = 2)
# For the moving object in the frame finding the coutours
cont,_ = cv2.findContours(thresh_frame.copy(),
cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for cur in cont:
if cv2.contourArea(cur) < 10000:
continue
var_motion = 1
(cur_x, cur_y,cur_w, cur_h) = cv2.boundingRect(cur)
# To create a rectangle of green color around the moving object
cv2.rectangle(cur_frame, (cur_x, cur_y), (cur_x + cur_w, cur_y + cur_h), (0, 255, 0), 3)
# from the frame adding the motion status
motionTrackList.append(var_motion)
motionTrackList = motionTrackList[-2:]
# Adding the Start time of the motion
if motionTrackList[-1] == 1 and motionTrackList[-2] == 0:
motionTime.append(datetime.now())
# Adding the End time of the motion
if motionTrackList[-1] == 0 and motionTrackList[-2] == 1:
motionTime.append(datetime.now())
# In the gray scale displaying the captured image
cv2.imshow("The image captured in the Gray Frame is shown below: ", gray_frame)
# To display the difference between inital static frame and the current frame
cv2.imshow("Difference between the inital static frame and the current frame: ", differ_frame)
# To display on the frame screen the black and white images from the video
cv2.imshow("Threshold Frame created from the PC or Laptop Webcam is: ", thresh_frame)
# Through the colour frame displaying the contour of the object
cv2.imshow("From the PC or Laptop webcam, this is one example of the Colour Frame:", cur_frame)
# Creating a key to wait
wait_key = cv2.waitKey(1)
# With the help of the 'm' key ending the whole process of our system
if wait_key == ord('m'):
# adding the motion variable value to motiontime list when something is moving on the screen
if var_motion == 1:
motionTime.append(datetime.now())
break
# At last we are adding the time of motion or var\_motion inside the data frame
for a in range(0, len(motionTime), 2):
如果你也是看准了Python,想自学Python,在这里为大家准备了丰厚的免费**学习**大礼包,带大家一起学习,给大家剖析Python兼职、就业行情前景的这些事儿。
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
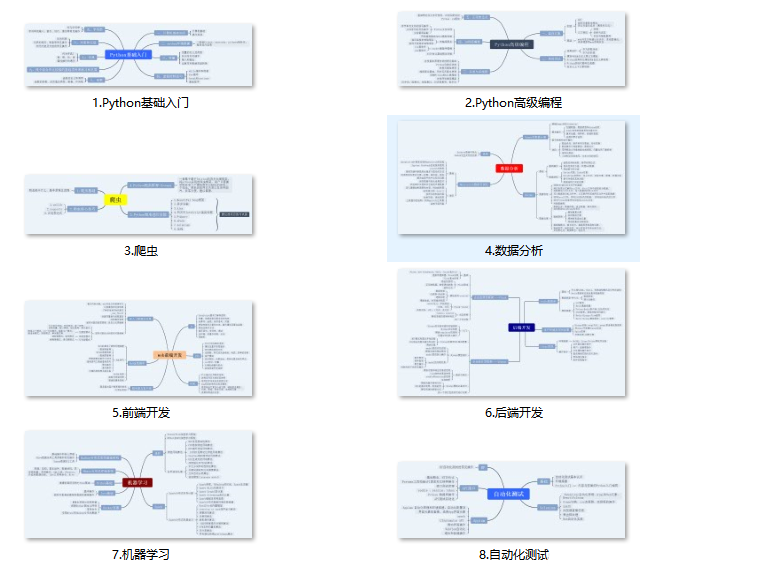
### 二、学习软件
工欲善其必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
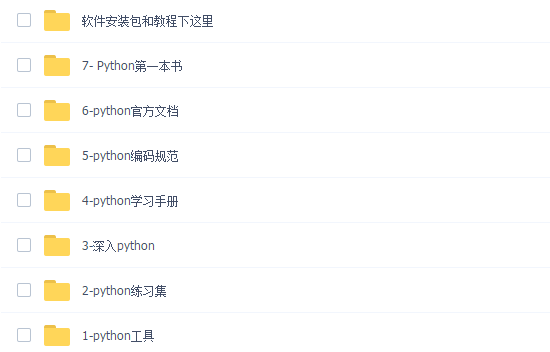
### 三、全套PDF电子书
书籍的好处就在于权威和体系健全,刚开始学习的时候你可以只看视频或者听某个人讲课,但等你学完之后,你觉得你掌握了,这时候建议还是得去看一下书籍,看权威技术书籍也是每个程序员必经之路。
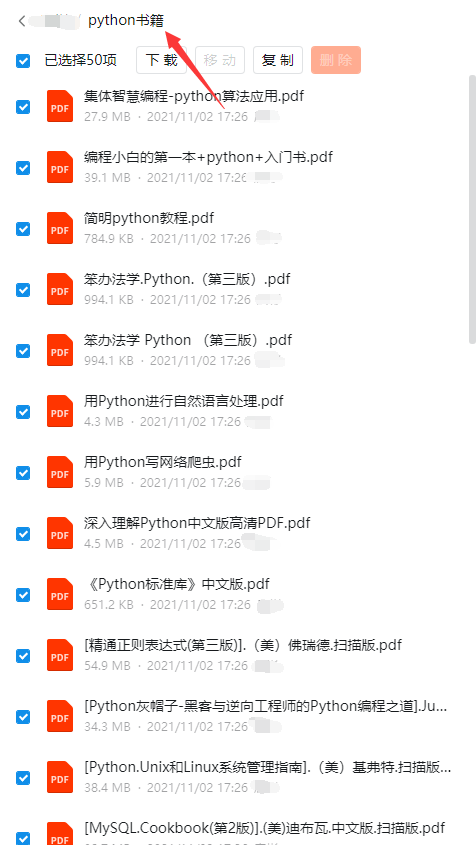
### 四、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
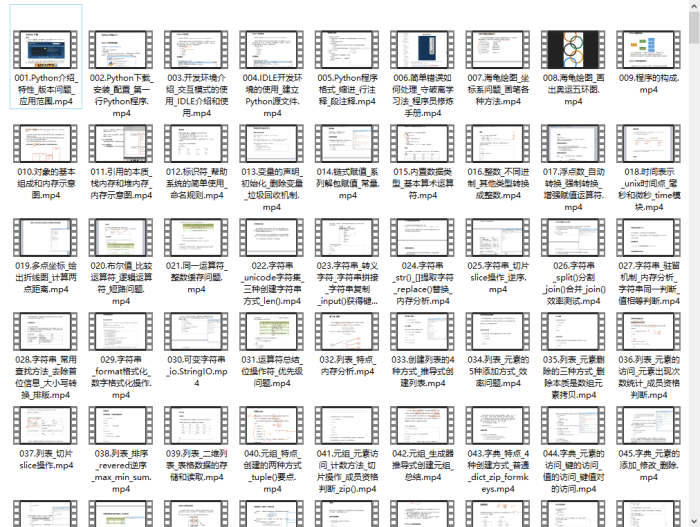
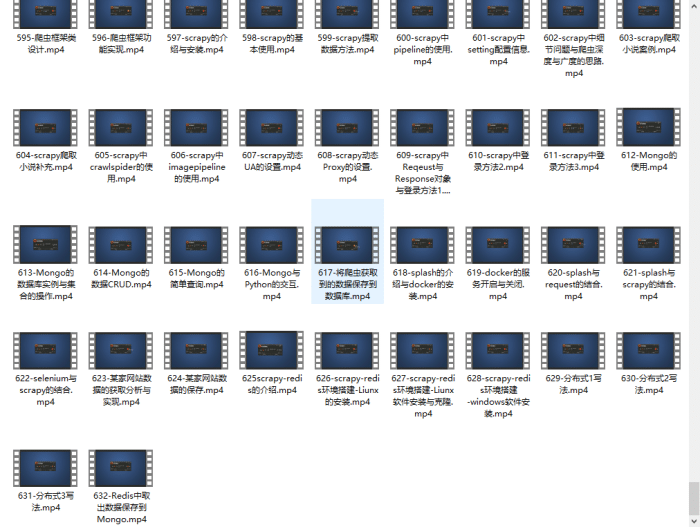
### 四、实战案例
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
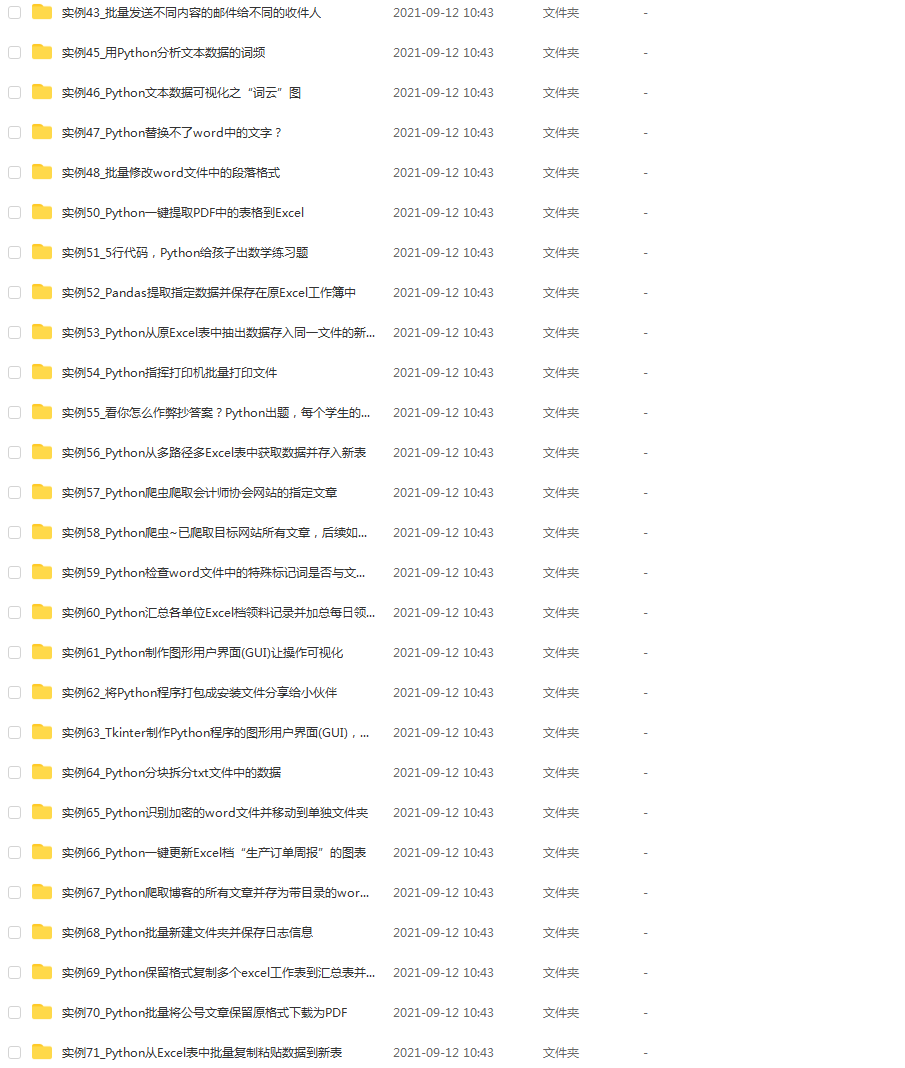
### 五、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
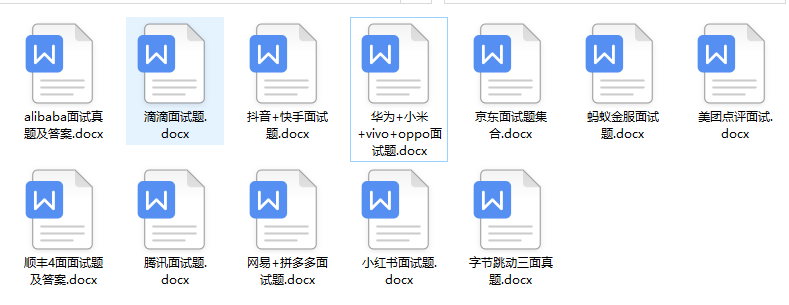
成为一个Python程序员专家或许需要花费数年时间,但是打下坚实的基础只要几周就可以,如果你按照我提供的学习路线以及资料有意识地去实践,你就有很大可能成功!
最后祝你好运!!!
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里无偿获取](https://bbs.csdn.net/topics/618317507)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**