#include<iostream>
using namespace std;
// 空间配置器 allocator 和C++标准库的allocator实现一样
// 泛型算法,给所有的容器都可以使用的
// 参数接受的都是容器的迭代器
template<typename T>
struct Allocator
{
T *allocator(size_t size)
{
return (T*)malloc(sizeof(T)*size);
}
void deallocate(void *p)
{
free(p);
}
void construct(T*p,const T &val)
{
new (p) T(val); //定位 new
}
void destroy(T *p)
{
p->~T(); //~T代表T类型
}
};
/*
容器底层内存开辟,和对象构造分开处理
容器底层内存开辟,内存释放,对象构造和析构,都是通过空间配置器来刷实现的
*/
template<typename T,typename Alloc=Allocator<T>>
class vector
{
public:
vector(int size=10)
{
//需要把内存开辟和对象构造分开
_first=_allocator.allocator(size);
_last=_first;
_end=_first+size;
}
~vector()
{
//析构容器有效原始,然后释放_first指针指向的堆内存
// delete[] _first;
for(T *p=_first;p!=_last;p++)
{
_allocator.destroy(p); //把_first指针指向的数组的有效元素进行析构
}
_allocator.deallocate(_first);
_first=_last=_end=nullptr;
}
vector(const vector<T> & rhs)
{
int size=rhs._end-rhs._first;
_first=_allocator.allocator(size);
int len=rhs._last-rhs._first;
for(int i=0;i<len;i++)
{
// _first[i]=rhs._first[i];
_allocator.construct(_first+i,rhs._first[i]);
}
_last=_first+len;
_end=_first+size;
}
vector<T> & operator=(const vector<T> &rhs)
{
if(this==&rhs)
{
return *this;
}
for(T &p=_first;p!=_last;++p)
{
_allocator.destroy(p);
}
_allocator.deallocate(_first);
int size=rhs._end-rhs._first;
_first=_allocator.allocator(size);
int len=rhs._last-rhs._first;
for(int i=0;i<len;i++)
{
_allocator.construct(_first+i,rhs._first[i]);
}
_last=_first+len;
_end=_first+size;
}
T& operator[] (int index)
{
if(index<0 || index>=size())
{
throw "OutOfRangeException";
}
return _first[index];
}
// 迭代器一般实现成容器的嵌套类型
void push_back(const T &val) //向容器末尾添加元素
{
if(full())
{
expand();
}
_allocator.construct(_last,val);
_last++;
}
void pop_back()
{
if(empty())
{
return;
}
//不仅要-- 还要析构删除的元素
_last--;
}
T back() const
{
return *(_last-1);
}
bool full() const
{
return _last==_end;
}
int size() const{
return _last-_first;
}
int capacity() const
{
return _end-_first;
}
bool empty() const
{
return _first==_last;
}
class iterator
{
public:
iterator(T*ptr=nullptr)
{
_ptr=ptr;
}
bool operator!=(const iterator &it) const
{
return _ptr !=it._ptr;
}
void operator++()
{
_ptr++;
}
T& operator*()
{
return *_ptr;
}
const T& operator*() const //提供方法
{
return *_ptr;
}
private:
T *_ptr;
};
iterator begin() {return iterator(_first);}
iterator end() {return iterator(_last);}
private:
T *_first;
T* _last;
T *_end;
Alloc _allocator;
void expand()
{
int size=_end-_first;
T *ptmp=_allocator.allocator(2*size);
for(int i=0;i<size;i++)
{
_allocator.construct(ptmp+i,_first[i]);
}
for(T *p=_first;p!=_last;++p)
{
_allocator.destroy(p);
}
_first=ptmp;
_last=_first+size;
_end=_first+2*size;
}
};
class Test
{
public:
Test()
{
cout<<"Test()"<<endl;
}
~Test()
{
cout<<"~Test()"<<endl;
}
};
int main(void)
{
// vector<Test> vec;
// Test t1,t2,t3;
// vec.push_back(t1);
// vec.push_back(t2);
// vec.push_back(t3);
vector<int> vec;
for(int i=0;i<20;i++)
{
vec.push_back(rand()%100+1);
}
for(int i=0;i<20;i++)
{
// vec.push_back(rand()%100+1);
cout<<vec[i]<<" ";
}
cout<<endl;
vector<int>::iterator it=vec.begin();
for(;it !=vec.end();++it)
{
cout<<*it<<" ";
}
cout<<endl;
for(auto iit:vec)
{
cout<<iit<<" ";
}
cout<<endl;
return 0;
}
vector的代码实现
于 2022-03-10 19:51:10 首次发布
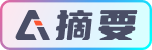