
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <pthread.h>
#include <unistd.h>
//线程执行体
void *create_pthread(void *arg)
{
//循环获取时间
time_t time1;
system("clear"); //让代码执行shell指令
while(1)
{
time(&time1);
struct tm *t = localtime(&time1);
printf("%d-%02d-%02d %02d:%02d:%02d\n",\
t->tm_year+1900,t->tm_mon+1,\
t->tm_mday,t->tm_hour,\
t->tm_min,t->tm_sec);
fflush(stdout);
sleep(3);
}
return NULL;
}
int main(int argc, const char *argv[])
{
//创建分支线程
pthread_t pth;
if(pthread_create(&pth, NULL, create_pthread, NULL) !=0)
{
perror("pthread_create");
return -1;
}
//主线程
char flag[10];
char a[10] = "quit";
while(1)
{
scanf("%s",flag);
if(strcmp(flag,a) == 0)
{
return 0;
}
}
return 0;
}
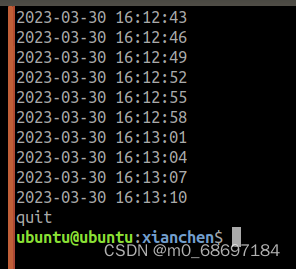
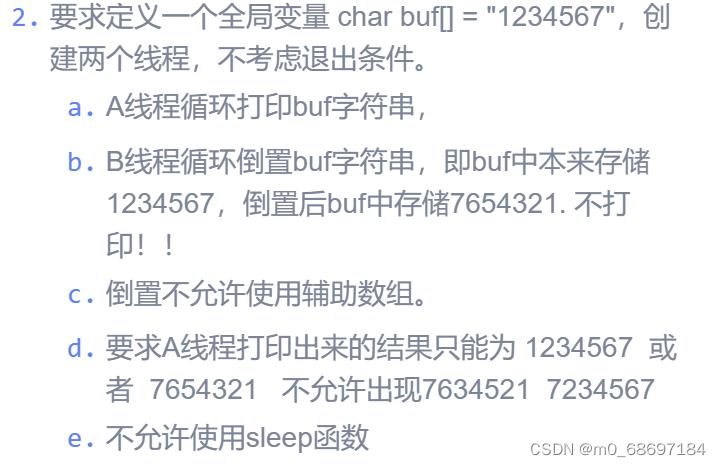
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/stat.h>
#include <sys/types.h>
#include <unistd.h>
#include <pthread.h>
//全局变量
char buf[]="1234567";
int flag =0;
//A分支线程
void*callbacka(void *arg)
{
while(1)
{
if(1==flag)
{
flag=0;
printf("%s\n",buf);
}
}
}
//B分支线程
void* callbackb(void* arf)
{
int temp=0;
while(1)
{
if(0 == flag)
{
for(int i=0;i<strlen(buf)/2;i++)
{
temp=buf[i];
buf[i]=buf[strlen(buf)-i-1];
buf[strlen(buf)-i-1]=temp;
}
flag=1;
}
}
}
int main(int argc, const char *argv[])
{ //主线程
pthread_t a; //创建一个A的tid号
pthread_t b; //创建一个B的tid号
//创建A的分支线程
if(pthread_create(&a, NULL, callbacka,NULL)!=0)
{
fprintf(stderr,"pthread_create failed");
return -1;
}
//创建B的分支线程
if(pthread_create(&b, NULL, callbackb,NULL)!=0)
{
fprintf(stderr,"pthread_create failed");
return -1;
}
//阻塞线程a b
pthread_join(a,NULL);
pthread_join(b,NULL);
return 0;
}
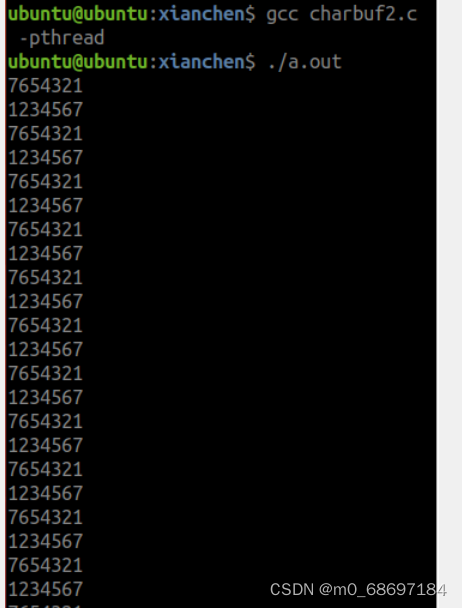