需求:根据后端返回的定位坐标数据实现定位渲染
1.效果图
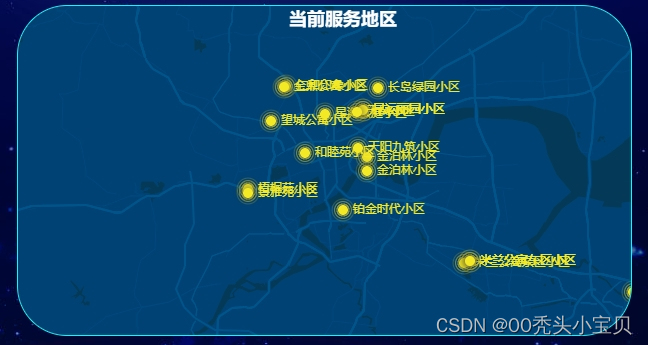
2.准备工作,在main.js和index.js文件中添加以下内容
main.js
app.use(BaiduMap, {
// ak 是在百度地图开发者平台申请的密钥 详见 http://lbsyun.baidu.com/apiconsole/key */
ak: 'sRDDfAKpCSG5iF1rvwph4Q95M6tDCApL',
// v:'3.0', // 默认使用3.0
// type: 'WebGL' // ||API 默认API (使用此模式 BMap=BMapGL)
});
index.js
<script type="text/javascript" src="https://api.map.baidu.com/getscript?v=3.0&ak=sRDDfAKpCSG5iF1rvwph4Q95M6tDCApL"></script>
3.html
<template>
<div>
<div id="main6"
style="width:650px;height:400px;"></div>
</div>
</template>
4.script
<script setup>
import * as echarts from 'echarts';
import 'echarts/extension/bmap/bmap';
import {onMounted,defineProps} from 'vue';
// import chinaMap from 'https://geo.datav.aliyun.com/areas_v3/bound/100000_full.json'
const props = defineProps(['data'])
var initData = JSON.parse(JSON.stringify(props)).data
console.log(initData,'88888888888888888888888888888888266')
const initMap = () =>{
var chartDom = document.getElementById('main6');
var myChart = echarts.init(chartDom);
var option;
option = {
backgroundColor: 'rgba(0,0,0,.1)',
title: {
text: '当前服务地区',
left: 'center',
textStyle: {
color: '#fff'
}
},
tooltip: {
trigger: 'item'
},
bmap: { //地理坐标系组件用于地图的绘制,支持在地图地理坐标系上绘制散点图,线集
center: [120.22221, 30.2084],
zoom: 10,
roam: true, //是否允许鼠标放大缩小
mapStyle: { //地图显示的样式
styleJson: [
{
featureType: 'water',
elementType: 'all',
stylers: {
color: '#044161'
}
},
{
featureType: 'land',
elementType: 'all',
stylers: {
color: '#004981'
}
},
{
featureType: 'boundary',
elementType: 'geometry',
stylers: {
color: '#064f85'
}
},
{
featureType: 'railway',
elementType: 'all',
stylers: {
visibility: 'off'
}
},
{
featureType: 'highway',
elementType: 'geometry',
stylers: {
color: '#004981'
}
},
{
featureType: 'highway',
elementType: 'geometry.fill',
stylers: {
color: '#005b96',
lightness: 1
}
},
{
featureType: 'highway',
elementType: 'labels',
stylers: {
visibility: 'off'
}
},
{
featureType: 'arterial',
elementType: 'geometry',
stylers: {
color: '#004981'
}
},
{
featureType: 'arterial',
elementType: 'geometry.fill',
stylers: {
color: '#00508b'
}
},
{
featureType: 'poi',
elementType: 'all',
stylers: {
visibility: 'off'
}
},
{
featureType: 'green',
elementType: 'all',
stylers: {
color: '#056197',
visibility: 'off'
}
},
{
featureType: 'subway',
elementType: 'all',
stylers: {
visibility: 'off'
}
},
{
featureType: 'manmade',
elementType: 'all',
stylers: {
visibility: 'off'
}
},
{
featureType: 'local',
elementType: 'all',
stylers: {
visibility: 'off'
}
},
{
featureType: 'arterial',
elementType: 'labels',
stylers: {
visibility: 'off'
}
},
{
featureType: 'boundary',
elementType: 'geometry.fill',
stylers: {
color: '#029fd4'
}
},
{
featureType: 'building',
elementType: 'all',
stylers: {
color: '#1a5787'
}
},
{
featureType: 'label',
elementType: 'all',
stylers: {
visibility: 'off'
}
}
]
}
},
series: [
{
name: '',
type: 'effectScatter', //样式
coordinateSystem: 'bmap', //该系列使用的坐标系
data:[], // data的数据格式为[{name:'海门',value:['121.15','31.89',200]},{name:'海门111',value:['121.15','31.89',200]}...]
encode: {
value: 2
},
showEffectOn: 'render',
rippleEffect: { //涟漪特效相关配置。
brushType: 'stroke' //波纹的绘制方式
},
symbolSize: 10, //标记的大小,可以设置数组或函数返回值的形式
hoverAnimation: true, // 鼠标移入放大圆
label: {
formatter: '{b}',
position: 'right',
show: true
},
itemStyle: { //样式
color: '#f4e925',
shadowBlur: 10,
shadowColor: '#333'
},
emphasis: { //高亮状态下的多边形和标签样式
scale: true
},
zlevel: 1
}
]
};
// 处理数据 将数据转换成echart可以识别的数据格式,例如[{name:'海门',value:['121.15','31.89',200]},{name:'海门111',value:['121.15','31.89',200]}...],详情请查echart地图坐标
let result = []
initData.map((item,index)=> {
let child = {name: '', value:['','']}
child.name = item.commName
child.value[0] = Number(item.mapX)
child.value[1] = Number(item.mapY)
child.value[2] = Number(item.mapY)
result.push(child)
})
console.log(result,'result')
option.series[0].data = result
// myChart.registerMap('china', { geoJSON: chinaMap })
option && myChart.setOption(option);
}
onMounted(() => {
initMap()
})
</script>