Pandas 数据处理工具(Pandas基础数据处理)
1.便捷的数据处理能力
2.读取文件方便
3.封装了Matplotlib ,Numpy的画图和计算
Pandas的核心数据结构
1.DataFrame
2.Panel
3.Series
import numpy as np
stock_change = np.random.normal(0,1,(10,5))
stock_change
array([[-8.95568808e-01, 4.06084744e-01, 2.39582146e+00,
5.11976280e-01, 2.77457517e-01],
[-4.51453894e-01, 6.31000994e-01, 1.24744949e+00,
-2.00202912e+00, -2.92207867e-01],
[ 6.55667369e-02, -7.84859167e-01, -4.12101825e-01,
-3.47667810e-01, 1.45793706e-03],
[-1.67876579e-01, -1.40968116e+00, 1.81237520e+00,
-1.92033529e+00, 9.38875605e-01],
[ 6.00839305e-02, -1.05816829e-01, -4.45666222e-01,
-1.81006228e-01, 2.05418850e-01],
[-6.77267207e-01, -7.53382789e-02, 3.97187494e-01,
1.07846908e-01, -1.32381297e+00],
[-1.68876926e+00, -1.70388042e-01, -3.94244637e-01,
2.25074192e+00, -6.01979955e-01],
[-1.91533157e+00, -1.22823000e-01, 3.91800427e-01,
-8.66979997e-01, 2.93894267e-01],
[ 1.36784986e+00, -7.75015875e-01, -1.71867214e+00,
4.12866314e-01, -1.37527880e+00],
[-1.04679747e-01, 4.59861072e-01, -7.55025583e-01,
-6.71892862e-01, -1.52619930e+00]])
import pandas as pd
pd.DataFrame(stock_change)
| 0 | 1 | 2 | 3 | 4 |
---|
0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
5 | -0.677267 | -0.075338 | 0.397187 | 0.107847 | -1.323813 |
---|
6 | -1.688769 | -0.170388 | -0.394245 | 2.250742 | -0.601980 |
---|
7 | -1.915332 | -0.122823 | 0.391800 | -0.866980 | 0.293894 |
---|
8 | 1.367850 | -0.775016 | -1.718672 | 0.412866 | -1.375279 |
---|
9 | -0.104680 | 0.459861 | -0.755026 | -0.671893 | -1.526199 |
---|
stock = ["股票{}".format(i) for i in range(10)]
pd.DataFrame(stock_change, index=stock)
| 0 | 1 | 2 | 3 | 4 |
---|
股票0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
股票1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
股票2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
股票3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
股票4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
股票5 | -0.677267 | -0.075338 | 0.397187 | 0.107847 | -1.323813 |
---|
股票6 | -1.688769 | -0.170388 | -0.394245 | 2.250742 | -0.601980 |
---|
股票7 | -1.915332 | -0.122823 | 0.391800 | -0.866980 | 0.293894 |
---|
股票8 | 1.367850 | -0.775016 | -1.718672 | 0.412866 | -1.375279 |
---|
股票9 | -0.104680 | 0.459861 | -0.755026 | -0.671893 | -1.526199 |
---|
date = pd.date_range(start="20180101", periods=5, freq="B")
date
DatetimeIndex(['2018-01-01', '2018-01-02', '2018-01-03', '2018-01-04',
'2018-01-05'],
dtype='datetime64[ns]', freq='B')
data = pd.DataFrame(stock_change, index=stock, columns=date)
data
| 2018-01-01 | 2018-01-02 | 2018-01-03 | 2018-01-04 | 2018-01-05 |
---|
股票0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
股票1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
股票2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
股票3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
股票4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
股票5 | -0.677267 | -0.075338 | 0.397187 | 0.107847 | -1.323813 |
---|
股票6 | -1.688769 | -0.170388 | -0.394245 | 2.250742 | -0.601980 |
---|
股票7 | -1.915332 | -0.122823 | 0.391800 | -0.866980 | 0.293894 |
---|
股票8 | 1.367850 | -0.775016 | -1.718672 | 0.412866 | -1.375279 |
---|
股票9 | -0.104680 | 0.459861 | -0.755026 | -0.671893 | -1.526199 |
---|
data.shape
(10, 5)
data.index
Index(['股票0', '股票1', '股票2', '股票3', '股票4', '股票5', '股票6', '股票7', '股票8', '股票9'], dtype='object')
data.columns
DatetimeIndex(['2018-01-01', '2018-01-02', '2018-01-03', '2018-01-04',
'2018-01-05'],
dtype='datetime64[ns]', freq='B')
data.values
array([[-8.95568808e-01, 4.06084744e-01, 2.39582146e+00,
5.11976280e-01, 2.77457517e-01],
[-4.51453894e-01, 6.31000994e-01, 1.24744949e+00,
-2.00202912e+00, -2.92207867e-01],
[ 6.55667369e-02, -7.84859167e-01, -4.12101825e-01,
-3.47667810e-01, 1.45793706e-03],
[-1.67876579e-01, -1.40968116e+00, 1.81237520e+00,
-1.92033529e+00, 9.38875605e-01],
[ 6.00839305e-02, -1.05816829e-01, -4.45666222e-01,
-1.81006228e-01, 2.05418850e-01],
[-6.77267207e-01, -7.53382789e-02, 3.97187494e-01,
1.07846908e-01, -1.32381297e+00],
[-1.68876926e+00, -1.70388042e-01, -3.94244637e-01,
2.25074192e+00, -6.01979955e-01],
[-1.91533157e+00, -1.22823000e-01, 3.91800427e-01,
-8.66979997e-01, 2.93894267e-01],
[ 1.36784986e+00, -7.75015875e-01, -1.71867214e+00,
4.12866314e-01, -1.37527880e+00],
[-1.04679747e-01, 4.59861072e-01, -7.55025583e-01,
-6.71892862e-01, -1.52619930e+00]])
data.T
| 股票0 | 股票1 | 股票2 | 股票3 | 股票4 | 股票5 | 股票6 | 股票7 | 股票8 | 股票9 |
---|
2018-01-01 | -0.895569 | -0.451454 | 0.065567 | -0.167877 | 0.060084 | -0.677267 | -1.688769 | -1.915332 | 1.367850 | -0.104680 |
---|
2018-01-02 | 0.406085 | 0.631001 | -0.784859 | -1.409681 | -0.105817 | -0.075338 | -0.170388 | -0.122823 | -0.775016 | 0.459861 |
---|
2018-01-03 | 2.395821 | 1.247449 | -0.412102 | 1.812375 | -0.445666 | 0.397187 | -0.394245 | 0.391800 | -1.718672 | -0.755026 |
---|
2018-01-04 | 0.511976 | -2.002029 | -0.347668 | -1.920335 | -0.181006 | 0.107847 | 2.250742 | -0.866980 | 0.412866 | -0.671893 |
---|
2018-01-05 | 0.277458 | -0.292208 | 0.001458 | 0.938876 | 0.205419 | -1.323813 | -0.601980 | 0.293894 | -1.375279 | -1.526199 |
---|
data.head()
| 2018-01-01 | 2018-01-02 | 2018-01-03 | 2018-01-04 | 2018-01-05 |
---|
股票0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
股票1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
股票2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
股票3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
股票4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
data.head(3)
| 2018-01-01 | 2018-01-02 | 2018-01-03 | 2018-01-04 | 2018-01-05 |
---|
股票0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
股票1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
股票2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
data.tail(2)
| 2018-01-01 | 2018-01-02 | 2018-01-03 | 2018-01-04 | 2018-01-05 |
---|
股票8 | 1.36785 | -0.775016 | -1.718672 | 0.412866 | -1.375279 |
---|
股票9 | -0.10468 | 0.459861 | -0.755026 | -0.671893 | -1.526199 |
---|
data.head()
| 2018-01-01 | 2018-01-02 | 2018-01-03 | 2018-01-04 | 2018-01-05 |
---|
股票0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
股票1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
股票2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
股票3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
股票4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
stock_ =["股票_{}".format(i) for i in range(10)]
data.index = stock_
data
| 2018-01-01 | 2018-01-02 | 2018-01-03 | 2018-01-04 | 2018-01-05 |
---|
股票_0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
股票_1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
股票_2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
股票_3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
股票_4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
股票_5 | -0.677267 | -0.075338 | 0.397187 | 0.107847 | -1.323813 |
---|
股票_6 | -1.688769 | -0.170388 | -0.394245 | 2.250742 | -0.601980 |
---|
股票_7 | -1.915332 | -0.122823 | 0.391800 | -0.866980 | 0.293894 |
---|
股票_8 | 1.367850 | -0.775016 | -1.718672 | 0.412866 | -1.375279 |
---|
股票_9 | -0.104680 | 0.459861 | -0.755026 | -0.671893 | -1.526199 |
---|
data.reset_index(drop = False)
| index | 2018-01-01 00:00:00 | 2018-01-02 00:00:00 | 2018-01-03 00:00:00 | 2018-01-04 00:00:00 | 2018-01-05 00:00:00 |
---|
0 | 股票_0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
1 | 股票_1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
2 | 股票_2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
3 | 股票_3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
4 | 股票_4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
5 | 股票_5 | -0.677267 | -0.075338 | 0.397187 | 0.107847 | -1.323813 |
---|
6 | 股票_6 | -1.688769 | -0.170388 | -0.394245 | 2.250742 | -0.601980 |
---|
7 | 股票_7 | -1.915332 | -0.122823 | 0.391800 | -0.866980 | 0.293894 |
---|
8 | 股票_8 | 1.367850 | -0.775016 | -1.718672 | 0.412866 | -1.375279 |
---|
9 | 股票_9 | -0.104680 | 0.459861 | -0.755026 | -0.671893 | -1.526199 |
---|
data.shape
(10, 5)
df = pd.DataFrame({'month':[1,4,7,10],
'year':[2012,2014,2013,2014],
'sale':[55,40,84,31]})
df
| month | year | sale |
---|
0 | 1 | 2012 | 55 |
---|
1 | 4 | 2014 | 40 |
---|
2 | 7 | 2013 | 84 |
---|
3 | 10 | 2014 | 31 |
---|
df.set_index("month",drop=True)
| year | sale |
---|
month | | |
---|
1 | 2012 | 55 |
---|
4 | 2014 | 40 |
---|
7 | 2013 | 84 |
---|
10 | 2014 | 31 |
---|
df.set_index("month",drop=False)
| month | year | sale |
---|
month | | | |
---|
1 | 1 | 2012 | 55 |
---|
4 | 4 | 2014 | 40 |
---|
7 | 7 | 2013 | 84 |
---|
10 | 10 | 2014 | 31 |
---|
new_df = df.set_index(["year","month"])
new_df
| | sale |
---|
year | month | |
---|
2012 | 1 | 55 |
---|
2014 | 4 | 40 |
---|
2013 | 7 | 84 |
---|
2014 | 10 | 31 |
---|
new_df.index
MultiIndex([(2012, 1),
(2014, 4),
(2013, 7),
(2014, 10)],
names=['year', 'month'])
new_df.index.names
FrozenList(['year', 'month'])
new_df.index.levels
FrozenList([[2012, 2013, 2014], [1, 4, 7, 10]])
Panel存储三维结构的数据
p = pd.Panel(np,arange(24).reshape(4,3,2),
items=list('ABCD'),
major_axis=pd.date_range('20130101',periods=3),
minor_axis=['first','second'])
---------------------------------------------------------------------------
AttributeError Traceback (most recent call last)
Cell In[71], line 1
----> 1 p = pd.Panel(np,arange(24).reshape(4,3,2),
2 items=list('ABCD'),
3 major_axis=pd.date_range('20130101',periods=3),
4 minor_axis=['first','second'])
AttributeError: module 'pandas' has no attribute 'Panel'
data
| 2018-01-01 | 2018-01-02 | 2018-01-03 | 2018-01-04 | 2018-01-05 |
---|
股票_0 | -0.895569 | 0.406085 | 2.395821 | 0.511976 | 0.277458 |
---|
股票_1 | -0.451454 | 0.631001 | 1.247449 | -2.002029 | -0.292208 |
---|
股票_2 | 0.065567 | -0.784859 | -0.412102 | -0.347668 | 0.001458 |
---|
股票_3 | -0.167877 | -1.409681 | 1.812375 | -1.920335 | 0.938876 |
---|
股票_4 | 0.060084 | -0.105817 | -0.445666 | -0.181006 | 0.205419 |
---|
股票_5 | -0.677267 | -0.075338 | 0.397187 | 0.107847 | -1.323813 |
---|
股票_6 | -1.688769 | -0.170388 | -0.394245 | 2.250742 | -0.601980 |
---|
股票_7 | -1.915332 | -0.122823 | 0.391800 | -0.866980 | 0.293894 |
---|
股票_8 | 1.367850 | -0.775016 | -1.718672 | 0.412866 | -1.375279 |
---|
股票_9 | -0.104680 | 0.459861 | -0.755026 | -0.671893 | -1.526199 |
---|
data.iloc[1,:]
2018-01-01 -0.451454
2018-01-02 0.631001
2018-01-03 1.247449
2018-01-04 -2.002029
2018-01-05 -0.292208
Freq: B, Name: 股票_1, dtype: float64
data.iloc[1,:].index
DatetimeIndex(['2018-01-01', '2018-01-02', '2018-01-03', '2018-01-04',
'2018-01-05'],
dtype='datetime64[ns]', freq='B')
sr = data.iloc[1,:]
sr.index
DatetimeIndex(['2018-01-01', '2018-01-02', '2018-01-03', '2018-01-04',
'2018-01-05'],
dtype='datetime64[ns]', freq='B')
sr.values
array([-0.45145389, 0.63100099, 1.24744949, -2.00202912, -0.29220787])
type(sr.values)
numpy.ndarray
pd.Series(np.arange(3,9,2),index=["a","b","c"])
a 3
b 5
c 7
dtype: int32
pd.Series({'red':100,'blue':200,'green':500,'yellow':1000})
red 100
blue 200
green 500
yellow 1000
dtype: int64
基本数据操作
import pandas as pd
data = pd.read_csv("./stock_day/stock_day.csv")
data
| open | high | close | low | volume | price_change | p_change | ma5 | ma10 | ma20 | v_ma5 | v_ma10 | v_ma20 | turnover |
---|
2018-02-27 | 23.53 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 22.942 | 22.142 | 22.875 | 53782.64 | 46738.65 | 55576.11 | 2.39 |
---|
2018-02-26 | 22.80 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 22.406 | 21.955 | 22.942 | 40827.52 | 42736.34 | 56007.50 | 1.53 |
---|
2018-02-23 | 22.88 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 21.938 | 21.929 | 23.022 | 35119.58 | 41871.97 | 56372.85 | 1.32 |
---|
2018-02-22 | 22.25 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 21.446 | 21.909 | 23.137 | 35397.58 | 39904.78 | 60149.60 | 0.90 |
---|
2018-02-14 | 21.49 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 21.366 | 21.923 | 23.253 | 33590.21 | 42935.74 | 61716.11 | 0.58 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-06 | 13.17 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 13.112 | 13.112 | 13.112 | 115090.18 | 115090.18 | 115090.18 | 6.16 |
---|
2015-03-05 | 12.88 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 12.820 | 12.820 | 12.820 | 98904.79 | 98904.79 | 98904.79 | 3.19 |
---|
2015-03-04 | 12.80 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 12.707 | 12.707 | 12.707 | 100812.93 | 100812.93 | 100812.93 | 2.30 |
---|
2015-03-03 | 12.52 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 12.610 | 12.610 | 12.610 | 117681.67 | 117681.67 | 117681.67 | 4.76 |
---|
2015-03-02 | 12.25 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 12.520 | 12.520 | 12.520 | 96291.73 | 96291.73 | 96291.73 | 3.30 |
---|
643 rows × 14 columns
data = data.drop(["ma5","ma10","ma20","v_ma5","v_ma10","v_ma20"], axis=1)
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2018-02-27 | 23.53 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 2.39 |
---|
2018-02-26 | 22.80 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 1.53 |
---|
2018-02-23 | 22.88 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 1.32 |
---|
2018-02-22 | 22.25 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 0.90 |
---|
2018-02-14 | 21.49 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 0.58 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-06 | 13.17 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 6.16 |
---|
2015-03-05 | 12.88 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-03-04 | 12.80 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-03 | 12.52 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-02 | 12.25 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data["open"]["2018-02-26"]
22.8
data.loc["2018-02-26"]["open"]
22.8
data.loc["2018-02-26","open"]
22.8
data.iloc[1,0]
22.8
data.loc[data.index[0:4],['open','close','high','low']]
| open | close | high | low |
---|
2018-02-27 | 23.53 | 24.16 | 25.88 | 23.53 |
---|
2018-02-26 | 22.80 | 23.53 | 23.78 | 22.80 |
---|
2018-02-23 | 22.88 | 22.82 | 23.37 | 22.71 |
---|
2018-02-22 | 22.25 | 22.28 | 22.76 | 22.02 |
---|
data.iloc[0:4,data.columns.get_indexer(['open','close','high','low'])]
| open | close | high | low |
---|
2018-02-27 | 23.53 | 24.16 | 25.88 | 23.53 |
---|
2018-02-26 | 22.80 | 23.53 | 23.78 | 22.80 |
---|
2018-02-23 | 22.88 | 22.82 | 23.37 | 22.71 |
---|
2018-02-22 | 22.25 | 22.28 | 22.76 | 22.02 |
---|
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2018-02-27 | 23.53 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 2.39 |
---|
2018-02-26 | 22.80 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 1.53 |
---|
2018-02-23 | 22.88 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 1.32 |
---|
2018-02-22 | 22.25 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 0.90 |
---|
2018-02-14 | 21.49 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 0.58 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-06 | 13.17 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 6.16 |
---|
2015-03-05 | 12.88 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-03-04 | 12.80 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-03 | 12.52 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-02 | 12.25 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data["open"]
2018-02-27 23.53
2018-02-26 22.80
2018-02-23 22.88
2018-02-22 22.25
2018-02-14 21.49
...
2015-03-06 13.17
2015-03-05 12.88
2015-03-04 12.80
2015-03-03 12.52
2015-03-02 12.25
Name: open, Length: 643, dtype: float64
data.open = 100
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2018-02-27 | 100 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 2.39 |
---|
2018-02-26 | 100 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 1.53 |
---|
2018-02-23 | 100 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 1.32 |
---|
2018-02-22 | 100 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 0.90 |
---|
2018-02-14 | 100 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 0.58 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-06 | 100 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 6.16 |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data.iloc[1,0]=222
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data.head()
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2018-02-27 | 100 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 2.39 |
---|
2018-02-26 | 222 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 1.53 |
---|
2018-02-23 | 100 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 1.32 |
---|
2018-02-22 | 100 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 0.90 |
---|
2018-02-14 | 100 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 0.58 |
---|
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2018-02-27 | 100 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 2.39 |
---|
2018-02-26 | 222 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 1.53 |
---|
2018-02-23 | 100 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 1.32 |
---|
2018-02-22 | 100 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 0.90 |
---|
2018-02-14 | 100 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 0.58 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-06 | 100 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 6.16 |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data.sort_values(by='high',ascending=False)
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data.sort_values(by=['high','p_change'],ascending=False)
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data.sort_index()
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-03-06 | 100 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 6.16 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2018-02-14 | 100 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 0.58 |
---|
2018-02-22 | 100 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 0.90 |
---|
2018-02-23 | 100 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 1.32 |
---|
2018-02-26 | 222 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 1.53 |
---|
2018-02-27 | 100 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 2.39 |
---|
643 rows × 8 columns
sr = data["price_change"]
sr
2015-06-10 0.51
2015-06-12 0.82
2017-10-31 2.38
2015-06-15 -3.52
2015-06-11 0.54
...
2015-03-05 0.26
2015-09-07 0.37
2015-03-03 0.18
2015-03-04 0.20
2015-03-02 0.32
Name: price_change, Length: 643, dtype: float64
sr.sort_values(ascending=False).head()
2015-06-09 3.03
2017-10-26 2.68
2015-05-21 2.57
2017-10-31 2.38
2017-06-22 2.36
Name: price_change, dtype: float64
sr.sort_index()
2015-03-02 0.32
2015-03-03 0.18
2015-03-04 0.20
2015-03-05 0.26
2015-03-06 1.12
...
2018-02-14 0.44
2018-02-22 0.36
2018-02-23 0.54
2018-02-26 0.69
2018-02-27 0.63
Name: price_change, Length: 643, dtype: float64
DataFrame运算
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data["open"].add(3).head()
2015-06-10 103
2015-06-12 225
2017-10-31 103
2015-06-15 103
2015-06-11 103
Name: open, dtype: int64
data+10
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 110 | 46.35 | 43.85 | 42.23 | 269043.12 | 10.51 | 11.53 | 19.21 |
---|
2015-06-12 | 232 | 45.98 | 45.21 | 44.01 | 159835.88 | 10.82 | 12.38 | 15.47 |
---|
2017-10-31 | 110 | 45.22 | 44.44 | 42.20 | 361670.88 | 12.38 | 17.42 | 19.05 |
---|
2015-06-15 | 110 | 44.99 | 41.69 | 41.69 | 199379.53 | 6.48 | 0.00 | 16.82 |
---|
2015-06-11 | 110 | 44.98 | 44.39 | 42.51 | 173085.73 | 10.54 | 11.59 | 15.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 110 | 23.45 | 23.16 | 22.87 | 93190.39 | 10.26 | 12.02 | 13.19 |
---|
2015-09-07 | 110 | 23.38 | 22.77 | 22.63 | 52500.04 | 10.37 | 12.98 | 11.80 |
---|
2015-03-03 | 110 | 23.06 | 22.70 | 22.52 | 139081.61 | 10.18 | 11.44 | 14.76 |
---|
2015-03-04 | 110 | 22.92 | 22.90 | 22.61 | 67085.44 | 10.20 | 11.57 | 12.30 |
---|
2015-03-02 | 110 | 22.67 | 22.52 | 22.20 | 96301.73 | 10.32 | 12.62 | 13.30 |
---|
643 rows × 8 columns
data.sub(100).head()
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 0 | -63.65 | -66.15 | -67.77 | 268933.12 | -99.49 | -98.47 | -90.79 |
---|
2015-06-12 | 122 | -64.02 | -64.79 | -65.99 | 159725.88 | -99.18 | -97.62 | -94.53 |
---|
2017-10-31 | 0 | -64.78 | -65.56 | -67.80 | 361560.88 | -97.62 | -92.58 | -90.95 |
---|
2015-06-15 | 0 | -65.01 | -68.31 | -68.31 | 199269.53 | -103.52 | -110.00 | -93.18 |
---|
2015-06-11 | 0 | -65.02 | -65.61 | -67.49 | 172975.73 | -99.46 | -98.41 | -94.08 |
---|
data["close"].sub(data["open"]).head()
2015-06-10 -66.15
2015-06-12 -186.79
2017-10-31 -65.56
2015-06-15 -68.31
2015-06-11 -65.61
dtype: float64
data["p_change"]>2
2015-06-10 False
2015-06-12 True
2017-10-31 True
2015-06-15 False
2015-06-11 False
...
2015-03-05 True
2015-09-07 True
2015-03-03 False
2015-03-04 False
2015-03-02 True
Name: p_change, Length: 643, dtype: bool
data[data["p_change"]>2].head()
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-16 | 100 | 33.48 | 32.35 | 29.61 | 153130.61 | 0.66 | 2.08 | 5.24 |
---|
2015-06-09 | 100 | 33.34 | 33.34 | 30.46 | 204438.47 | 3.03 | 10.00 | 7.00 |
---|
2017-10-27 | 100 | 33.20 | 33.11 | 31.45 | 333824.31 | 0.70 | 2.16 | 8.35 |
---|
data[(data["p_change"]>2) &(data["low"]<15)]
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-09-11 | 100 | 16.07 | 16.07 | 14.61 | 114900.25 | 1.46 | 9.99 | 3.93 |
---|
2015-09-29 | 100 | 16.00 | 15.47 | 14.81 | 78247.20 | 0.38 | 2.52 | 2.68 |
---|
2016-01-14 | 100 | 16.00 | 15.94 | 14.39 | 68228.87 | 0.70 | 4.59 | 2.34 |
---|
2015-03-27 | 100 | 15.86 | 15.77 | 14.90 | 120352.13 | 0.84 | 5.63 | 4.12 |
---|
2015-03-17 | 100 | 15.44 | 15.18 | 14.63 | 158770.77 | 0.31 | 2.08 | 5.43 |
---|
2016-02-04 | 100 | 15.25 | 15.15 | 14.52 | 40090.40 | 0.67 | 4.63 | 1.37 |
---|
2016-03-02 | 100 | 15.20 | 15.18 | 14.36 | 49128.62 | 0.77 | 5.34 | 1.68 |
---|
2015-09-22 | 100 | 15.19 | 14.97 | 14.48 | 81399.43 | 0.44 | 3.03 | 2.79 |
---|
2015-03-16 | 100 | 15.05 | 14.87 | 14.51 | 94468.30 | 0.40 | 2.76 | 3.23 |
---|
2015-09-09 | 100 | 14.88 | 14.63 | 13.95 | 95082.47 | 0.77 | 5.56 | 3.25 |
---|
2015-03-10 | 100 | 14.80 | 14.65 | 14.01 | 101213.51 | 0.34 | 2.38 | 3.46 |
---|
2016-02-02 | 100 | 14.71 | 14.55 | 14.05 | 42175.52 | 0.46 | 3.27 | 1.44 |
---|
2015-09-16 | 100 | 14.65 | 14.64 | 13.40 | 79799.99 | 1.32 | 9.91 | 2.73 |
---|
2016-03-01 | 100 | 14.60 | 14.40 | 14.02 | 29640.23 | 0.38 | 2.71 | 1.01 |
---|
2015-09-21 | 100 | 14.57 | 14.55 | 13.73 | 60466.03 | 0.54 | 3.85 | 2.07 |
---|
2015-03-13 | 100 | 14.50 | 14.47 | 14.08 | 61342.22 | 0.36 | 2.55 | 2.10 |
---|
2015-03-06 | 100 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 6.16 |
---|
2016-01-29 | 100 | 14.21 | 13.97 | 13.30 | 38279.97 | 0.40 | 2.95 | 1.31 |
---|
2015-09-08 | 100 | 13.98 | 13.85 | 12.67 | 60627.79 | 1.09 | 8.54 | 2.08 |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
data.query("p_change>2 & low<15").head()
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-09-11 | 100 | 16.07 | 16.07 | 14.61 | 114900.25 | 1.46 | 9.99 | 3.93 |
---|
2015-09-29 | 100 | 16.00 | 15.47 | 14.81 | 78247.20 | 0.38 | 2.52 | 2.68 |
---|
2016-01-14 | 100 | 16.00 | 15.94 | 14.39 | 68228.87 | 0.70 | 4.59 | 2.34 |
---|
2015-03-27 | 100 | 15.86 | 15.77 | 14.90 | 120352.13 | 0.84 | 5.63 | 4.12 |
---|
2015-03-17 | 100 | 15.44 | 15.18 | 14.63 | 158770.77 | 0.31 | 2.08 | 5.43 |
---|
data[data["turnover"].isin([4.19,2.39])]
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2018-02-27 | 100 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 2.39 |
---|
2017-07-25 | 100 | 24.20 | 23.70 | 22.64 | 167489.48 | 0.67 | 2.91 | 4.19 |
---|
2016-09-28 | 100 | 20.98 | 20.86 | 19.71 | 95580.75 | 0.98 | 4.93 | 2.39 |
---|
2015-04-07 | 100 | 17.98 | 17.54 | 16.50 | 122471.85 | 0.88 | 5.28 | 4.19 |
---|
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data.describe()
| open | high | close | low | volume | price_change | p_change | turnover |
---|
count | 643.000000 | 643.000000 | 643.000000 | 643.000000 | 643.000000 | 643.000000 | 643.000000 | 643.000000 |
---|
mean | 100.379471 | 21.900513 | 21.336267 | 20.771835 | 99905.519114 | 0.018802 | 0.190280 | 2.936190 |
---|
std | 6.798778 | 4.077578 | 3.942806 | 3.791968 | 73879.119354 | 0.898476 | 4.079698 | 2.079375 |
---|
min | 100.000000 | 12.670000 | 12.360000 | 12.200000 | 1158.120000 | -3.520000 | -10.030000 | 0.040000 |
---|
25% | 100.000000 | 19.500000 | 19.045000 | 18.525000 | 48533.210000 | -0.390000 | -1.850000 | 1.360000 |
---|
50% | 100.000000 | 21.970000 | 21.450000 | 20.980000 | 83175.930000 | 0.050000 | 0.260000 | 2.500000 |
---|
75% | 100.000000 | 24.065000 | 23.415000 | 22.850000 | 127580.055000 | 0.455000 | 2.305000 | 3.915000 |
---|
max | 222.000000 | 36.350000 | 35.210000 | 34.010000 | 501915.410000 | 3.030000 | 10.030000 | 12.560000 |
---|
data.max()
open 222.00
high 36.35
close 35.21
low 34.01
volume 501915.41
price_change 3.03
p_change 10.03
turnover 12.56
dtype: float64
data.idxmax()
open 2015-06-12
high 2015-06-10
close 2015-06-12
low 2015-06-12
volume 2017-10-26
price_change 2015-06-09
p_change 2015-08-28
turnover 2017-10-26
dtype: object
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data["p_change"].sort_index().cumsum()
2015-03-02 2.62
2015-03-03 4.06
2015-03-04 5.63
2015-03-05 7.65
2015-03-06 16.16
...
2018-02-14 112.59
2018-02-22 114.23
2018-02-23 116.65
2018-02-26 119.67
2018-02-27 122.35
Name: p_change, Length: 643, dtype: float64
data["p_change"].sort_index().cumsum().plot()
<Axes: >
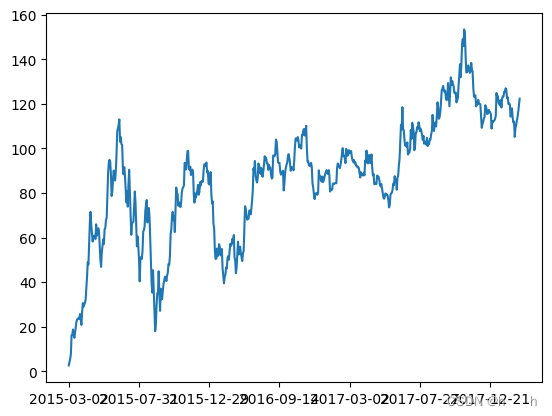
data.apply(lambda x:x.max() - x.min())
open 122.00
high 23.68
close 22.85
low 21.81
volume 500757.29
price_change 6.55
p_change 20.06
turnover 12.52
dtype: float64
data["open"].max()-data["open"].min()
122
data
| open | high | close | low | volume | price_change | p_change | turnover |
---|
2015-06-10 | 100 | 36.35 | 33.85 | 32.23 | 269033.12 | 0.51 | 1.53 | 9.21 |
---|
2015-06-12 | 222 | 35.98 | 35.21 | 34.01 | 159825.88 | 0.82 | 2.38 | 5.47 |
---|
2017-10-31 | 100 | 35.22 | 34.44 | 32.20 | 361660.88 | 2.38 | 7.42 | 9.05 |
---|
2015-06-15 | 100 | 34.99 | 31.69 | 31.69 | 199369.53 | -3.52 | -10.00 | 6.82 |
---|
2015-06-11 | 100 | 34.98 | 34.39 | 32.51 | 173075.73 | 0.54 | 1.59 | 5.92 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-05 | 100 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 3.19 |
---|
2015-09-07 | 100 | 13.38 | 12.77 | 12.63 | 52490.04 | 0.37 | 2.98 | 1.80 |
---|
2015-03-03 | 100 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 4.76 |
---|
2015-03-04 | 100 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 2.30 |
---|
2015-03-02 | 100 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 3.30 |
---|
643 rows × 8 columns
data.plot(x="volume",y="turnover",kind="scatter")
<Axes: xlabel='volume', ylabel='turnover'>
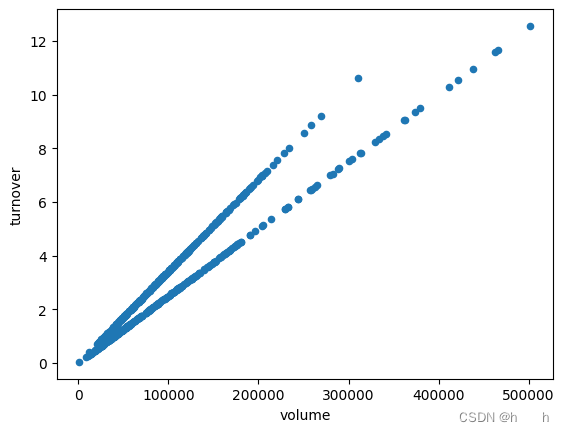
Pandas文件操作
pd.read_csv("./stock_day/stock_day.csv",usecols=["high","low","open","close"]).head()
| open | high | close | low |
---|
2018-02-27 | 23.53 | 25.88 | 24.16 | 23.53 |
---|
2018-02-26 | 22.80 | 23.78 | 23.53 | 22.80 |
---|
2018-02-23 | 22.88 | 23.37 | 22.82 | 22.71 |
---|
2018-02-22 | 22.25 | 22.76 | 22.28 | 22.02 |
---|
2018-02-14 | 21.49 | 21.99 | 21.92 | 21.48 |
---|
pd.read_csv("stock_day2.csv")
| 2018-02-27 | 23.53 | 25.88 | 24.16 | 23.53.1 | 95578.03 | 0.63 | 2.68 | 22.942 | 22.142 | 22.875 | 53782.64 | 46738.65 | 55576.11 | 2.39 |
---|
0 | 2018-02-26 | 22.80 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 22.406 | 21.955 | 22.942 | 40827.52 | 42736.34 | 56007.50 | 1.53 |
---|
1 | 2018-02-23 | 22.88 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 21.938 | 21.929 | 23.022 | 35119.58 | 41871.97 | 56372.85 | 1.32 |
---|
2 | 2018-02-22 | 22.25 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 21.446 | 21.909 | 23.137 | 35397.58 | 39904.78 | 60149.60 | 0.90 |
---|
3 | 2018-02-14 | 21.49 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 21.366 | 21.923 | 23.253 | 33590.21 | 42935.74 | 61716.11 | 0.58 |
---|
4 | 2018-02-13 | 21.40 | 21.90 | 21.48 | 21.31 | 30802.45 | 0.28 | 1.32 | 21.342 | 22.103 | 23.387 | 39694.65 | 45518.14 | 65161.68 | 0.77 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
637 | 2015-03-06 | 13.17 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 13.112 | 13.112 | 13.112 | 115090.18 | 115090.18 | 115090.18 | 6.16 |
---|
638 | 2015-03-05 | 12.88 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 12.820 | 12.820 | 12.820 | 98904.79 | 98904.79 | 98904.79 | 3.19 |
---|
639 | 2015-03-04 | 12.80 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 12.707 | 12.707 | 12.707 | 100812.93 | 100812.93 | 100812.93 | 2.30 |
---|
640 | 2015-03-03 | 12.52 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 12.610 | 12.610 | 12.610 | 117681.67 | 117681.67 | 117681.67 | 4.76 |
---|
641 | 2015-03-02 | 12.25 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 12.520 | 12.520 | 12.520 | 96291.73 | 96291.73 | 96291.73 | 3.30 |
---|
642 rows × 15 columns
pd.read_csv("stock_day2.csv",names=["open", "high", "close", "low", "volume", "price_change", "p_change", "ma5", "ma10", "ma20", "v_ma5", "v_ma10", "v_ma20", "turnover"])
| open | high | close | low | volume | price_change | p_change | ma5 | ma10 | ma20 | v_ma5 | v_ma10 | v_ma20 | turnover |
---|
2018-02-27 | 23.53 | 25.88 | 24.16 | 23.53 | 95578.03 | 0.63 | 2.68 | 22.942 | 22.142 | 22.875 | 53782.64 | 46738.65 | 55576.11 | 2.39 |
---|
2018-02-26 | 22.80 | 23.78 | 23.53 | 22.80 | 60985.11 | 0.69 | 3.02 | 22.406 | 21.955 | 22.942 | 40827.52 | 42736.34 | 56007.50 | 1.53 |
---|
2018-02-23 | 22.88 | 23.37 | 22.82 | 22.71 | 52914.01 | 0.54 | 2.42 | 21.938 | 21.929 | 23.022 | 35119.58 | 41871.97 | 56372.85 | 1.32 |
---|
2018-02-22 | 22.25 | 22.76 | 22.28 | 22.02 | 36105.01 | 0.36 | 1.64 | 21.446 | 21.909 | 23.137 | 35397.58 | 39904.78 | 60149.60 | 0.90 |
---|
2018-02-14 | 21.49 | 21.99 | 21.92 | 21.48 | 23331.04 | 0.44 | 2.05 | 21.366 | 21.923 | 23.253 | 33590.21 | 42935.74 | 61716.11 | 0.58 |
---|
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
---|
2015-03-06 | 13.17 | 14.48 | 14.28 | 13.13 | 179831.72 | 1.12 | 8.51 | 13.112 | 13.112 | 13.112 | 115090.18 | 115090.18 | 115090.18 | 6.16 |
---|
2015-03-05 | 12.88 | 13.45 | 13.16 | 12.87 | 93180.39 | 0.26 | 2.02 | 12.820 | 12.820 | 12.820 | 98904.79 | 98904.79 | 98904.79 | 3.19 |
---|
2015-03-04 | 12.80 | 12.92 | 12.90 | 12.61 | 67075.44 | 0.20 | 1.57 | 12.707 | 12.707 | 12.707 | 100812.93 | 100812.93 | 100812.93 | 2.30 |
---|
2015-03-03 | 12.52 | 13.06 | 12.70 | 12.52 | 139071.61 | 0.18 | 1.44 | 12.610 | 12.610 | 12.610 | 117681.67 | 117681.67 | 117681.67 | 4.76 |
---|
2015-03-02 | 12.25 | 12.67 | 12.52 | 12.20 | 96291.73 | 0.32 | 2.62 | 12.520 | 12.520 | 12.520 | 96291.73 | 96291.73 | 96291.73 | 3.30 |
---|
643 rows × 14 columns
data[:10].to_csv("test.csv",columns=["open"],index=False,mode='a',header=False)
pd.read_csv(“test.csv”)
import pandas as pd
#HDF5文件的读取和存储 二进制文件
#:读取和存储一般需要指定一个键,值即为要存储的DataFrame 相当于存入一个三维数组,每一个键都是一个二维
day_close=pd.read_hdf(“./stock_data/day/day_close.h5”)
day_close.to_hdf(“test.h5”,key=“close”)pd.read_hdf(“test.h5”,key=“close”)day_open = pd.read_hdf(“./stock_data/day/day_open.h5”)day_open.to_hdf(“test.h5”,key=“open”)
sa =pd.read_json("Sarcasm_Headlines_Dataset.json", orient="records", lines=True)
sa
| article_link | headline | is_sarcastic |
---|
0 | https://www.huffingtonpost.com/entry/versace-b... | former versace store clerk sues over secret 'b... | 0 |
---|
1 | https://www.huffingtonpost.com/entry/roseanne-... | the 'roseanne' revival catches up to our thorn... | 0 |
---|
2 | https://local.theonion.com/mom-starting-to-fea... | mom starting to fear son's web series closest ... | 1 |
---|
3 | https://politics.theonion.com/boehner-just-wan... | boehner just wants wife to listen, not come up... | 1 |
---|
4 | https://www.huffingtonpost.com/entry/jk-rowlin... | j.k. rowling wishes snape happy birthday in th... | 0 |
---|
... | ... | ... | ... |
---|
26704 | https://www.huffingtonpost.com/entry/american-... | american politics in moral free-fall | 0 |
---|
26705 | https://www.huffingtonpost.com/entry/americas-... | america's best 20 hikes | 0 |
---|
26706 | https://www.huffingtonpost.com/entry/reparatio... | reparations and obama | 0 |
---|
26707 | https://www.huffingtonpost.com/entry/israeli-b... | israeli ban targeting boycott supporters raise... | 0 |
---|
26708 | https://www.huffingtonpost.com/entry/gourmet-g... | gourmet gifts for the foodie 2014 | 0 |
---|
26709 rows × 3 columns
sa.to_json("test.json",orient="records",lines=True)