一,比较两个元素的大小,在程序编写时会经常遇到,特别是在进行元素排序时,“比大小”必不可少。编程高手们总喜欢想着“一劳永逸”,编写一个程序可以适用不同情况,而且容易拓展。小王同学以编程高手为目标,想编写一个类,其中包含了各种各样的“比大小”方法,而且名称全部相同,调用形式也基本一样,小王同学已经将程序的主类设计完成,并添加了注释,请你帮他完善各个功能类的代码。
//比较两个对象的大小,每个类的对象大小比较规则不尽相同
import java.util.Scanner;
public class Main {
public static Scanner reader=new Scanner(System.in);
public static void main(String[] args) {
Main ct=new Main(); //新建一个Main对象ct,
Point p1=new Point(),p2=new Point();//定义两个Point型对象p1,p2;
ct.input(p1,p2);//输入p1和p2对象的成员变量值
System.out.println(ct.compare(p1,p2));//输入对象p1和p2的比较结果,如果p1大,输出true,否则输出false
Fraction f1=new Fraction(),f2=new Fraction();//新建两个Fraction型对象f1,f2;
f1.input(); f2.input();//输入f1,f2对象的成员变量值;
System.out.println(ct.compare(f1,f2));//输入对象f1和f2的比较结果,如果f1大,输出true,否则输出false
///
MyArray a1=new MyArray();
MyArray a2=new MyArray();
ct.input(a1, a2);
System.out.println(ct.compare(a1,a2));
}
//输入对比的对象
void input(Point a,Point b){
a.input(); b.input();
}
void input(Fraction a,Fraction b){
a.input(); b.input();
}
void input(MyArray a,MyArray b){
a.input(); b.input();
}
public boolean compare(Point a,Point b){
if(a.bigTo(b))return true;
else return false;
}
public boolean compare(Fraction f1,Fraction f2){
if(f1.compare(f2)==true)return true;
else return false;
}
public boolean compare(MyArray m1,MyArray m2){
if(MyArray.compare(m1,m2)>=0)return true;
else return false;
}
}
class Point{//创建一个point类
int x,y; //对象x,y
public void input(){
Scanner reader=Main.reader;
x=reader.nextInt(); y=reader.nextInt();//输入x,y
}
public boolean bigTo(Point p){//定义一个bigTo的方法
if(this.x*this.y>=p.x*p.y)return true;
else return false;
}
}
说明1:Fraction类的对象大小比较规则:根据分数值的大小进行比较;MyArray类的对象大小比较规则:认为对应位置大于对方数组元素的个数多于小于对方数组元素个数的那个数组更大,若元素缺失,则补0。
说明2:所有数据都是合法的
说明3:在Fraction类与MyArray类中读入数据时必须使用Main.reader对象作为数据输入对象。
输入
两个点,两个分数,两个数组
输出
三个判断结果
样例输入 Copy
1 2
3 4
1 2
3 4
2
1 2
3
2 1 -1
样例输出 Copy
false
false
true
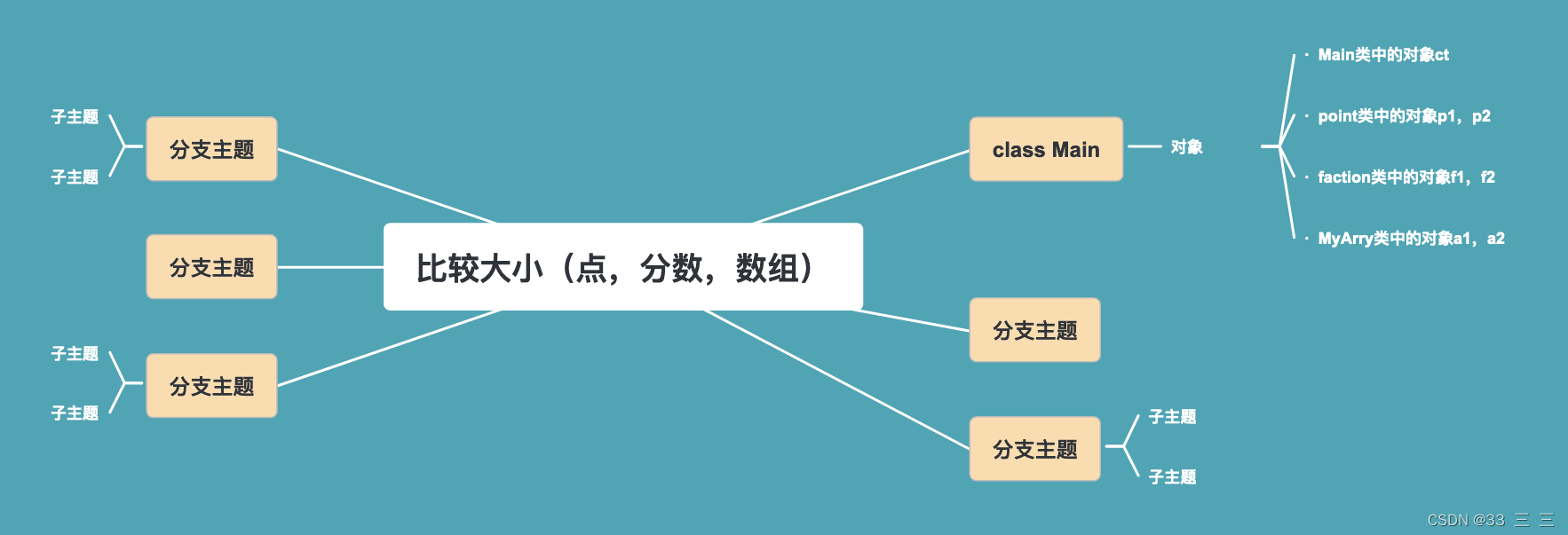
import java.util.Scanner;
public class Main {
public static Scanner reader=new Scanner(System.in);
public static void main(String[] args) {
Main ct=new Main();//新建一个Main对象ct,
Point p1=new Point(),p2=new Point();//定义两个Point型对象
ct.input(p1,p2);//调用构造方法,输入p1和p2对象的成员变量值
System.out.println(ct.compare(p1,p2));//输入对象p1和p2的比较结果,如果p1大,输出true,否则输出false
Fraction f1=new Fraction(),f2=new Fraction();//新建两个Fraction型对象f1,f2;
ct.input(f1,f2);//输入f1,f2对象的成员变量值;
System.out.println(ct.compare(f1,f2));//输入对象f1和f2的比较结果,如果f1大,输出true,否则输出false
MyArray a1=new MyArray();
MyArray a2=new MyArray();
ct.input(a1, a2);
System.out.println(ct.compare(a1,a2));
}
void input(Point a,Point b){
a.input(); b.input();
}
void input(Fraction a,Fraction b){
a.input(); b.input();
}
void input(MyArray a,MyArray b){
a.input(); b.input();
}
public boolean compare(Point a,Point b){
if(a.bigTo(b))return true;
else return false;
}
public boolean compare(Fraction f1,Fraction f2){
if(f1.compare(f2)==true)return true;
else return false;
}
public boolean compare(MyArray m1,MyArray m2){
if(MyArray.compare(m1,m2)>=0)return true;
else return false;
}
}
class Point{//创建一个point类,比较点的大小
int x,y; //对象x,y
public void input(){
Scanner reader=Main.reader;
x=reader.nextInt(); y=reader.nextInt();//输入x,y
}
public boolean bigTo(Point p){//定义一个bigTo的方法
if(this.x*this.y>=p.x*p.y)return true;
else return false;
}
class MyArray{//创建一个MyArray的类,比较数组大小
int [] arr;
public void input(){
Scanner reader=Main.reader;
int n = reader.nextInt();//输入数组元素个数和数组元素
this.arr = new int[n];
for (int i = 0; i < n; i++) {
this.arr[i] = reader.nextInt();
}
}
public static int compare(MyArray p1,MyArray p2){
int cnt1=0,cnt2=0;
int sum = Math.max(p1.arr.length,p2.arr.length);
for (int i = 0; i < sum; i++) {
if(i<p1.arr.length && i<p2.arr.length){
if(p1.arr[i]>p2.arr[i]) cnt1++;
else if(p1.arr[i]<p2.arr[i]) cnt2++;
}
if(i<p1.arr.length && p1.arr[i]>0) cnt1++;
else if(i<p1.arr.length && p1.arr[i]<0) cnt2++;
if(i<p2.arr.length && p2.arr[i]>0) cnt2++;
else if(i<p2.arr.length && p2.arr[i]<0) cnt1++;
}
return (cnt1-cnt2);
}
}
class Fraction{
int son,mom;
public void input(){
Scanner reader=Main.reader;
son=reader.nextInt(); mom=reader.nextInt();
}
public boolean compare(Fraction p){
if((double)this.son/(double)this.mom>(double)p.son/(double)p.mom || Math.abs((double)this.son/(double)this.mom-(double)p.son/(double)p.mom)<1e-6)return true;
else return false;
}
}
二冬奥会自由式滑雪大跳台决赛的计分规则是:六名裁判给分(0-100分,整数分数),去掉一个最高分,去掉一个最低分,取平均值作为(除以4.0)参数选手得分(double类型);每位选手可以出场三次,取最高的两次得分和作为该选手的最终总分。
假设有12名选手参加自由式滑雪大跳台决赛,请你编写Java程序,根据每位选手每次出场的得分,计算选手总分,并按照总分降序排列输出选手的基本信息。如果总分相同,则按姓名升序排序。
说明1:字符串s与t比较大小使用s.compareTo(t),若返回值<0,则s串<t串,若返回值>0,则s串>t串,若返回值为0,则s、t串相等。
说明2:计算每次的平均分时记得除以4.0而不是4,否则会视为整除,无法得到正确的结果
输入
每个选手姓名、国家、三次六位裁判的评分
输出
按选手总分降序输出每个选手的姓名、国家与总分
样例输入 Copy
a5
SUI
92 35 100 7 65 6
65 90 4 78 43 45
61 5 35 51 52 62
a1
DEN
73 54 94 64 39 38
63 35 48 61 91 52
40 68 47 59 82 30
a9
CHN
49 62 78 84 79 37
52 5 38 14 33 39
13 44 13 25 87 42
a12
JPN
61 13 75 51 89 35
89 85 33 6 62 8
60 50 55 54 24 9
a6
USA
99 52 8 48 47 9
85 37 64 22 26 7
36 29 41 67 89 81
a4
GER
5 68 66 34 32 17
48 50 71 51 37 65
59 28 51 28 64 86
a11
USA
64 41 9 14 96 94
29 89 33 20 60 13
22 88 28 36 74 28
a3
AUS
86 48 70 19 1 38
17 14 89 74 81 40
56 39 42 71 47 47
a8
CHN
18 71 46 78 51 23
94 77 85 76 90 7
59 46 66 59 63 29
a10
GBR
6 80 41 64 47 56
0 5 68 77 95 6
85 33 96 97 72 31
a2
ISL
95 96 11 44 12 41
36 6 68 71 89 7
31 41 16 3 82 35
a7
SWE
9 77 79 93 21 93
88 24 92 55 56 56
97 27 27 13 98 15
样例输出 Copy
a8,CHN,138.75
a7,SWE,131.25
a10,GBR,123.5
a1,DEN,113.5
a5,SUI,107.5
a4,GER,104.0
a12,JPN,102.5
a3,AUS,101.0
a9,CHN,98.0
a6,USA,95.25
a11,USA,94.75
a2,ISL,93.5
import java.util.Arrays;
import java.util.Comparator;
import java.util.Scanner;
public class Main{
public static void main(String[] args) {
Player[] cp = new Player[12];
Scanner sc = new Scanner(System.in);
for (int i = 0; i < 12; i++) {
String name = sc.next();
String country = sc.next();
int [] sco = new int[6];
for (int i1 = 0; i1 < 6; i1++) {
sco[i1] = sc.nextInt();
}
Arrays.sort(sco,0, sco.length);
double[] sum = new double[3];
for (int i1 = 1; i1 < 5; i1++) {
sum[0] += (double)sco[i1];
}
sum[0] /= 4.0;
for (int i1 = 0; i1 < 6; i1++) {
sco[i1] = sc.nextInt();
}
Arrays.sort(sco,0, sco.length);
for (int i1 = 1; i1 < 5; i1++) {
sum[1] += (double)sco[i1];
}
sum[1] /= 4.0;
for (int i1 = 0; i1 < 6; i1++) {
sco[i1] = sc.nextInt();
}
Arrays.sort(sco,0, sco.length);
for (int i1 = 1; i1 < 5; i1++) {
sum[2] += (double)sco[i1];
}
sum[2] /= 4.0;
Arrays.sort(sum,0,sum.length);
cp[i] = new Player(name,country,(sum[1]+sum[2]));
}
Arrays.sort(cp, new Comparator<Player>() {
public int compare(Player o1, Player o2) {
if(Math.abs(o1.getScore()-o2.getScore())<1e-6){
return o1.getName().compareTo(o2.getName());//分数相等
}else{
if((o2.getScore()-o1.getScore())>0) return 1;
else if((o2.getScore()-o1.getScore())<0) return -1;
else return 0;
}
}
});
for (int i = 0; i < 12; i++) {
System.out.println(cp[i].getName()+","+cp[i].getCountry()+","+cp[i].getScore());
}
}
}
class Player{
private String name;
private String country;
private double score;
public Player() {
}
public Player(String name, String country, double score) {
this.name = name;
this.country = country;
this.score = score;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
public double getScore() {
return score;
}
public void setScore(double score) {
this.score = score;
}
}
三,某村庄有一口公共水井,为了保护该水井及饮用水的质量,当地规定当该水井内的水量不高于100时,禁止取水6小时,在此期间,水井水量自动恢复到最大容量1000;如果预计村民当次取水后水量低于100,则拒绝该村民本次取水。
其实每个村民自家也有口水井,自家的水井容量各不相同,范围在(100,200]内。村民优先去公共水井取水,若发现公共水井禁止取水或因容量不够拒绝取水时,再从自家水井取水。
自家水井容量不高于100时,禁止取水1小时,在此期间,水井水量自动恢复到初始水量;如果预计村民当次取水后水量低于100,则允许该村民本次从自家水井取水至水井容量到100。
请模拟两个村民用水情况:先设置公共水井水量及两位村民自家水井容量,再模拟村民1取水,村民2取水,村民1再次取水,村民2再次取水的情形。村民每次取水时需输入年、月、日、时、分、秒和取水量,若成功从公共水井取水则输出“public:m”形式,m表示公共水井剩余水量;若成功从自家水井取水则输出“private:n”形式,n表示自家水井剩余水量; 若没有取到水,则输出“wait”。
输入
公共水井初始水量(可以比1000小),两位村民自家水井水量(最大容量,也是初始水量),两位村民两次交替取水情况
输出
每次取水后的情况
样例输入 Copy
1000 150 200
2022 3 1 18 25 30 800
2022 3 1 20 25 20 150
2022 3 1 23 25 19 100
2022 3 2 6 25 25 150
样例输出 Copy
public:200
private:100
public:100
public:850
import java.time.LocalDateTime;
import java.time.ZoneOffset;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Well pub = new Well(sc.nextInt(),6*3600);
Well Vlg1 = new Well(sc.nextInt(),3600);
Well Vlg2 = new Well(sc.nextInt(),3600);
for (int i = 0; i < 4; i++) {
Time t = new Time(sc.nextInt(),sc.nextInt(),sc.nextInt(),sc.nextInt(),sc.nextInt(),sc.nextInt());
int water = sc.nextInt();
if(pub.DrWater(water,t)){
System.out.println("public:"+pub.getNowW());
}else if(i%2==0){
if(Vlg1.DrWater(water,t)){
System.out.println("private:"+Vlg1.getNowW());
}else{
System.out.println("wait");
}
}else{
if(Vlg2.DrWater(water,t)){
System.out.println("private:"+Vlg2.getNowW());
}else{
System.out.println("wait");
}
}
}
}
}
class Well{
private int maxW;
private int nowW;
private int waitTime;
private Time time;
boolean GetWater = true;
public boolean DrWater(int num,Time t){
if(GetWater){
if(nowW-num > 100){
nowW -= num;
return true;
}else if(nowW-num==100){
nowW = 100;
time = t;
GetWater = false;
return true;
}else if(waitTime==3600){
nowW = 100;
time = t;
GetWater = false;
return true;
}
}else if(time.sub(t)>=waitTime){
if(waitTime==3600) {
nowW = maxW;
}else{
nowW = 1000;
}
GetWater=true;
return DrWater(num,t);
}
return false;
}
public Well() {
}
public Well(int maxW, int nowW,int waitTime) {
this.maxW = maxW;
this.nowW = nowW;
this.waitTime = waitTime;
}
public Well(int maxW,int waitTime) {
this.maxW = maxW;
this.nowW = maxW;
this.waitTime = waitTime;
}
public int getMaxW() {
return maxW;
}
public void setTime(Time time) {
this.time = time;
}
public Time getTime() {
return this.time;
}
public void setMaxW(int maxW) {
this.maxW = maxW;
}
public int getNowW() {
return nowW;
}
public void setNowW(int nowW) {
this.nowW = nowW;
}
}
class Time{
private int year=0;
private int month=0;
private int day=0;
private int hour=0;
private int minute=0;
private int second=0;
public Time() {
}
public Time(int year, int month, int day, int hour, int minute, int second) {
this.year = year;
this.month = month;
this.day = day;
this.hour = hour;
this.minute = minute;
this.second = second;
}
public long sub(Time t2){
LocalDateTime dateTime = LocalDateTime.of(this.year, this.month, this.day, this.hour, this.minute, this.second);
long timestamp = dateTime.toEpochSecond(ZoneOffset.UTC);
LocalDateTime dateTime2 = LocalDateTime.of(t2.getYear(), t2.getMonth(), t2.getDay(), t2.getHour(), t2.getMinute(), t2.getSecond() );
long timestamp2 = dateTime2.toEpochSecond(ZoneOffset.UTC);
return timestamp2 - timestamp;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
public int getHour() {
return hour;
}
public void setHour(int hour) {
this.hour = hour;
}
public int getMinute() {
return minute;
}
public void setMinute(int minute) {
this.minute = minute;
}
public int getSecond() {
return second;
}
public void setSecond(int second) {
this.second = second;
}
}
四,一元二次方程的根,在整数范围内无解时,可以扩展到在实数范围内求解,如果在实数范围内无解,还可以扩展到复数范围内求解。请你根据一个一元二次方程的系数,求其在各类数范围内的解。
输入
n和n组一元二次方程的系数(整数)
输出
整数、实数(输出四舍五入保留2位小数)、复数(实部、虚部输出时均四舍五入保留2位小数)范围内的解,有整数解时不必输出实数、复数解,有实数解时不必输出复数解;若两个解相同,只输出1个,若两个解不同,按降序输出,本题定义复数的大小关系时不考虑虚数单位i。
样例输入 Copy
4
1 1 1
1 2 1
1 3 1
1 0 1
样例输出 Copy
Complex:-0.50+0.87i,-0.50-0.87i
Integer:-1
Real:-0.38,-2.62
Complex:i,-i
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
for (int i = 0; i < n; i++) {
Equation f1 = new Equation(sc.nextInt(),sc.nextInt(),sc.nextInt());
f1.getAns();
}
}
}
class Equation{
private int a;
private int b;
private int c;
private double det;
public Equation() {
}
public Equation(int a, int b, int c) {
this.a = a;
this.b = b;
this.c = c;
this.det = 1.0*b*b-4.0*a*c;
}
public void getAns(){
if(a!=0) {
if (det == 0) {
double ans = -1.0 * b / (2.0 * a);
if (ans == Math.round(ans)) {//整数
System.out.printf("Integer:%d\n", Math.round(ans));
} else {
System.out.printf("Real:%.2f\n", ans);
}
} else if (det > 0) {
double ans1 = (-1.0 * b + Math.sqrt(this.det)) / (2.0 * a);
double ans2 = (-1.0 * b - Math.sqrt(this.det)) / (2.0 * a);
if (ans1 == Math.round(ans1) && ans2 == Math.round(ans2)) {//整数
System.out.printf("Integer:%d,%d\n", Math.round(ans1), Math.round(ans2));
} else if (ans1 == Math.round(ans1) && ans2 != Math.round(ans2)) {
System.out.printf("Integer:%d\n", Math.round(ans1));
} else if (ans1 != Math.round(ans1) && ans2 == Math.round(ans2)) {
System.out.printf("Integer:%d\n", Math.round(ans2));
} else {
System.out.printf("Real:%.2f,%.2f\n", ans1, ans2);
}
} else if (det < 0) {
double t = -1.0 * b / (2.0 * a);
double ur = Math.sqrt(4.0 * a * c - 1.0 * b * b) / (2.0 * a);
if (Math.abs(t - 0) < 1e-6 && Math.abs(ur - 1) > 1e-6) {//实部0 虚部不为1
if(ur== Math.round(ur)){
System.out.printf("Complex:%di,-%di\n", Math.round(ur), Math.round(ur));
}else {
System.out.printf("Complex:%.2fi,-%.2fi\n", ur, ur);
}
} else if (Math.abs(t - 0) < 1e-6 && Math.abs(ur - 1) < 1e-6) {//实部0 虚部1
System.out.print("Complex:i,-i\n");
} else if (Math.abs(t - 0) > 1e-6 && Math.abs(ur - 1) < 1e-6) {//实部不为0 虚部1
if(t==Math.round(t)){
System.out.printf("Complex:%d+i,%d-i\n", Math.round(t), Math.round(t));
}else {
System.out.printf("Complex:%.2f+i,%.2f-i\n", t, t);
}
} else if(t==Math.round(t) && ur!=Math.round(ur)){
System.out.printf("Complex:%d+%.2fi,%d-%.2fi\n", Math.round(t), ur, Math.round(t), ur);
}else if(t!=Math.round(t) && ur==Math.round(ur)){
System.out.printf("Complex:%.2f+%di,%.2f-%di\n", t, Math.round(ur), t, Math.round(ur));
}else if(t==Math.round(t) && ur==Math.round(ur)){
System.out.printf("Complex:%d+%di,%d-%di\n", Math.round(t), Math.round(ur), Math.round(t), Math.round(ur));
}else{
System.out.printf("Complex:%.2f+%.2fi,%.2f-%.2fi\n", t, ur, t, ur);
}
}
}else{
double ans = -1.0*c/b;
if(ans == Math.round(ans)){
System.out.printf("Integer:%d\n",Math.round(ans));
}else{
System.out.printf("Real:%.2f\n",ans);
}
}
}
public int getA() {
return a;
}
public void setA(int a) {
this.a = a;
}
public int getB() {
return b;
}
public void setB(int b) {
this.b = b;
}
public int getC() {
return c;
}
public void setC(int c) {
this.c = c;
}
//ax^2 + bx + c = 0;
}
五,定义一个小车类Car,其初始位置为(0,0),属性包括:当前位置,行驶速度,行驶朝向(东1、南2、西3、北4);方向变化:直行(1)、左转(2)、右转(3);速度变化(正数为加速,负数为减速)。
约束条件:(1)采用直角坐标系,上北下南左西右东;(2)速度为0时,车辆停止,减速无效;(3)速度达到20时,加速无效。
输入
小车的初始位置(x,y)、初始速度(v)和初始朝向(f),时刻数量(<=1000),每个时刻内的方向变化(c)、速度变化(s)
输出
当前小车的位置
样例输入 Copy
0 0 0 1
10
1 1 1 1 1 1 1 0 1 0 1 0 2 0 1 0 1 2 1 -1
样例输出 Copy
15,15
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner reader = new Scanner(System.in);
Car a = new Car(reader.nextInt(),reader.nextInt(),reader.nextInt(),reader.nextInt());
int n=reader.nextInt();
for (int i = 0; i <n; i++) {
a.changeDirection(reader.nextInt());
a.changV(reader.nextInt());
a.changXY();
}
a.Print();
}
}
class Car{
private int x;
private int y;
private int v;
private int d;
public Car(){
}
public Car(int x,int y,int v,int d){
this.x=x;
this.y=y;
this.v=v;
this.d=d;
}
public void setX(int x) {
this.x = x;
}
public void setY(int y) {
this.y = y;
}
public void setV(int v) {
this.v = v;
}
public void setD(int d) {
this.d = d;
}
public void changeDirection(int d){
if(d==2){
if(this.d==1) {
this.d = 4;
}
else if(this.d==2){
this.d=1;
}
else if(this.d==3){
this.d=2;
}
else if(this.d==4){
this.d=3;
}
}
if(d==3){
if(this.d==1){
this.d=2;
}
else if(this.d==2) {
this.d = 3;
}
else if(this.d==3){
this.d=4;
}
else if(this.d==4){
this.d=1;
}
}
}
public void changV(int cv){
v+=cv;
if(v>20){
v=20;
}
if(v<0){
v=0;
}
}
public void changXY(){
if(d==1){
x+=v;
}
if(d==3){
x-=v;
}
if(d==4){
y+=v;
}
if(d==2){
y-=v;
}
}
public void Print(){
System.out.println(x+","+y);
}
}