演讲比赛流程管理系统
1.比赛规则
- 学校举办一个演讲比赛,一共12人参加。比赛有两轮,分别为淘汰赛和决赛。
- 每位选手都有对应的 10001 ~ 10012 的选手编号
- 比赛方式:分组比赛,每组 6 个人
- 第一轮分为两个小组,整体按照选手编号 进行抽签后顺序演讲
- 十个评委给选手打分(这个过程生成随机数来模拟),除去最高分和最低分,平均分为选手的成绩
- 当小组演讲完之后,淘汰赛组内的前三名晋级。决赛前三名胜出,根据最终成绩决出冠军,亚军,季军
- 每轮比赛过后需要显示晋级选手的信息
2.程序功能
3.功能代码
1. 菜单功能
void SpeechManager::show_menu() {
cout << "*************************************************" << endl;
cout << "****************欢迎参加演讲比赛*****************" << endl;
cout << "****************1.开始参加演讲比赛***************" << endl;
cout << "****************2.查看往届比赛记录***************" << endl;
cout << "****************3.清空比赛记录*******************" << endl;
cout << "****************0.退出比赛程序*******************" << endl;
cout << "*************************************************" << endl;
cout << endl;
}
2. 退出功能
void SpeechManager::exitSystem() {
cout << "欢迎下次使用" << endl;
system("pause");
exit(0);
}
3. 创建选手类 Speaker
class Speaker {
public:
string name;
double score[2];
};
4. 添加成员属性 和 函数
class SpeechManager {
public:
SpeechManager();
//菜单功能
void show_menu();
//退出功能
void exitSystem();
//初始化
void initSpeech();
//创建12名选手
void createSpeaker();
//开始比赛
void startSpeech();
//抽签
void speechDraw();
//比赛
void speechContest();
//显示晋级的信息
void showScore();
//保存记录
void saveRecord();
//读取记录
void readRecord();
//显示往届记录
void showRecord();
//清空记录
void clearRecord();
~SpeechManager();
//文件为空 或者 不存在 的标志
bool fileIsEmpty;
//存放往届比赛记录
map<int, vector<string>> m_record;
//存放第一轮选手的编号
vector<int> v1;
//存放第二轮选手的编号
vector<int> v2;
//存放三名胜出者编号
vector<int> victory;
//保存 选手编号 对应的 选手
map<int, Speaker> m_speaker;
//比赛轮数
int m_idx;
};
5. 创建选手
//创建12名选手
void SpeechManager::createSpeaker() {
string candidate = "ABCDEFGHIJKL";
int len = candidate.size();
//编号
int id = 10001;
//选手名称
string name = "选手";
for (int i = 0; i < len; i++) {
//创建选手
Speaker speaker;
speaker.name = name + candidate[i];
//选手分数
for (int j = 0; j < 2; j++) {
speaker.score[j] = 0;
}
//选手编号存入v1
this->v1.push_back(id + i);
//选手编号 与 对应选手 存入map
this->m_speaker.insert(make_pair(id + i, speaker));
}
}
6. 比赛
1.抽签
//抽签
void SpeechManager::speechDraw() {
cout << "<< 第" << this->m_idx << "轮抽签 >>" << endl;
cout << "------------------------------------------------" << endl;
if (this->m_idx == 1) {
//第一轮抽签
random_shuffle(this->v1.begin(), this->v1.end());
for (auto it = this->v1.begin(); it != this->v1.end(); it++) {
cout << *it << " ";
}
cout << endl;
}
else {
//第二轮抽签
random_shuffle(this->v2.begin(), this->v2.end());
for (auto it = this->v2.begin(); it != this->v2.end(); it++) {
cout << *it << " ";
}
cout << endl;
}
cout << "------------------------------------------------" << endl;
system("pause");
cout << endl;
}
2.比赛
//比赛
void SpeechManager::speechContest() {
//设置随机数种子
srand((unsigned int)time(nullptr));
//存放选手编号
vector<int> v;
//存放6人一组的选手编号 和 成绩
multimap<double, int, greater<double>> groupScore;
cout << "--------------------------------- 第 " << this->m_idx << " 轮比赛开始 ---------------------------------" << endl;
//判断是第 几 轮比赛
if (this->m_idx == 1) {
v = v1;
}
else {
v = v2;
}
//统计已经打分的人数 便于分组
int num = 0;
for (auto it = v.begin(); it != v.end(); it++) {
num++;
//记录10位评委 对于 选手的打分(去掉了最高分 和 最低分)
deque<double> d;
for (int j = 0; j < 10; j++) {
//评委打分的 范围是 60.0~100.0
double score = (rand() % 401 + 600) / 10.0;
d.push_back(score);
}
//分数按照从大到小排序 方便去掉最高分 和 最低分
sort(d.begin(), d.end(), greater<double>());
//求平均分
double sum = accumulate(d.begin(), d.end(), 0.0);
double avg = sum / (double)d.size();
this->m_speaker[*it].score[this->m_idx - 1] = avg;
groupScore.insert(make_pair(avg, *it));
//已经有6个人打分了 该6个人即为一个小组
if (num % 6 == 0) {
cout << "第 " << num / 6 << " 小组成员得分情况:" << endl;
for (auto it = groupScore.begin(); it != groupScore.end(); it++) {
cout << "选手编号 : " << it->second << " 选手姓名 : " << this->m_speaker[it->second].name << " 选手分数 : " << it->first << endl;
}
cout << endl;
int cnt = 0;
if (this->m_idx == 1) {
for (auto it = groupScore.begin(); it != groupScore.end() && cnt < 3; it++) {
v2.push_back(it->second);
cnt++;
}
}
else {
for (auto it = groupScore.begin(); it != groupScore.end() && cnt < 3; it++) {
victory.push_back(it->second);
cnt++;
}
}
//清空groupScore
groupScore.clear();
}
}
cout << "--------------------------------- 第 " << this->m_idx << " 轮比赛结束 ---------------------------------" << endl;
system("pause");
}
3.显示晋级信息
//显示晋级的信息
void SpeechManager::showScore() {
cout << "--------------------------------- 第 " << this->m_idx << " 轮晋级结果信息如下 ---------------------------------" << endl;
vector<int> v;
if (this->m_idx == 1) v = this->v2;
else v = this->victory;
//输出结果信息
for (auto it = v.begin(); it != v.end(); it++) {
cout << "选手编号 : " << *it << " 选手姓名 : " << this->m_speaker[*it].name << " 选手得分 : " << this->m_speaker[*it].score[this->m_idx - 1] << endl;
}
system("pause");
//this->show_menu();
}
4.保存记录
//保存记录
void SpeechManager::saveRecord() {
ofstream ofs;
ofs.open("speech.csv", ios::out | ios::app);
for (auto it = this->victory.begin(); it != this->victory.end(); it++) {
ofs << *it << "," << this->m_speaker[*it].score[1] << ",";
}
ofs << endl;
ofs.close();
cout << "记录保存完毕" << endl;
this->fileIsEmpty = false;
}
5.开始比赛
//开始比赛
void SpeechManager::startSpeech() {
//第一轮抽签
this->speechDraw();
//第一轮比赛
this->speechContest();
//显示晋级信息
this->showScore();
//第二轮比赛
this->m_idx++;
//第二轮抽签
this->speechDraw();
//第二轮比赛
this->speechContest();
//显示晋级信息
this->showScore();
//保存记录
this->saveRecord();
//重置比赛
this->initSpeech();
this->createSpeaker();
this->readRecord();
cout << "本届比赛完毕!!!" << endl;
system("pause");
system("cls");
}
7. 查看记录
1.读取记录
//读取记录
void SpeechManager::readRecord() {
ifstream ifs;
ifs.open("speech.csv", ios::in);
//文件不存在
if (!ifs.is_open()) {
this->fileIsEmpty = true;
//cout << "文件不存在" << endl;
ifs.close();
return;
}
//文件内容为空
char c;
ifs >> c;
if (ifs.eof()) {
this->fileIsEmpty = true;
//cout << "文件内容为空" << endl;
ifs.close();
return;
}
//正常情况
ifs.putback(c);
this->fileIsEmpty = false;
string data;
int index = 0;
while (ifs >> data) {
vector<string> v;
int pos = -1;
int start = 0;
while (true) {
pos = data.find(",",start);
if (pos == -1) break;
string s = data.substr(start, pos - start);
v.push_back(s);
start = pos + 1;
}
this->m_record.insert(make_pair(index, v));
index++;
}
cout << endl;
ifs.close();
}
2.显示往届信息
//显示往届记录
void SpeechManager::showRecord() {
if (this->fileIsEmpty) {
cout << "文件为空或者不存在" << endl;
}
else {
int n = this->m_record.size();
for (int i = 0; i < n; i++) {
cout << "第" << i + 1 << "届比赛结果:"
<< " 冠军编号: " << this->m_record[i][0] << " 冠军成绩: " << this->m_record[i][1]
<< " 亚军编号: " << this->m_record[i][2] << " 亚军成绩: " << this->m_record[i][3]
<< " 季军编号: " << this->m_record[i][4] << " 季军成绩: " << this->m_record[i][5] << endl;
}
}
system("pause");
system("cls");
}
```
### 8.清空记录
```cpp
//清空记录
void SpeechManager::clearRecord() {
cout << "请确认是否清空文件内容" << endl;
cout << "1、是" << endl;
cout << "2、否" << endl;
int choice = 0;
cin >> choice;
if (choice == 1) {
//清空文件
ofstream ofs("speech.csv", ios::trunc);
ofs.close();
this->initSpeech();
this->createSpeaker();
this->readRecord();
cout << "清空成功!!!" << endl;
}
system("pause");
system("cls");
}
```
## 4. 完整代码
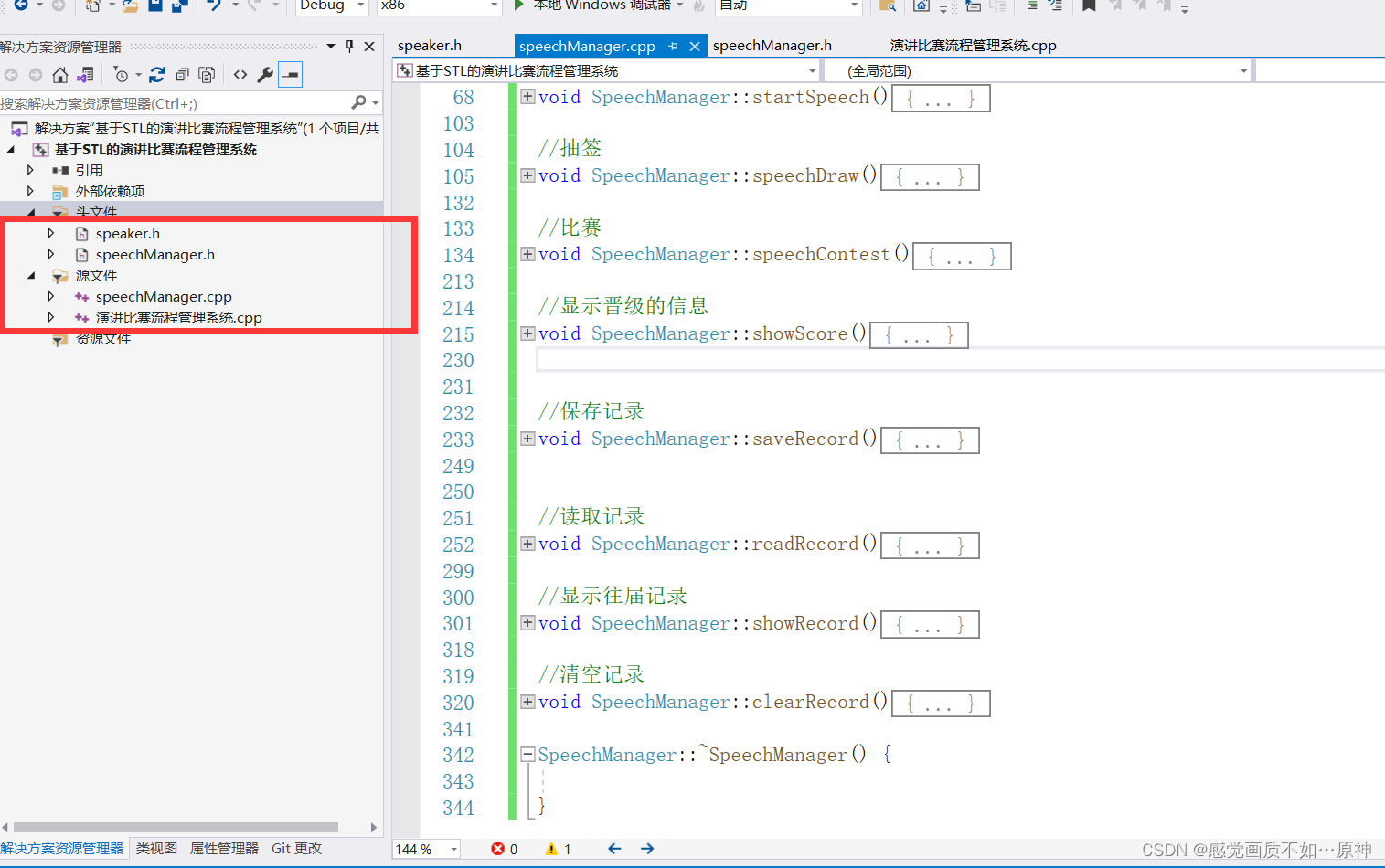
### 4.1 speaker.h
```cpp
#pragma once
#include<iostream>
using namespace std;
class Speaker {
public:
string name;
double score[2];
};
```
### 4.2 speechManager.h
```cpp
#pragma once
#include<iostream>
#include<vector>
#include<map>
#include<deque>
#include<functional>
#include<numeric>
#include<algorithm>
#include<fstream>
using namespace std;
#include"speaker.h"
class SpeechManager {
public:
SpeechManager();
//菜单功能
void show_menu();
//退出功能
void exitSystem();
//初始化
void initSpeech();
//创建12名选手
void createSpeaker();
//开始比赛
void startSpeech();
//抽签
void speechDraw();
//比赛
void speechContest();
//显示晋级的信息
void showScore();
//保存记录
void saveRecord();
//读取记录
void readRecord();
//显示往届记录
void showRecord();
//清空记录
void clearRecord();
~SpeechManager();
//文件为空 或者 不存在 的标志
bool fileIsEmpty;
//存放往届比赛记录
map<int, vector<string>> m_record;
//存放第一轮选手的编号
vector<int> v1;
//存放第二轮选手的编号
vector<int> v2;
//存放三名胜出者编号
vector<int> victory;
//保存 选手编号 对应的 选手
map<int, Speaker> m_speaker;
//比赛轮数
int m_idx;
};
```
### 4.3 speechManager.cpp
```cpp
#include"speechManager.h"
SpeechManager::SpeechManager() {
this->initSpeech();
this->createSpeaker();
this->readRecord();
}
void SpeechManager::show_menu() {
cout << "*************************************************" << endl;
cout << "****************欢迎参加演讲比赛*****************" << endl;
cout << "****************1.开始参加演讲比赛***************" << endl;
cout << "****************2.查看往届比赛记录***************" << endl;
cout << "****************3.清空比赛记录*******************" << endl;
cout << "****************0.退出比赛程序*******************" << endl;
cout << "*************************************************" << endl;
cout << endl;
}
void SpeechManager::exitSystem() {
cout << "欢迎下次使用" << endl;
system("pause");
exit(0);
}
//初始化
void SpeechManager::initSpeech() {
this->v1.clear();
this->v2.clear();
this->victory.clear();
this->m_speaker.clear();
this->m_record.clear();
//比赛轮数初始化为第一轮
this->m_idx = 1;
}
//创建12名选手
void SpeechManager::createSpeaker() {
string candidate = "ABCDEFGHIJKL";
int len = candidate.size();
//编号
int id = 10001;
//选手名称
string name = "选手";
for (int i = 0; i < len; i++) {
//创建选手
Speaker speaker;
speaker.name = name + candidate[i];
//选手分数
for (int j = 0; j < 2; j++) {
speaker.score[j] = 0;
}
//选手编号存入v1
this->v1.push_back(id + i);
//选手编号 与 对应选手 存入map
this->m_speaker.insert(make_pair(id + i, speaker));
}
}
//开始比赛
void SpeechManager::startSpeech() {
//第一轮抽签
this->speechDraw();
//第一轮比赛
this->speechContest();
//显示晋级信息
this->showScore();
//第二轮比赛
this->m_idx++;
//第二轮抽签
this->speechDraw();
//第二轮比赛
this->speechContest();
//显示晋级信息
this->showScore();
//保存记录
this->saveRecord();
//重置比赛
this->initSpeech();
this->createSpeaker();
this->readRecord();
cout << "本届比赛完毕!!!" << endl;
system("pause");
system("cls");
}
//抽签
void SpeechManager::speechDraw() {
cout << "<< 第" << this->m_idx << "轮抽签 >>" << endl;
cout << "------------------------------------------------" << endl;
if (this->m_idx == 1) {
//第一轮抽签
random_shuffle(this->v1.begin(), this->v1.end());
for (auto it = this->v1.begin(); it != this->v1.end(); it++) {
cout << *it << " ";
}
cout << endl;
}
else {
//第二轮抽签
random_shuffle(this->v2.begin(), this->v2.end());
for (auto it = this->v2.begin(); it != this->v2.end(); it++) {
cout << *it << " ";
}
cout << endl;
}
cout << "------------------------------------------------" << endl;
system("pause");
cout << endl;
}
//比赛
void SpeechManager::speechContest() {
//设置随机数种子
srand((unsigned int)time(nullptr));
//存放选手编号
vector<int> v;
//存放6人一组的选手编号 和 成绩
multimap<double, int, greater<double>> groupScore;
cout << "--------------------------------- 第 " << this->m_idx << " 轮比赛开始 ---------------------------------" << endl;
//判断是第 几 轮比赛
if (this->m_idx == 1) {
v = v1;
}
else {
v = v2;
}
//统计已经打分的人数 便于分组
int num = 0;
for (auto it = v.begin(); it != v.end(); it++) {
num++;
//记录10位评委 对于 选手的打分(去掉了最高分 和 最低分)
deque<double> d;
for (int j = 0; j < 10; j++) {
//评委打分的 范围是 60.0~100.0
double score = (rand() % 401 + 600) / 10.0;
d.push_back(score);
}
//分数按照从大到小排序 方便去掉最高分 和 最低分
sort(d.begin(), d.end(), greater<double>());
//求平均分
double sum = accumulate(d.begin(), d.end(), 0.0);
double avg = sum / (double)d.size();
this->m_speaker[*it].score[this->m_idx - 1] = avg;
groupScore.insert(make_pair(avg, *it));
//已经有6个人打分了 该6个人即为一个小组
if (num % 6 == 0) {
cout << "第 " << num / 6 << " 小组成员得分情况:" << endl;
for (auto it = groupScore.begin(); it != groupScore.end(); it++) {
cout << "选手编号 : " << it->second << " 选手姓名 : " << this->m_speaker[it->second].name << " 选手分数 : " << it->first << endl;
}
cout << endl;
int cnt = 0;
if (this->m_idx == 1) {
for (auto it = groupScore.begin(); it != groupScore.end() && cnt < 3; it++) {
v2.push_back(it->second);
cnt++;
}
}
else {
for (auto it = groupScore.begin(); it != groupScore.end() && cnt < 3; it++) {
victory.push_back(it->second);
cnt++;
}
}
//清空groupScore
groupScore.clear();
}
}
cout << "--------------------------------- 第 " << this->m_idx << " 轮比赛结束 ---------------------------------" << endl;
system("pause");
}
//显示晋级的信息
void SpeechManager::showScore() {
cout << "--------------------------------- 第 " << this->m_idx << " 轮晋级结果信息如下 ---------------------------------" << endl;
vector<int> v;
if (this->m_idx == 1) v = this->v2;
else v = this->victory;
//输出结果信息
for (auto it = v.begin(); it != v.end(); it++) {
cout << "选手编号 : " << *it << " 选手姓名 : " << this->m_speaker[*it].name << " 选手得分 : " << this->m_speaker[*it].score[this->m_idx - 1] << endl;
}
system("pause");
//this->show_menu();
}
//保存记录
void SpeechManager::saveRecord() {
ofstream ofs;
ofs.open("speech.csv", ios::out | ios::app);
for (auto it = this->victory.begin(); it != this->victory.end(); it++) {
ofs << *it << "," << this->m_speaker[*it].score[1] << ",";
}
ofs << endl;
ofs.close();
cout << "记录保存完毕" << endl;
this->fileIsEmpty = false;
}
//读取记录
void SpeechManager::readRecord() {
ifstream ifs;
ifs.open("speech.csv", ios::in);
//文件不存在
if (!ifs.is_open()) {
this->fileIsEmpty = true;
//cout << "文件不存在" << endl;
ifs.close();
return;
}
//文件内容为空
char c;
ifs >> c;
if (ifs.eof()) {
this->fileIsEmpty = true;
//cout << "文件内容为空" << endl;
ifs.close();
return;
}
//正常情况
ifs.putback(c);
this->fileIsEmpty = false;
string data;
int index = 0;
while (ifs >> data) {
vector<string> v;
int pos = -1;
int start = 0;
while (true) {
pos = data.find(",",start);
if (pos == -1) break;
string s = data.substr(start, pos - start);
v.push_back(s);
start = pos + 1;
}
this->m_record.insert(make_pair(index, v));
index++;
}
cout << endl;
ifs.close();
}
//显示往届记录
void SpeechManager::showRecord() {
if (this->fileIsEmpty) {
cout << "文件为空或者不存在" << endl;
}
else {
int n = this->m_record.size();
for (int i = 0; i < n; i++) {
cout << "第" << i + 1 << "届比赛结果:"
<< " 冠军编号: " << this->m_record[i][0] << " 冠军成绩: " << this->m_record[i][1]
<< " 亚军编号: " << this->m_record[i][2] << " 亚军成绩: " << this->m_record[i][3]
<< " 季军编号: " << this->m_record[i][4] << " 季军成绩: " << this->m_record[i][5] << endl;
}
}
system("pause");
system("cls");
}
//清空记录
void SpeechManager::clearRecord() {
cout << "请确认是否清空文件内容" << endl;
cout << "1、是" << endl;
cout << "2、否" << endl;
int choice = 0;
cin >> choice;
if (choice == 1) {
//清空文件
ofstream ofs("speech.csv", ios::trunc);
ofs.close();
this->initSpeech();
this->createSpeaker();
this->readRecord();
cout << "清空成功!!!" << endl;
}
system("pause");
system("cls");
}
SpeechManager::~SpeechManager() {
}
```
### 4.4 演讲比赛流程管理系统.cpp
```cpp
#include<iostream>
using namespace std;
#include"speechManager.h"
int main() {
SpeechManager sm;
/*for (auto it = sm.m_speaker.begin(); it != sm.m_speaker.end(); it++) {
cout << "选手编号 : " << it->first << " 选手姓名 : " << it->second.name << " 选手分数 : " << it->second.score[0] << endl;
}*/
int choice;
while (true) {
sm.show_menu();
cout << "请输入您的选择:" << endl;
cin >> choice;
switch (choice) {
case 1: //开始演讲比赛
sm.startSpeech();
break;
case 2: //查看往届比赛记录
sm.showRecord();
break;
case 3: //清空比赛记录
sm.clearRecord();
break;
case 0: //退出比赛程序
sm.exitSystem();
break;
default:
system("cls");
break;
}
}
system("pause");
return 0;
}
```