p136 this 原理部分
p137 this关键字
p138 this关键字
p139 this关键字
package com.bjpowernode.javase.test003;
/**
* 最终结论:
* 在带有static的方法中,不能直接访问实例变量和实例方法。
* 因为实例变量和实例方法需要对象的存在。
* 而static的方法中是没有this的,也就是说当前对象是不存在的
* 自然也就无法访问当前对象的实例变量和实例方法
*
* @author ZhongjieMa
*
*/
public class ThisTest {
//带有static,主方法
public static void main(String[] args) {
//调用doSome,类名.方法名
ThisTest.doSome();
//或者方法名
doSome();
//调用doOther方法,引用.方法名
//当前对象.doOther();//编译出错
//实例方法调用必须要有对象存在
//以下代码表示的含义:调用当前对象的doOther方法
//但是由于main方法中没有this,所以以下方法不能调用
//doOther();
//ThisTest.doOther();
//解决方法
ThisTest t = new ThisTest();
t.doOther();
//调用run,实例方法。引用.方法名
t.run();
}
//带有static
public static void doSome() {
System.out.println("doSome");
}
//不带static,实例方法
public void doOther() {
//this表示当前对象
System.out.println("do Other");
}
//实例方法
public void run() {
//在大括号中的代码执行过程中一定是有对象被创建出来的
//也就是说这里一定有this的存在
System.out.println("run execute!");
//doOther是一个实例方法,实例方法调用必须要有对象的存在
//以下代码表示的含义就是:调用当前对象的doOther方法
//
doOther();//为什么此处可以调用实例方法?
this.doOther();
}
}
package com.bjpowernode.javase.test003;
public class ThisTest2 {
//实例变量
String name;
//实例方法
public void doSome() {
}
//带有static的主方法中无法直接通过this访问以上的实例变量和实例方法
public static void main(String[] args) {
//这里没有this
//编译错误
//doSome();
//System.out.println(name);
//解决方法
ThisTest2 tt = new ThisTest2();
tt.name = "马仲杰";
tt.doSome();
System.out.println(tt.name);
}
}
p140 this什么时候不能省略
package com.bjpowernode.javase.test003;
public class UserTest {
public static void main(String[] args) {
User u = new User(1056,"马仲杰");
System.out.println(u.getName());
System.out.println(u.getId());
//重新设置姓名
u.setId(1055);
u.setName("哈金玮");
System.out.println(u.getName());
System.out.println(u.getId());
//使用缺省构造器创建对象,会赋默认值
User u1 = new User();
System.out.println(u1.getName());
System.out.println(u1.getId());
}
}
p141 this最后一个语法
- Date.java
package com.bjpowernode.javase.test004;
public class Date {
//属性
private int year;
private int month;
private int day;
public int getYear() {
return year;
}
//setter和getter
public void setYear(int year) {
this.year = year;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
//无参数构造器
public Date() {
/**
* 这样写的话,与下面的带参数构造器中的代码重复了
* 在这个无参数构造器中可以使用this关键字调用下面的带参数构造器
this.year = 1997;
this.month = 4;
this.day = 10;
**/
this(1997,4,11);//记住就行。
}
//带参数构造器
public Date(int year, int month, int day) {
this.year = year;
this.month = month;
this.day = day;
}
//打印方法。不带static,是实例方法
public void print() {
System.out.println(this.year+"年"+this.month+"月"+this.day+"日");
}
}
- DateTest.java
package com.bjpowernode.javase.test004;
public class DateTest {
public static void main(String[] args) {
// 创建对象Date1
Date time1 = new Date();
time1.print();
Date time2 = new Date(2020,12,11);
time2.print();
}
}
p143 this 综合例子
package com.bjpowernode.javase.test005;
public class Test {
//带有static的方法
public static void method1() {
//要求在这里编写程序调用doSome
//完整方式
Test.doSome();
//省略方式
doSome();
//要求在这里编写程序调用doOther
//完整方式
Test t = new Test();
t.doOther();
//无省略方式
//访问i,i是实例变量
//完整方式
System.out.println(t.i);
//无省略方式
}
//不带static的方法
public void method2() {
//要求在这里编写程序调用doSome
//完整方式
Test.doSome();
//省略方式
doSome();
//要求在这里编写程序调用doOther
//完整方式
this.doOther();
//省略方式
doOther();
//访问i
//完整方式
System.out.println(this.i);
//省略方式
System.out.println(i);
}
//主方法
public static void main(String[] args) {
//要求在这里编写程序调用method1
//完整方式
Test.method1();
//省略方式
method1();
//要求在这里编写程序调用method2
//完整方式
Test t = new Test();
t.method2();
//无省略方式
}
//没有static的变量
int i = 10;
//带有static的方法
public static void doSome() {
System.out.println("do some");
}
//没有static的方法
//不带static的方法中有this关键字
public void doOther() {
System.out.println("do other");
}
}
p144 带static的方法
- 既可以用类名.方法名的方式访问,也可以通过引用.方法名的方式访问
p145 static关键字
使用实例变量定义chinese
package com.bjpowernode.javase.test006;
/**
* 定义一个中国人类.使用实例变量
* @author ZhongjieMa
*
*/
public class Chinese {
//身份证号,每一个对象的身份证号都不一样
String id;
//姓名,灭一个对象的姓名不同
String name;
//国籍,每一个对象的国籍一样
//无论通过Chinese创建多少个对象,这些对象的国籍都是“中国”
//实例变量,【实例变量是一个对象就有一份,100个对象就有100个country】
//实例变量存储在java对象内部,在堆内存中,在构造方法执行的时候初始化
//
String country;
//构造方法,无参数
public Chinese() {
/**
* this.id = 0;
* this.name = null;
* this.country = Chinese;
*/
}
public Chinese(String id, String name, String country) {
this.id = id;
this.name = name;
this.country = country;
}
}
package com.bjpowernode.javase.test006;
public class ChineseTest {
public static void main(String[] args) {
//创建中国人对象1
Chinese zhangsan = new Chinese("1","张三","Chinese");
System.out.println(zhangsan.id+","+zhangsan.name+","+zhangsan.country);
//创建中国人对象2
Chinese lisi = new Chinese("2","李四","Chinese");
System.out.println(lisi.id+","+lisi.name+","+lisi.country);
}
}
使用静态变量定义Chinese
package com.bjpowernode.javase.test007.copy;
/**
* 定义一个中国人类,使用静态变量
* @author ZhongjieMa
*
*/
public class Chinese {
//身份证号,每一个对象的身份证号都不一样
String id;
//姓名,灭一个对象的姓名不同
String name;
//国籍,每一个对象的国籍一样
//无论通过Chinese创建多少个对象,这些对象的国籍都是“中国”
//实例变量,【实例变量是一个对象就有一份,100个对象就有100个country】
//实例变量存储在java对象内部,在堆内存中,在构造方法执行的时候初始化
//
static String country = "Chinese";
//构造方法,无参数
public Chinese() {
/**
* this.id = 0;
* this.name = null;
* this.country = Chinese;
*/
}
public Chinese(String id, String name) {
this.id = id;
this.name = name;
}
}
package com.bjpowernode.javase.test007.copy;
public class ChineseTest {
public static void main(String[] args) {
//创建中国人对象1
Chinese zhangsan = new Chinese("1","张三");
System.out.println(zhangsan.id+","+zhangsan.name+","+Chinese.country);
//创建中国人对象2
Chinese lisi = new Chinese("2","李四");
System.out.println(lisi.id+","+lisi.name+","+Chinese.country);
}
}
static定义静态代码块
public class StaticTest01 {
static {
System.out.println("类加载-->1");
System.out.println("类加载-->2");
System.out.println("类加载-->3");
}
public static void main(String[] args) {
System.out.println("main begin");
}
}
p147 实例代码块
package com.bjpowernode.javase.test009;
/**
* 实例代码块【了解内容】
* 1、实例代码块可以编写多个,自上而下执行
* 2、实例代码块在构造方法执行之前执行,构造方法执行一次,实例代码块执行一次
* 3、实例代码块也是java为程序员准备的一个时机,叫作:对象初始化时机
*
* @author ZhongjieMa
*
*/
public class staticTest {
//构造函数
public staticTest(){
System.out.println("缺省构造器执行");
}
{
System.out.println(1);
}
{
System.out.println(2);
}
{
System.out.println(3);
}
public static void main(String[] args) {
System.out.println("main begin");
staticTest st = new staticTest();
staticTest st2 = new staticTest();
}
}
p148 static 总结
package com.bjpowernode.javase.test009;
public class MainTest {
public static void main(String[] args) {
main(20);
main("Hello World");
}
public static void main(int i) {
System.out.println(i);
}
public static void main(String args) {
System.out.println(args);
}
}
package com.bjpowernode.javase.test010;
public class staticTest {
//实例变量
int i = 10;
//实例方法
public void doSome() {
System.err.println("调用实例方法");
}
public static void main(String[] args) {
//无法方法实例对象
//System.out.println(i);
//doSome();
//要想访问实例对象,需要先创建对象
staticTest st = new staticTest();
System.out.println(st.i);
st.doSome();
}
}
p149继承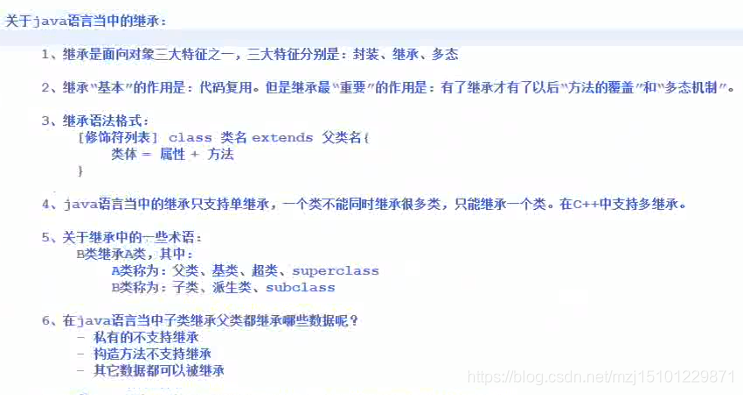
方法重写
p150方法的覆盖
回顾方法重载:
方法覆盖建议使用使用工具生成