java反射操作注解
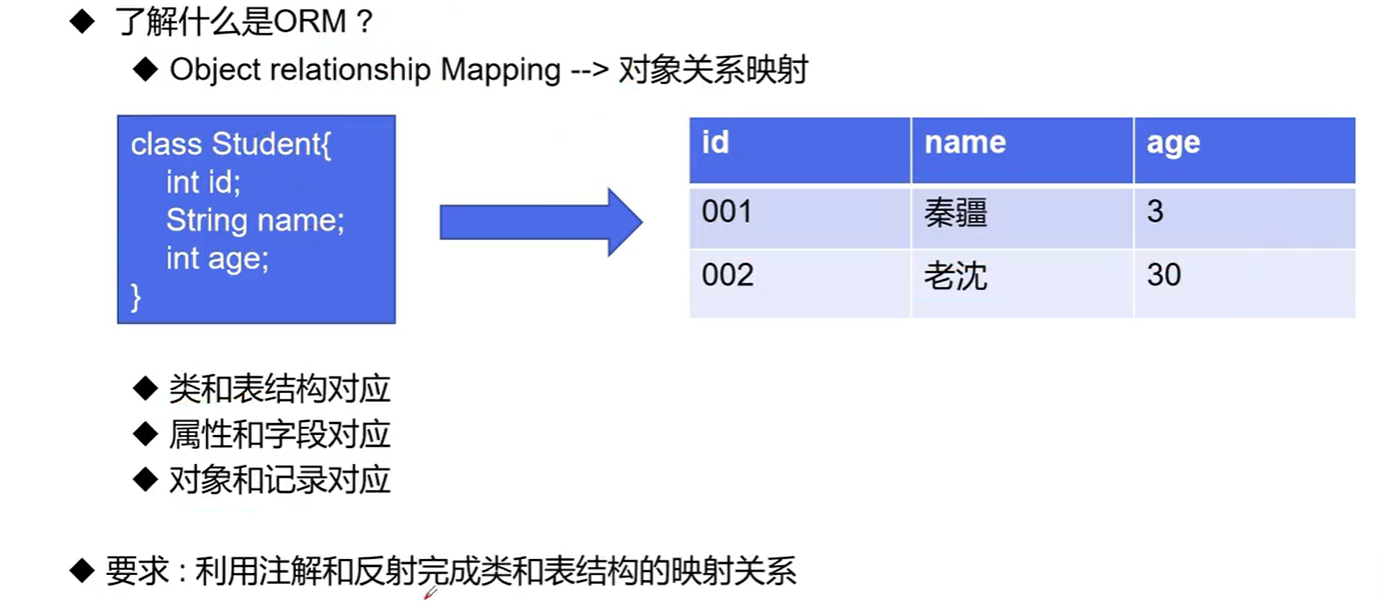
package day10;
import java.lang.annotation.*;
import java.lang.reflect.Field;
public class ReflectionTest4 {
public static void main(String[] args) throws ClassNotFoundException, NoSuchFieldException {
Class aClass = Class.forName("day10.People");
Annotation[] annotations = aClass.getAnnotations();
for (Annotation annotation : annotations) {
System.out.println(annotation);
}
table c1 = (table)aClass.getAnnotation(table.class);
System.out.println(c1.value());
Field name = aClass.getDeclaredField("id");
FiledAnnotation annotation = (FiledAnnotation)name.getAnnotation(FiledAnnotation.class);
System.out.println(annotation.filedName());
System.out.println(annotation.filedType());
System.out.println(annotation.length());
}
}
@table("people_table")
class People{
@FiledAnnotation(filedName = "id",filedType = "int",length = 10)
int id;
@FiledAnnotation(filedName = "age",filedType = "int",length = 10)
int age;
@FiledAnnotation(filedName = "name",filedType = "int",length = 10)
String name;
public People() {
}
public People(int id, int age, String name) {
this.id = id;
this.age = age;
this.name = name;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "People{" +
"id=" + id +
", age=" + age +
", name='" + name + '\'' +
'}';
}
}
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
@interface table{
String value();
}
@Target(ElementType.FIELD)
@Retention(RetentionPolicy.RUNTIME)
@interface FiledAnnotation{
String filedName();
String filedType();
int length();
}