1.ElasticSearch安装
注:最低要求JDK1.8
下载地址:官网:https://www.elastic.co
下载页面地址 :https://www.elastic.co/cn/downloads/elasticsearch
1.1 路径
1.2 目录了解
bin 启动文件
config 配置文件
log4j2 日志配置文件
jvm.options java虚拟机相关的配置
elasticsearch.yml elasticsearch默认配置文件 默认9200端口
lib 相关jar包
logs 日志
modules 功能模块
pligins 插件
2.安装ES可视化插件
1.下载地址 https://github.com/mobz/elasticsearch-head
2.启动
cnpm install
npm run start
3.连接有跨域问题,修改elasticsearch.yml
http.cors.enabled : true
http.cors.allow-origin: "*"
4.重启es服务器,再次连接
3.安装Kibana
下载地址:https://www.elastic.co/cn/downloads/kibana
Kibana的版本要与es的版本一致,解压之后就可以使用。
kibana-7.6.1-windows-x86_64\x-pack\plugins\translations\translations为中文包文件夹地址。修改中文页面时修改kibana.yml配置文件
4.ES核心概念
1.索引 -> 数据库
2.字段类型(mapping) -> 数据库字段
3.文档(documents) -> 一行数据
IK分词器
下载地址:https://github.com/medcl/elasticsearch-analysis-ik
下载后放入plugins目录下,重新启动即可
使用Kibana测试
- ik_smart :会将文本做最粗粒度的拆分
- ik_max_word:会将文本做最细粒度的拆分
CRUD
1.创建一个索引
PUT /索引名/类型名(后期不要->_doc)/文档id
{请求体}
指定字段类型,获取表信息
如果没有字段指定,es会自动配置默认字段。
GET _cat/health 看健康值
GET _cat/indices?v 看所有库
第一次修改效率不高,如果修改一个其他值就没有了。第二个效率高
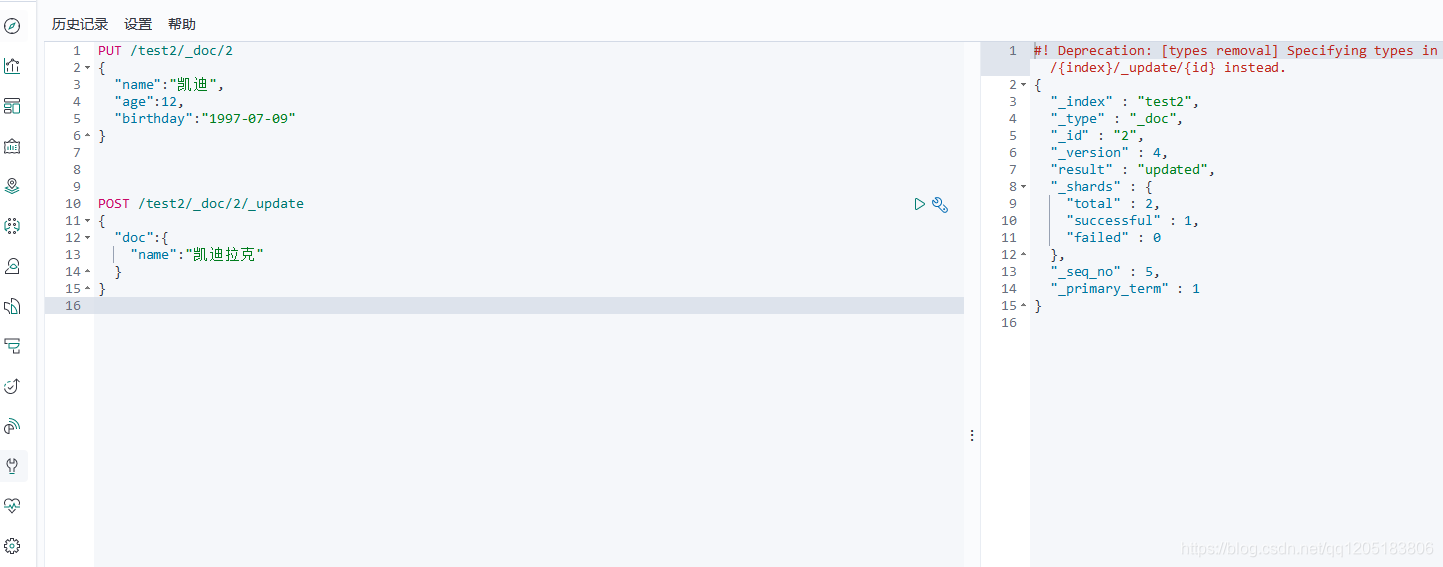 {
RestHighLevelClient client = new RestHighLevelClient(
RestClient.builder(
new HttpHost("localhost", 9200, "http")));
return client;
}
}
api代码参考
`package com.hskj;
import com.alibaba.fastjson.JSON;
import com.pojo.User;
import org.elasticsearch.action.admin.indices.delete.DeleteIndexRequest;
import org.elasticsearch.action.bulk.BulkRequest;
import org.elasticsearch.action.delete.DeleteRequest;
import org.elasticsearch.action.delete.DeleteResponse;
import org.elasticsearch.action.get.GetRequest;
import org.elasticsearch.action.get.GetResponse;
import org.elasticsearch.action.index.IndexRequest;
import org.elasticsearch.action.search.SearchRequest;
import org.elasticsearch.action.search.SearchResponse;
import org.elasticsearch.action.support.master.AcknowledgedResponse;
import org.elasticsearch.action.update.UpdateRequest;
import org.elasticsearch.action.update.UpdateResponse;
import org.elasticsearch.client.RequestOptions;
import org.elasticsearch.client.RestHighLevelClient;
import org.elasticsearch.client.indices.CreateIndexRequest;
import org.elasticsearch.client.indices.CreateIndexResponse;
import org.elasticsearch.client.indices.GetIndexRequest;
import org.elasticsearch.common.text.Text;
import org.elasticsearch.common.xcontent.XContentType;
import org.elasticsearch.index.query.MatchQueryBuilder;
import org.elasticsearch.index.query.QueryBuilders;
import org.elasticsearch.index.query.TermQueryBuilder;
import org.elasticsearch.search.SearchHit;
import org.elasticsearch.search.builder.SearchSourceBuilder;
import org.elasticsearch.search.fetch.subphase.highlight.HighlightBuilder;
import org.elasticsearch.search.fetch.subphase.highlight.HighlightField;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Map;
@SpringBootTest
class ElasticsearchApplicationTests {
@Autowired
private RestHighLevelClient restHighLevelClient;
@Test
void contextLoads() throws IOException {
//创建索引
CreateIndexRequest index = new CreateIndexRequest("owen_index2");
//客户端执行请求
CreateIndexResponse createIndexResponse = restHighLevelClient.indices().create(index, RequestOptions.DEFAULT);
System.out.println(createIndexResponse);
}
@Test
void GetIndexRequest() throws IOException {
//获取索引是否存在
GetIndexRequest index = new GetIndexRequest("owen_index");
//客户端执行请求
boolean exists = restHighLevelClient.indices().exists(index, RequestOptions.DEFAULT);
System.out.println(exists);
}
@Test
void DeleteIndexRequest() throws IOException {
//删除索引
DeleteIndexRequest index = new DeleteIndexRequest("owen_index2");
//客户端执行请求
AcknowledgedResponse delete = restHighLevelClient.indices().delete(index, RequestOptions.DEFAULT);
System.out.println(delete.isAcknowledged());
}
@Test
void addDocument() throws IOException {
//创建对象
User user = new User("欧文", 22);
//创建请求
IndexRequest request = new IndexRequest("owen_index");
request.id("1");
request.source(JSON.toJSONString(user), XContentType.JSON);
//客户端发送请求
restHighLevelClient.index(request, RequestOptions.DEFAULT);
}
//获取文档信息
@Test
void getDocument() throws IOException {
GetRequest request = new GetRequest("owen_index", "1");
//客户端发送请求
GetResponse documentFields = restHighLevelClient.get(request, RequestOptions.DEFAULT);
System.out.println(documentFields.getSourceAsString());
System.out.println(documentFields);
}
//修改文档信息
@Test
void updateDocument() throws IOException {
UpdateRequest request = new UpdateRequest("owen_index", "NYFmK3UBKXraCExfsVm6");
//创建对象
User user = new User("张无忌", 24);
request.doc(JSON.toJSONString(user), XContentType.JSON);
//客户端发送请求
UpdateResponse update = restHighLevelClient.update(request, RequestOptions.DEFAULT);
System.out.println(update.status());
}
//删除文档信息
@Test
void deleteDocument() throws IOException {
DeleteRequest request = new DeleteRequest("owen_index", "1");
//客户端发送请求
DeleteResponse update = restHighLevelClient.delete(request, RequestOptions.DEFAULT);
System.out.println(update.status());
}
//批量插入
@Test
void bulkDocument() throws IOException {
BulkRequest bulkRequest = new BulkRequest();
ArrayList<User> list = new ArrayList<>();
list.add(new User("张三", 3));
list.add(new User("李四", 3));
list.add(new User("王五", 3));
list.add(new User("赵六", 3));
list.add(new User("田七", 3));
for (User user : list) {
bulkRequest.add(
new IndexRequest("owen_index").source(JSON.toJSONString(user), XContentType.JSON)
);
}
restHighLevelClient.bulk(bulkRequest, RequestOptions.DEFAULT);
}
//查询文档信息
@Test
void searchDocument() throws IOException {
SearchRequest request = new SearchRequest("owen_index");
//构建搜索条件
SearchSourceBuilder builder = new SearchSourceBuilder();
//高亮显示
HighlightBuilder highlightBuilder = new HighlightBuilder();
highlightBuilder.field("name");
highlightBuilder.preTags("<p style='color='red''>");
highlightBuilder.postTags("</p>");
builder.highlighter(highlightBuilder);
//查询条件
//MatchQueryBuilder query = QueryBuilders.matchQuery("name", "张无忌");
MatchPhraseQueryBuilder query = QueryBuilders.matchPhraseQuery("name", "张无忌");
builder.query(query);
request.source(builder);
SearchResponse search = restHighLevelClient.search(request, RequestOptions.DEFAULT);
/* System.out.println(JSON.toJSONString(search.getHits()));
System.out.println(JSON.toJSONString(search.getHits().getHits()));
for (SearchHit hit : search.getHits().getHits()) {
System.out.println(hit.getSourceAsMap());
}*/
ArrayList<Map<String, Object>> list = new ArrayList<>();
for (SearchHit hit : search.getHits().getHits()) {
Map<String, HighlightField> highlightFields = hit.getHighlightFields();
HighlightField name = highlightFields.get("name");
Map<String, Object> sourceAsMap = hit.getSourceAsMap();
if (name != null) {
Text[] fragments = name.fragments();
String n_name = "";
for (Text fragment : fragments) {
n_name += fragment;
}
sourceAsMap.put("name", n_name);
}
list.add(sourceAsMap);
}
System.out.println(list);
}
}
`