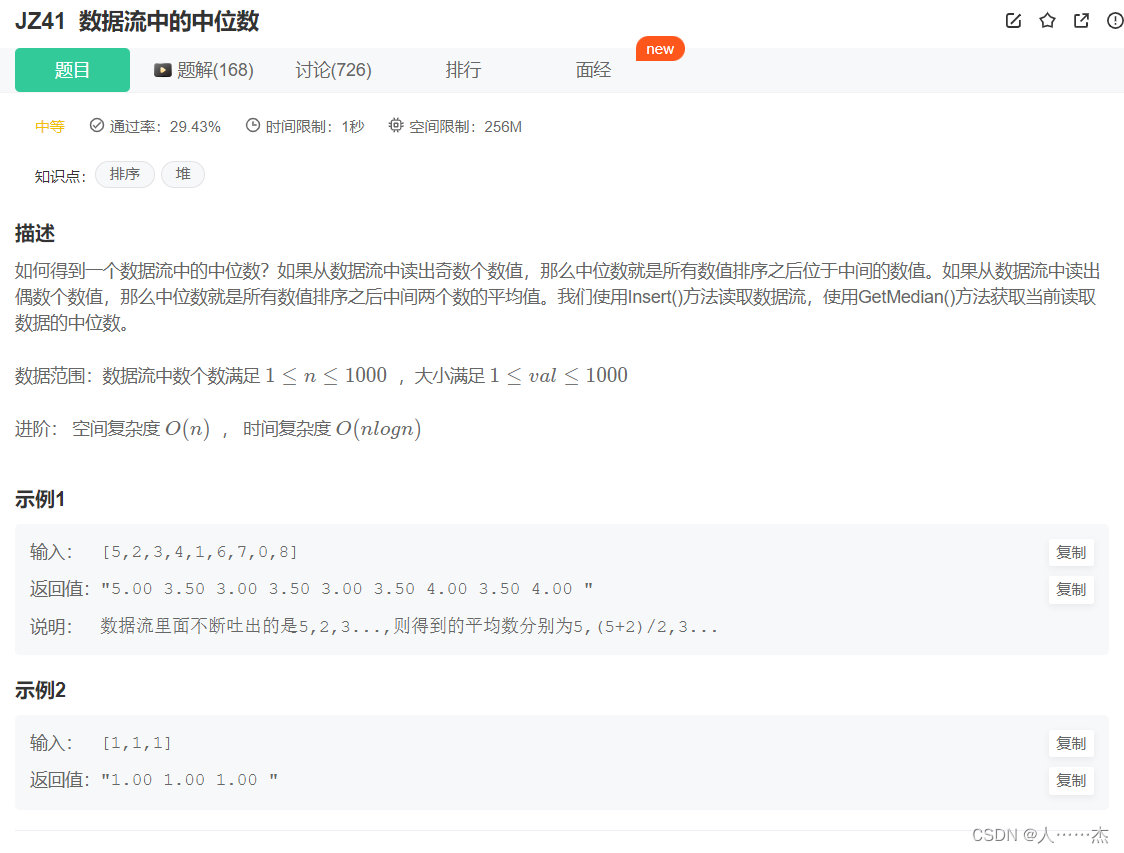
解题思路一:
import java.util.*;
public class Solution {
//用栈来存储所有数据
Stack<Integer> s = new Stack<Integer>();
//入栈
public void Insert(Integer num) {
s.push(num);
}
//出栈
public Double GetMedian() {
Double result = 0.0;
if(s.size() < 1){
return 0.0;
}
if(s.size() == 1){
return s.peek()*1.0;
}
Integer[] nums = new Integer[s.size()];
int index = 0;
//将数据写入nums中为排序做准备
while(!s.isEmpty()){
nums[index] = s.pop();
index++;
}
//s中数据已经空了,所以要重新写回来存储
for(Integer i : nums){
s.push(i);
}
Integer temp = null;
//对nums进行升序排序 冒泡排序
for(int i = 0 ; i < nums.length ;i++){
for(int j = 0 ; j < nums.length - i -1; j++){
if(nums[j] > nums[j + 1]){
temp = nums[j];
nums[j] = nums[j + 1];
nums[j + 1] = temp;
}
}
}
//flag为true 表示偶数 false表示奇数
Boolean flag = nums.length % 2 == 0 ? true : false;
//因为index是从0开始的,所以 -1
int sIndex = nums.length / 2 - 1;
if(flag){
//为偶数
result = (nums[sIndex] + nums[sIndex + 1])/2.0;
}else{
//为奇数
result = (nums[sIndex + 1])*1.0;
}
return result;
}
}
解题思路二:
import java.util.*;
public class Solution {
//用来存储数据
private List<Integer> lists = new ArrayList<Integer>();
//用来控制数组的大小
private Integer maxInt = 0;
public void Insert(Integer num) {
//用来获取最大数,即数组下标最大值
if(maxInt < num){
maxInt = num;
}
lists.add(num);
}
public Double GetMedian() {
//获取list长度
int size = lists.size();
//若list长度为1,则直接返回
if(size == 1){
return (double)lists.get(0);
}
//创建临时数组
int[] array = new int[maxInt + 1];
//将list中的数据存储到数组array中
int i = size - 1;
while(i >= 0){
array[lists.get(i)]++;
i--;
}
//获取中位数下标
int index = size/2;
//index-1位置的数字
int num1 = 0;
//index位置的数字
int num2 = 0;
int count = 0;
//结束标记:true继续查找 false结束
boolean flag = true;
for(int j = 0 ; j < array.length; j++){
//直接跳过
if(array[j] == 0){
continue;
}
//判断是否结束
if(!flag){
break;
}
while(array[j]!=0){
if(count == (index - 1)){
num1 = j;
}else if(count == index){
num2 = j;
//标志这两个数字都取到了,可以退出for循环
flag = false;
break;
}
count++;
array[j]--;
}
}
return size%2 == 0 ? (double)(num1 + num2)/2:num2;
}
}