#include <iostream>
using namespace std;
struct ListNode {
int data;
ListNode* next;
ListNode(int val):data(val), next(nullptr){}
};
class Solution {
public:
ListNode* reverseList(ListNode* head) {
if (head == nullptr || head->next == nullptr) {
return head;
}
ListNode* last = reverseList(head->next);
head->next->next = head;
head->next = nullptr;
return last;
}
};
int main()
{
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
Solution S;
ListNode* cur = S.reverseList(head);
while(cur) {
cout << cur->data << "->" << " ";
cur = cur->next;
}
cout << "NULL" << endl;
return 0;
}
C++链表反转
最新推荐文章于 2024-11-14 19:11:37 发布
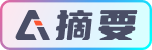