这里写目录标题
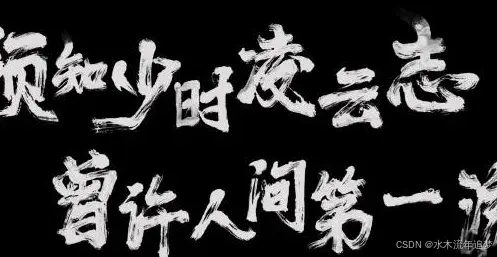
实践练习
- 设置Kubeflow Pipelines SDK
# 导入Kubeflow Pipelines SDK中的必要模块
from kfp import dsl, compiler
# 抑制来自Kubeflow Pipelines SDK的FutureWarning警告
import warnings
warnings.filterwarnings("ignore", category=FutureWarning, module='kfp.*')
这段脚本导入了dsl
和compiler
模块,并抑制了所有以kfp.
开头的模块产生的FutureWarning
警告。
- 定义一个简单的管道组件
from kfp import dsl
# 定义一个简单的组件来加两个数
@dsl.component
def add_numbers(num1: int, num2: int) -> int:
return num1 + num2
这个Python函数,使用@dsl.component
装饰器,定义了一个简单的Kubeflow Pipeline组件add_numbers
,它接受两个整数作为输入(num1
和num2
)并返回它们的和。
- 抑制特定警告
import warnings
warnings.filterwarnings("ignore", category=DeprecationWarning)
这段更新的脚本抑制了所有模块产生的DeprecationWarning
警告。
- 在管道中链接组件
from kfp import dsl
# 定义一个生成固定数的组件
@dsl.component
def generate_number() -> int:
return 42
# 定义一个加倍输入数的组件
@dsl.component
def double_number(input_number: int) -> int:
return input_number * 2
# 定义一个链接两个组件的管道
@dsl.pipeline(
name="加倍数字管道",
description="一个生成数字并加倍它的管道。"
)
def number_doubling_pipeline():
# 第一步:生成一个数字
generated_number_task = generate_number()
# 第二步:加倍生成的数字
double_number_task = double_number(input_number=generated_number_task.output)
这个管道由两个组件组成:generate_number
返回一个固定的整数,而double_number
接收一个整数输入并返回它的两倍。管道通过将generate_number
的输出传递给double_number
来演示组件的链接。
- 编译和准备管道以供执行
from kfp import compiler
# 假设管道定义名为`number_doubling_pipeline`
pipeline_func = number_doubling_pipeline
# 编译管道
compiler.Compiler().compile(
pipeline_func=pipeline_func,
package_path='number_doubling_pipeline.yaml'
)
这段脚本将number_doubling_pipeline
编译成一个名为number_doubling_pipeline.yaml
的YAML文件。编译后的管道可以上传到Kubeflow Pipelines环境以供执行。
- 处理
PipelineTask
对象
# 这是一个假设性的函数,不能直接执行。它是为了说明概念。
def handle_pipeline_task():
# 假设函数调用一个名为`my_component`的组件
# 在实际情况下,这应该是在管道函数内部
task = my_component(param1="value")
# 访问组件的输出
# 这一行是说明性的,通常用于在管道中传递组件的输出
output = task.output
print("访问了组件的输出:", output)
# 注意:在实际使用中,`my_component`应该定义为一个Kubeflow Pipeline组件
# 并且对任务的操作应该在管道函数的上下文中发生。
这个Python函数说明了调用一个Kubeflow Pipeline组件,返回一个PipelineTask
对象,然后通过task.output
访问其输出的概念。请注意,这是一个理论示例,实际实现需要在管道上下文中进行。
- 在管道定义中的错误处理
from kfp import dsl
# 错误的管道定义
@dsl.pipeline(
name='错误的管道',
description='一个试图直接返回`PipelineTask`对象的例子。'
)
def incorrect_pipeline_example():
@dsl.component
def generate_number() -> int:
return 42
generated_number_task = generate_number()
# 错误地试图直接返回`PipelineTask`对象
return generated_number_task # 这会导致错误
# 正确的管道定义
@dsl.pipeline(
name='正确的管道',
description='一个改正的例子,不试图直接返回`PipelineTask`对象。'
)
def correct_pipeline_example():
@dsl.component
def generate_number() -> int:
return 42
generated_number_task = generate_number()
# 正确的做法:不要直接从管道函数返回`PipelineTask`对象。
# 管道函数不需要直接返回任何数据。
# 解释:
# 在Kubeflow Pipelines中,管道函数组织组件间的数据流,但不直接返回数据。
# 直接从管道函数返回`PipelineTask`对象是不正确的,因为管道定义
# 应该描述组件的结构和依赖关系,而不是直接处理数据。
# 改正版本去掉了返回语句,符合管道函数的预期行为。
- 为模型训练自动化数据准备
import json
# 模拟为模型训练准备数据
def preprocess_data(input_file_path, output_file_path):
# 从JSON文件读取数据
with open(input_file_path, 'r') as infile:
data = json.load(infile)
# 执行简单的转换:过滤数据
# 作为示例,我们只想要那些“useful”字段为True的项
filtered_data = [item for item in data if item.get("useful", False)]
# 将转换后的数据保存到另一个JSON文件
with open(output_file_path, 'w') as outfile:
json.dump(filtered_data, outfile, indent=4)
# 示例使用
preprocess_data('input_data.json', 'processed_data.json')
# 注意:这个脚本假设当前目录下存在`input_data.json`文件
# 并将处理后的数据保存到`processed_data.json`。
# 在实际情况下,路径和转换逻辑应根据具体要求进行调整。
这个脚本演示了一个简单的数据准备流程,从JSON文件读取数据,执行转换(在这个例子中是基于条件的过滤),然后将处理后的数据保存到另一个JSON文件。这种任务可以在Kubeflow Pipeline组件中封装,用于自动化ML模型训练工作流中的数据准备步骤。
- 在管道中实现模型版本控制
from datetime import datetime
def generate_model_name(base_model_name: str) -> str:
# 生成一个格式为"YYYYMMDD-HHMMSS"的时间戳
timestamp = datetime.now().strftime("%Y%m%d-%H%M%S")
# 将时间戳附加到基础模型名称上以创建一个独特的模型名称
model_name = f"{base_model_name}-{timestamp}"
return model_name
# 示例使用
base_model_name = "my_model"
model_name = generate_model_name(base_model_name)
print("生成的模型名称:", model_name)
# 这个函数通过将当前日期和时间附加到基础模型名称上来生成一个独特的模型名称。
# 这种做法有助于模型版本控制,使得跟踪和管理不同版本的模型更加容易。
- 参数化并执行Kubeflow Pipeline
# 假设存在必要的导入和配置以与执行环境交互
def submit_pipeline_execution(compiled_pipeline_path: str, pipeline_arguments: dict):
# 占位符方法用于提交管道执行
# 在实际情况下,这将涉及到使用Kubeflow Pipelines SDK或云提供商的SDK
# 例如,使用Kubeflow Pipelines SDK或Google Cloud AI Platform Pipelines的服务
# 假设存在一个名为`submit_pipeline_job`的方法,可用于提交
# 这个方法将是执行环境的SDK或API的一部分
submit_pipeline_job(compiled_pipeline_path, pipeline_arguments)
# 示例管道参数
pipeline_arguments = {
"recipient_name": "Alice"
}
# 编译后的Kubeflow Pipeline YAML文件路径
compiled_pipeline_path = "path_to_compiled_pipeline.yaml"
# 提交管道执行
submit_pipeline_execution(compiled_pipeline_path, pipeline_arguments)
# 注意:这个示例假设存在一个名为`submit_pipeline_job`的方法
# 它将是执行环境API或SDK的一部分。在实际实现中,您将替换这个占位符
# 以与Kubeflow Pipelines API或托管服务如Google Cloud AI Platform交互。
这段脚本概述了如何参数化并提交一个编译好的Kubeflow Pipeline以供执行,假设存在一个适合的API或SDK方法(在这个假设示例中为submit_pipeline_job
)。实际提交作业的方法取决于您的执行环境或云服务提供商的具体情况。