文章目录
【一】 Stack(堆栈)- First In Last Out
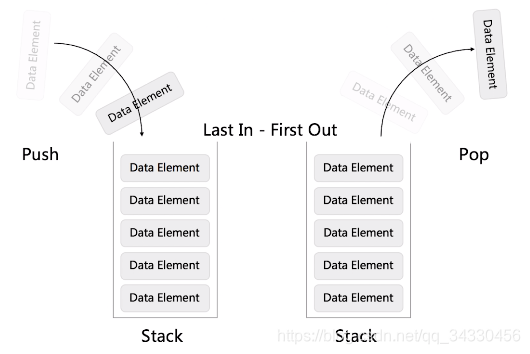
【二】 Queue(队列)- First In First Out

【三】 Time Complexity(时间复杂度)
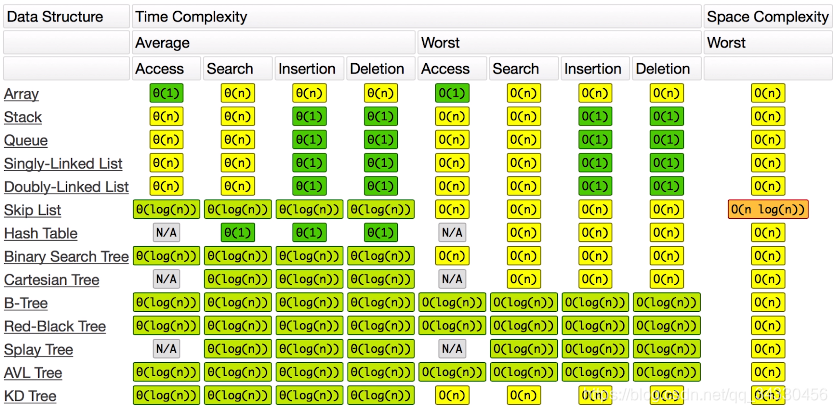
【四】 Interview(面试题)
【4.1】 LeetCode 20:Valid Parentheses(验证括号)
# Python(堆栈)
class Solution:
def isValid(self, s: str) -> bool:
# 数组结构,用于做栈
stack = []
# 字典结构
paren_map = {')':'(', ']':'[', '}':'{'}
for c in s:
if c not in paren_map:
stack.append(c)
elif not stack or paren_map[c] != stack.pop():
return False
return not stack
// c++(堆栈)
class Solution {
public:
bool isValid(string s) {
stack<char> parentheses;
for (int i = 0; i < s.size(); i++) {
if (s[i] == '(' || s[i] == '[' || s[i] == '{'){
parentheses.push(s[i]); // 压栈
} else {
if (parentheses.empty()) return false; // 一开始需要判空
if (s[i] == ')' && parentheses.top() != '(') return false;
if (s[i] == ']' && parentheses.top() != '[') return false;
if (s[i] == '}' && parentheses.top() != '{') return false;
parentheses.pop(); // 弹出栈顶元素
}
}
return parentheses.empty(); // 若都匹配,则栈为空
}
};
【4.2】 LeetCode 232:Implement Queue using Stacks(用栈来实现队列)
// c++(用两个栈)
class MyQueue {
public:
/** Initialize your data structure here. */
MyQueue() {
// 构造函数
}
/** Push element x to the back of queue. */
void push(int x) {
_new.push(x);
}
/** Removes the element from in front of queue and returns that element. */
int pop() {
shiftStack(); // 重点
int val = _old.top();
_old.pop();
return val;
}
/** Get the front element. */
int peek() {
shiftStack(); // 重点
return _old.top();
}
/** Returns whether the queue is empty. */
bool empty() {
return _new.empty() && _old.empty();
}
// 重点 判断 _old 是否为空,若为空则直接返回,否则将 _new 中的元素压入 _old
void shiftStack() {
if(!_old.empty()) return;
while(!_new.empty()) {
_old.push(_new.top());
_new.pop();
}
}
private:
stack<int> _old, _new; // 定义两个栈
};
【4.3】 LeetCode 225:Implement Stacks using Queue(用队列来实现栈)
// c++(用一个队列,对 push 进行倒转即可)
class MyStack {
public:
/** Initialize your data structure here. */
MyStack() {
// 构造函数
}
/** Push element x onto stack. */
void push(int x) {
q.push(x);
// 将队列倒转,之后的操作即按队列的操作即可
for (int i = 0; i < (int)q.size() - 1; i++) {
q.push(q.front());
q.pop();
}
}
/** Removes the element on top of the stack and returns that element. */
int pop() {
int x = q.front();
q.pop();
return x;
}
/** Get the top element. */
int top() {
return q.front();
}
/** Returns whether the stack is empty. */
bool empty() {
return q.empty();
}
private:
queue<int> q; // 定义一个队列
};