Boring Class
Time Limit: 6000/3000 MS (Java/Others) Memory Limit: 65536/65536 K (Java/Others)Total Submission(s): 1824 Accepted Submission(s): 575
Problem Description
Mr. Zstu and Mr. Hdu are taking a boring class , Mr. Zstu comes up with a problem to kill time, Mr. Hdu thinks it’s too easy, he solved it very quickly, what about you guys?
Here is the problem:
Give you two sequences L1,L2,...,Ln and R1,R2,...,Rn.
Your task is to find a longest subsequence v1,v2,...vm satisfies
v1≥1, vm≤n, vi<vi+1 .(for i from 1 to m - 1)
Lvi≥Lvi+1, Rvi≤Rvi+1(for i from 1 to m - 1)
If there are many longest subsequence satisfy the condition, output the sequence which has the smallest lexicographic order.
Here is the problem:
Give you two sequences L1,L2,...,Ln and R1,R2,...,Rn.
Your task is to find a longest subsequence v1,v2,...vm satisfies
v1≥1, vm≤n, vi<vi+1 .(for i from 1 to m - 1)
Lvi≥Lvi+1, Rvi≤Rvi+1(for i from 1 to m - 1)
If there are many longest subsequence satisfy the condition, output the sequence which has the smallest lexicographic order.
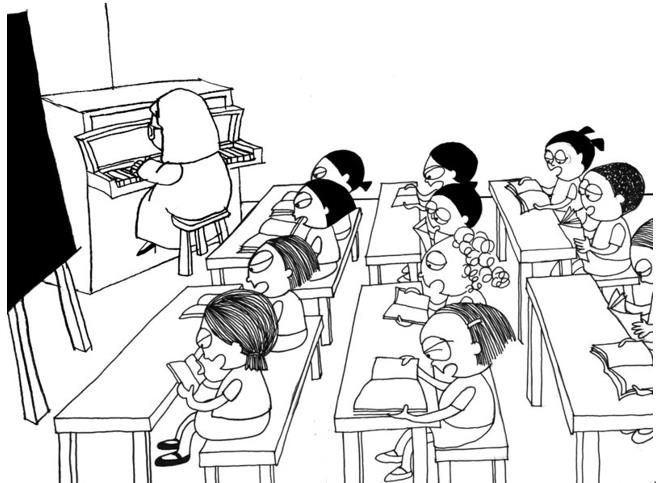
Input
There are several test cases, each test case begins with an integer n.
1≤n≤50000
Both of the following two lines contain n integers describe the two sequences.
1≤Li,Ri≤109
1≤n≤50000
Both of the following two lines contain n integers describe the two sequences.
1≤Li,Ri≤109
Output
For each test case ,output the an integer m indicates the length of the longest subsequence as described.
Output m integers in the next line.
Output m integers in the next line.
Sample Input
5 5 4 3 2 1 6 7 8 9 10 2 1 2 3 4
Sample Output
5 1 2 3 4 5 1 1
题意:
给你两个序列,序列内元素个数都为n个,问你找到最长的子序列,使得子序列内的数v1v
vi<vi+1 .(for i from 1 to m - 1)
Lvi≥Lvi+1,Rvi≤Rvi+1(for i from 1 to m - 1) ,换个意思就是让你找最多的点使得(v1,l1,r1) ,(v2,l2,r2) ,v1<v2,l1>=l2,r1<=r2
解析:
如果是
光找满足条件的点的数量,那么我们直接CDQ分治就可以做出来了,但这里要找最长的子序列,那么我们就需要用到动态规划 l为i时,这个点到最右边的最长子序列长度dp[i]=max{dp[i],query(l)+1},寻找在当前分治的区间内,比l小,但v,r比他大的节点当中最大的序列长度,然后将其加上1(表示这个点),这个就是分治里面嵌套的DP。并且这里要输出最小字典序,所以就需要倒着搜,因为如果你正着搜的话,右边区间的值永远是由离他最近左区间的值影响的,即这个序列的值一直是往右边靠的,这个显然和题目不符合,所以需要逆着搜,由右边影响左边,左边区间的值由离他最近的右区间影响,这个序列的值就会往左靠,这样就满足最小字典序的条件了
!!这里注意因为要把树状数组和记录左右两个序列的值的数组开成两倍
#include <bits/stdc++.h>
using namespace std;
const int MAXN =5e4+100;
typedef struct node
{
int L;
int R;
int pos;
}node;
typedef struct nno
{
int L;
int R;
int pos;
nno(int l=0,int r=0,int p=0):L(l),R(r),pos(p){}
}nno;
node a[MAXN];
nno pp1[MAXN],pp2[MAXN];
int num[MAXN*2],cnt,tot; //!
int dp[MAXN];
int res[MAXN];
int bit[MAXN*2],n;//!
inline int lowbit(int x)
{
return x&(-x);
}
bool cmp(nno a,nno b)
{
if(a.R!=b.R) return a.R<b.R;
else if(a.L!=b.L) return a.L>b.L;
else return a.pos<b.pos;
}
bool cmp2(node a,node b)
{
return a.pos<b.pos;
}
void add(int c,int v) //bit[i]的L在i表示的区间内范围的这些点的最大序列长度(这个点的L在i表示的区间范围内)
{
for(int i=c;i<tot+10;i+=lowbit(i))
bit[i]=max(bit[i],v);
}
int query(int c)
{
int ans=-1;
for(int i=c;i>0;i-=lowbit(i))
ans=max(bit[i],ans);
return ans;
}
void delet(int x)
{
for(int i=x;i<tot+10;i+=lowbit(i))
{
bit[i]=0;
}
}
void CDQ(int l,int r) //[l,r]
{
if(l==r)
{
//dp[a[l].pos]=max(dp[a[l].pos],1);
return;
}
int mid=(l+r)>>1;
CDQ(mid+1,r);
for(int i=l;i<=mid;i++) //因为CDQ(l,mid);之后还要往左区间搜,所以不能打乱原来按照v(下标)从小到大的顺序
{
pp1[i]=nno(a[i].L,a[i].R,a[i].pos);
}
for(int i=mid+1;i<=r;i++)
{
pp2[i]=nno(a[i].L,a[i].R,a[i].pos);
}
sort(pp1+l,pp1+mid+1,cmp);
sort(pp2+mid+1,pp2+r+1,cmp);
for(int i=mid,j=r;i>=l;i--)
{
while(j>mid&&pp1[i].R<=pp2[j].R)
{
add(pp2[j].L,dp[pp2[j].pos]);
j--;
}
dp[pp1[i].pos]=max(dp[pp1[i].pos],query(pp1[i].L)+1);
}
for(int i=mid+1;i<=r;i++) delet(pp2[i].L);
CDQ(l,mid);
}
int main()
{
while(scanf("%d",&n)!=EOF)
{
cnt=0;
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].L);
a[i].pos=i;
num[cnt++]=a[i].L;
}
for(int i=1;i<=n;i++)
{
scanf("%d",&a[i].R);
num[cnt++]=a[i].R;
}
sort(num,num+cnt);
tot=unique(num,num+cnt)-num; //去重
for(int i=1;i<=n;i++) //把这些点缩小,1 100 1100 2000这样就可以化成1 2 3 4,效果是一样的,因为L,R可以达到1e9,树状数组时开不了这么大的
{
a[i].L=lower_bound(num,num+tot,a[i].L)-num+1;
a[i].R=lower_bound(num,num+tot,a[i].R)-num+1;
}
for(int i=1;i<=n;i++) dp[i]=1;
CDQ(1,n);
//sort(a+1,a+n+1,cmp2);
int ans=-1;
for(int i=1;i<=n;i++)
ans=max(ans,dp[i]);
printf("%d\n",ans);
int pre=-1;
int pnt=0;
for(int i=1;i<=n;i++)
{
if(dp[i]==ans&&(pre==-1||(a[i].L<=a[pre].L&&a[i].R>=a[pre].R)))
{
ans--;
pre=i;
res[pnt++]=i;
}
}
for(int i=0;i<pnt;i++)
{
if(i==0) printf("%d",res[i]);
else printf(" %d",res[i]);
}
printf("\n");
}
return 0;
}