轮播图
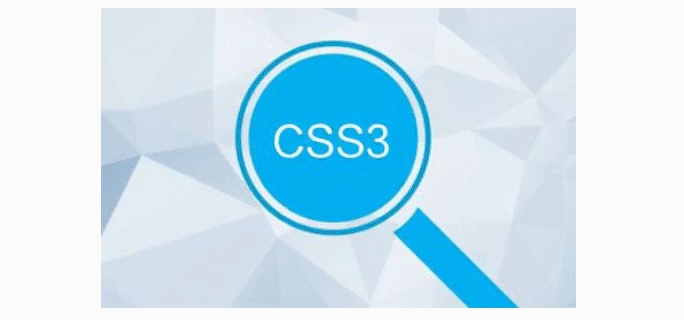
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>advertise</title>
</head>
<body>
<div class="view-port">
<div class="lay-out">
<div class="common item1">
<img src="IMG/CSS.jpg">
</div>
<div class="common item2">
<img src="IMG/HTML.jpg">
</div>
<div class="common item3">
<img src="IMG/JS.jpg">
</div>
<div class="common item4">
<img src="IMG/Vue.jpg">
</div>
<div class="common item5">
<img src="IMG/Node.jpg">
</div>
</div>
</div>
</body>
<style type="text/css">
:root{
--item-height:240px;
--item-width:380px;
}
.view-port{
height: var(--item-height);
width: var(--item-width);
margin: 0 auto;
overflow: hidden;
position: relative;
}
.lay-out{
display: flex;
position: absolute;
justify-content: space-evenly;
align-items: center;
height: var(--item-height);
width:calc(var(--item-width)*5);
background-color: #9dba64;
left: 0;
transition: left ease-out 0.3s;
}
.common {
height: 240px;
width: 380px;
overflow: hidden;
display: flex;
align-items: center;
justify-content: center;
background-color: darkblue;
}
.common img{
height: 100%;
}
</style>
<script type="text/javascript">
let index = 1;
const longItem = document.querySelector(".lay-out")
const longItemWidth = longItem.offsetWidth
const childrenNum = longItem.children.length;
const itemWidth = longItemWidth/childrenNum
console.log(itemWidth);
setInterval(next,3000)
function next() {
longItem.style.left = index * (-itemWidth) +"px"
if(index>=4){
index = 0;
}else {
index ++
}
}
</script>
</html>
省市区三级联动
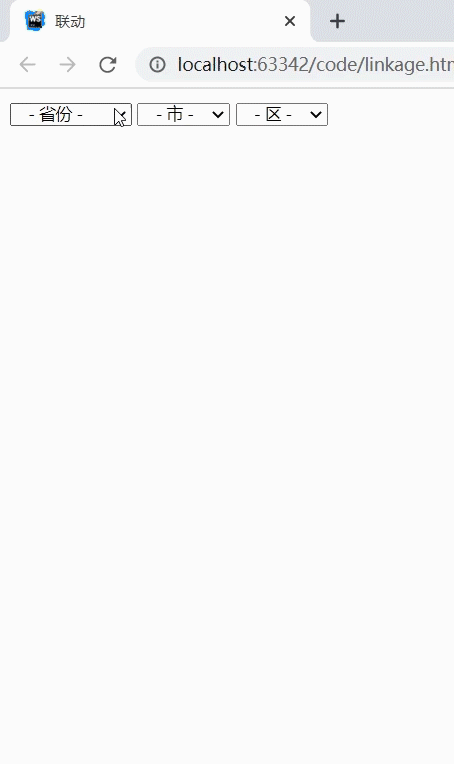
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>联动</title>
</head>
<body>
<div class="layout">
<select class="province common" onchange="handleSelectProvince(event)">
<option value="default">- 省份 -</option>
</select>
<select class="city common" onchange="handleSelectCity(event)">
<option value="default">- 市 -</option>
</select>
<select class="block common" >
<option value="default">- 区 -</option>
</select>
</div>
</body>
<style type="text/css">
.common{
padding: 0 10px;
}
</style>
<script src="cityData.js">
</script>
<script type="text/javascript">
console.log(province)
let provinceList = province.map(item => item.name)
console.log(provinceList);
let provinceNode = document.querySelector('.province')
let cityNode = document.querySelector('.city')
let blockNode = document.querySelector('.block')
let provinceFlag = null;
function init() {
for (let i=0;i<provinceList.length;i++){
let node = document.createElement('option');
node.value = provinceList[i];
let text = document.createTextNode(provinceList[i])
node.appendChild(text)
provinceNode.appendChild(node)
}
}
function handleSelectProvince(event) {
if (event.target.value=="default"){
return
}
cityNode.innerHTML = "<option>- 市 -</option>"
blockNode.innerHTML = "<option>- 区 -</option>"
const value = event.target.value
let [{city}] = province.filter(item => item.name==value)
for (let i=0;i<city.length;i++){
let node = document.createElement('option');
node.value = city[i].name
let text = document.createTextNode(city[i].name)
node.appendChild(text)
cityNode.appendChild(node)
}
provinceFlag = event.target.value
}
function handleSelectCity(event) {
if (event.target.value=="default"){
return
}
blockNode.innerHTML = "<option>- 区 -</option>"
const value = event.target.vaAlue
let targetProvince = null;
for (let i =0 ;i<province.length;i++){
if (province[i].name == provinceFlag){
targetProvince = province[i].city
}
}
targetProvince.filter(item => item.name == value)
let [{districtAndCounty}] = targetProvince.filter(item => item.name == value)
console.log(districtAndCounty)
for (let i=0;i<districtAndCounty.length;i++){
let node = document.createElement('option');
node.value = districtAndCounty[i]
let text = document.createTextNode(districtAndCounty[i])
node.appendChild(text)
blockNode.appendChild(node)
}
}
init()
</script>
</html>
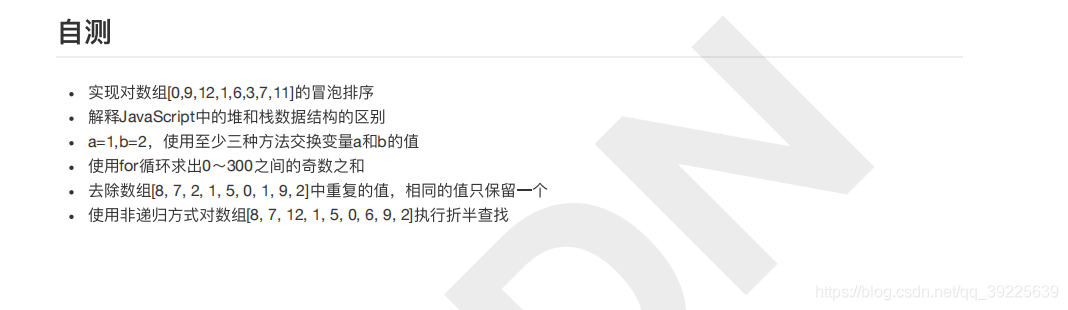
let arr = [0,9,12,1,66,3,7,11]
for (let i = 0; i<arr.length;i++){
for (let j = 0;j<arr.length-i-1;j++){
let tem = null;
if (arr[j]>arr[j+1]){
tem=arr[j+1]
arr[j+1] = arr[j]
arr[j] = tem
}
}
}
console.log(arr)
let a1 = 1;
let b1 = 2;
[a1,b1] = [b1 , a1];
console.log(a1,b1);
let c = 1;
let d = 2;
let tem = c;
c = d;
d = tem;
console.log(c,d);
let e = 1;
let f = 2;
e = e^f;
f = e^f
e = e^f
console.log(e,f);
let counter = 0;
for (let i = 0;i<=300;i++){
if (i%2!=0){
counter+=i
}
}
console.log(counter)
let repetitionArr = [8,7,2,1,5,0,1,9,2]
let newArr = []
for (let i = 0;i<repetitionArr.length;i++){
if (newArr.length ==0){
newArr.push(repetitionArr[i])
continue
}
for (let j = 0;j<newArr.length;j++){
if (newArr[j]==repetitionArr[i]){
break;
}else if (j==newArr.length-1){
newArr.push(repetitionArr[i])
}
}
}
console.log(newArr)
let searchArr = [8,7,12,1,5,0,6,9,2];
function search(target,arr) {
let sortArr = arr.sort(function (a,b) {
return a-b
})
let low = 0;
let high = sortArr.length
while (low<=high){
let mid = Math.floor((low+high)/2)
if (target == sortArr[mid]){
return mid
}else if (target<sortArr[mid]){
high = mid-1
}else {
low = mid+1
}
}
return 0
}
console.log('结果:',search(5, searchArr));